This section explains how to obtain a timestamp value from a date string .
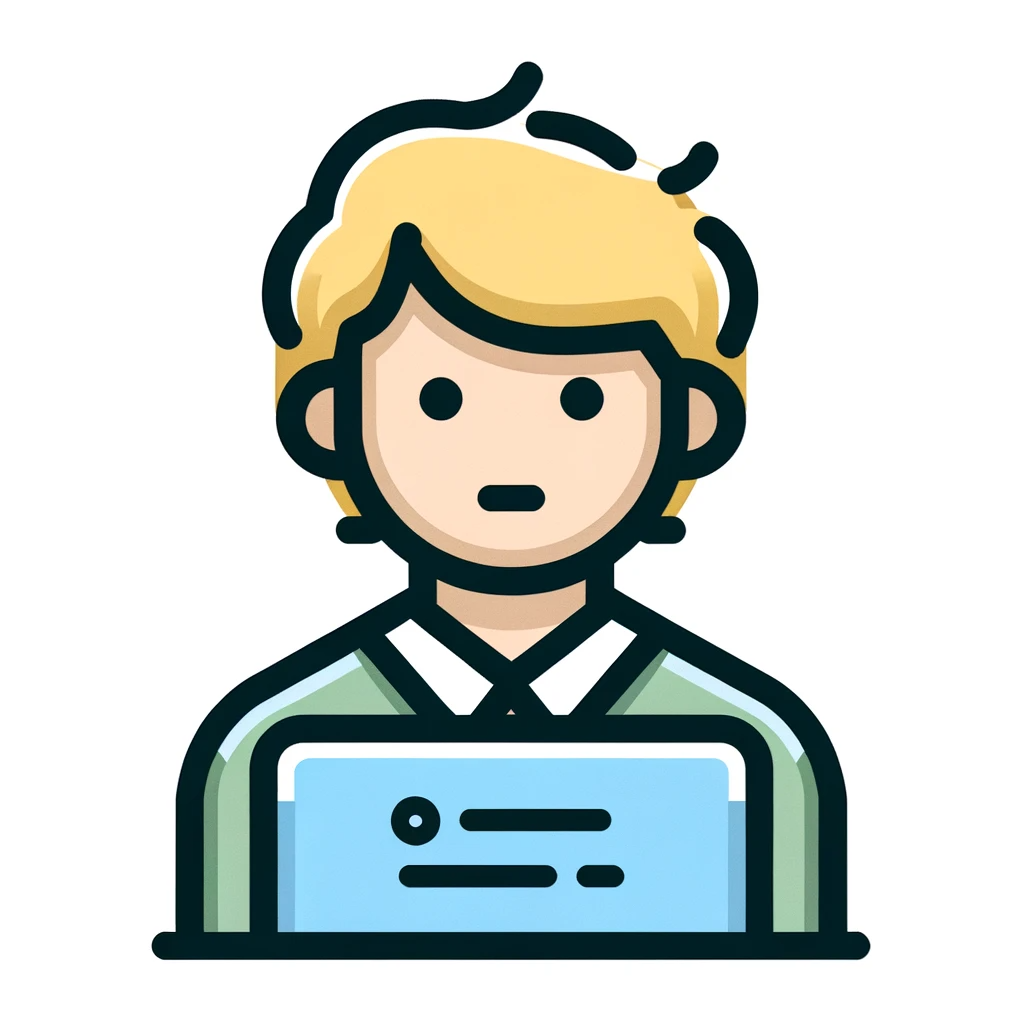
How can I convert a date string to a timestamp?
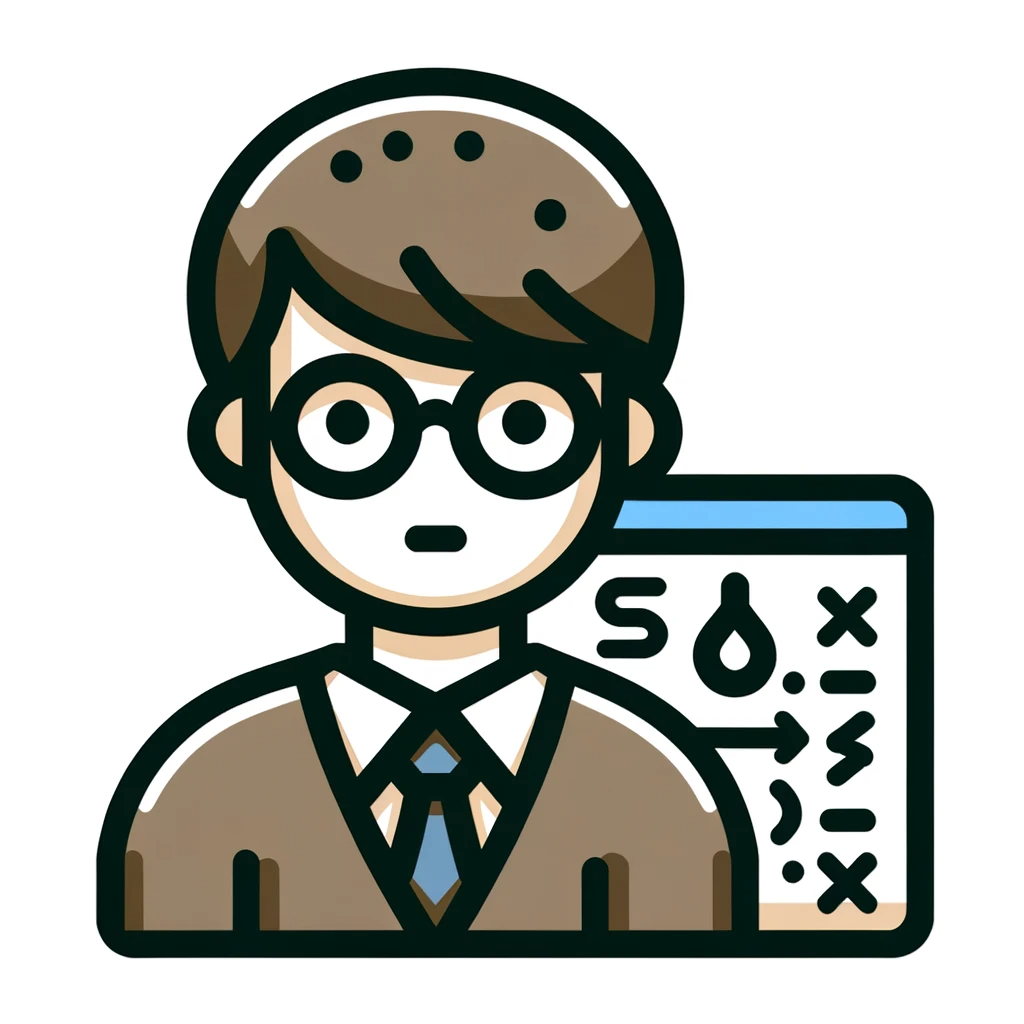
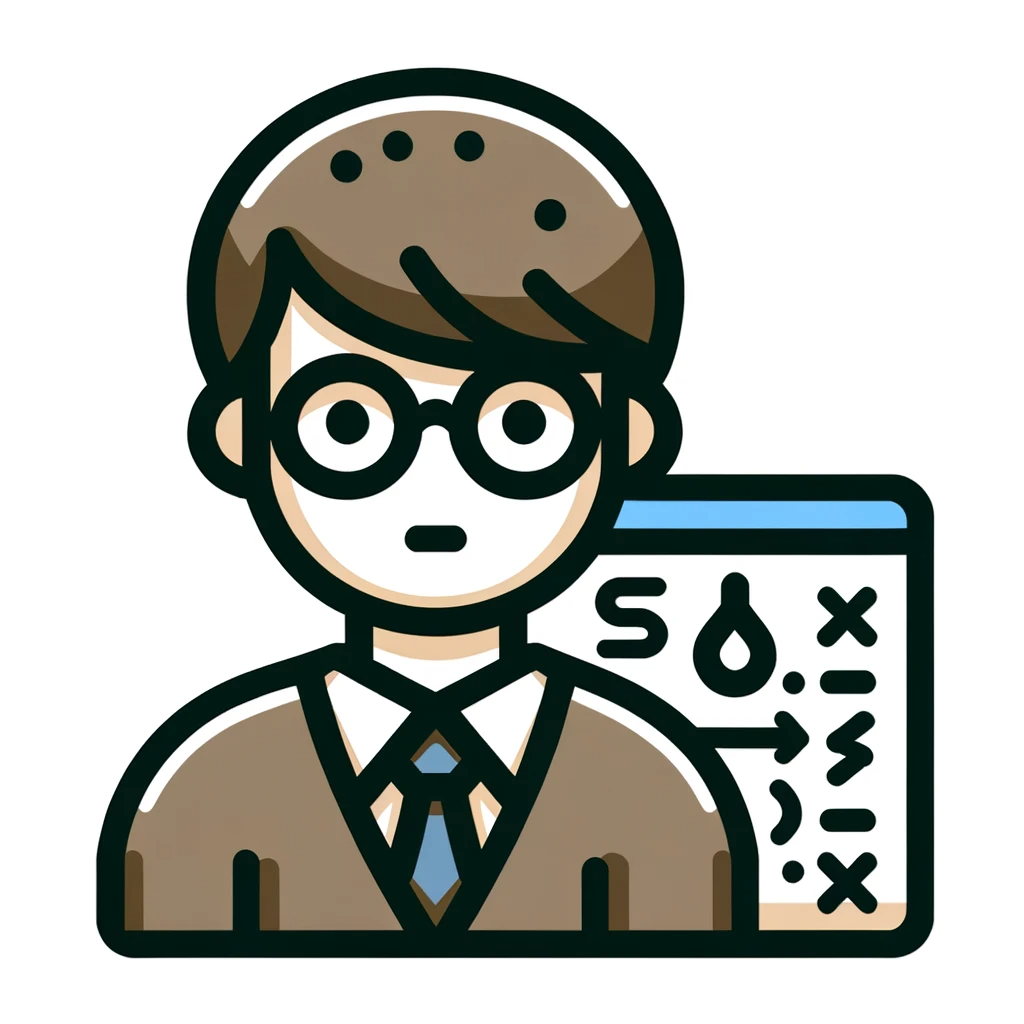
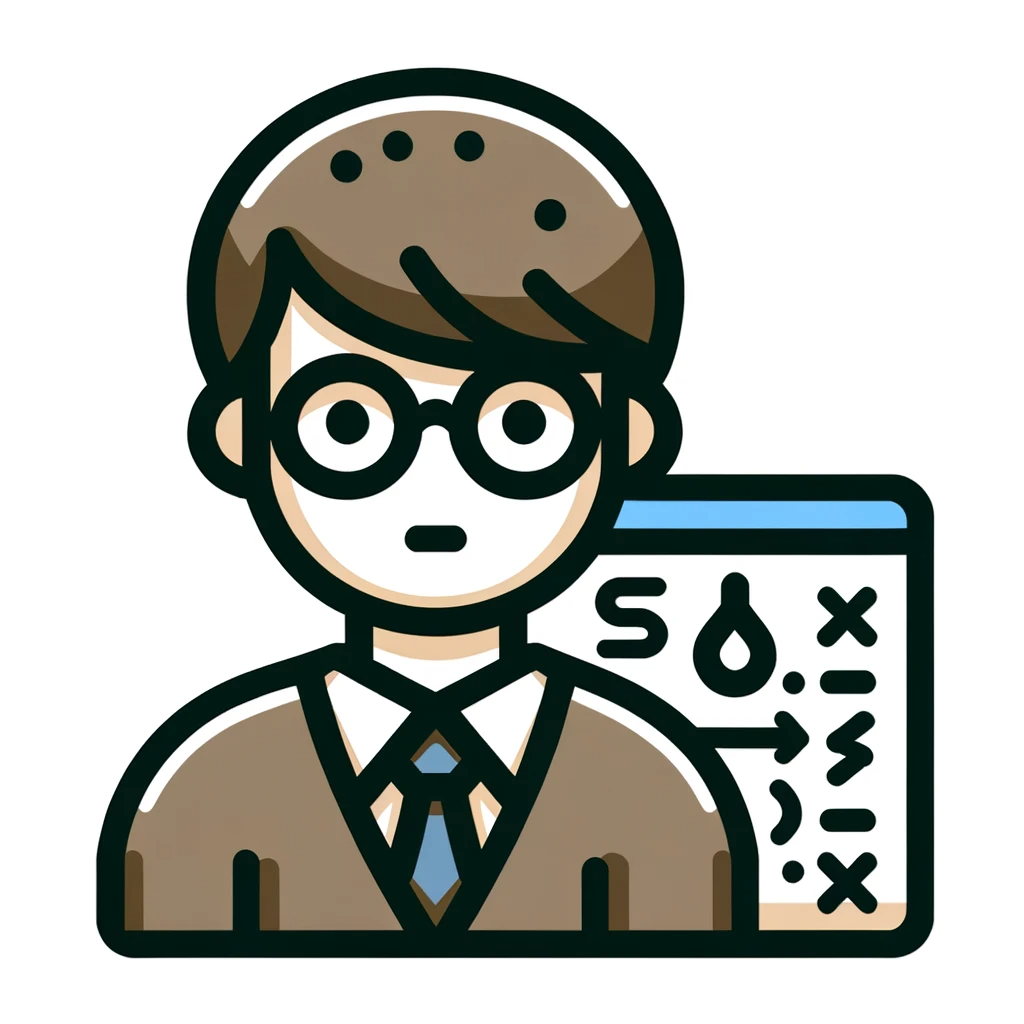
You can use the Date object to get a timestamp value from a date string.
How to obtain timestamp value from date string using Date object
You can use the Date object to get a timestamp value from a date string.
To use a Date object, first pass a date string to the Date constructor.
Use the getTime method to retrieve the timestamp value from a Date object.
Date object.getTime();
// Date string
var dateString = "2022-12-31";
// Convert date string to Date object
var date = new Date(dateString);
// Get timestamp value
var timestamp = date.getTime();
console.log(timestamp); // 1609459200000
Create a timestamp using Date.parse
In addition to the getTime method, if you want to retrieve the date from a string, you can use the Date.parse method .
Date.parse(datetime string);
// Date string
var dateString1 = "2022-12-31";
var dateString2 = "2022-12-31T10:30:00";
// 1. using Date.parse
// Convert date string to timestamp
var timestamp1 = Date.parse(dateString1);
console.log(timestamp1); // 1609459200000
// 2. using Date.parse
// Convert date string to timestamp
var timestamp2 = Date.parse(dateString2);
console.log(timestamp2); // 1609481400000
In the above example, we are using the Date.parse function to get the timestamp value from the date string.
It also shows using “new Date” to get the current date and time, and from there using the getTime method.
summary
The following is a summary of how to obtain a timestamp value from a date string.
- To get a timestamp value from a date string, you can use the Date.parse function or a Date object.
- You can get a timestamp value by passing a date string to the Date.parse function.
- If you use a Date object, you can get the current date and time with new Date() and from there use the getTime() method.
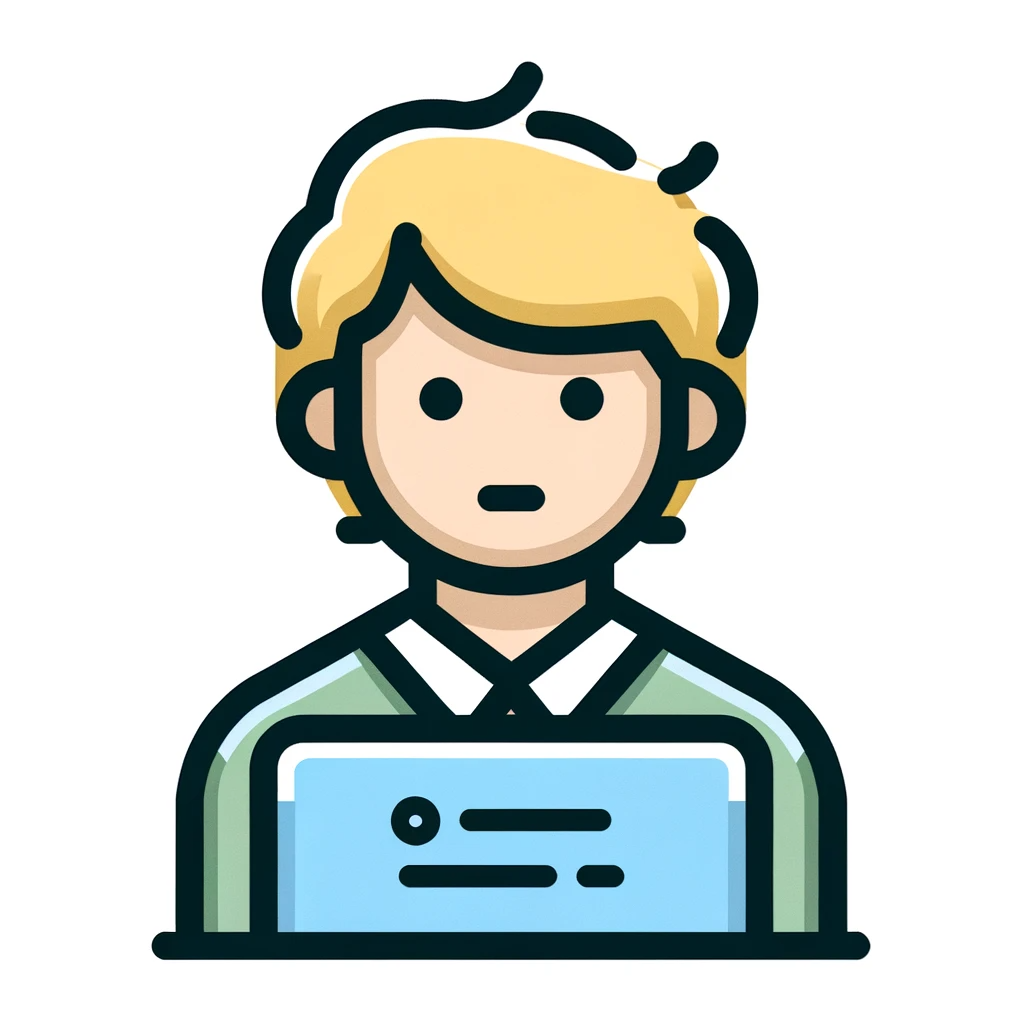
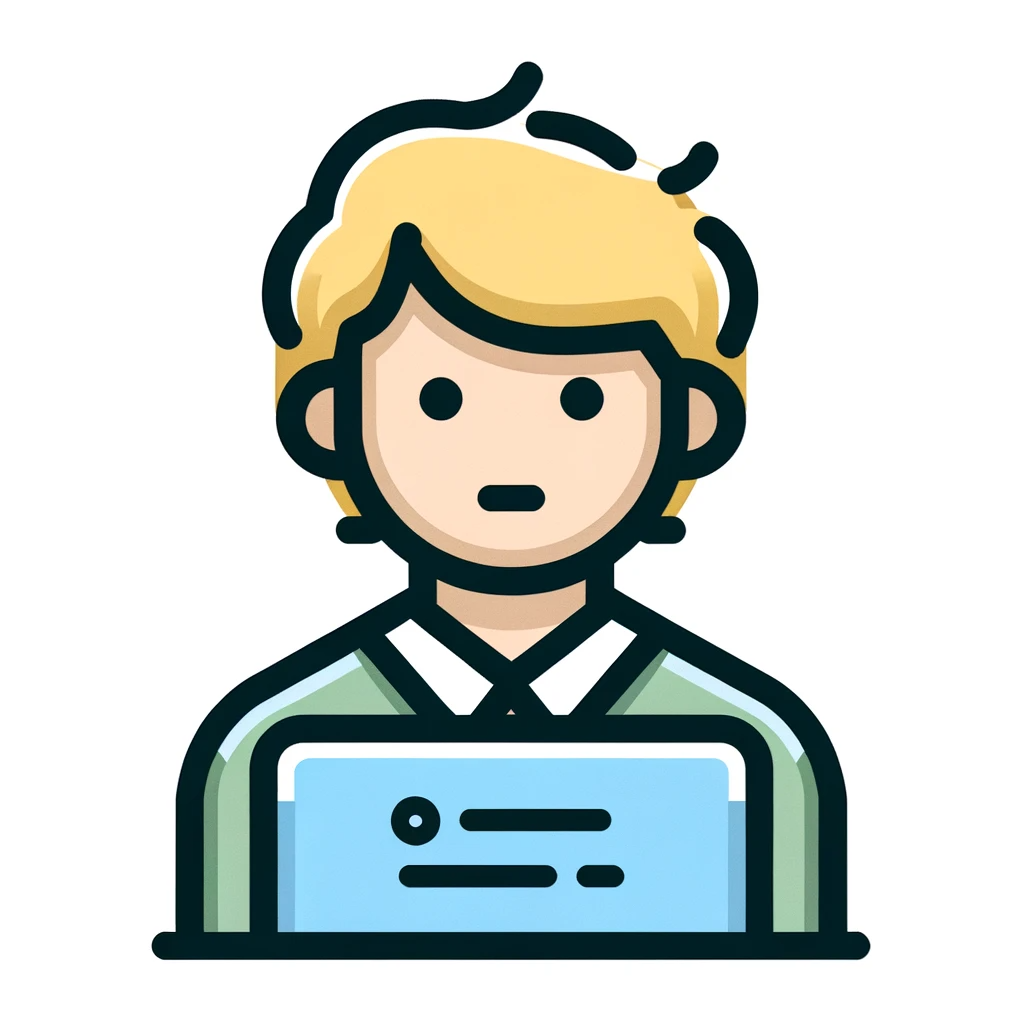
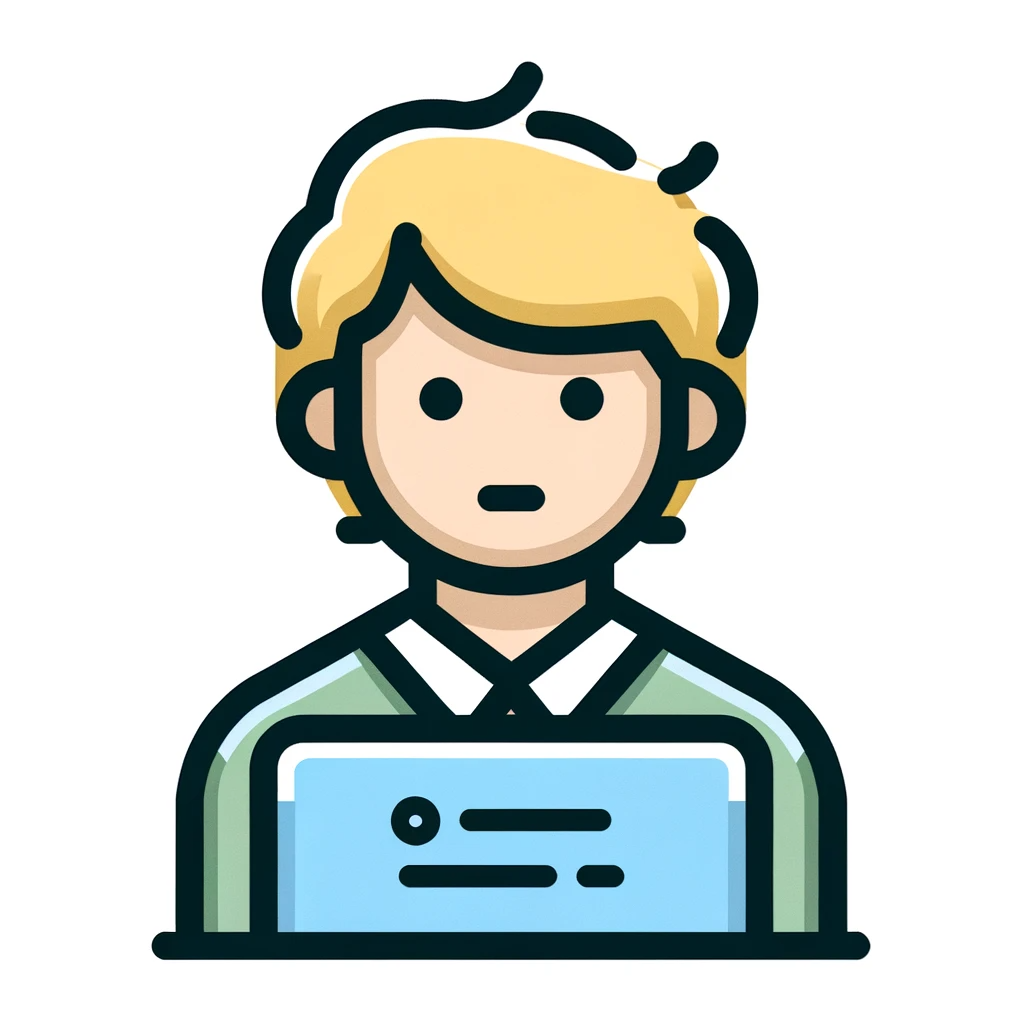
By using the Date.parse function and the Date object, we were able to easily get a timestamp value from a date string!
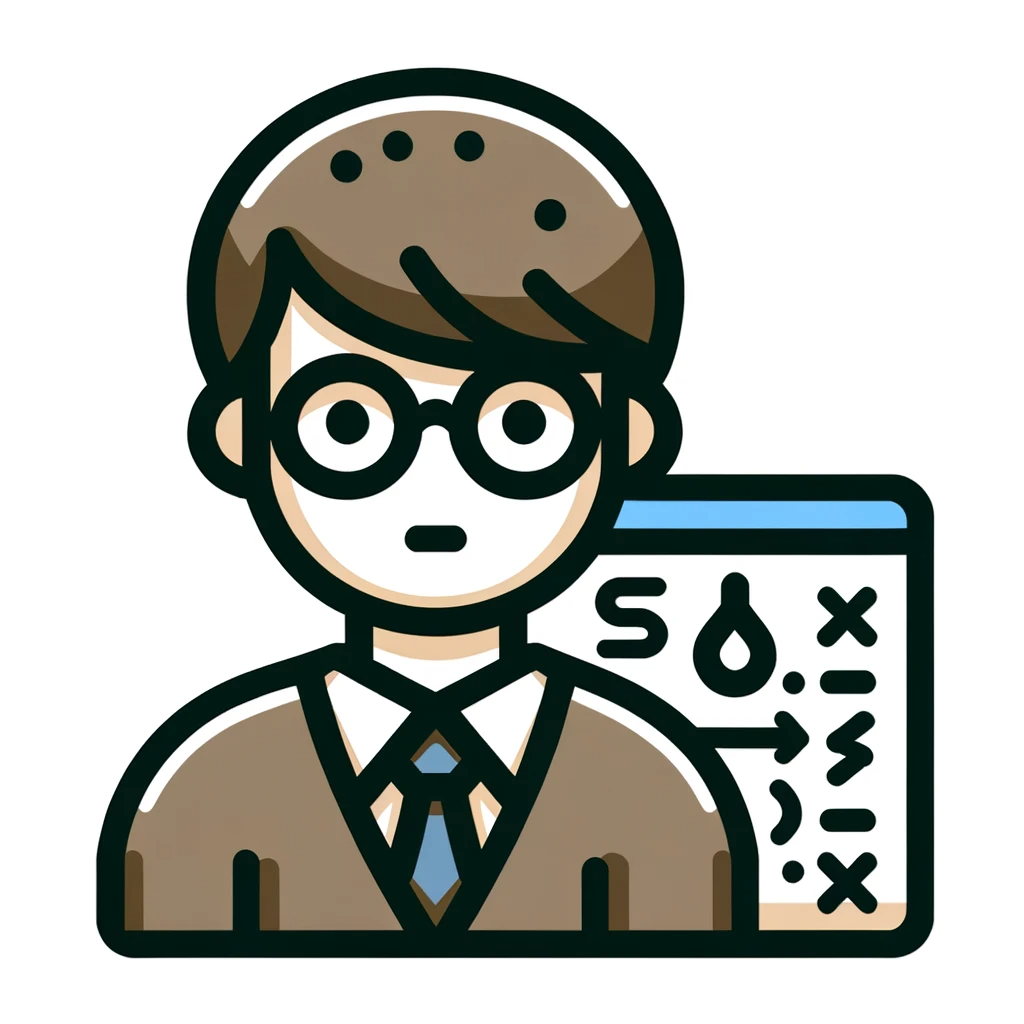
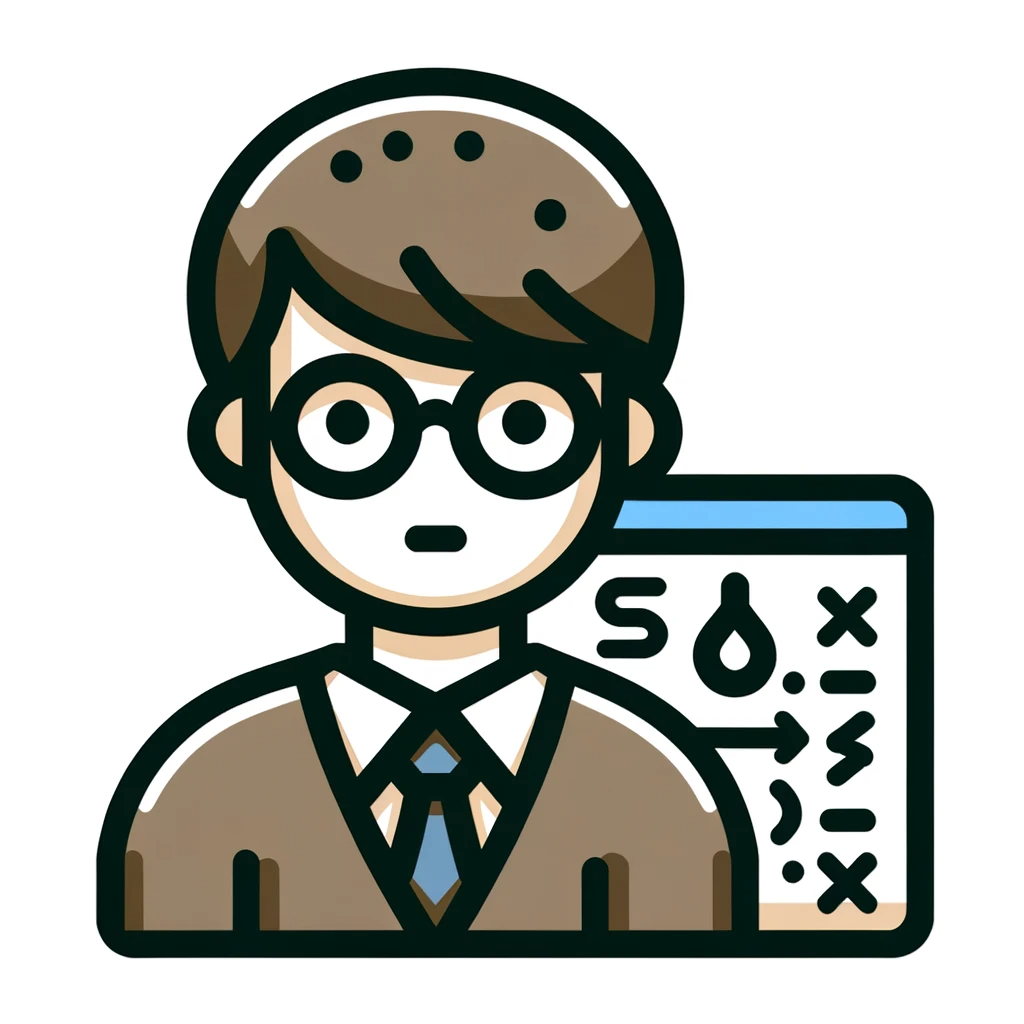
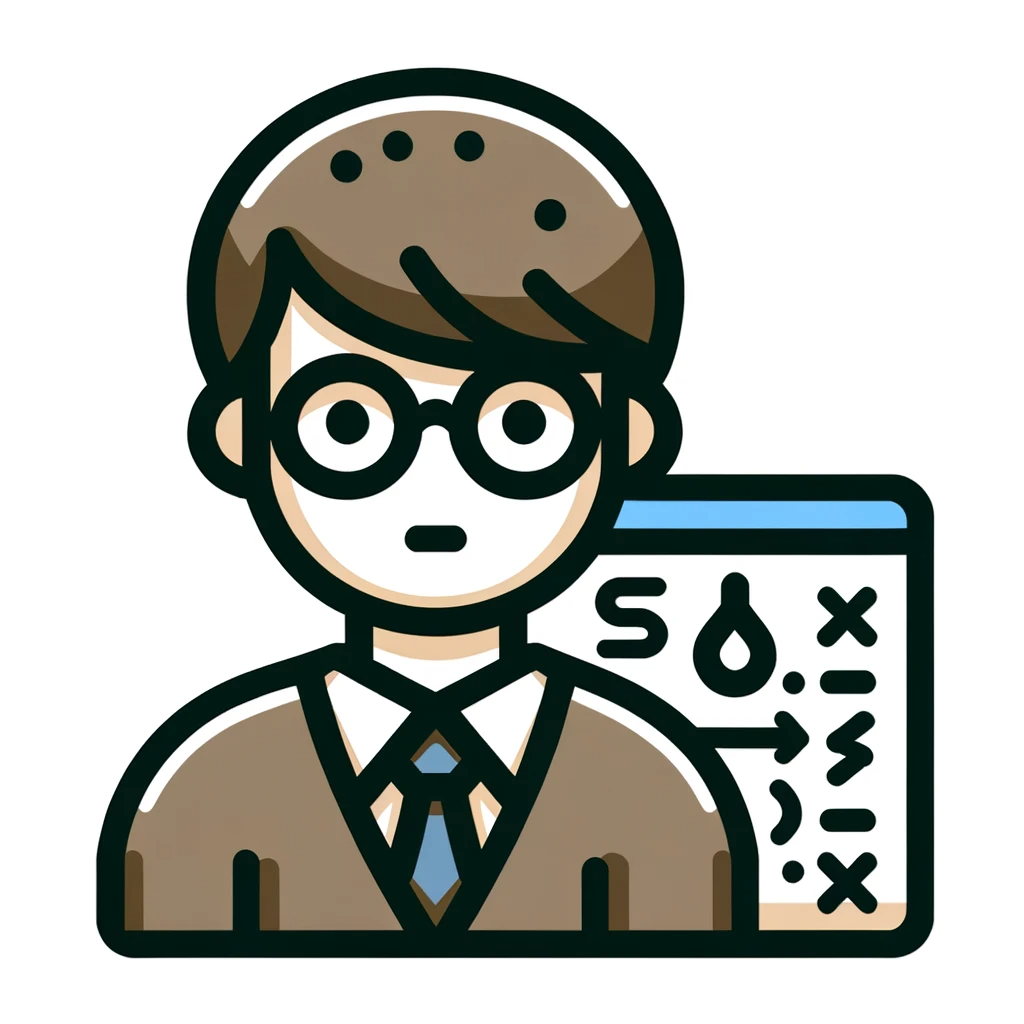
JavaScript is a language that allows you to easily perform various date manipulations.
This time we explained how to obtain a timestamp value from a date string, but you can also easily change the date format, add/subtract dates, etc.
Let’s get used to date manipulation in JavaScript.
Comments