This article explains how to obtain the date and time difference using JavaScript .
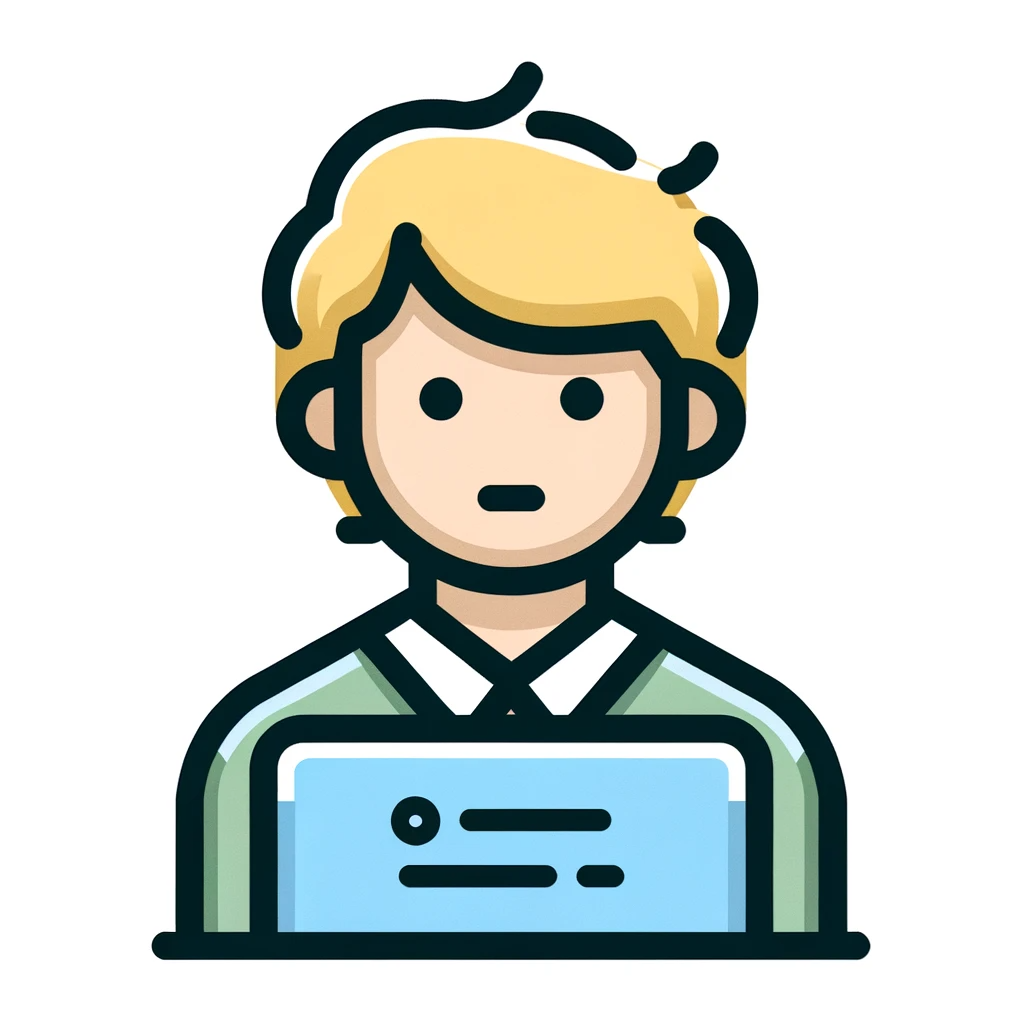
How can I get the difference in date and time?
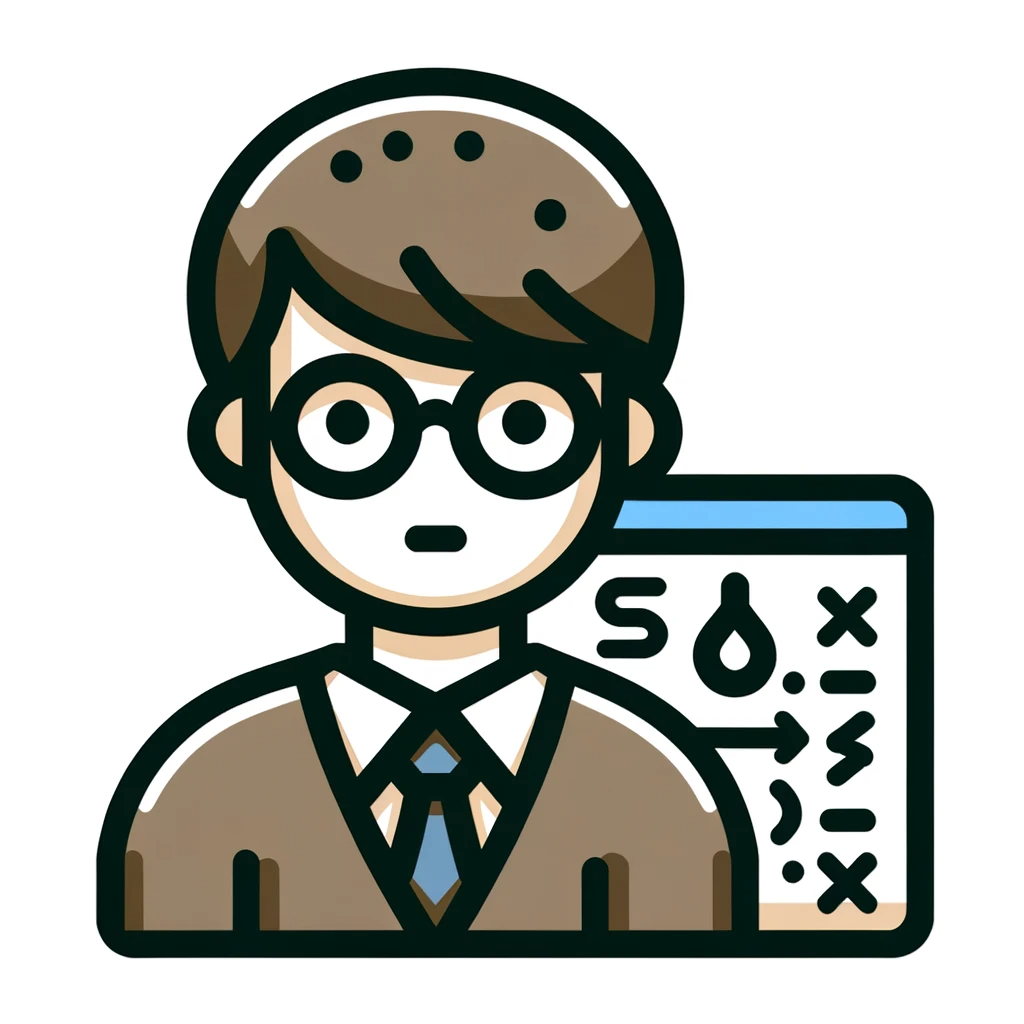
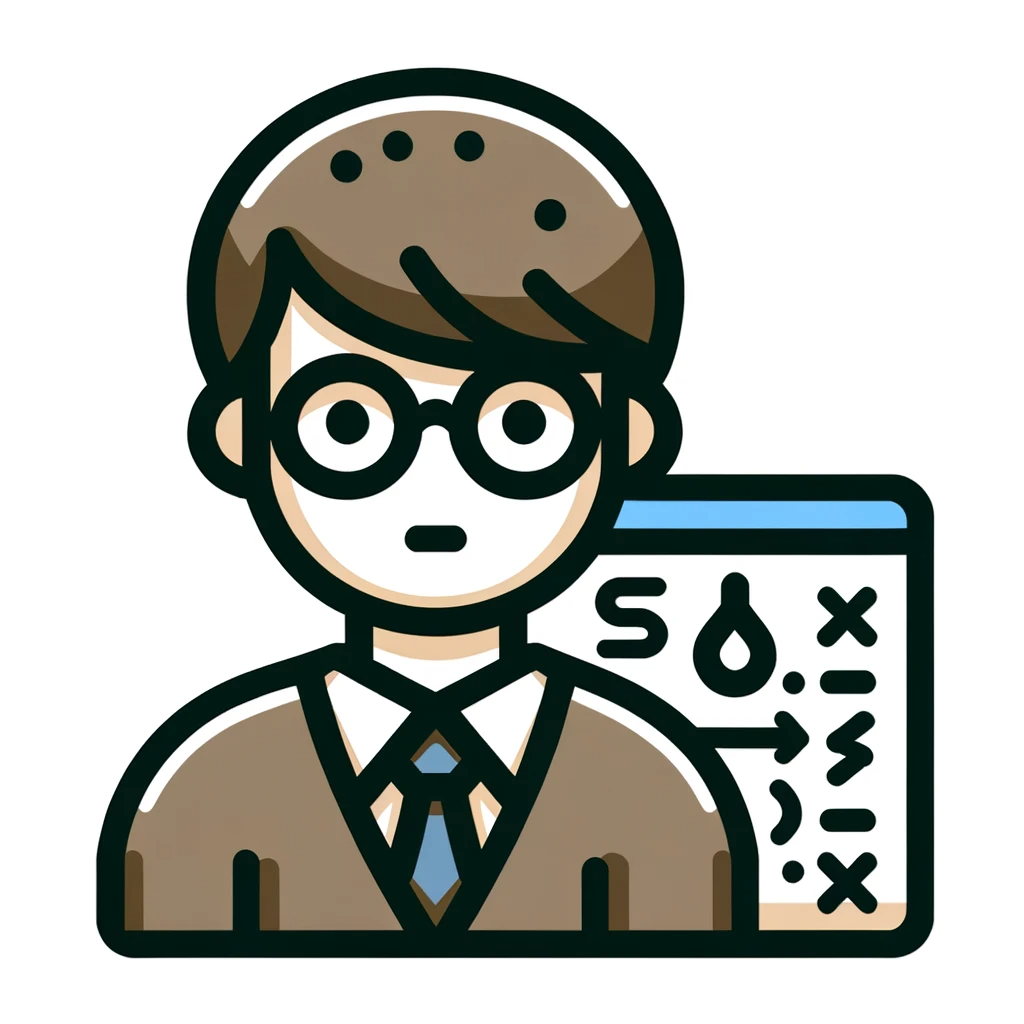
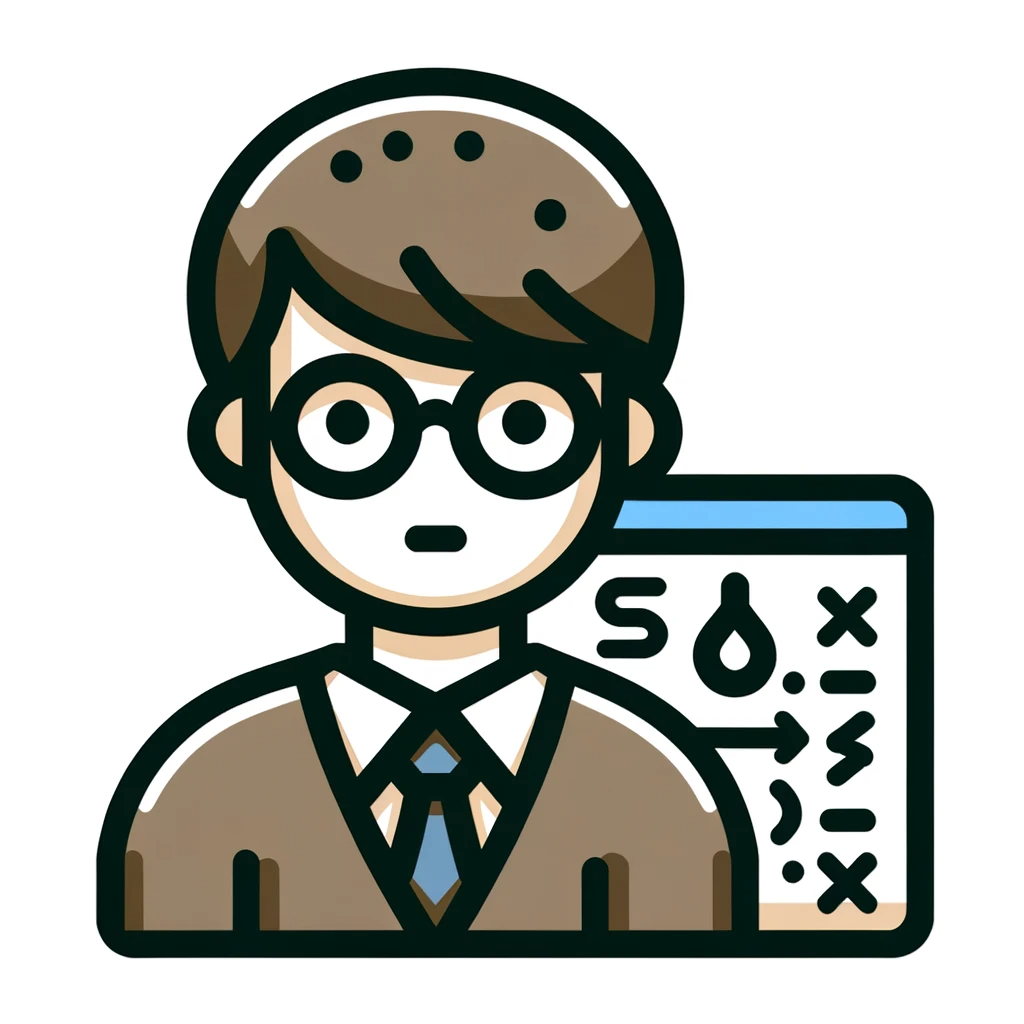
You can use the Date object to get the difference in date and time.
How to get the difference in date and time
To obtain the difference between dates and times, use the Date object .
The Date object is an object for handling dates and times, and also has functions for obtaining the difference between dates and times.
If you want to get the difference between two dates and times, do as below.
// Date and time 1
let date1 = new Date("2022-01-01");
// Date and time 2
let date2 = new Date("2022-01-31");
// Difference in date/time (ms)
let diff = date2.getTime() - date1.getTime();
// Difference in date and time (days)
let diffDays = diff / (1000 * 60 * 60 * 24);
console.log(diffDays + "There is a difference of days.");
In the above sample, “2022-01-01” is entered in date1 and “2022-01-31” is entered in date2, and the difference between the two days and times is obtained. The getTime() method is used to retrieve two dates and times in milliseconds and convert the difference to days.
summary
The following is a summary of how to obtain the date and time difference.
- To get the difference in date and time, use the Date object.
- Use getTime() method to get two dates and times in milliseconds.
- By converting the obtained milliseconds to days, you can obtain the difference in date and time.
You can use a Date object and get the difference between two dates and times using the getTime() method.
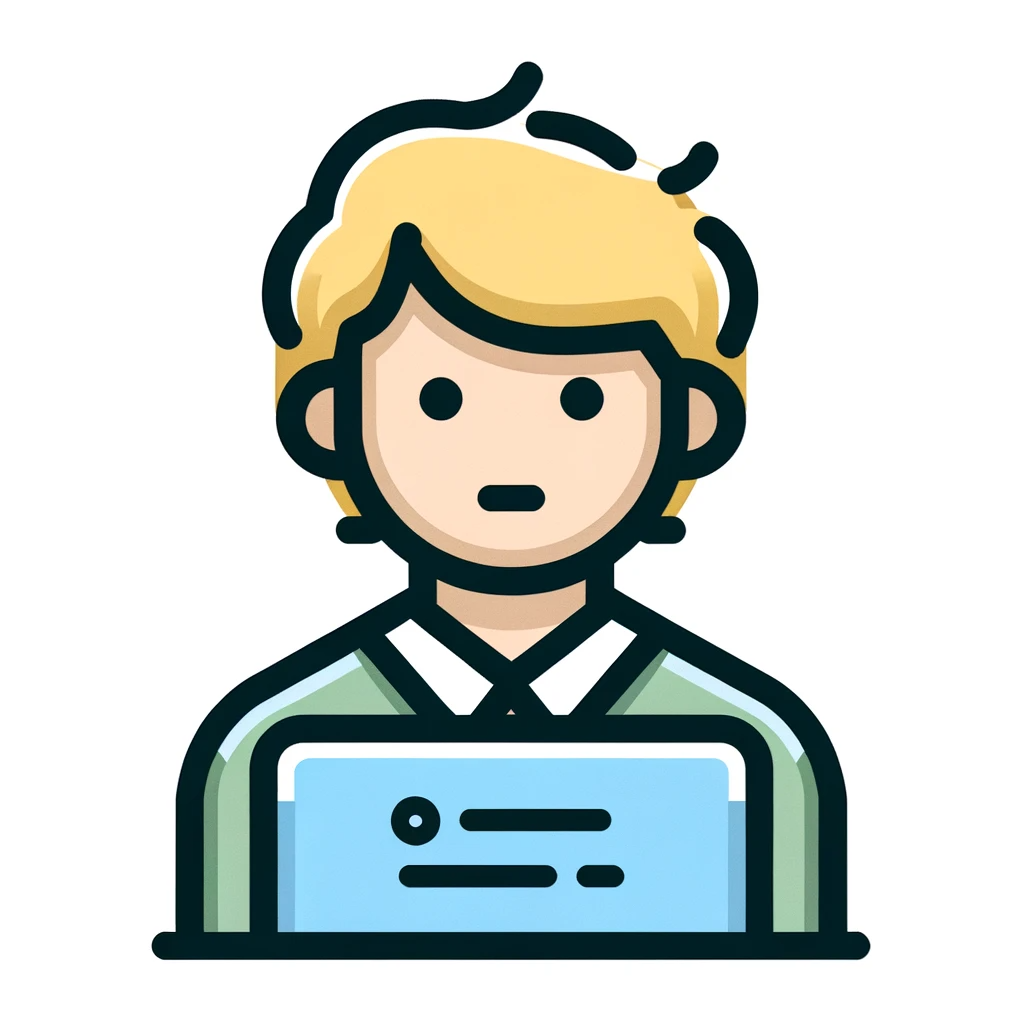
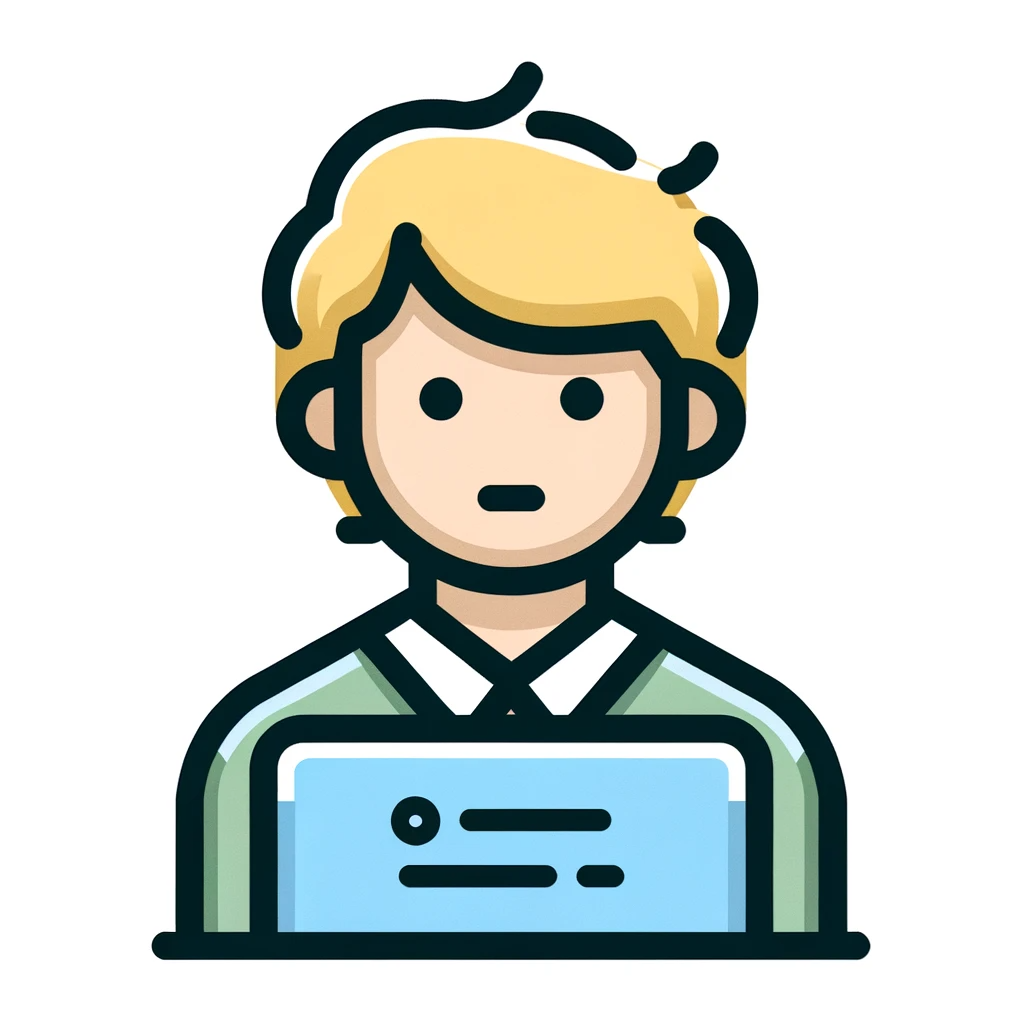
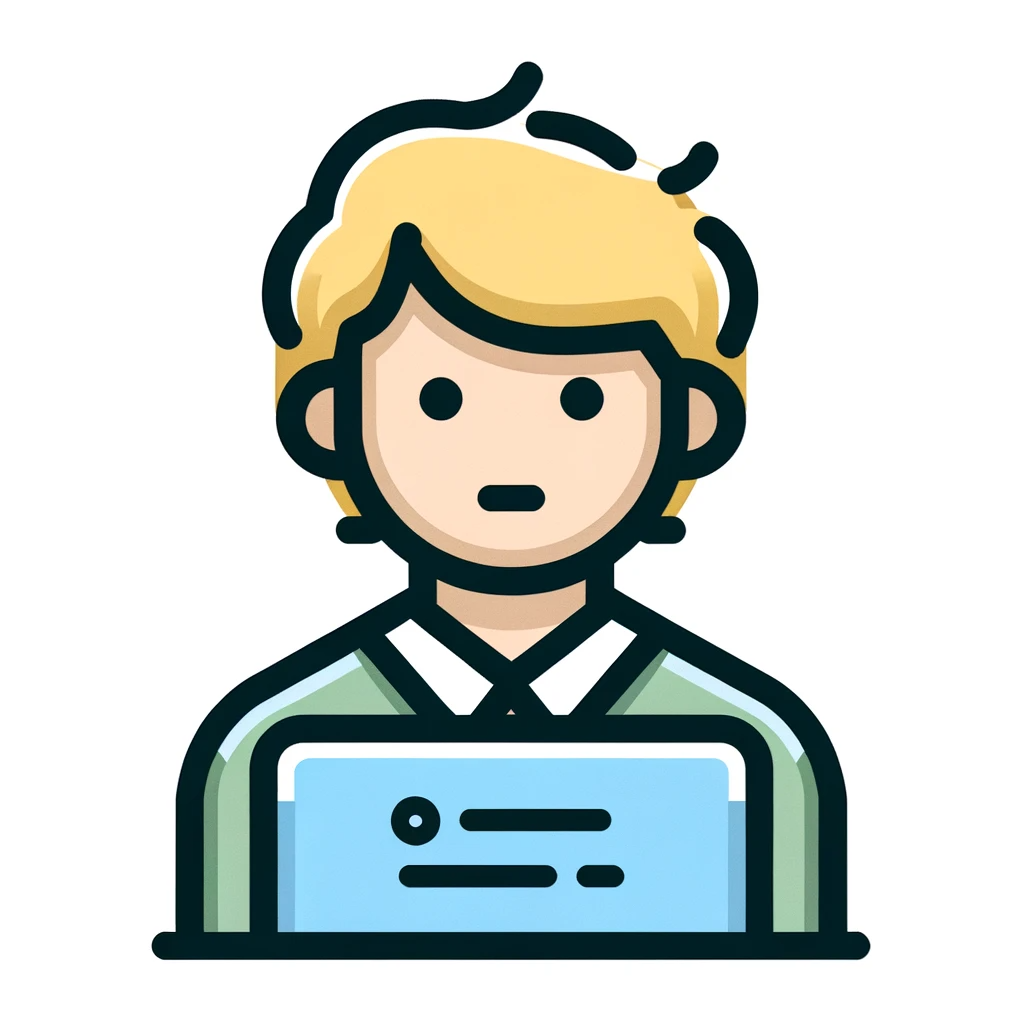
With this method, we were able to easily obtain the date and time difference!
Comments