This article explains how to register an event listener on a non-existing element using JavaScript .
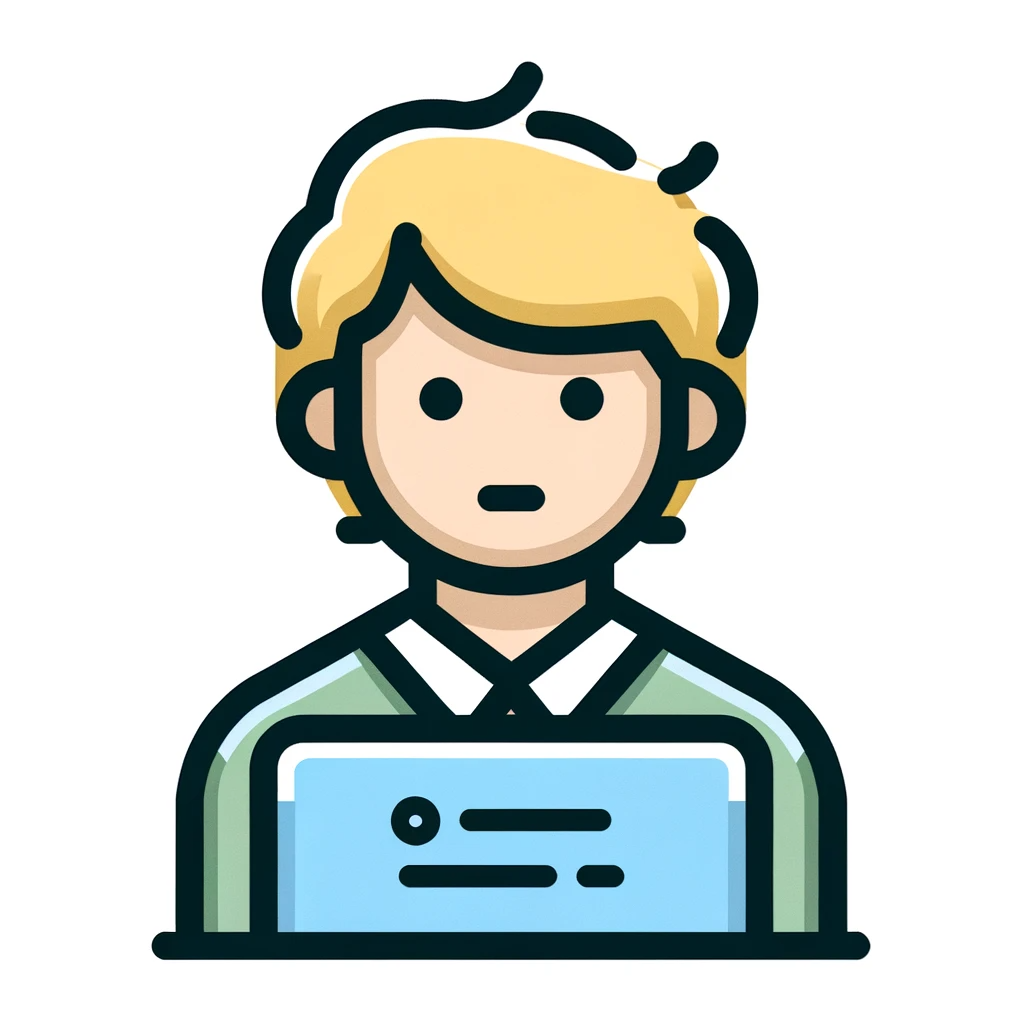
I would like to know how to register an event listener on an element that does not exist. What should I do?
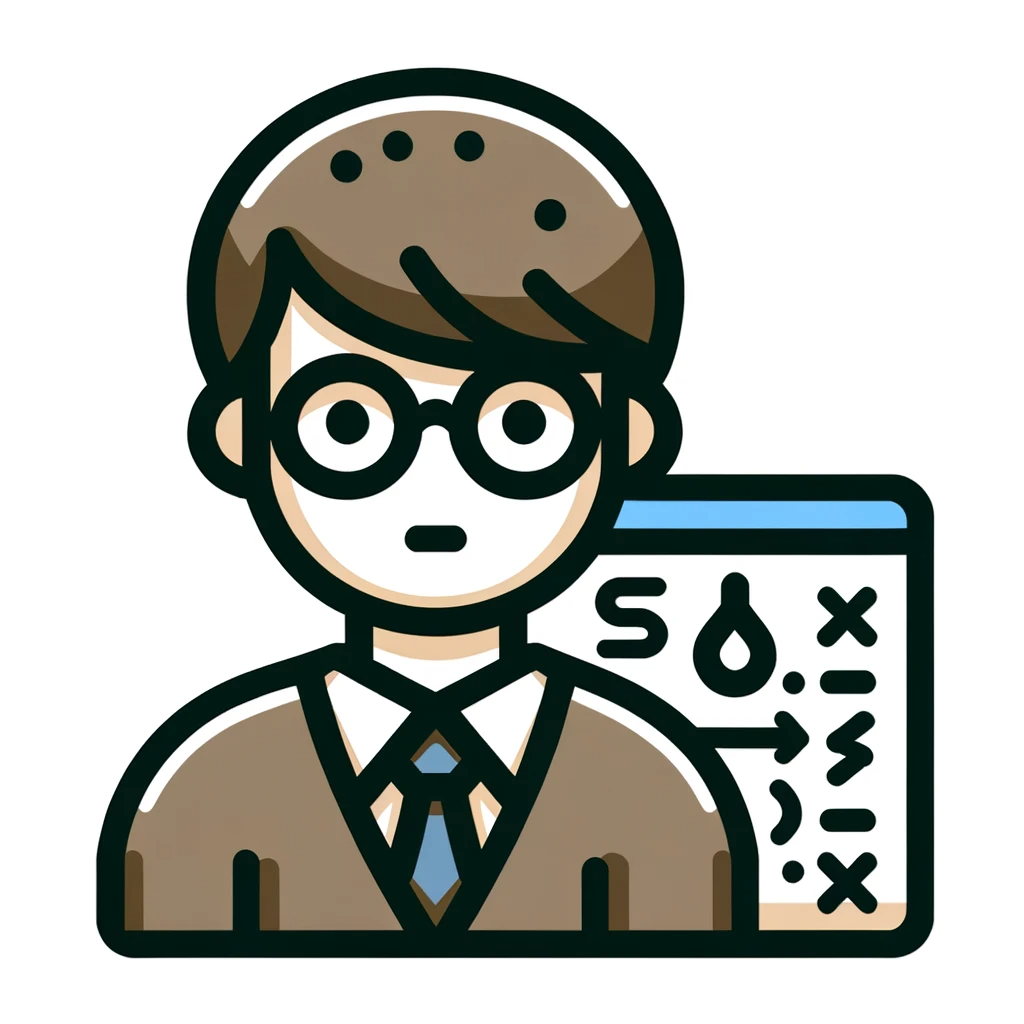
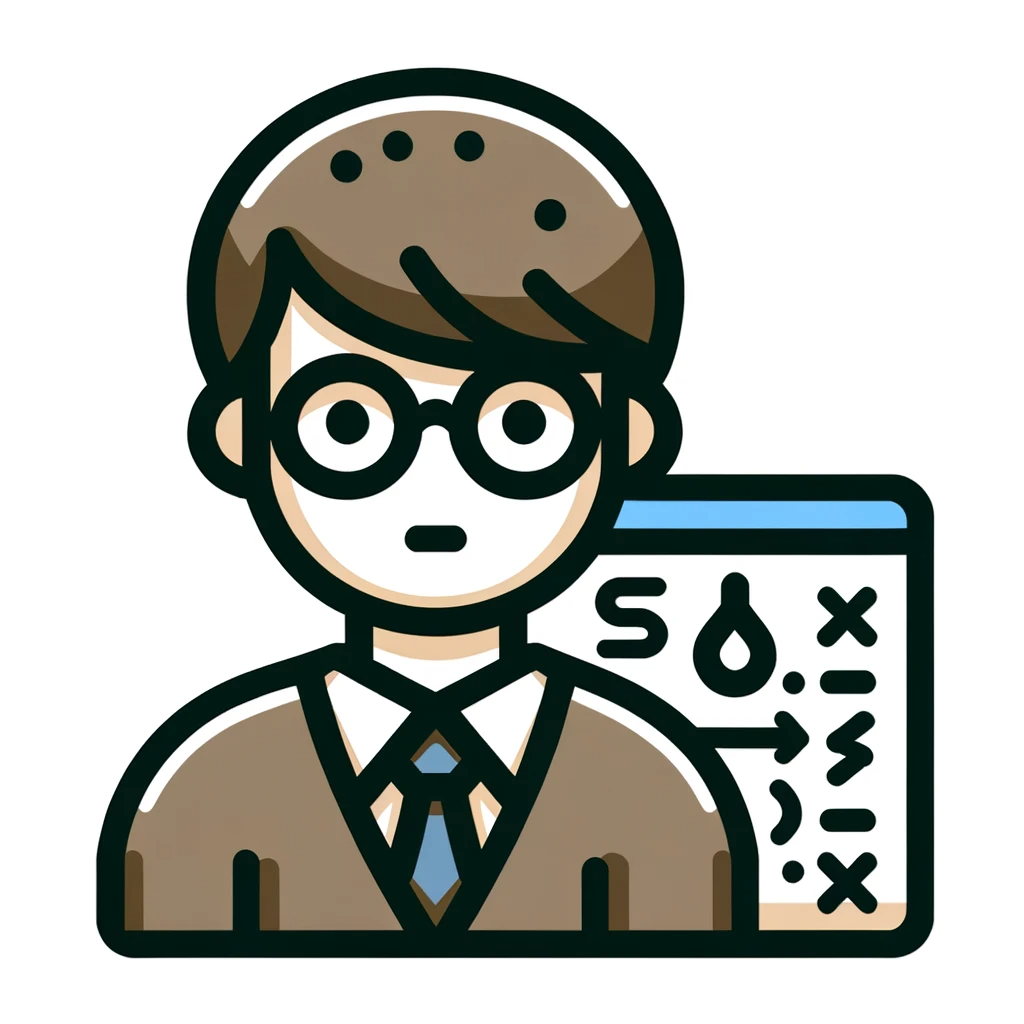
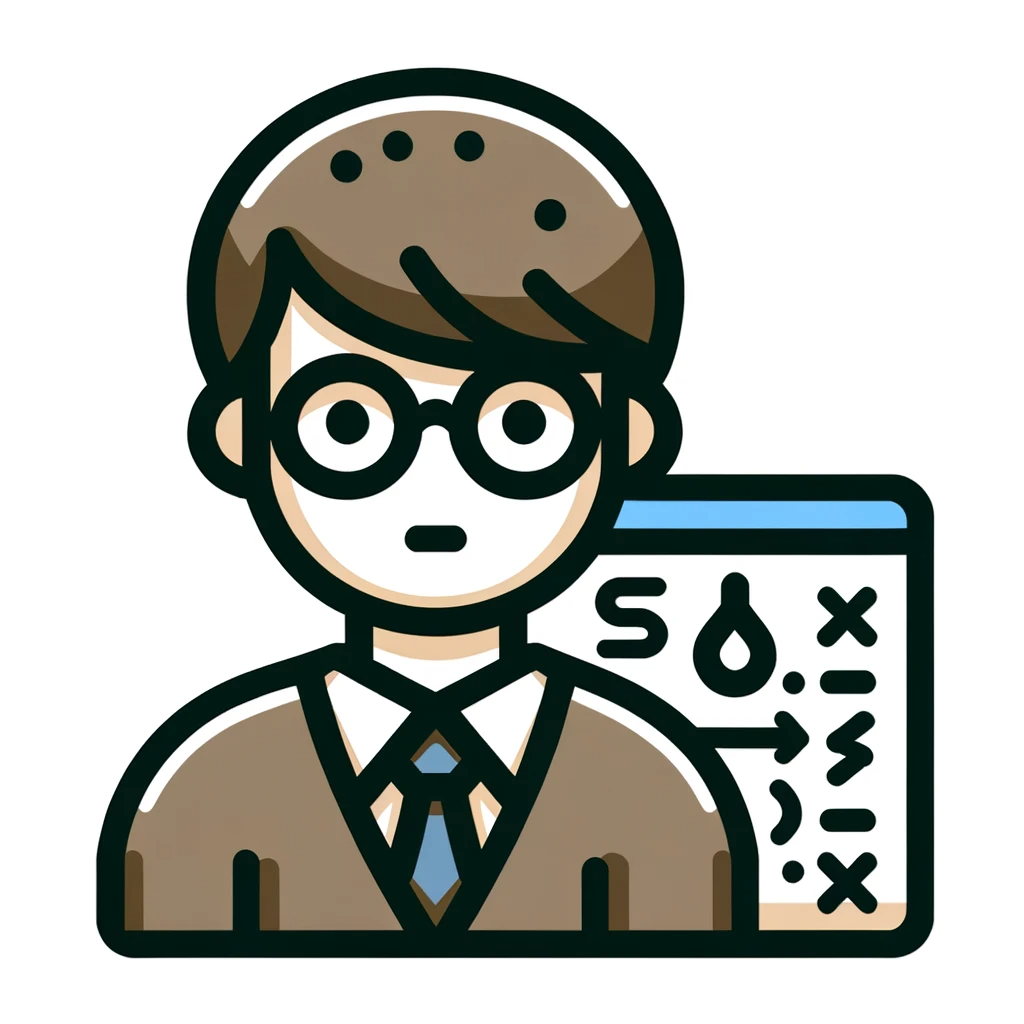
You can easily register an event listener for an existing element, but you cannot register it for an element that does not exist.
In that case, the following methods are available.
How to register an event listener on a non-existing element
1. Create an element using the document object
If you want to register an event listener for an element that doesn’t exist, first create the element using a document object. For example, you can create a button element as below.
const button = document.createElement("button");
2. Register event listener on element
Register an event listener for the created element. For example, to register a click event, use the addEventListener method as shown below.
button.addEventListener("click", function() {
// Processing to be performed when clicked
});
3. Add the created element to the DOM
Add the created element to the DOM. For example, if you want to add it to the body element, use the appendChild method as shown below.
document.body.appendChild(button);
Now you can register event listeners for the elements you have created and process events for elements that do not exist.
Explanation using a sample program
Below is a sample program that registers an event listener on an element that does not exist.
const button = document.createElement("button");
button.innerHTML = "Click me";
button.addEventListener("click", function() {
alert("Hello, world!");
});
document.body.appendChild(button);
This example creates a button element, registers an event listener to display an alert when it is clicked, and adds the button element to the DOM.
This is how to register an event listener on an element that does not exist. Note that you need to create the element and add it to the DOM before registering the event listener.
How to register event listeners for other non-existing elements
In addition to the methods introduced above, there are other methods for registering event listeners for non-existing elements, such as the following.
Register event listeners for existing elements and use event bubbling
This method registers an event listener on an existing parent element and uses event bubbling to retrieve the clicked child element. For example, register an event listener on the parent element for the click event as shown below.
const parent = document.querySelector("#parent");
parent.addEventListener("click", function(event) {
const target = event.target;
if (target.tagName === "BUTTON") {
// Processing when the button is clicked
}
});
Monitor element changes using MutationObserver
How to use MutationObserver to monitor DOM changes and register event listeners when elements are added. For example, you can use MutationObserver to monitor changes in the body element and register a click event when a button element is added, as shown below.
const observer = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if (mutation.addedNodes) {
mutation.addedNodes.forEach(function(node) {
if (node.tagName === "BUTTON") {
node.addEventListener("click", function() {
// Processing when the button is clicked
});
}
});
}
});
});
observer.observe(document.body, { childList: true, subtree: true });
By using these methods, you can register event listeners for elements that do not exist.
summary
We explained how to register an event listener to an element that does not exist.
- To register an event listener for an element that does not exist, you need to create the element using a document object.
- Register an event listener for the created element.
- Add the created element to the DOM.
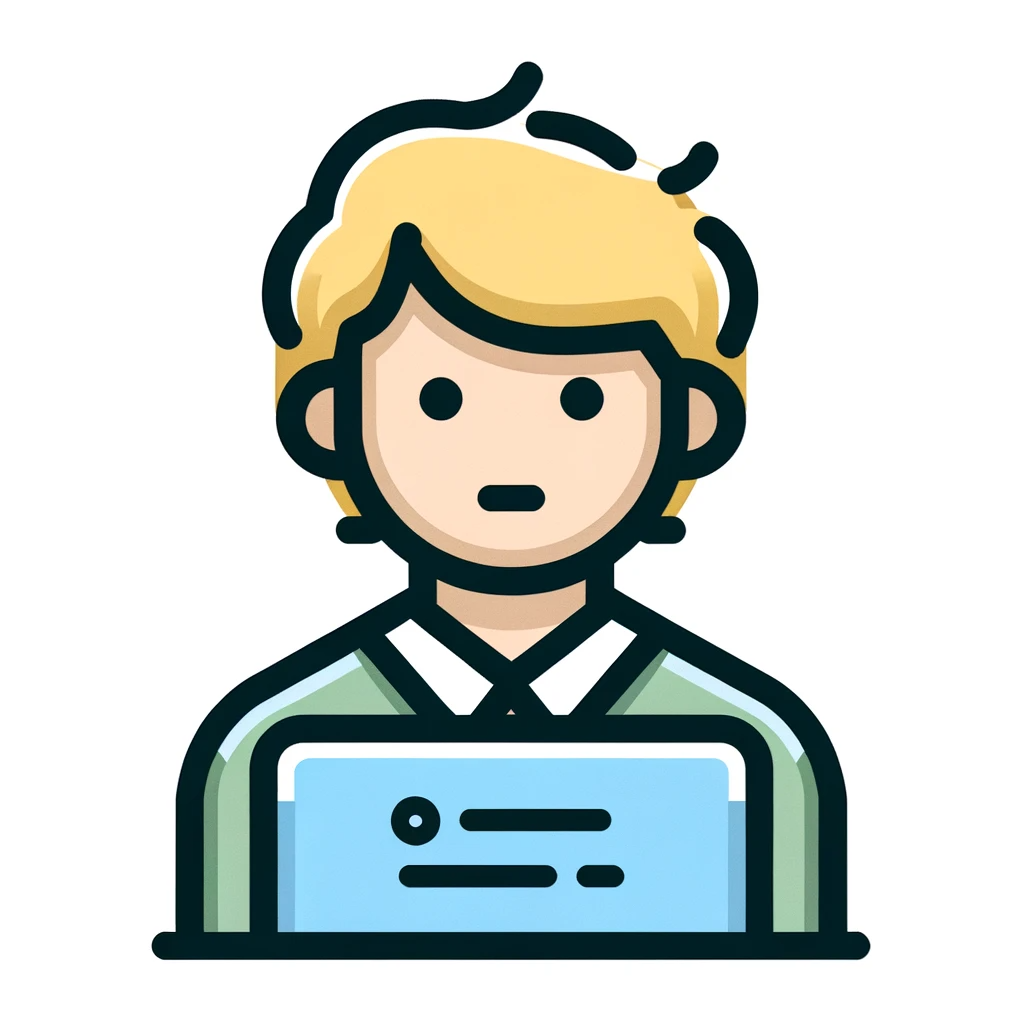
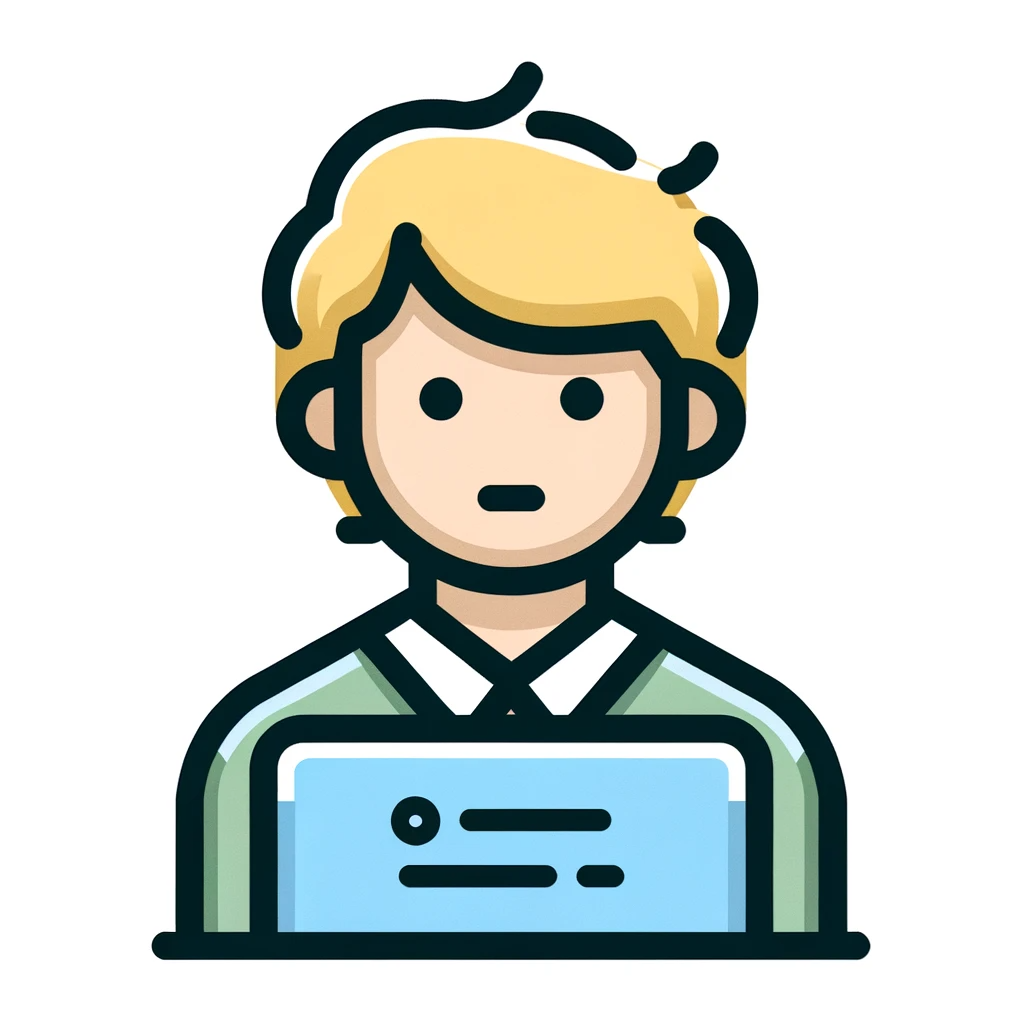
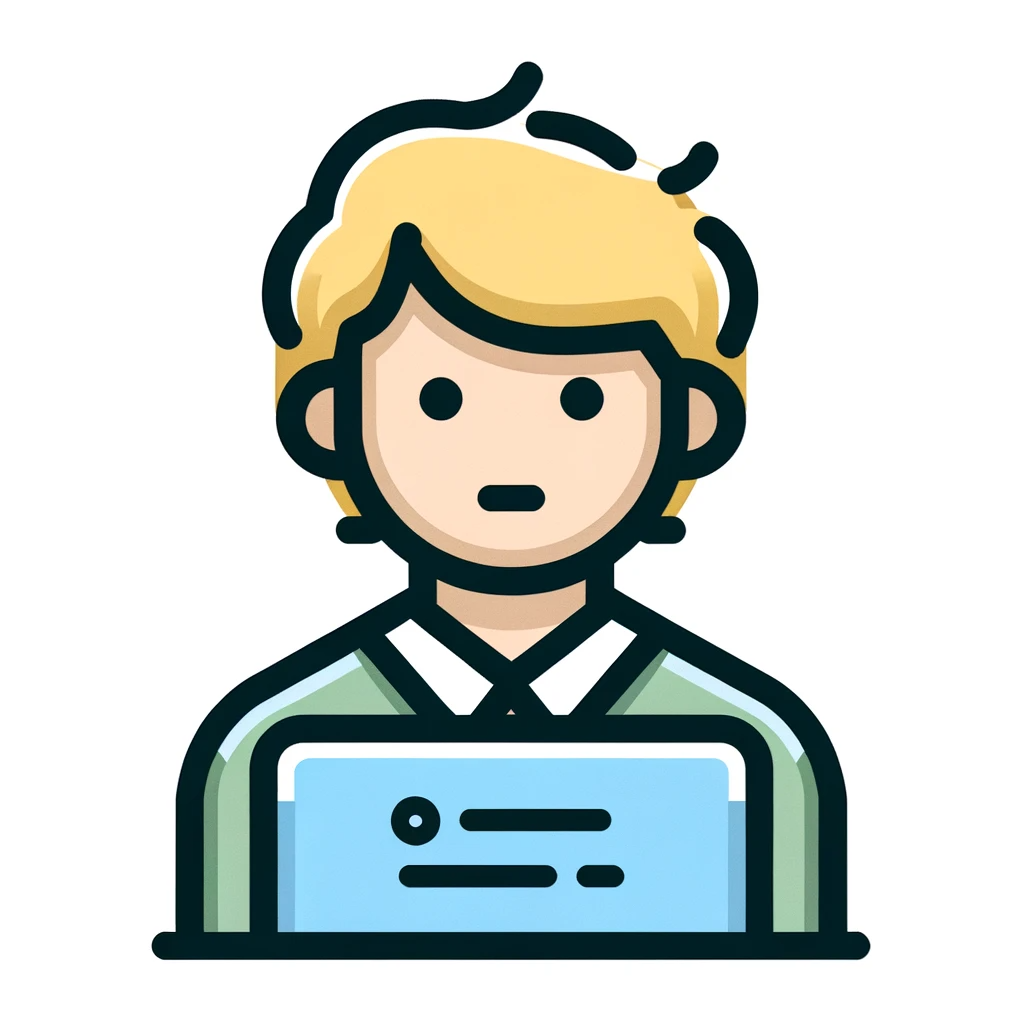
By creating an element using a document object, you can register an event listener for an element that does not exist.
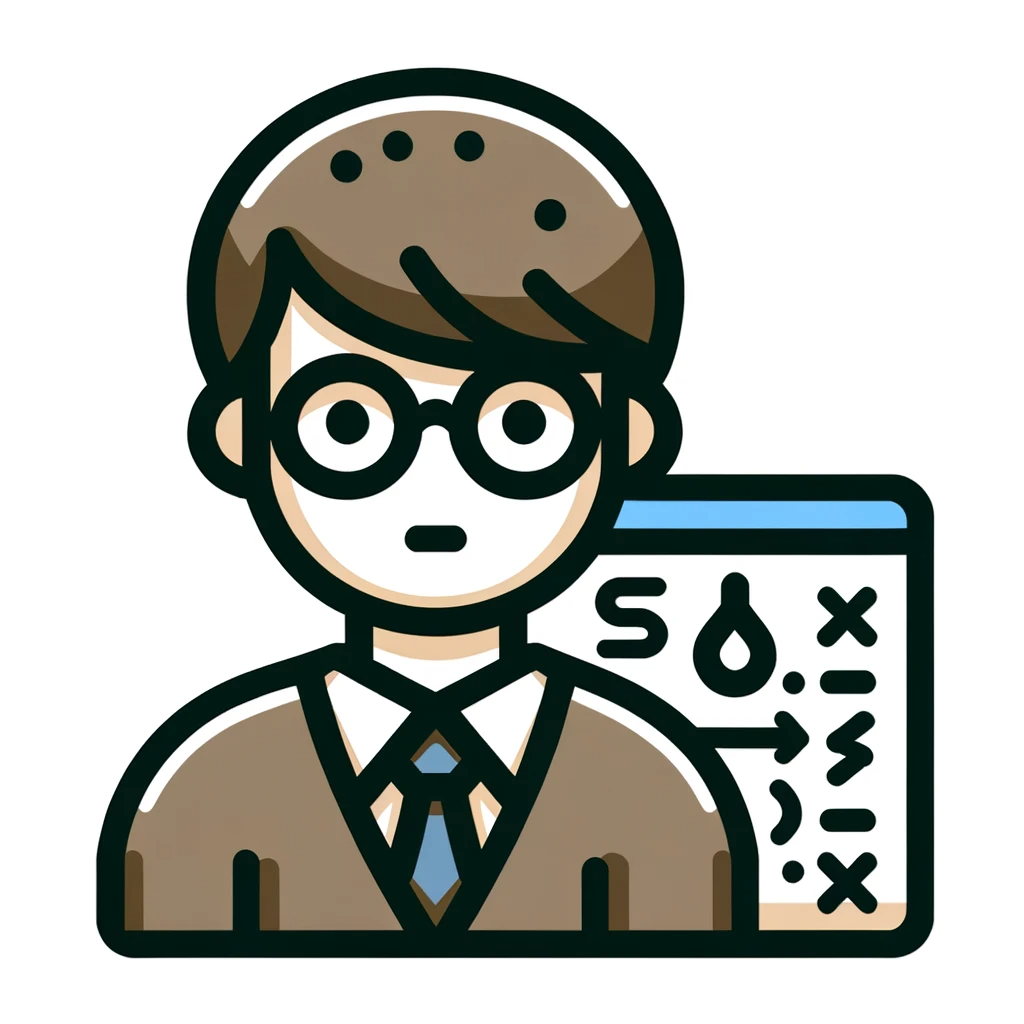
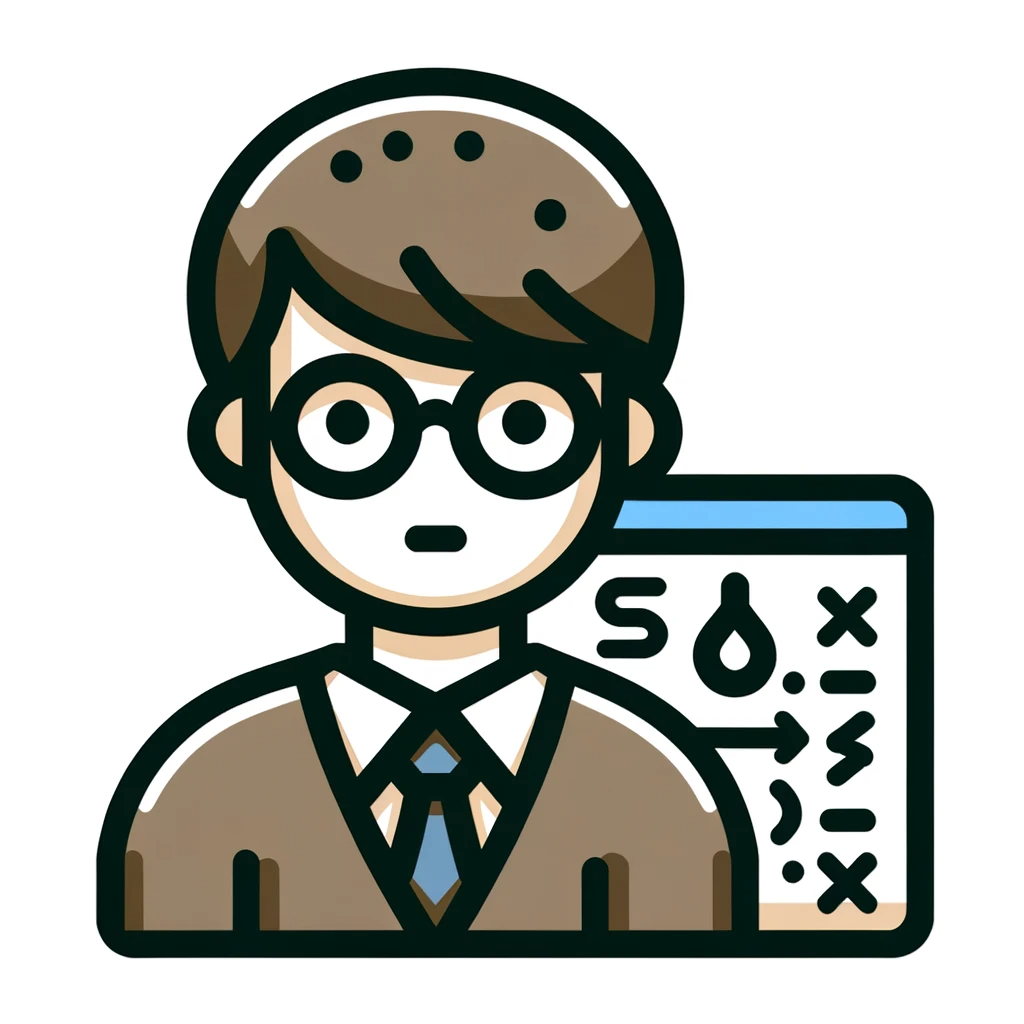
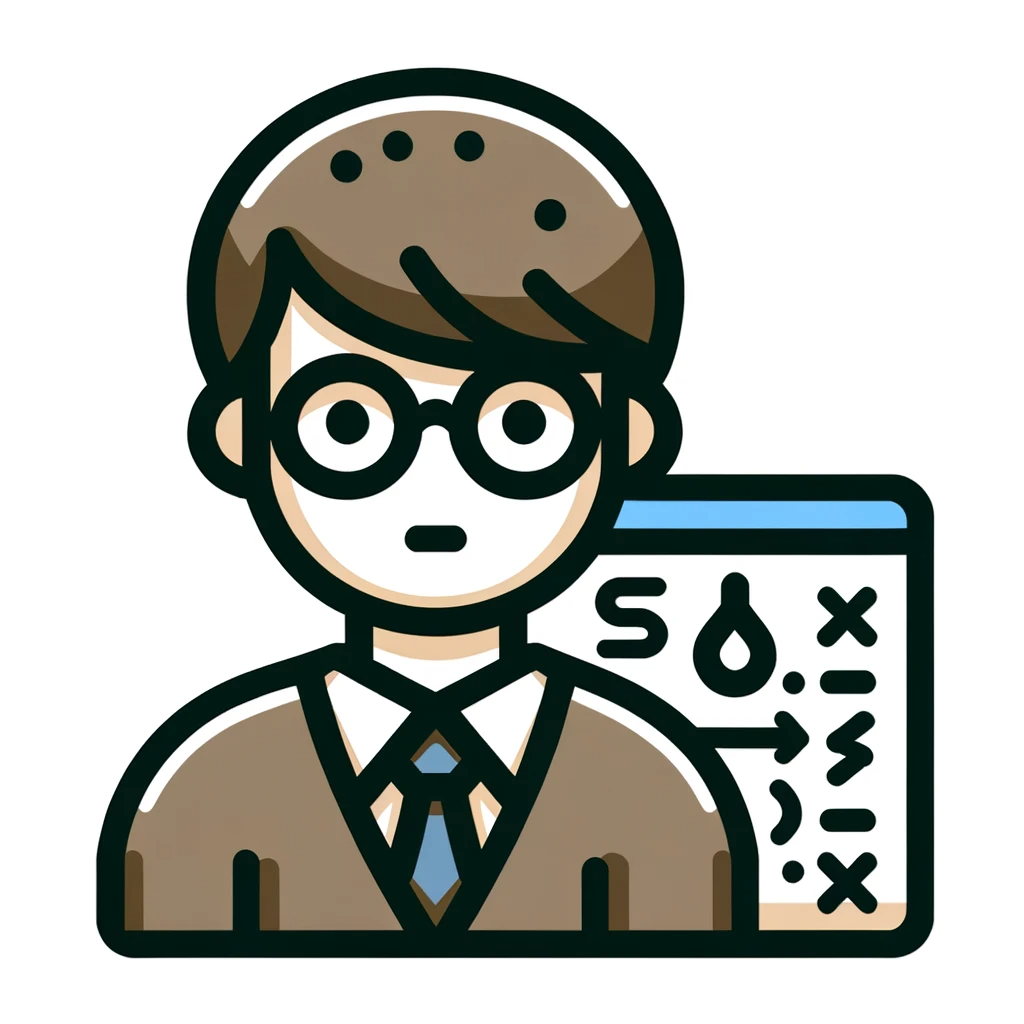
If you want to register an event listener for an element that does not exist, create the element using the document object, register the event listener for the created element, and then add it to the DOM.
Comments