This article explains in detail how to set, retrieve, delete, and update cookies using JavaScript.
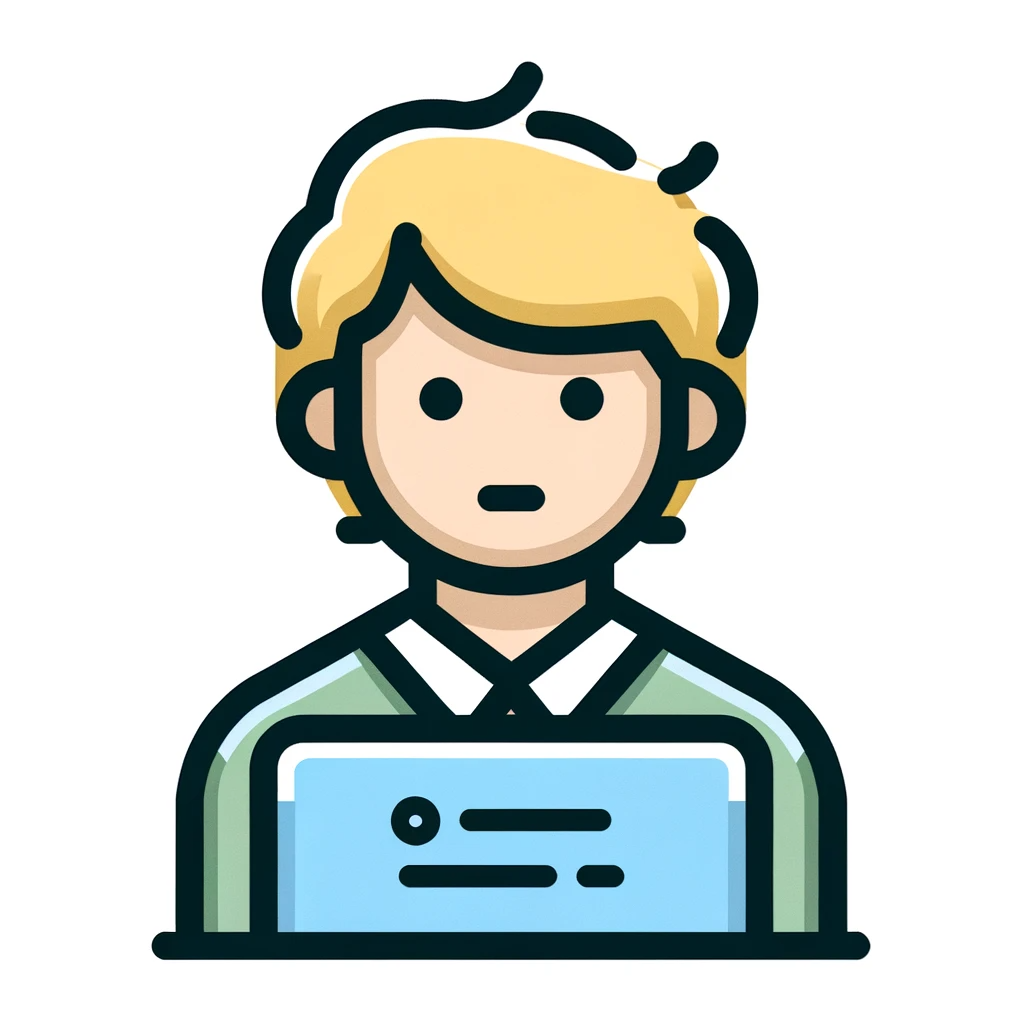
How do I set cookies?
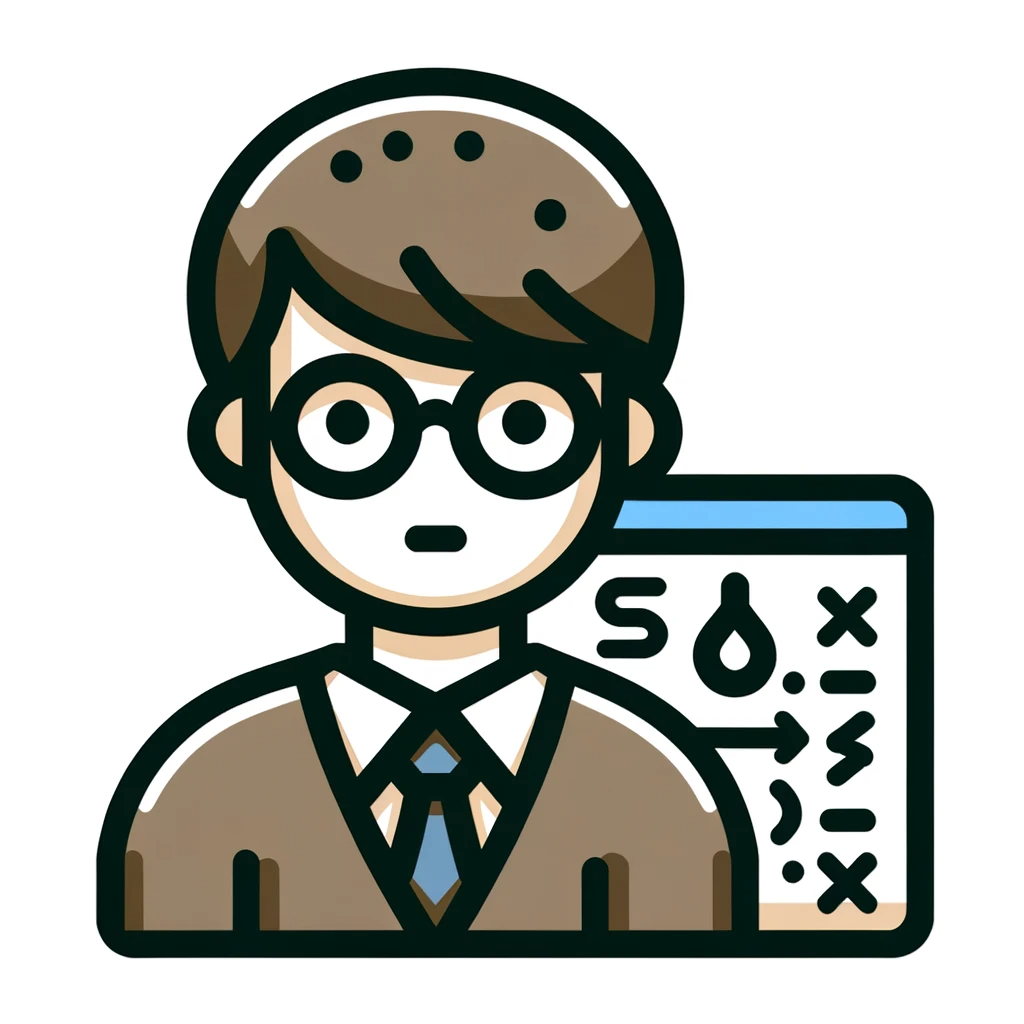
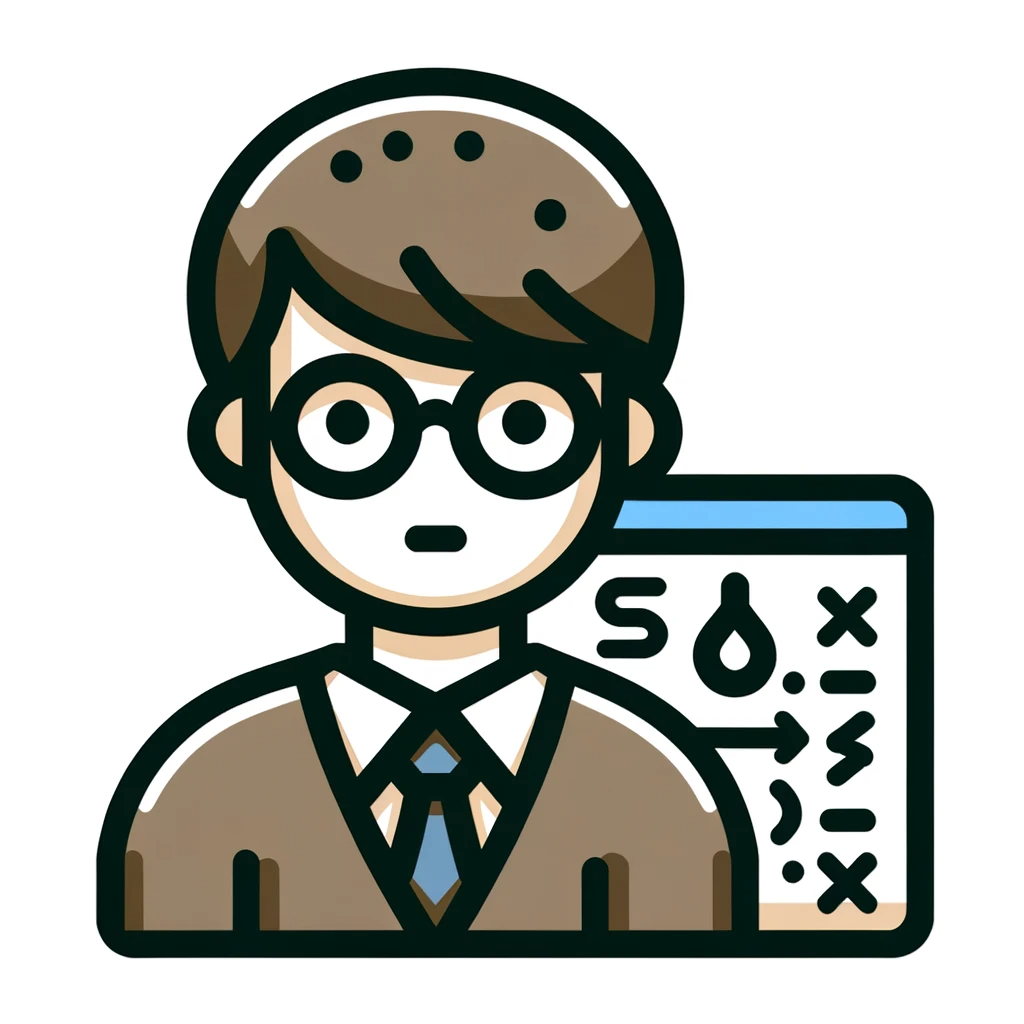
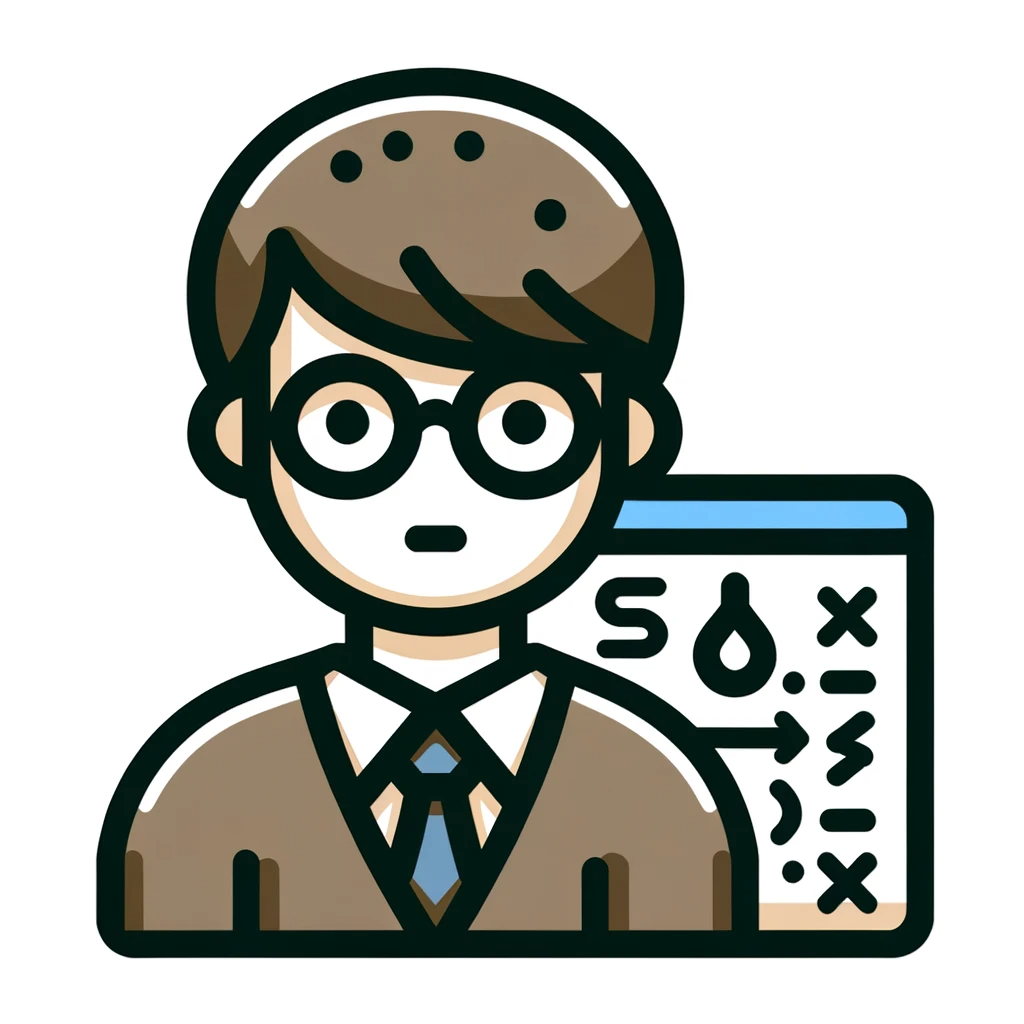
To set cookies, use the document.cookie property.
This article provides a detailed explanation of how to set, retrieve, delete, and update cookies using JavaScript.
What is a cookie?
Cookies are small text files that websites send to your browser and are stored on your device (computer or smartphone) . Cookies make your web browsing experience more convenient by allowing web pages to remember your preferences and settings so that you can refer to them later.
Main uses of cookies
The main uses of cookies include:
- Session management:
- Track and manage user actions on your website, such as login information and shopping cart contents.
- Personalization:
- Save your preferences and settings (e.g. language settings, theme, layout, etc.) and provide customized content.
- tracking:
- Track user behavior and history and use it for advertising and marketing activities.
Types of cookies
The types of cookies include the following:
- Session cookies:
- Session cookies last only while you are browsing the website and are automatically deleted when you close your browser. It is primarily used to hold temporary data such as login information.
- Persistent cookies:
- Persistent cookies remain stored on your device until the set period (e.g. 30 days) has elapsed. This allows users to retain their previous settings and status when they return to your website.
- Third party cookies:
- Third-party cookies are cookies that are issued by a domain other than the website you are visiting (e.g. an advertising company). This allows users’ browsing history and behavior to be tracked across multiple websites and used for targeted advertising and analysis.
When using cookies, please be aware of privacy protection and legal regulations and use them appropriately. It is important to properly explain the purposes and types of cookies used to users and obtain consent where necessary.
In particular, the General Data Protection Regulation (GDPR) applies in the EU, which has strict rules regarding the use of cookies. By implementing proper information and consent processes, you can respect user privacy and avoid legal issues.
What is the document.cookie property?
The document.cookie property is a property that allows you to access and manipulate cookies on web pages using JavaScript.
This allows website developers to read, create, update, and delete cookies . By using this property, you can implement various functions such as managing the state of the web page and saving user settings.
Main characteristics of document.cookie property
Below are the main characteristics of the document.cookie property.
- reading:
- You can retrieve all cookies associated with a web page in the form of a semicolon-separated string by accessing the document.cookie property.
- Create/Update:
- You can create new cookies or update existing cookies by assigning a string to the document.cookie property. You can specify information such as cookie name, value, expiration date, path, domain, and secure attributes.
- delete:
- To delete a cookie, assign cookie information with a past expiration date to the document.cookie property. This will cause your browser to disable and delete cookies.
- The document.cookie property cannot access cookies with the HTTP-only attribute set. Cookies with the HTTP-only attribute are used only for communication between the browser and the server, and access via JavaScript is restricted. This helps reduce security risks such as cross-site scripting (XSS) attacks.
- Please be aware of privacy protection and legal regulations when using cookies, and use them appropriately. It is important to properly explain the purposes and types of cookies used to users and obtain consent where necessary.
Key functions that control cookies
The table below summarizes the main functions for controlling cookies .
function name | argument | function | Example of use |
---|---|---|---|
setCookie | name, value, days | Set cookies | setCookie(‘username’, ‘JohnDoe’, 7) |
getCookie | name | Get cookie value | let username = getCookie(‘username’) |
deleteCookie | name | Delete cookies | deleteCookie(‘username’) |
updateCookie | name, newValue, newDays (optional) | Update cookies (set new value and expiration date) | updateCookie(‘username’, ‘JaneDoe’) |
An example implementation of each function is shown below.
// Function to set cookies
function setCookie(name, value, days) {
let date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
let expires = "; expires=" + date.toUTCString();
document.cookie = name + "=" + value + expires + "; path=/";
}
// Function to retrieve cookies
function getCookie(name) {
let cookieName = name + "=";
let decodedCookie = decodeURIComponent(document.cookie);
let cookies = decodedCookie.split(';');
for (let i = 0; i < cookies.length; i++) {
let cookie = cookies[i].trim();
if (cookie.indexOf(cookieName) === 0) {
return cookie.substring(cookieName.length, cookie.length);
}
}
return "";
}
// Function to delete cookies
function deleteCookie(name) {
setCookie(name, "", -1);
}
// Function to update cookies
function updateCookie(name, newValue, newDays = null) {
let existingCookieValue = getCookie(name);
if (existingCookieValue !== "") {
let days = newDays ? newDays : getCookieDays(name);
setCookie(name, newValue, days);
}
}
// Auxiliary function to get the cookie expiration date (in days)
function getCookieDays(name) {
// This function is used to retrieve the expiration date when the cookie is created.
// Add implementations as needed.
}
Explanation using a sample program: Creating, reading, and deleting cookies
The following sample program illustrates how to create, read, and delete cookies using the document.cookie property of JavaScript.
// Function to set cookies
function setCookie(name, value, days) {
let date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
let expires = "; expires=" + date.toUTCString();
document.cookie = name + "=" + value + expires + "; path=/";
}
// Function to retrieve cookies
function getCookie(name) {
let cookieName = name + "=";
let decodedCookie = decodeURIComponent(document.cookie);
let cookies = decodedCookie.split(';');
for (let i = 0; i < cookies.length; i++) {
let cookie = cookies[i].trim();
if (cookie.indexOf(cookieName) === 0) {
return cookie.substring(cookieName.length, cookie.length);
}
}
return "";
}
// Function to delete cookies
function deleteCookie(name) {
setCookie(name, "", -1);
}
// Example of setting a cookie
setCookie("username", "JohnDoe", 7);
// Example of Retrieving Cookies
let username = getCookie("username");
console.log("username: " + username);
// Example of deleting a cookie
deleteCookie("username");
The setCookie function takes a cookie name, value, and expiration date (in days) as arguments and assigns them to the document.cookie property to create a cookie.
The getCookie function takes the name of the cookie as an argument and retrieves the value of the corresponding cookie from the document.cookie property. If the cookie is not found, an empty string is returned.
The deleteCookie function takes the name of the cookie as an argument and deletes the cookie with a past expiration date. This is accomplished by using the setCookie function to set the expiration date to -1 day.
How to check cookies in Chrome: Check cookies using developer tools
To check cookies on the Google Chrome browser, use developer tools (DevTools). The steps are explained below.
- Open developer tools:
- With a webpage open, press the keyboard shortcut
Ctrl + Shift + I
(Windows) orCmd + Opt + I
(Mac) or click the menu icon (three dots) in the top right corner and select More Tools > Developer Tools to develop. Open the user tool.
- With a webpage open, press the keyboard shortcut
- Go to Application tab:
- Click the Application tab from the tabs at the top of the developer tools. If the tab is not visible, click the >> icon and select Application from the menu that appears.
- Check cookies:
- Expand the Storage section in the left sidebar and click Cookies to open the submenu. You can then
https://example.com
click on a domain name (e.g. ) to see a list of cookies associated with that domain. This list provides details such as cookie name, value, domain, path, expiration date, size, secure attribute, and HTTP-only attribute.
- Expand the Storage section in the left sidebar and click Cookies to open the submenu. You can then
- Delete/edit cookies (optional):
- If you wish, you can also delete or edit cookies in the displayed cookie list. To delete a cookie, select it and
Delete
press the key. You can also edit information such as cookie values and expiration dates by double-clicking the cell to enter edit mode, making changes, and then pressing the key toEnter
save.
- If you wish, you can also delete or edit cookies in the displayed cookie list. To delete a cookie, select it and
You can view and manipulate cookies in your Chrome browser by following these steps. Developer tools provide powerful features to help you debug and analyze web pages, and can be used for a variety of purposes beyond cookie management.
summary
We explained how to set, retrieve, delete, and update cookies.
- Cookies can be set, retrieved, and deleted using the JavaScript document.cookie property.
- Cookies help manage the state of web pages and save user settings.
- It is important to pay attention to privacy protection and legal regulations and use it appropriately.
- To check cookies in Chrome, use the “Application” tab in developer tools.
- You can also edit and delete cookies using developer tools.
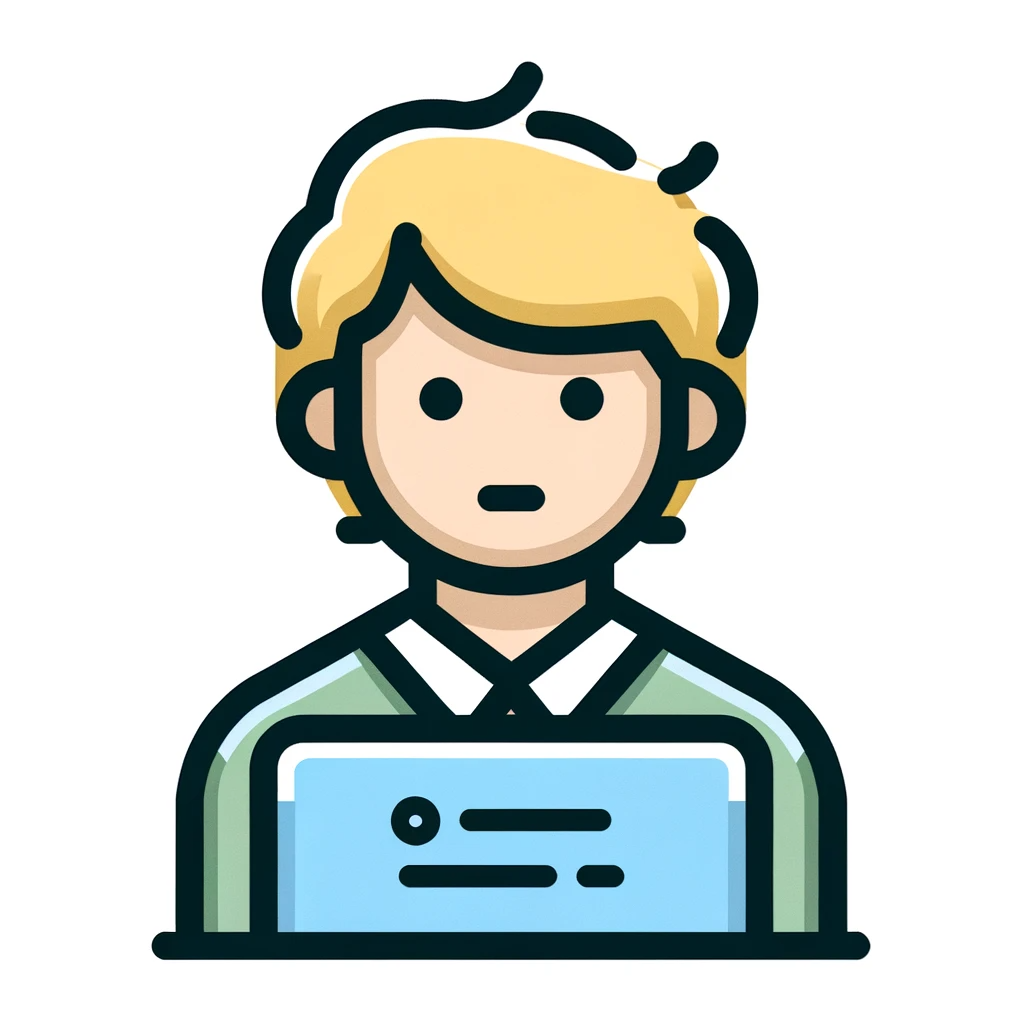
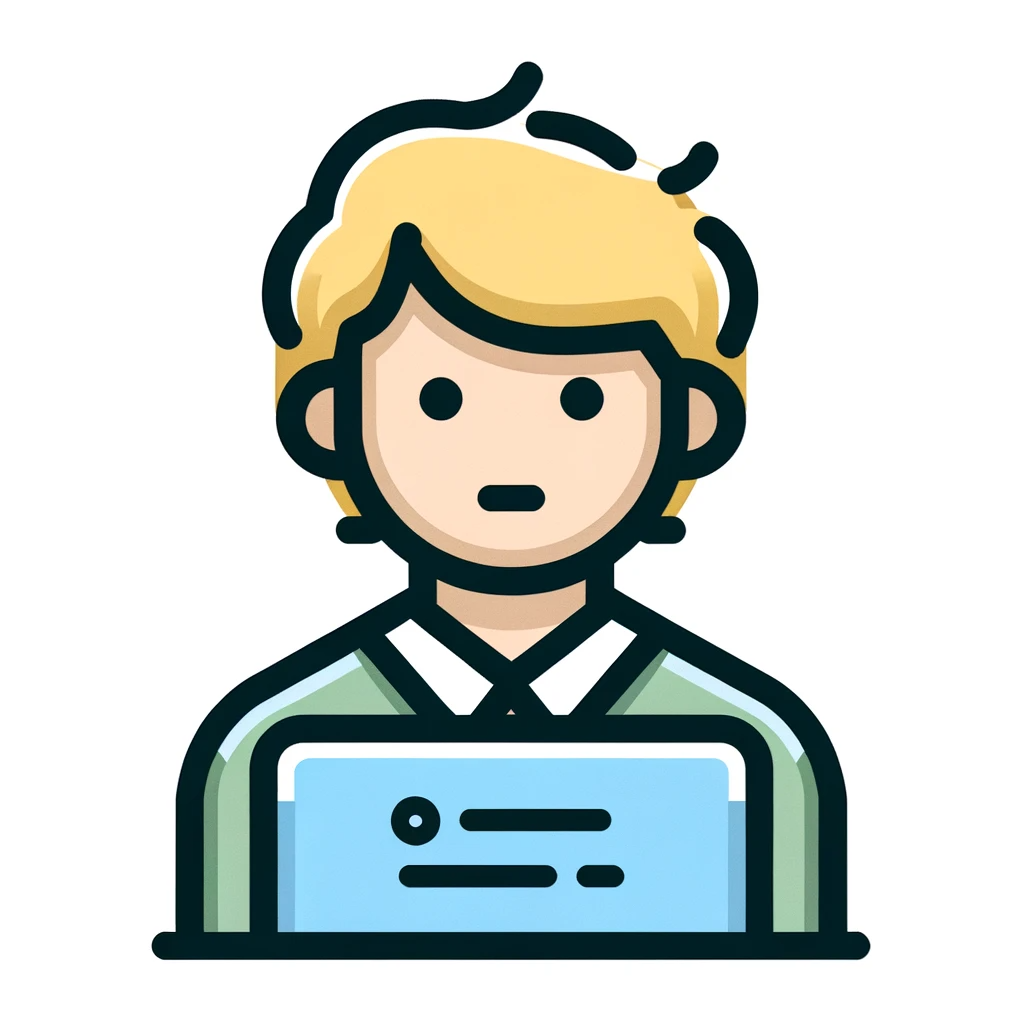
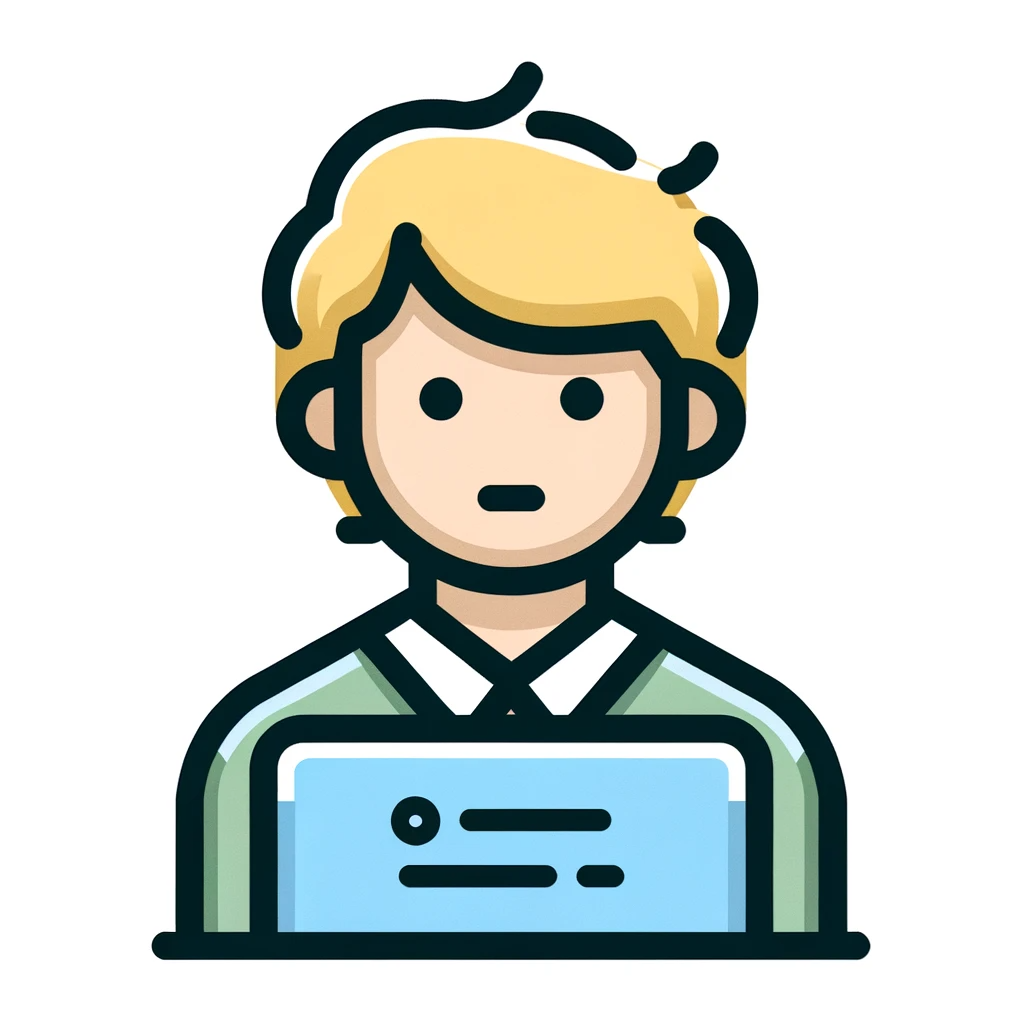
I was able to easily understand how to set and check cookies.
I would like to use it for web development in the future.
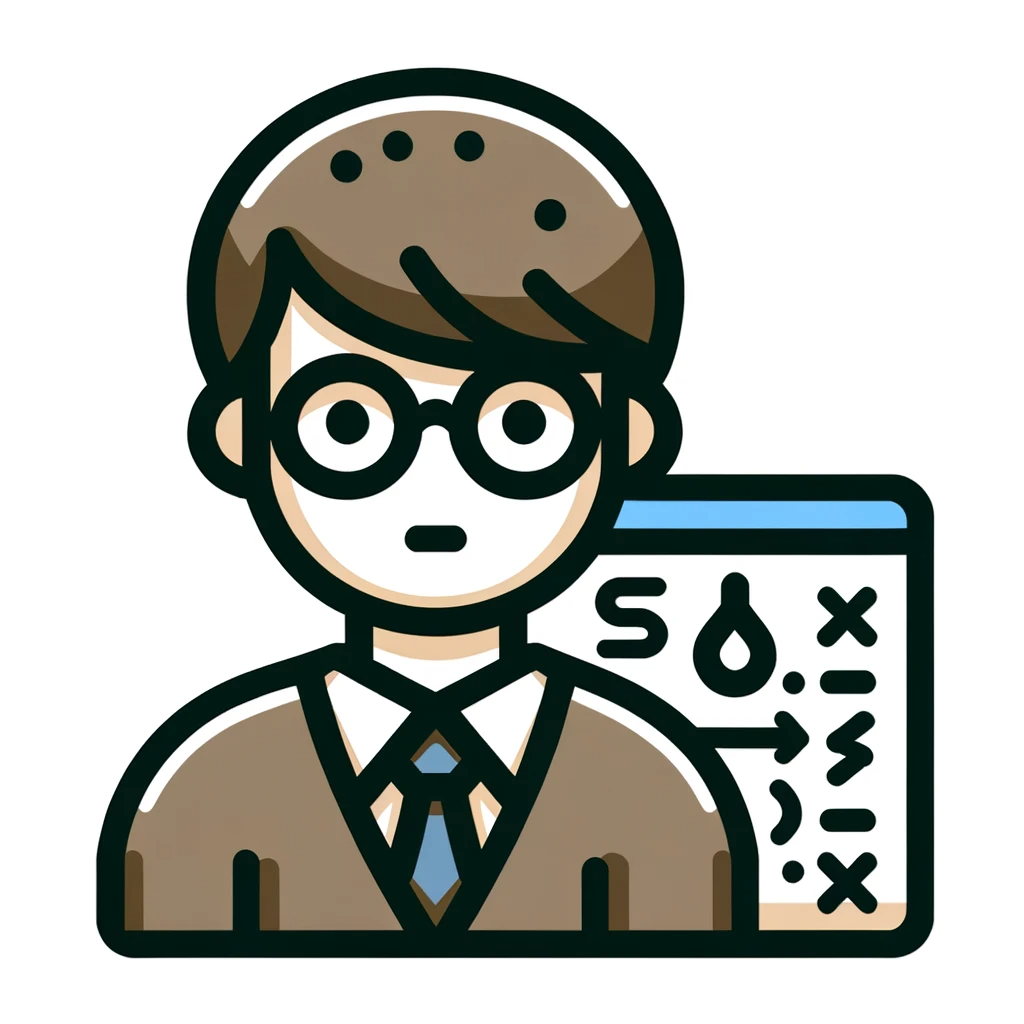
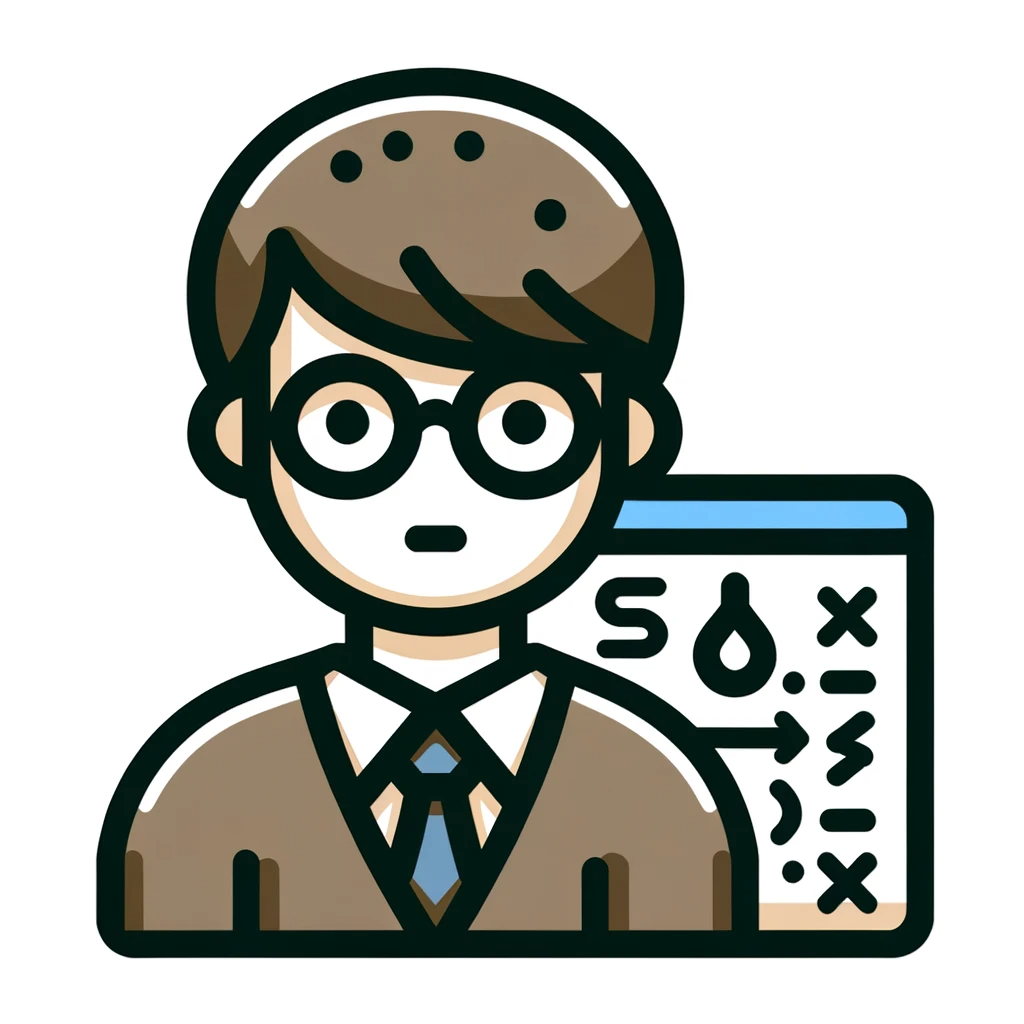
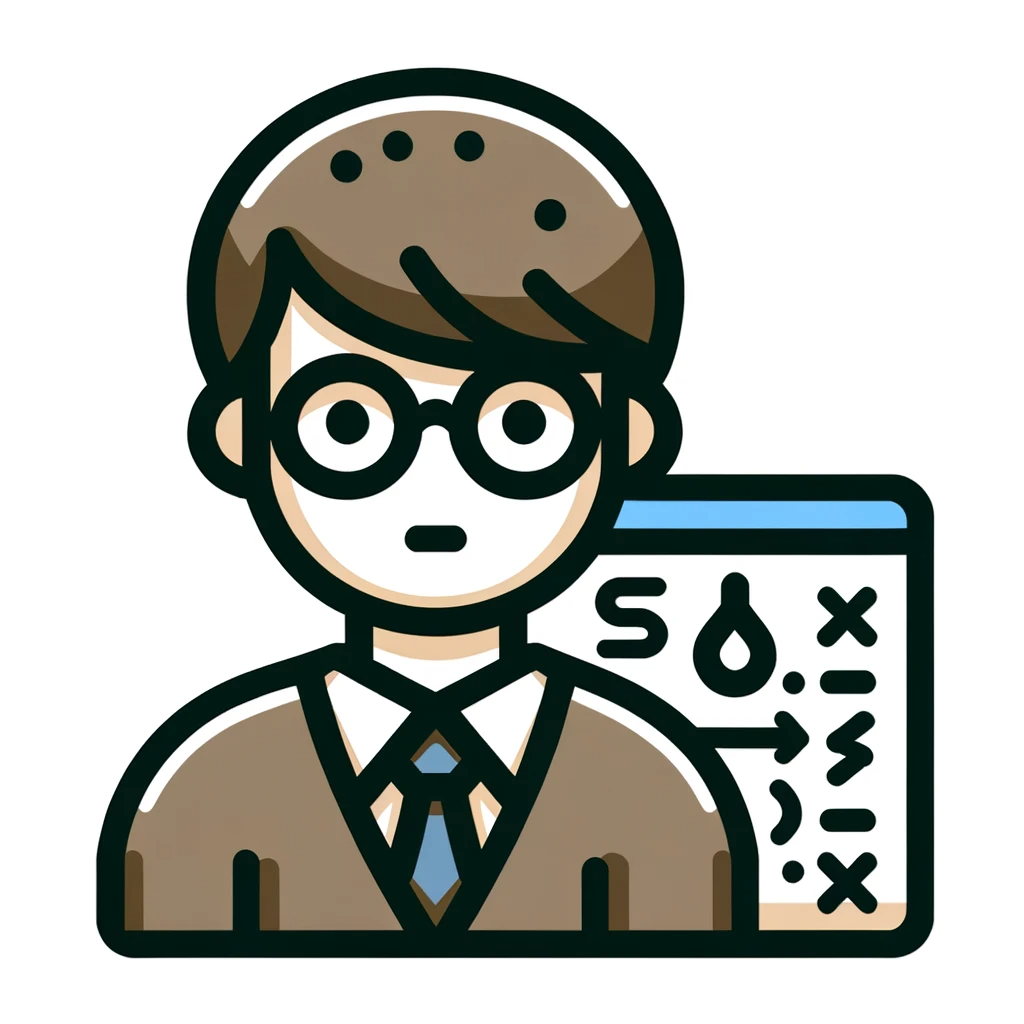
Cookies are a very useful feature in web development, but they should be used with consideration for user privacy and legal restrictions.
In addition to the document.cookie property, there are libraries and new technologies (e.g., Web Storage API) that allow for more secure and flexible cookie operations, and we encourage you to consider these as well. We hope you find them useful for your future web development.
Comments