This article explains how to obtain date and time for each element using JavaScript.
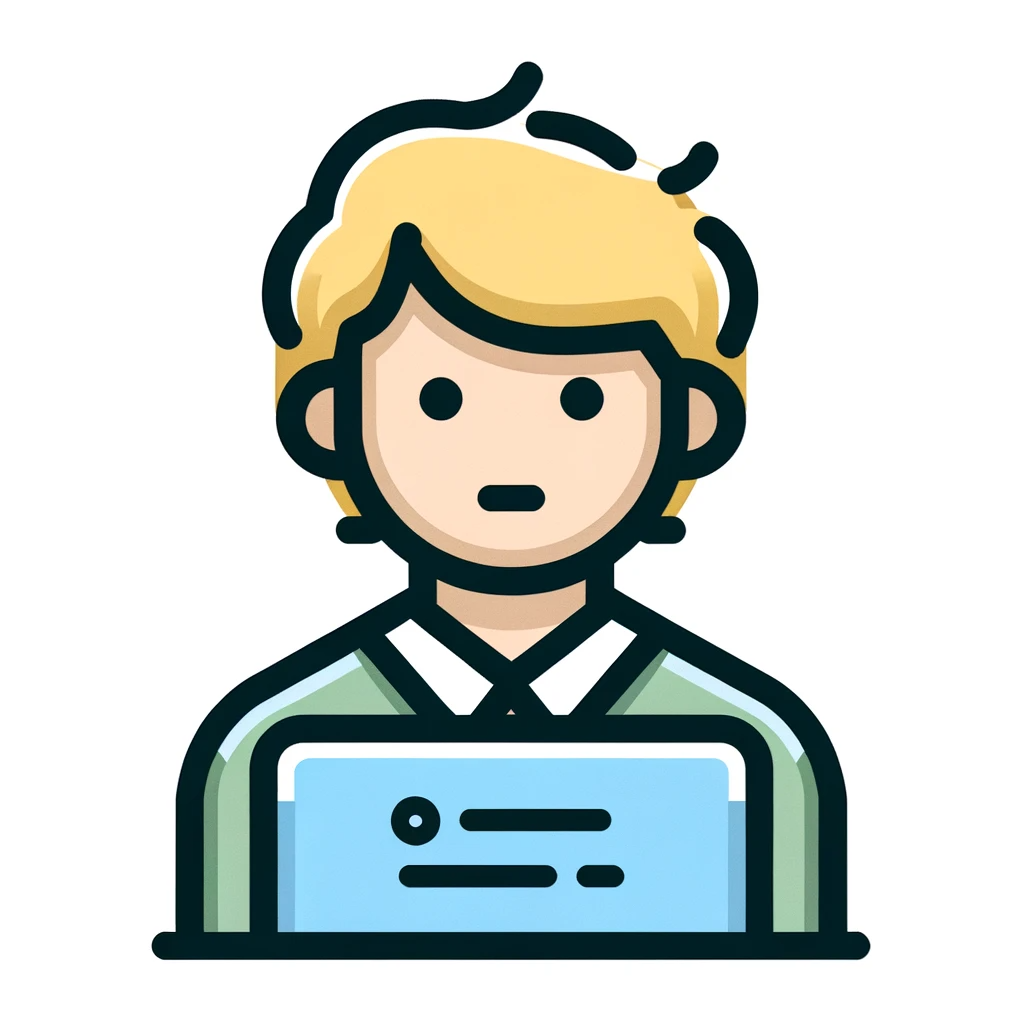
Is there a way to get the date and time by element?
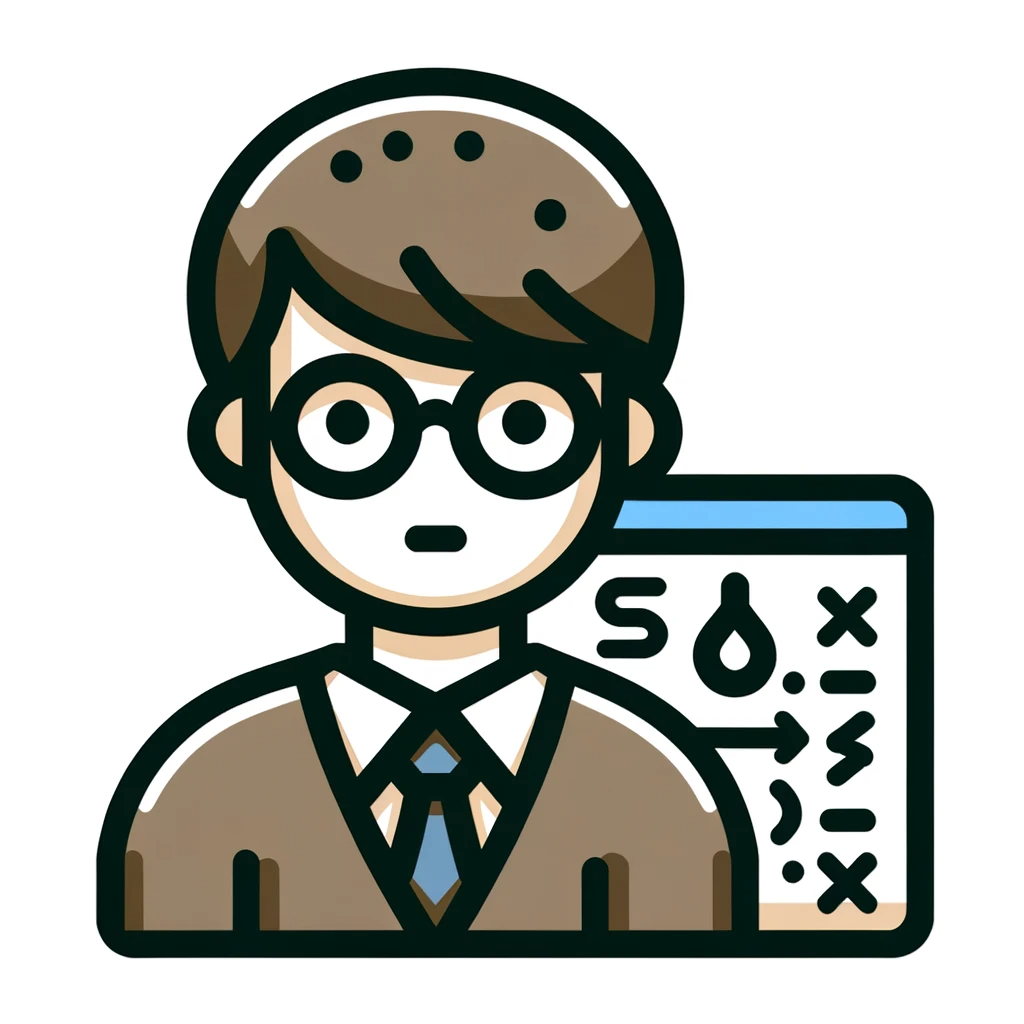
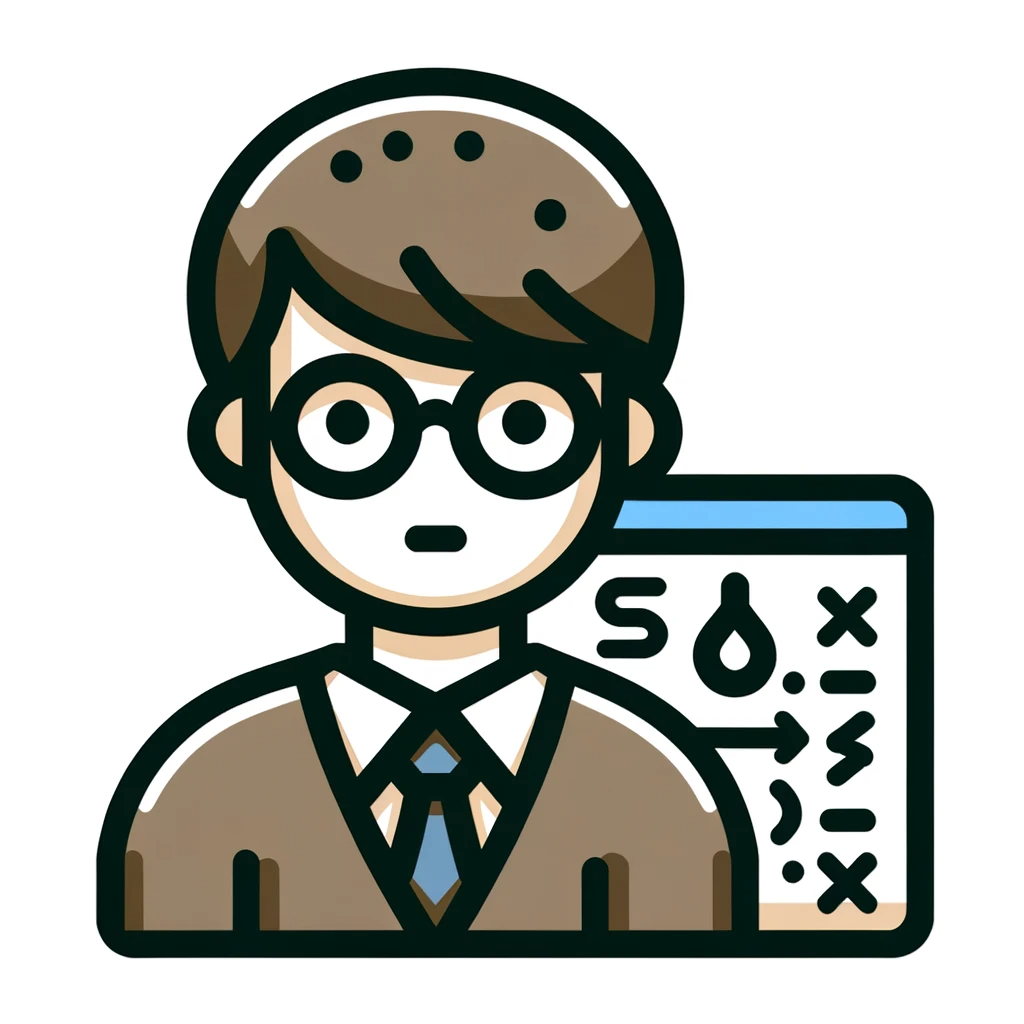
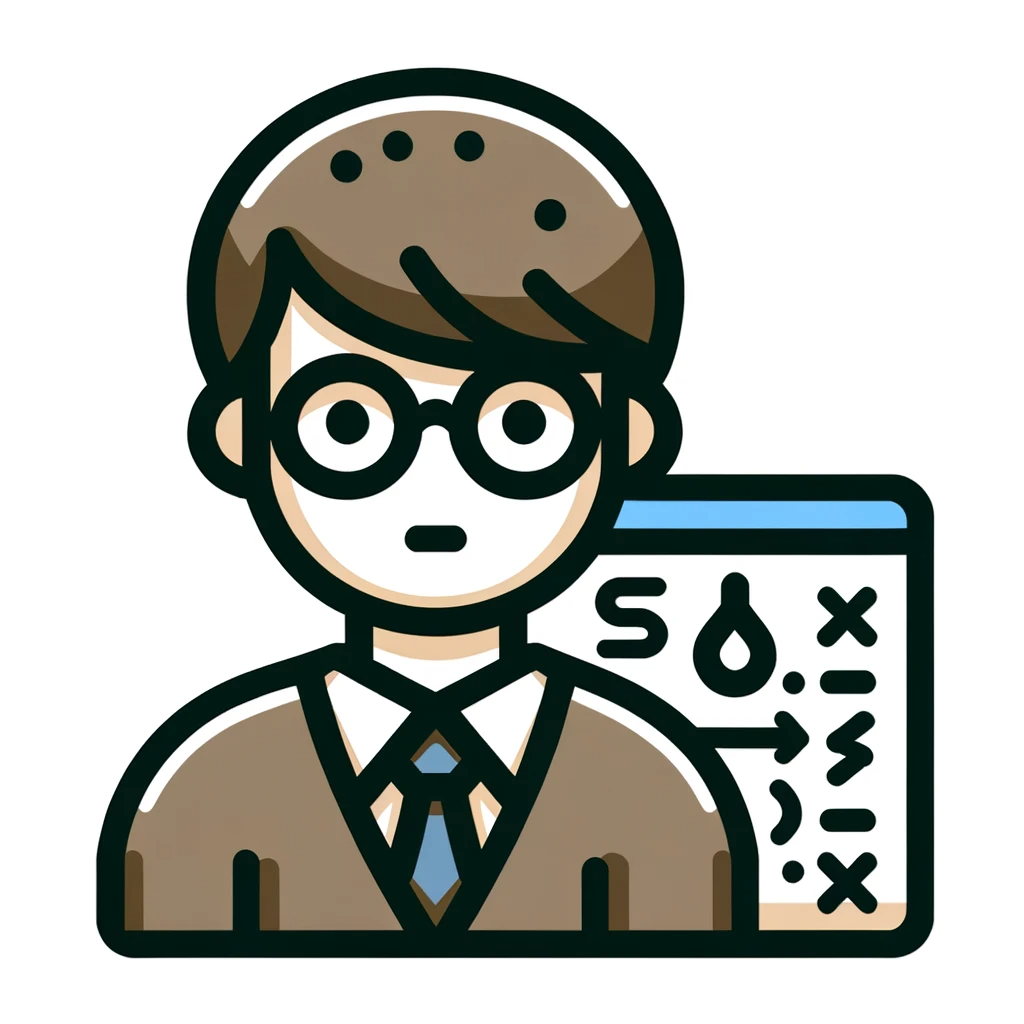
I have a Date object to get the date and time.
There are many methods available for retrieving date and time elements.
Method to get date and time elements
Date object is an object for handling date and time.
This object has a number of methods for retrieving date and time elements starting with “get”, as shown in the table below.
method | explanation |
---|---|
getFullYear | Gets the year as a 4-digit number. |
getMonth | Gets the month as a number from 0 to 11. |
getDate | Gets the day as a number from 1 to 31. |
getDay | Gets the day of the week as a number from 0 to 6. |
getHours | Gets the hour as a number from 0 to 23. |
getMinutes | Gets the minute as a number from 0 to 59. |
getSeconds | Gets the seconds as a number from 0 to 59. |
getMilliseconds | Gets the millisecond as a number from 0 to 999. |
getTime | Gets the elapsed time in milliseconds since January 1, 1970 00:00:00 UTC. |
getTimezoneOffset | Gets the difference in minutes between the current time zone and UTC (Coordinated Universal Time). |
Below are samples using these.
// Get present date and time
let now = new Date();
// Obtained a year
console.log(now.getFullYear()); // 2021
// Get Month (0 to 11)
console.log(now.getMonth()); // 1
// Retrieved on
console.log(now.getDate()); // 17
// Get day of the week (0:Sunday to 6:Saturday)
console.log(now.getDay()); // 1
// Retrieve the time
console.log(now.getHours()); // 11
// Get minutes
console.log(now.getMinutes()); // 33
// Get seconds
console.log(now.getSeconds()); // 5
// Get milliseconds
console.log(now.getMilliseconds()); // 123
// Get elapsed time since January 1, 1970 00:00:00 UTC
console.log(now.getTime()); // 1611031685123
// Get the difference between the current time zone and UTC (Coordinated Universal Time)
console.log(now.getTimezoneOffset()); // -540
summary
The Date object has many methods for retrieving the date and time element by element. These methods allow you to have fine-grained control over dates and times, and to determine time differences.
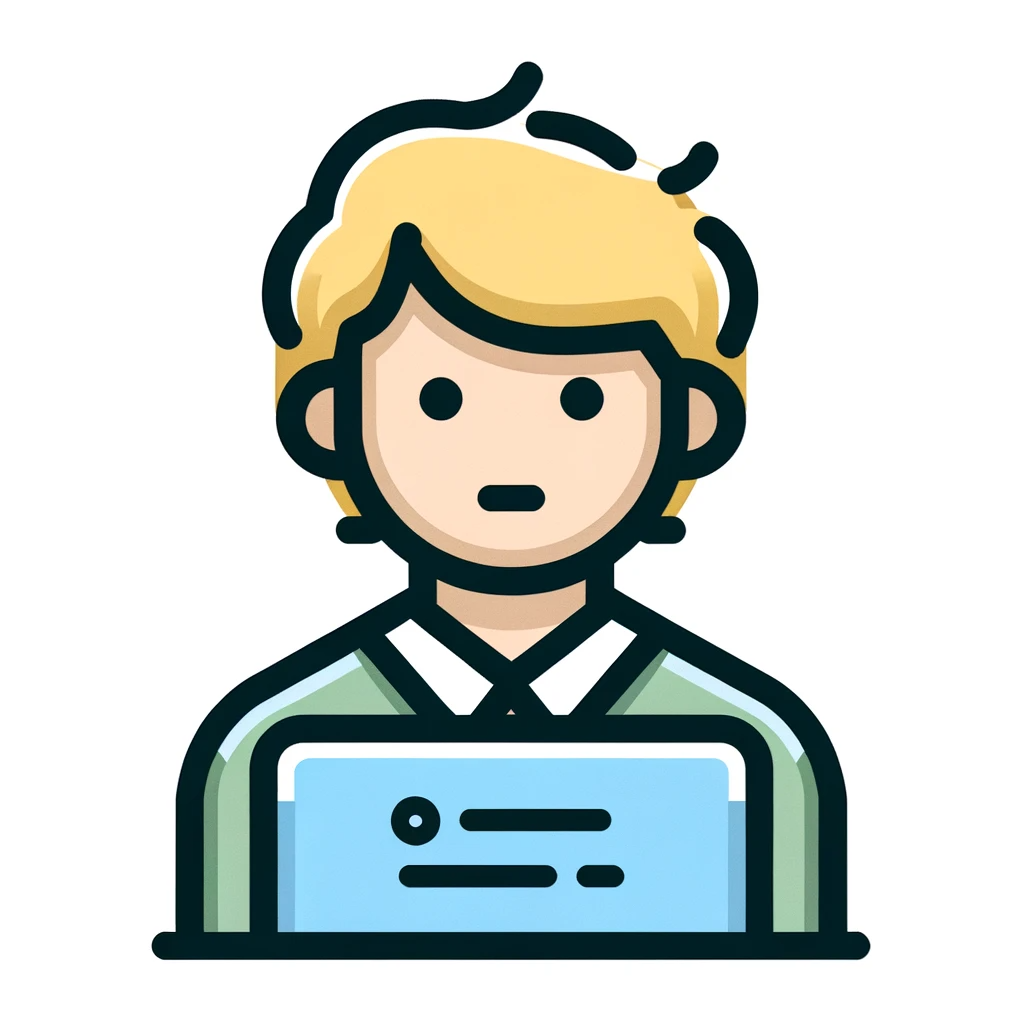
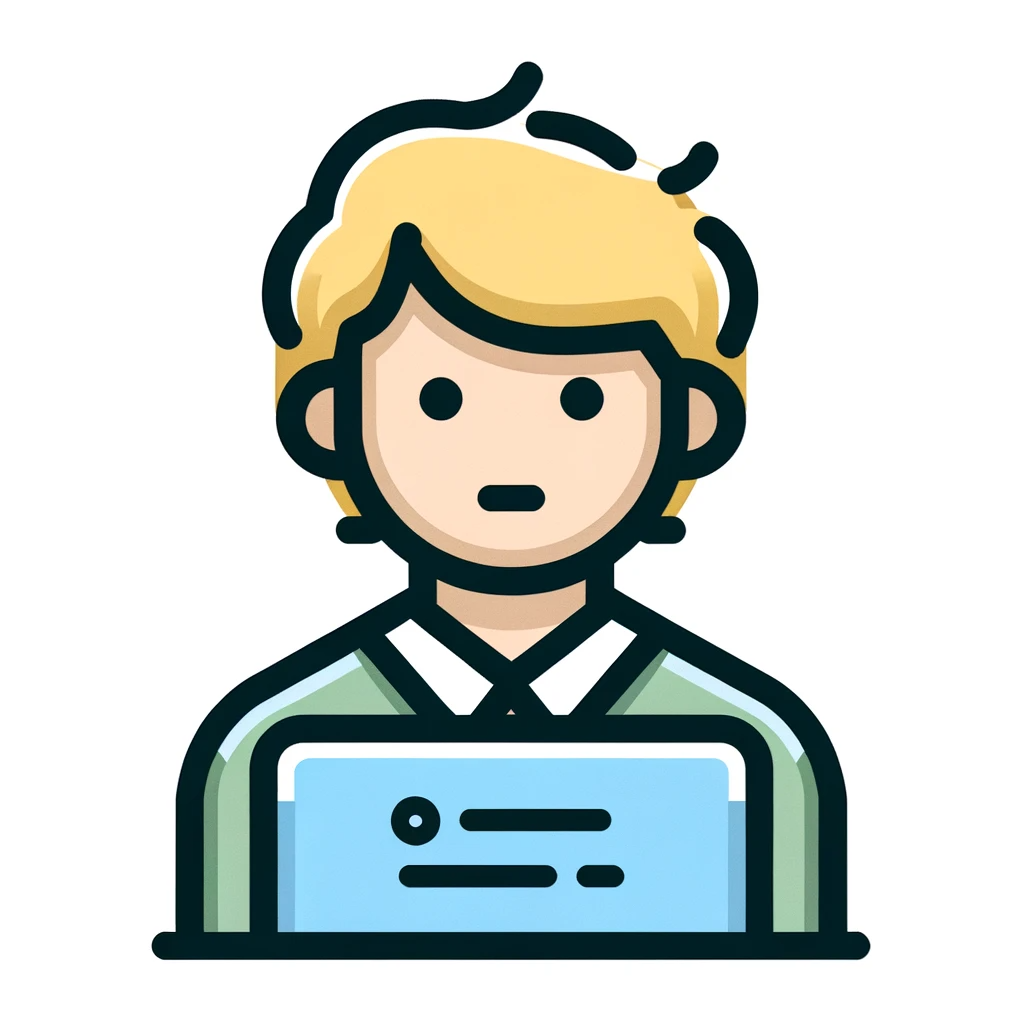
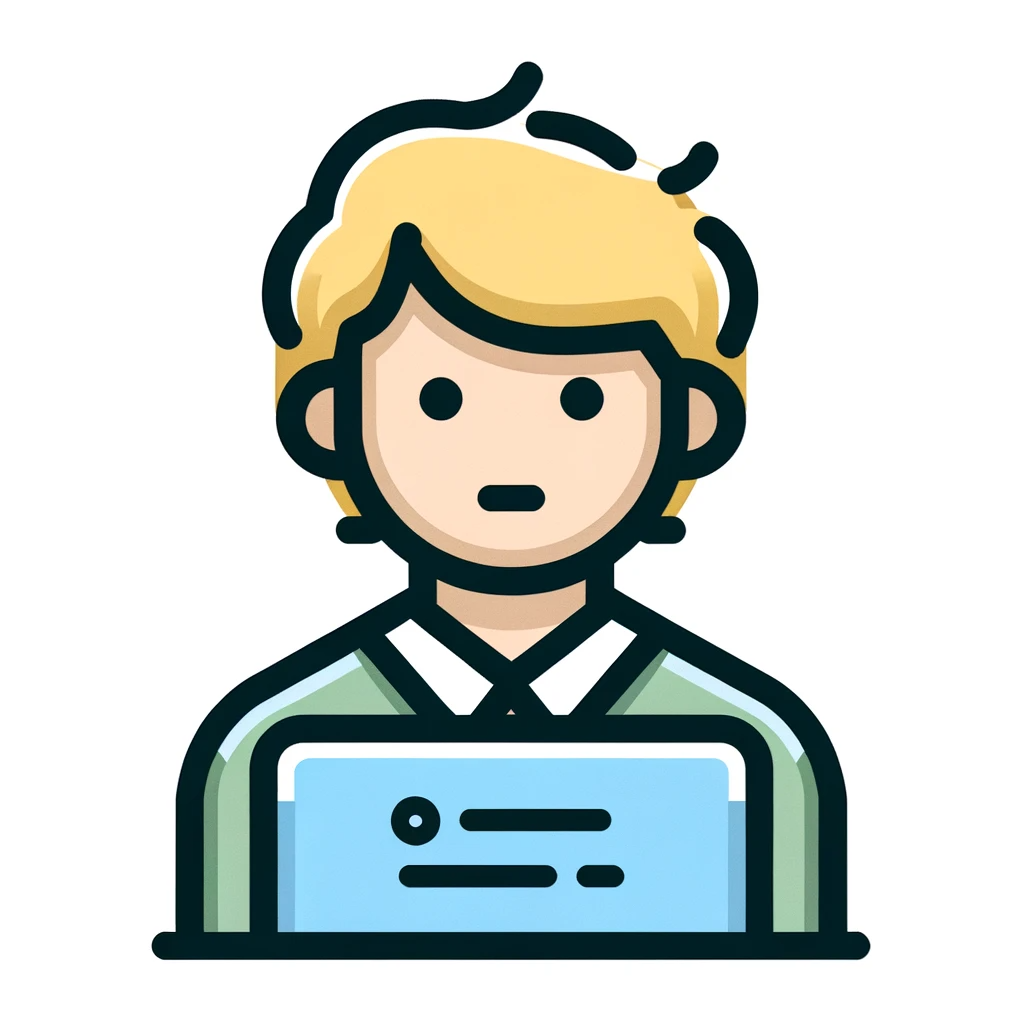
I now know how to get the date and time by element.
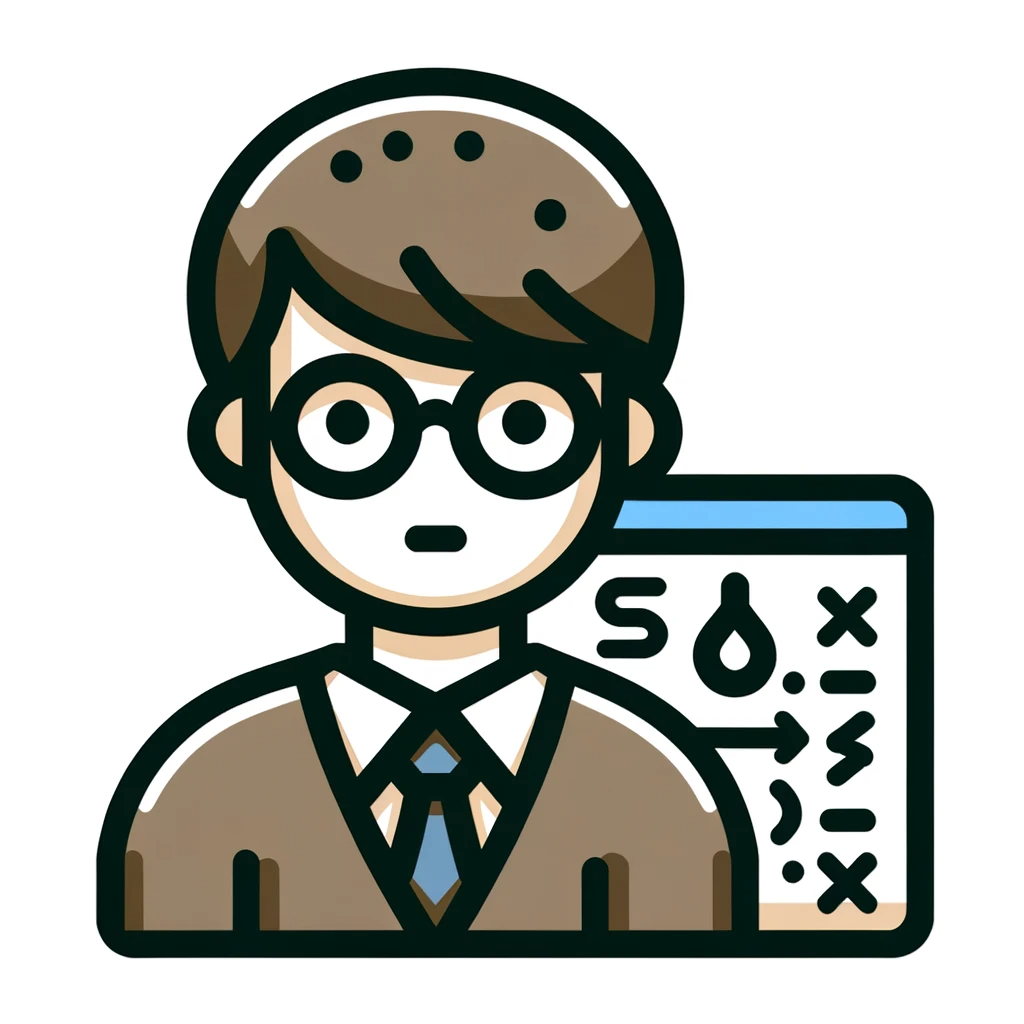
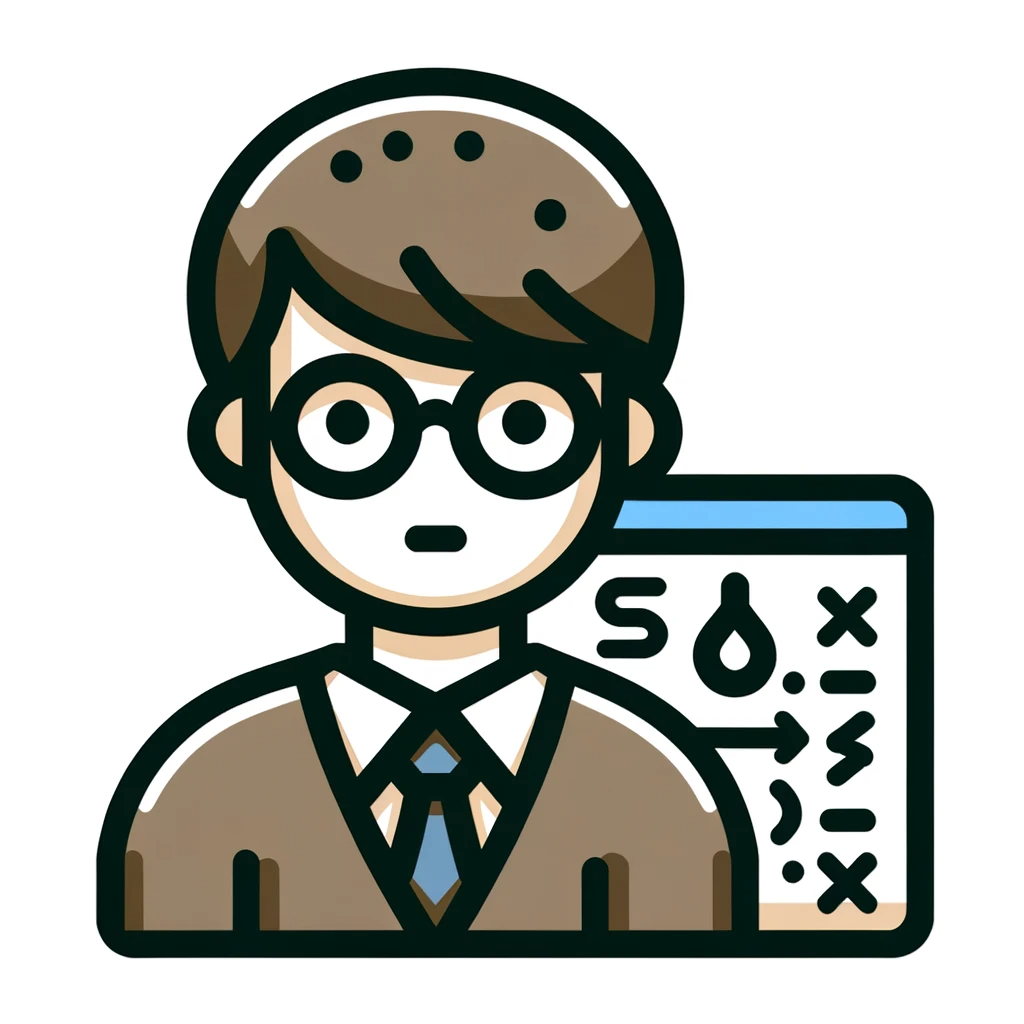
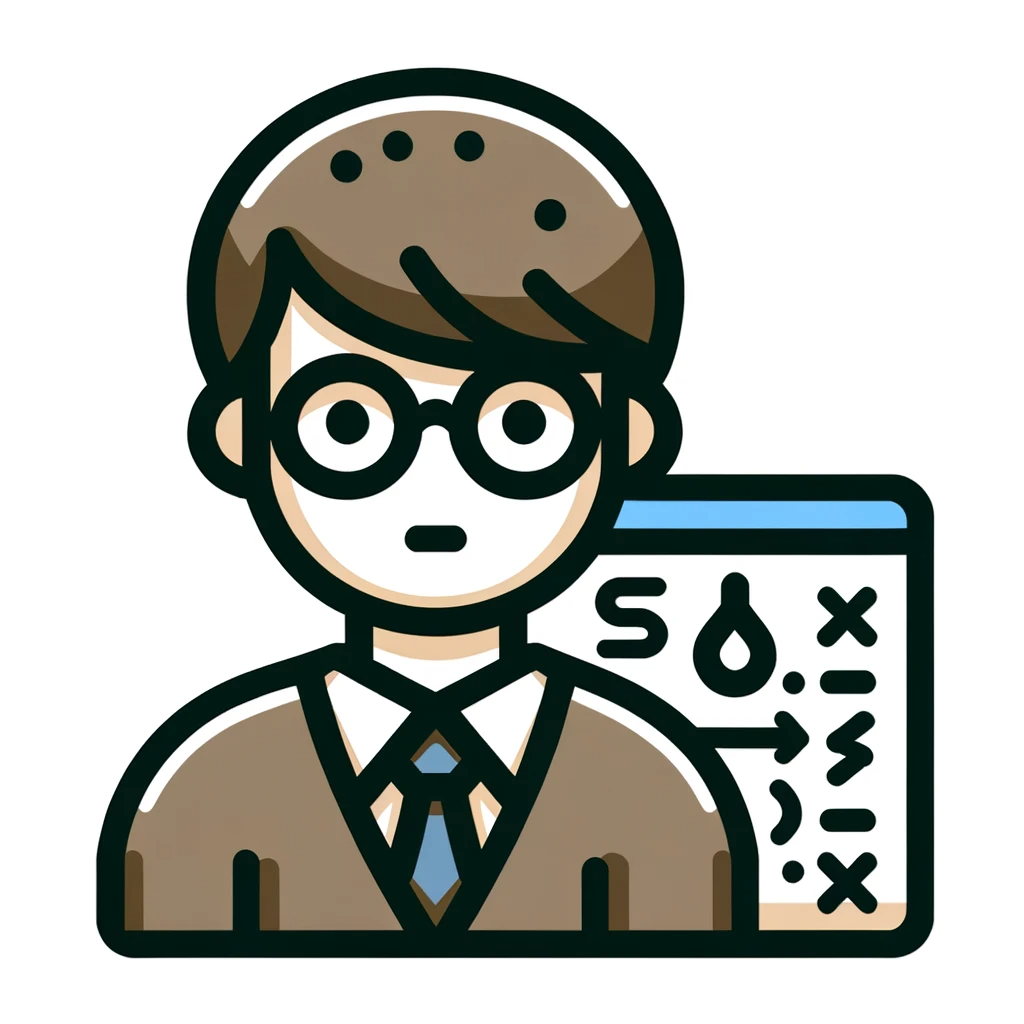
Please note that the results are returned when executed against an instance in UTC (Coordinated Universal Time), so if executed against a local date and time, the results may vary depending on the time zone.
Comments