I will show you how to execute code in JavaScript after the text has finished loading.
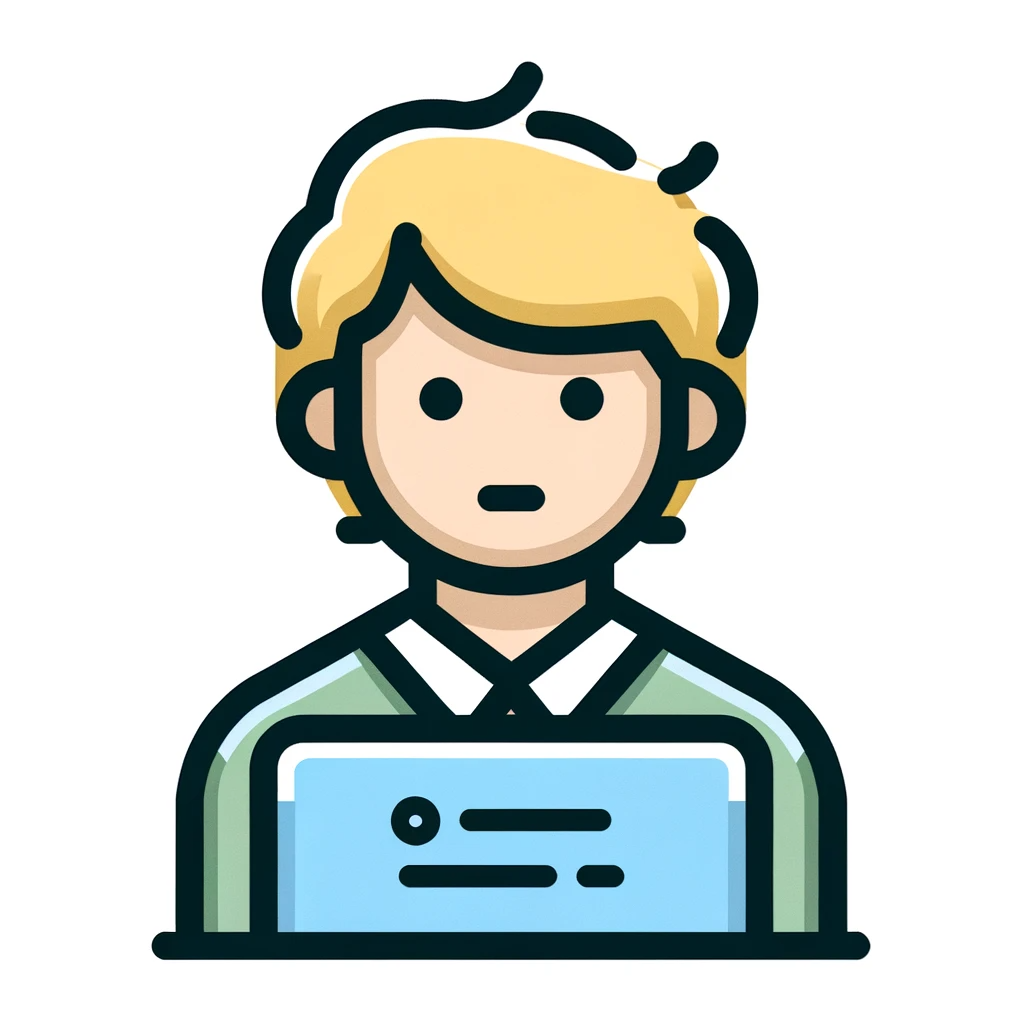
When writing code in JavaScript, I would like to know how to execute it after all the text on the page has been loaded, but what should I do?
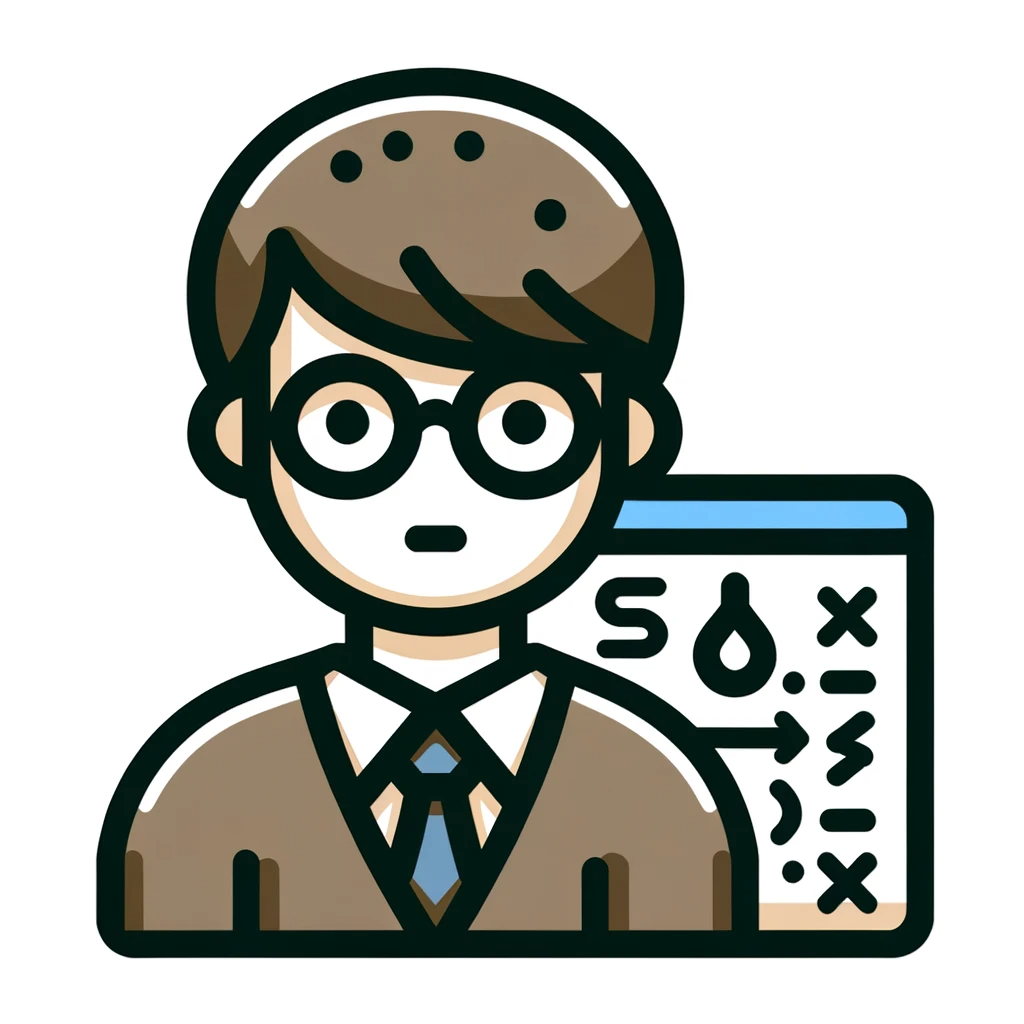
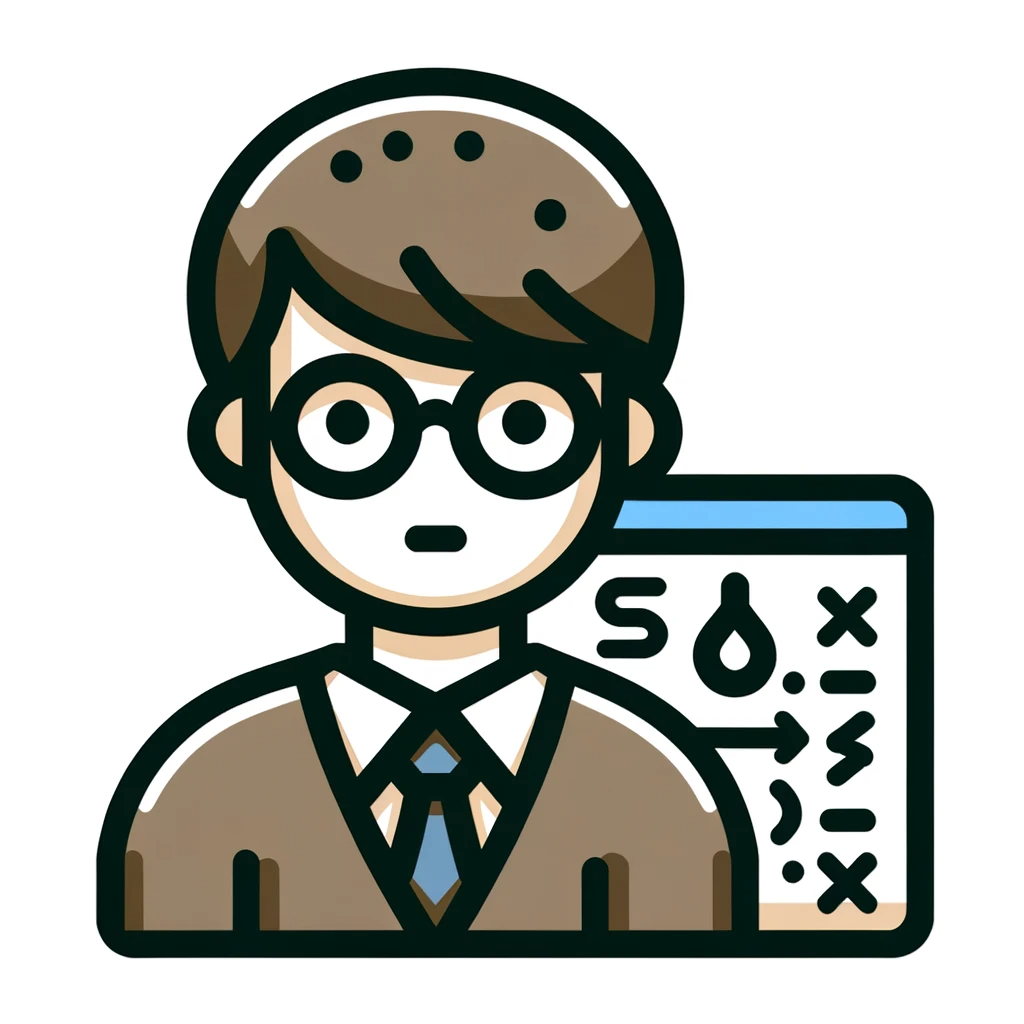
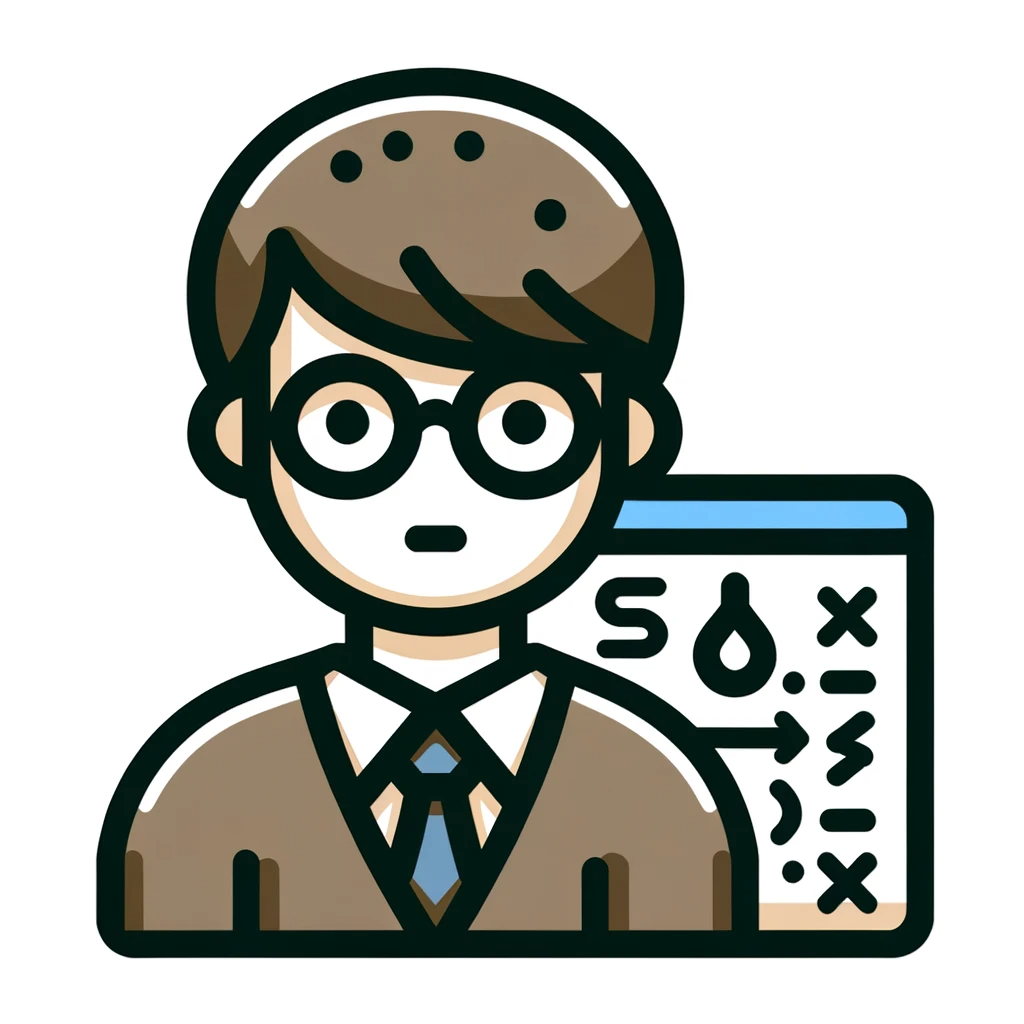
There are two ways to execute JavaScript code after all the text on the page has been loaded: using the DOMContentLoaded event or using the window.onload method.
How to implement using DOMContentLoaded event
The DOMContentLoaded event is an event that occurs after the page’s DOM tree is built. This means that the event will fire when all the HTML has been parsed and the DOM tree has been built.
The DOMContentLoaded event fires without waiting for all images and stylesheets to load, making the page faster. This is because the screen can be displayed earlier without delaying the execution of the JavaScript code.
To use the DOMContentLoaded event, write as follows:
document.addEventListener('DOMContentLoaded', function() {
// When the DOMContentLoaded event occurs, write the code here
});
Thus, for the document object, define the DOMContentLoaded event using the addEventListener method and write the code inside it.
Sample program using DOMContentLoaded event
Below is a sample program using the DOMContentLoaded event.
<!DOCTYPE html>
<html>
<head>
<title>Sample of DOMContentLoaded event</title>
<script>
document.addEventListener('DOMContentLoaded', function() {
// When the DOMContentLoaded event occurs, write the code here
var titleElement = document.getElementById('title');
titleElement.innerHTML = 'Hello, JavaScript!';
});
</script>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<p>This is a sample program in JavaScript.</p>
</body>
</html>
The purpose of this program is to rewrite the content of the HTML h1 element using JavaScript. When the DOMContentLoaded event occurs, the content of the h1 element is rewritten to “Hello, JavaScript!”.
To define the DOMContentLoaded event, use the addEventListener method and specify “DOMContentLoaded” in the first argument and the function to be executed when the event occurs in the second argument.
Inside this function, I use the document object to access the DOM element and use the innerHTML property to rewrite the element’s content.
Implementation method using window.onload method
The window.onload method is an event that occurs after all of the page’s resources, such as HTML elements and external files, have been loaded. This means that you can run your code after images, stylesheets, etc. have finished loading.
To use the window.onload method, write as follows.
window.onload = function() {
// When window.onload occurs, write code here
};
In this way, by assigning a function to the onload property of the window object, define the window.onload event and write the code inside it.
Note that the window.onload event waits until all resources are loaded, so it may take some time for the page to finish displaying. Therefore, it can be used in conjunction with the DOMContentLoaded event to improve page display speed.
Sample program using window.onload method
Below is a sample code using window.onload method.
<!DOCTYPE html>
<html>
<head>
<title>Sample of window.onload method</title>
<script>
window.onload = function() {
// When window.onload occurs, write code here
var titleElement = document.getElementById('title');
titleElement.innerHTML = 'Hello, JavaScript!';
};
</script>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<p>This is a sample program in JavaScript.</p>
</body>
</html>
The purpose of this program is to rewrite the content of the HTML h1 element using JavaScript. When the window.onload event occurs, the content of the h1 element is rewritten to “Hello, JavaScript!”.
You can define the window.onload event by assigning a function to the onload property of the window object.
Inside this function, I use the document object to access the DOM element and use the innerHTML property to rewrite the element’s content.
Difference between DOMContentLoaded and window.onload
DOMContentLoaded and window.onload are both JavaScript events that detect when a page has finished loading, but there are differences in their behavior.
The DOMContentLoaded event occurs when the HTML document has finished loading. In other words, the event fires when all HTML elements are loaded. On the other hand, the window.onload event occurs after all visible elements on the page (including external files such as HTML, CSS, and JavaScript) have been loaded.
This means that the DOMContentLoaded event can execute JavaScript code before the page has finished rendering. The window.onload event, on the other hand, allows you to execute JavaScript code after the page has finished displaying. Also, the window.onload event may be slower than the DOMContentLoaded event because it waits until all resources have finished loading.
Given these differences, the DOMContentLoaded event is suitable for executing JavaScript code that accesses and manipulates DOM elements. On the other hand, the window.onload event is suitable for running code that needs to be executed after an image or external script has finished loading.
Sample code combining DOMContentLoaded and window.onload events
Below is a sample code that combines the DOMContentLoaded and window.onload events.
<!DOCTYPE html>
<html>
<head>
<title>Sample of DOMContentLoaded and window.onload</title>
<script>
document.addEventListener("DOMContentLoaded", function(event) {
// If DOMContentLoaded occurs, write code here
var titleElement = document.getElementById('title');
titleElement.innerHTML = 'Hello, JavaScript!';
});
window.onload = function() {
// When window.onload occurs, write code here
var messageElement = document.getElementById('message');
messageElement.innerHTML = 'All resources have been loaded.';
};
</script>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<p>This is a sample program in JavaScript.</p>
<p id="message"></p>
</body>
</html>
This program uses the DOMContentLoaded and window.onload events to execute JavaScript code at different times.
The purpose is to rewrite the content of the HTML h1 element using JavaScript when the DOMContentLoaded event occurs.
Also, when the window.onload event occurs, a message is displayed indicating that all elements displayed on the page (including external files such as HTML, CSS, and JavaScript) have been loaded.
You can define the DOMContentLoaded event by using the addEventListener method on the document object and passing a function.
You can also define the window.onload event by assigning a function to the onload property of the window object.
summary
We explained how to execute code after the text has finished loading.
- The DOMContentLoaded event occurs when the DOM is constructed, even if external resources such as images and stylesheets have not finished loading.
- The window.onload event occurs after all external resources have finished loading and all elements of the page are available.
- By using both events, you can provide a smoother experience for your users.
Which method to adopt should be selected appropriately depending on the situation. You can also combine DOMContentLoaded and window.onload. For example, you can use DOMContentLoaded to perform the minimum necessary processing and window.onload to perform heavier processing.
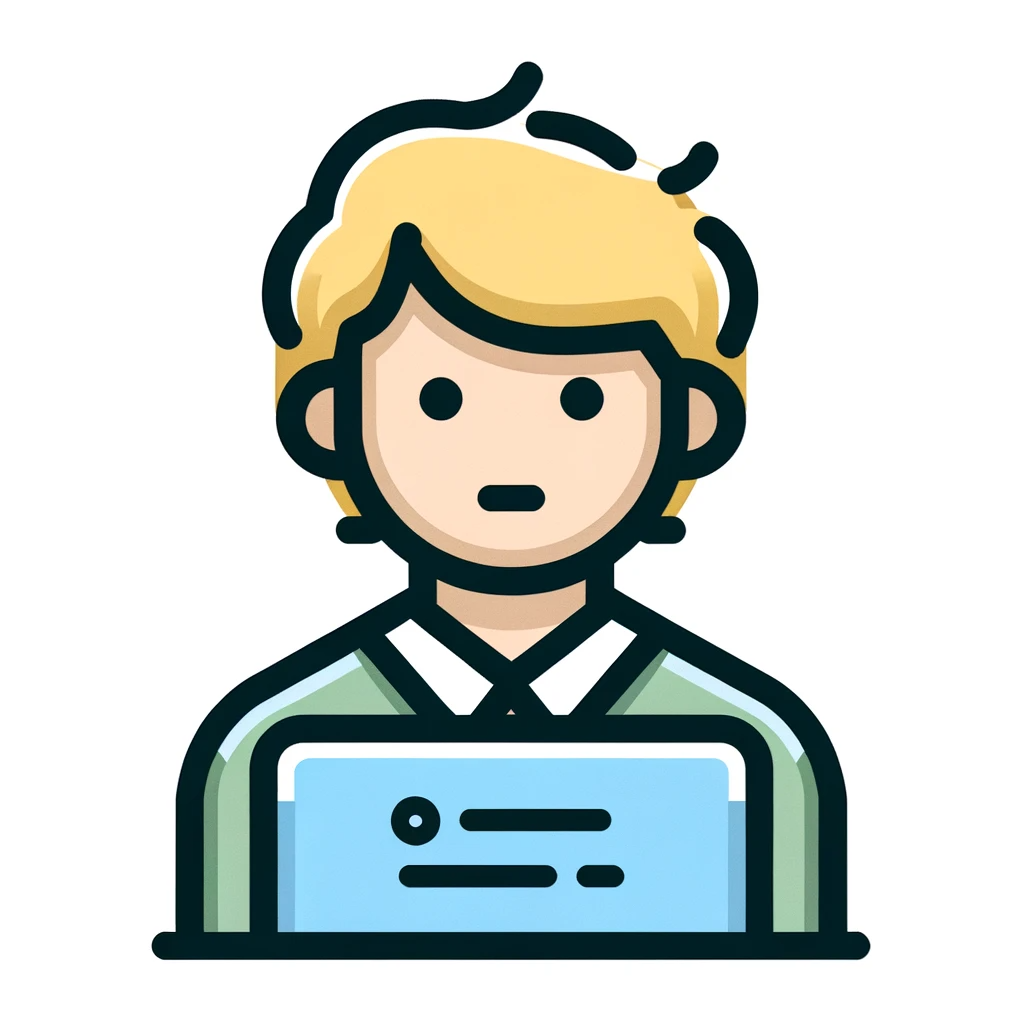
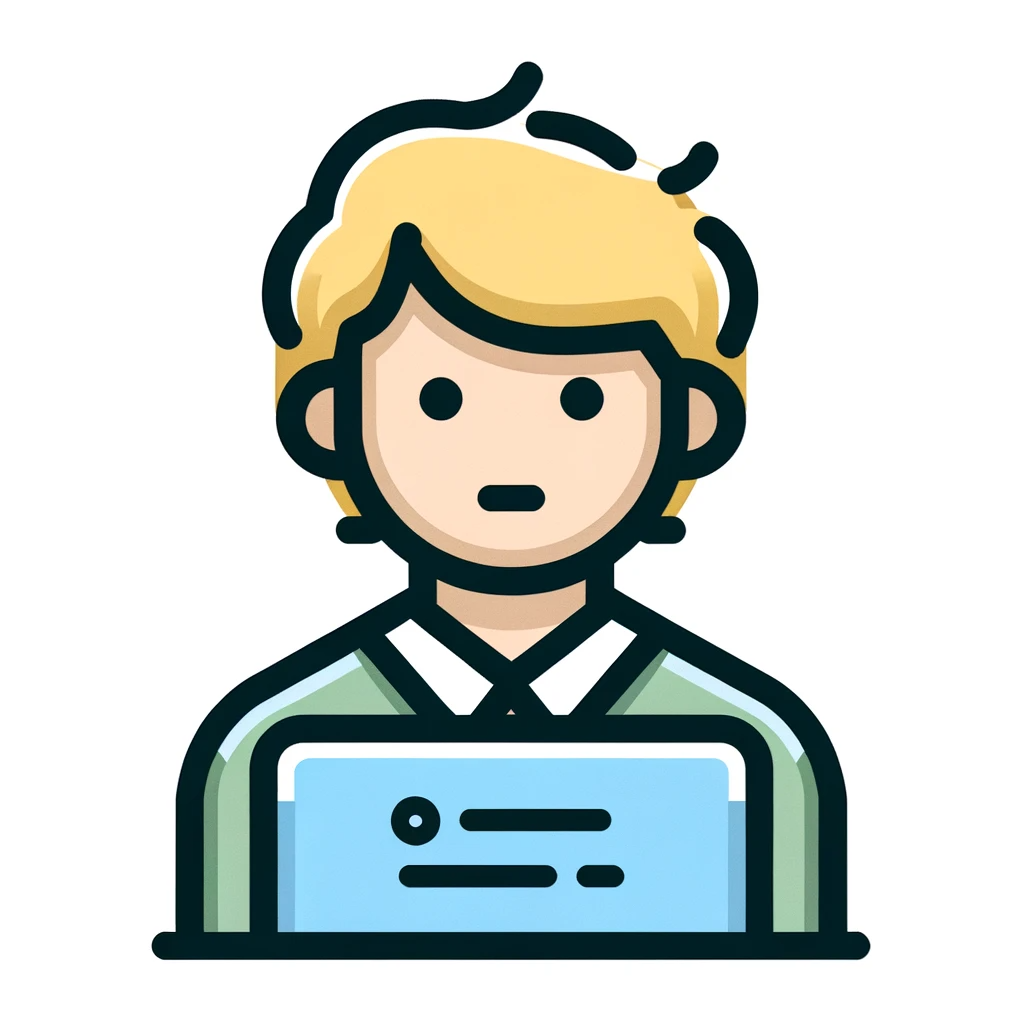
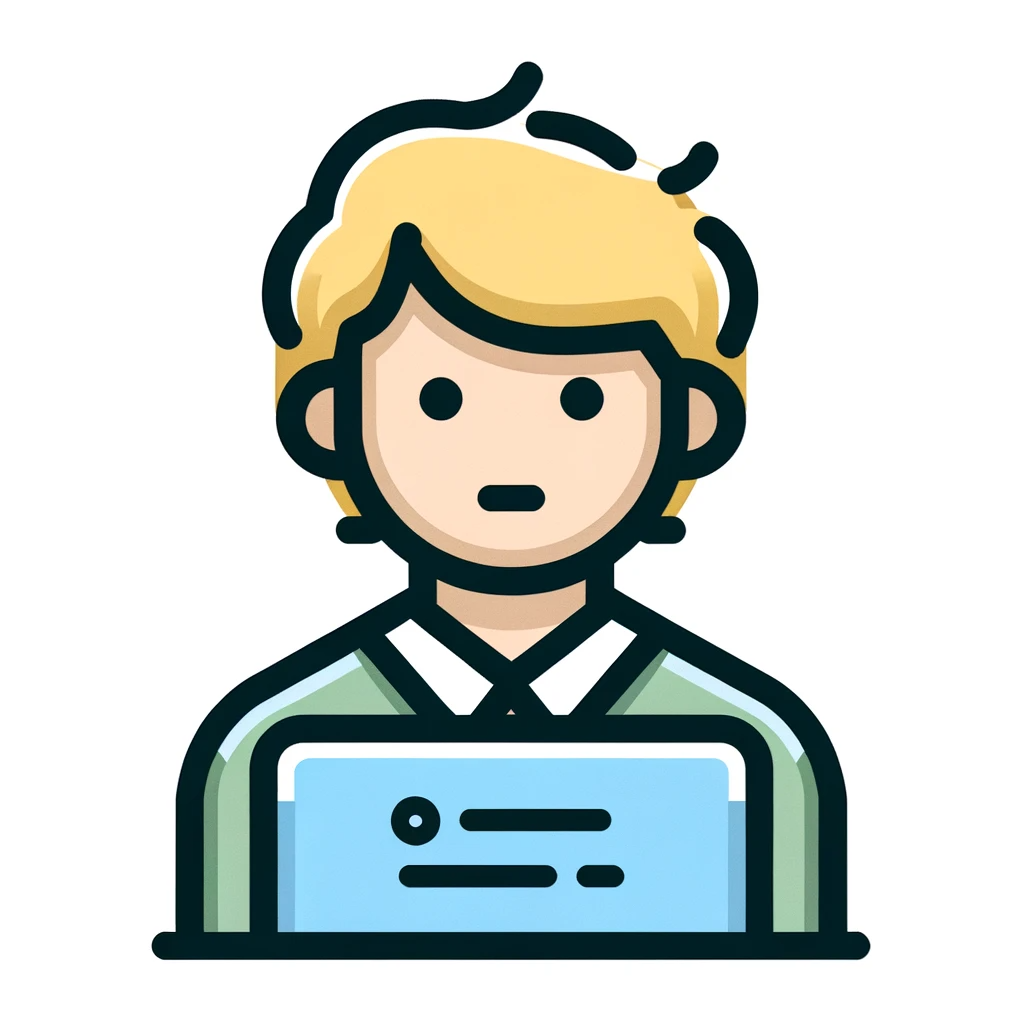
By combining the DOMContentLoaded event and the window.onload event, you can optimize page speed and provide a better experience for your users!
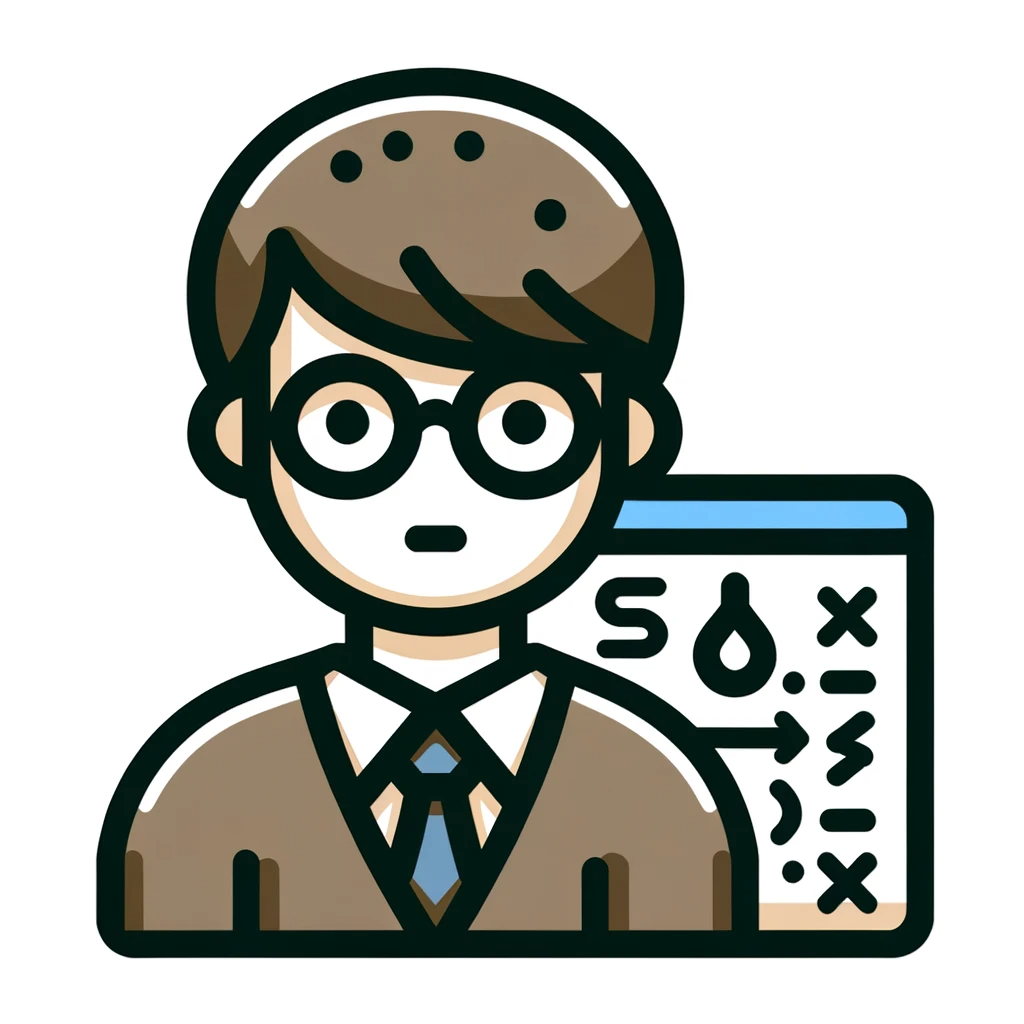
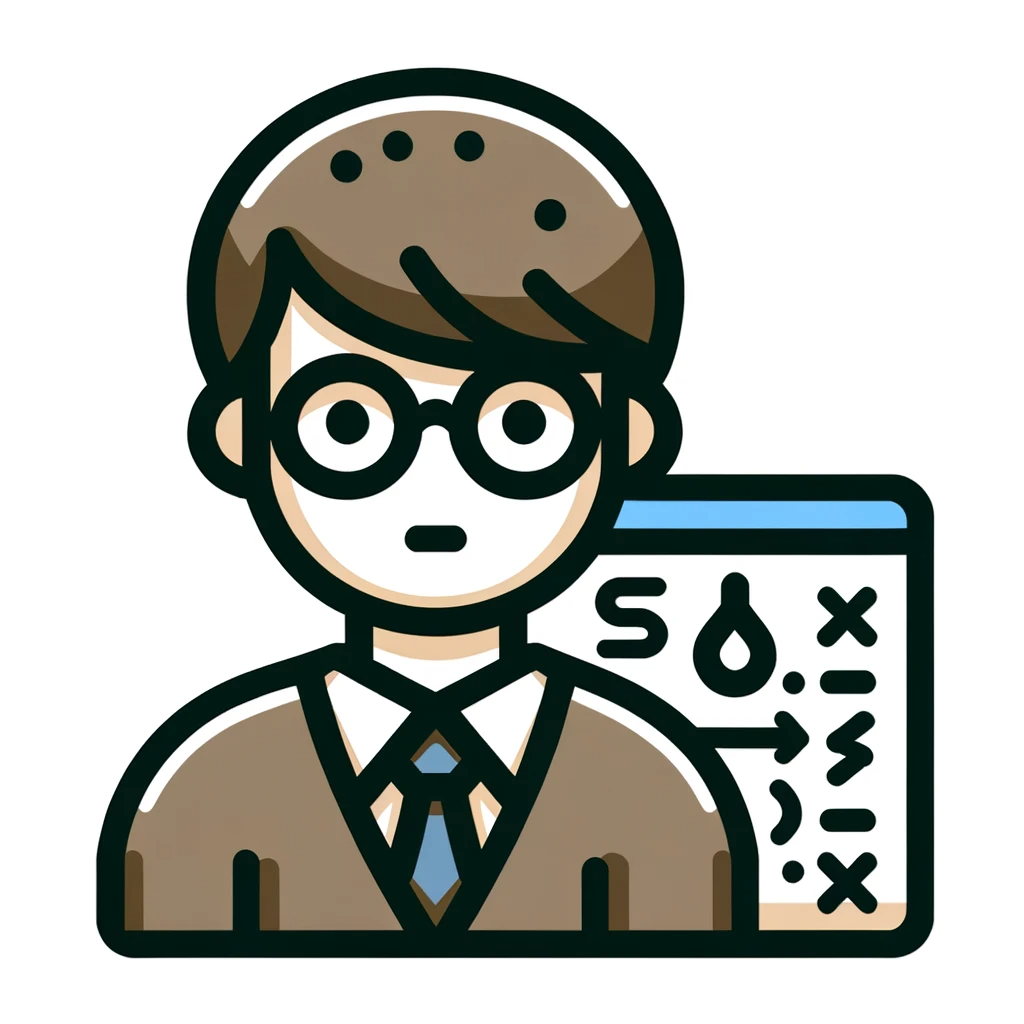
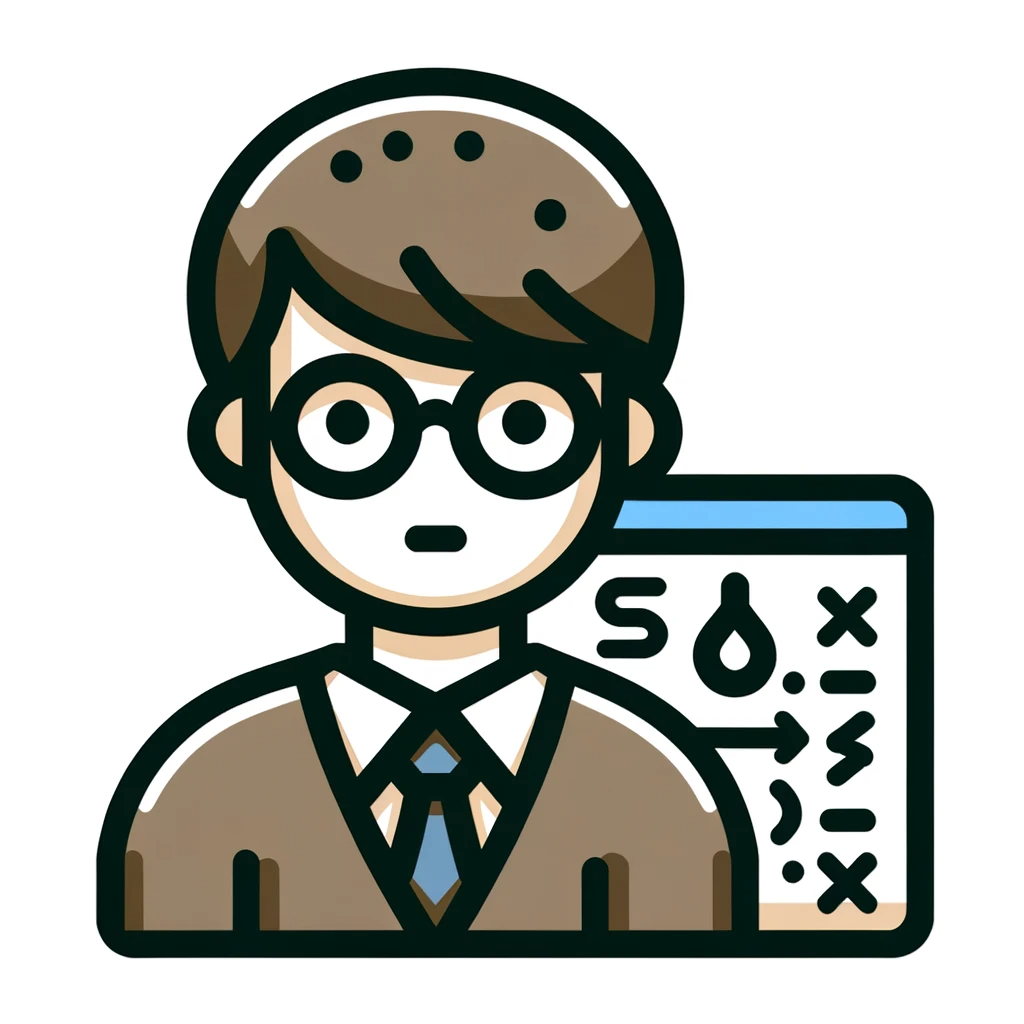
Being aware of JavaScript execution timing is an important point that is directly connected to web page loading speed and user experience.
It is important to choose and implement the correct method to avoid delays and processing delays.
The DOMContentLoaded and window.onload explained this time are essential functions for web development, and we recommend that you understand them.
Comments