We will explain debugging using the console, which is one of the debugging methods in JavaScript. It can be used in a variety of ways, including outputting logs, displaying objects, and measuring execution time.
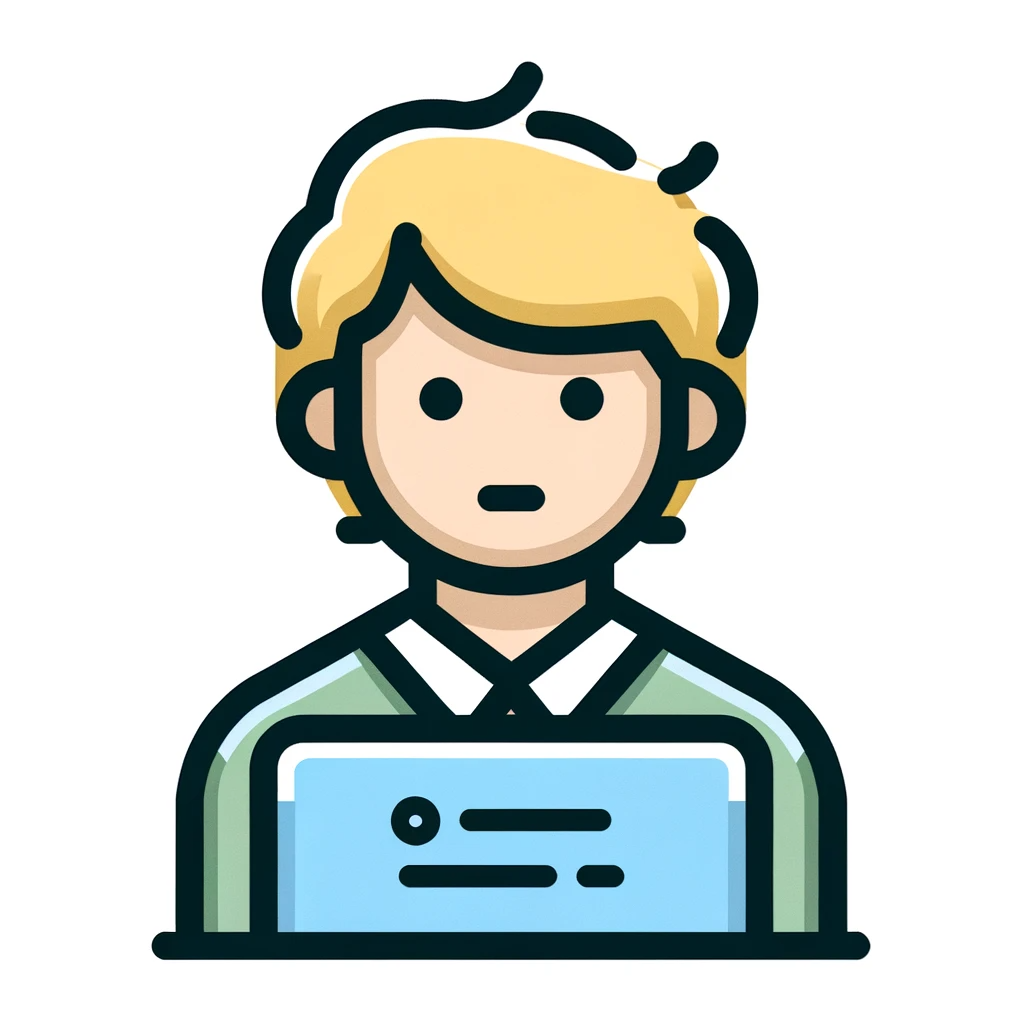
What can I do with debugging using the console?
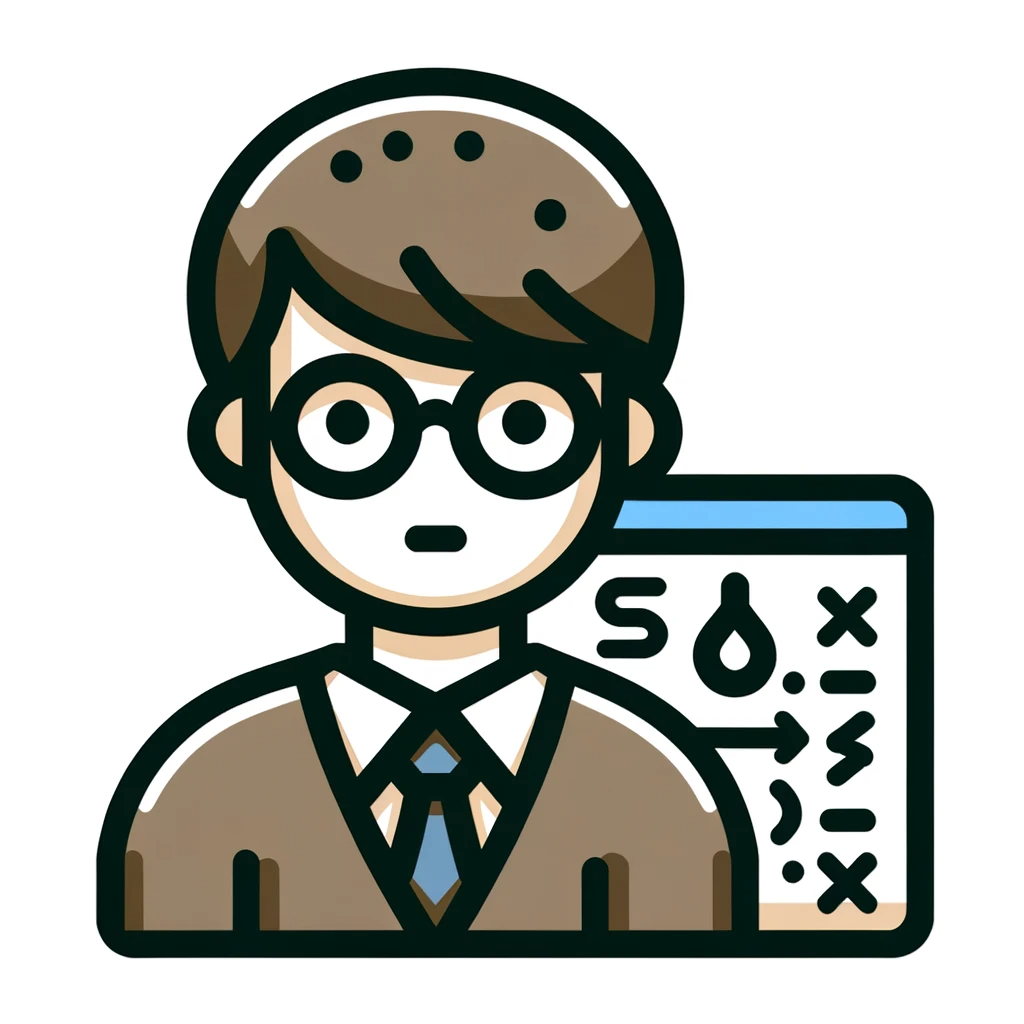
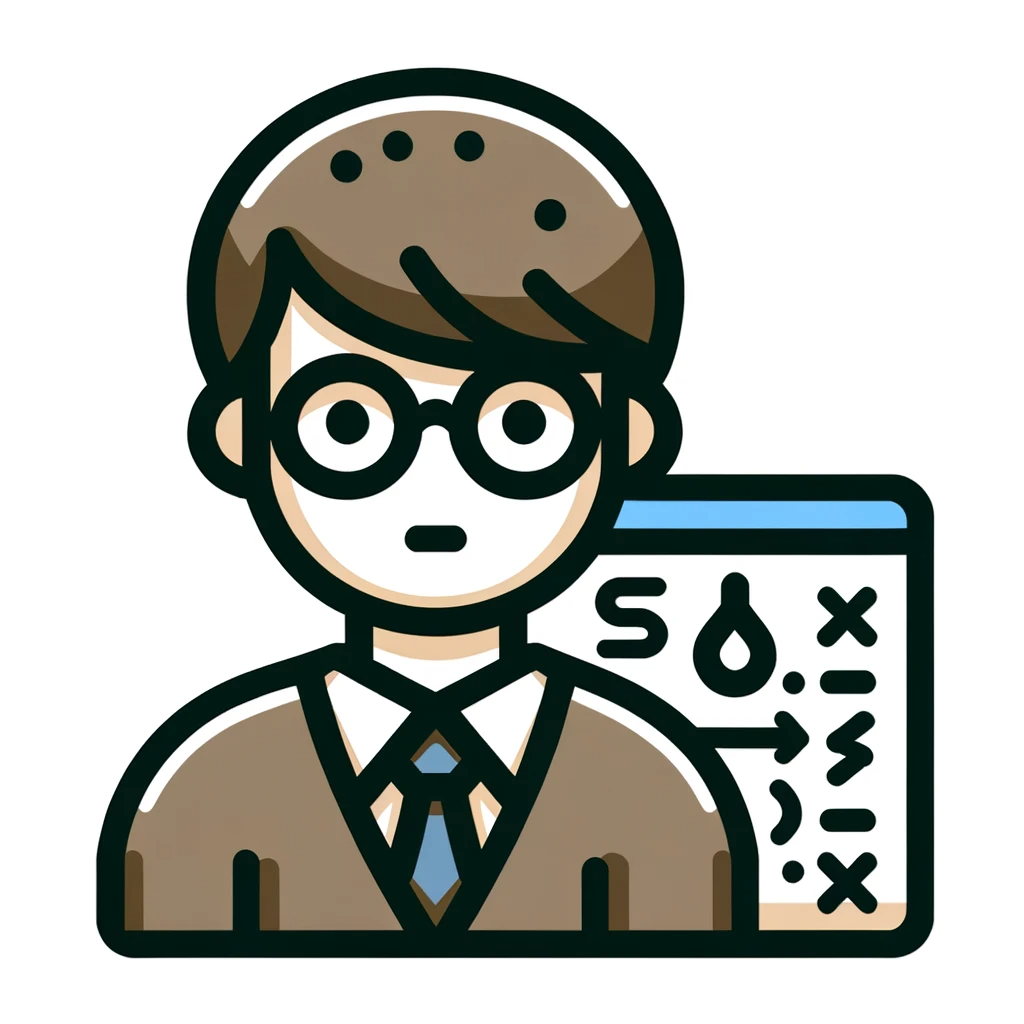
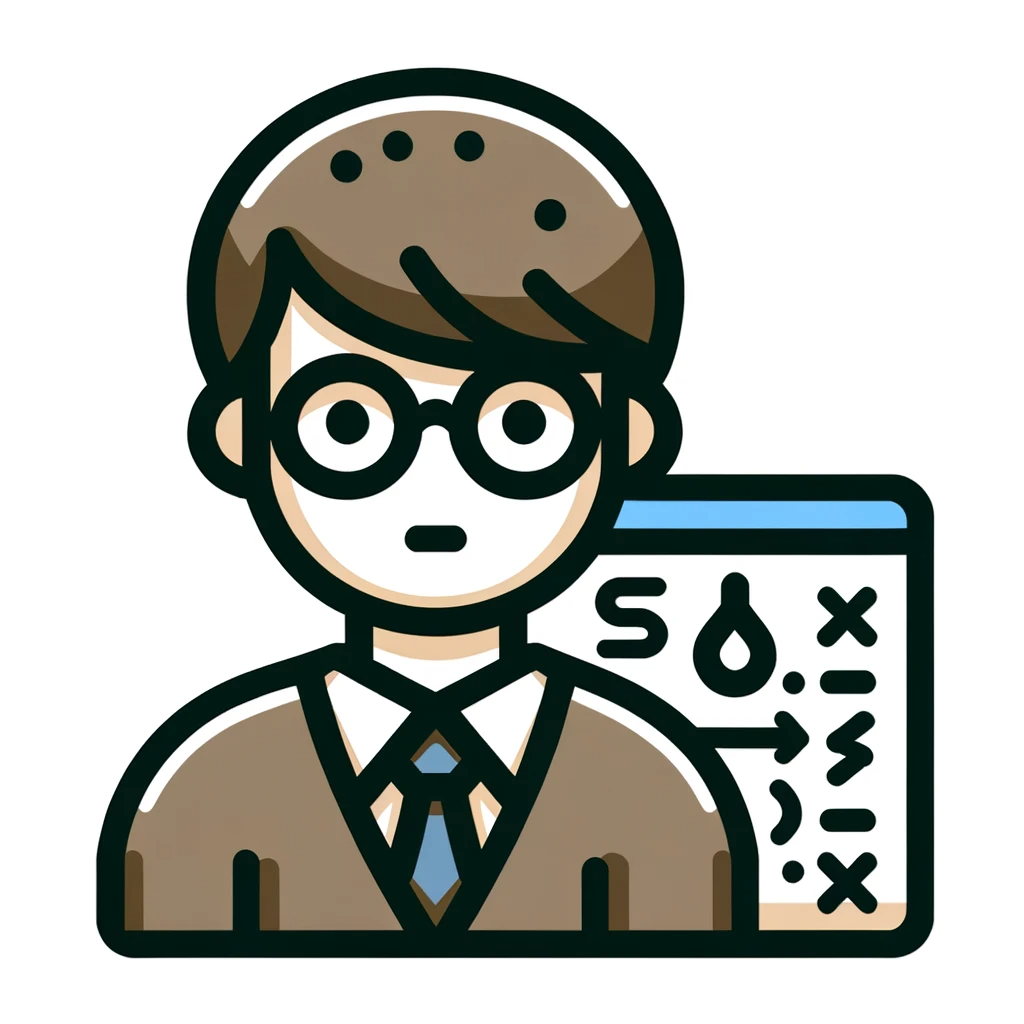
You can output logs, display objects, measure execution time, etc.
How to output logs to the console
In JavaScript, you can use the console object to output logs to the console. The main methods of the console object are explained below.
Normal output: console.log()
The console.log() method outputs the value passed as an argument to the console as a string. For example, you can use:
console.log('Hello, world!');
In this case, the console displays the string “Hello, world!”.
Information output: console.info()
The console.info() method outputs the value passed as an argument to the console as information. For example, you can use:
console.info('This is an information message.');
In this case, the console displays the string “This is an information message.” As with the console.log() method, you can pass not only strings but also objects and arrays as arguments.
Warning output: console.warn()
The console.warn() method prints the value passed as an argument to the console as a warning. For example, you can use:
console.warn('This is a warning message.');
In this case, the console displays the string “This is a warning message.” with a yellow background.
Error output: console.error()
The console.error() method prints the value passed as an argument to the console as an error. For example, you can use:
console.error('This is an error message.');
In this case, the console displays the string “This is an error message.” with a red background.
Debug information output: console.debug()
The console.debug() method outputs the value passed as an argument to the console as debugging information. Although similar to the console.info() method, the console.debug() method may be ignored by some browsers.
How to display objects in the console
In JavaScript, you can use the console object to print objects to the console. The following explains how to display objects using the console object.
Directory format: console.dir()
The console.dir() method displays the properties and methods of the object passed as an argument in directory format. For example, you can use:
const obj = {name: 'John', age: 25, gender: 'Male'};
console.dir(obj);
In this case, the console will display something like this:
Object
age: 25
gender: "Male"
name: "John"
__proto__: Object
XML format: console.dirxml()
The console.dirxml() method displays the object passed as an argument in XML format. For example, you can use:
console.dirxml(document.body);
In this case, the console will display the XML for the body element.
Table format: console.table()
The console.table() method prints the properties and values of the object passed as an argument to the console in tabular format. For example, you can use:
console.table({name: 'John', age: 25, gender: 'Male'});
How to measure code execution time
JavaScript allows you to measure code execution time. Below, we will explain how to measure the execution time of your code.
console.time() / console.timeEnd()
You can measure the execution time of your code by using the console.time() and console.timeEnd() methods . You can use it like this:
console.time('label');
// Code to be measured
console.timeEnd('label');
In the above example, measurement is started with the label name ‘label’, and measurement is ended with the same label name specified. Measurement starts when you call the console.time() method, and ends when you call the console.timeEnd() method. The measurement results are displayed on the console with a label name.
performance.now()
The performance.now() method returns a DOMHighResTimeStamp object, representing the current time. You can use this to measure code execution time as shown below.
const t0 = performance.now();
// Code to be measured
const t1 = performance.now();
console.log(' Processing time:', (t1 - t0).toFixed(2), 'ms');
In the above example, the performance.now() method is used to obtain the start time and end time, and the difference is calculated to find the processing time. The number of digits after the decimal point is specified using the toFixed() method.
How to output logs according to conditions
JavaScript allows you to output logs only when certain conditions are met. The following explains how to output logs according to conditions.
Output log when condition is false: console.assert()
By using the console.assert() method , you can output logs only when the conditional expression is false. You can use it like this:
console.assert(condition, message);
In the above example, the message will be output to the log if the condition is false. Does nothing if condition is true. This method allows you to easily check whether a certain condition is met.
Conditional branching using if statements
By using an if statement to perform conditional branching, you can output logs according to conditions. You can write code like below.
if (condition) {
console.log(message);
}
In the above example, the message will be output to the log only if the condition is true. If condition is false, logs will not be output.
How to output execution stack trace
JavaScript allows you to print a stack trace of running code. A stack trace shows the order in which code is executed, allowing you to see which functions were called from which functions. The following explains how to output an execution stack trace.
Output stack trace: console.trace()
You can output a stack trace using the console.trace() method. You can use it like this:
console.trace();
The above example prints the stack trace starting from the caller of the current function. By using this method, you can check which function called it.
Printing stack trace using Error object
You can also print a stack trace using the Error object . You can use it like this:
try {
// some code here
} catch(error) {
console.error(error.stack);
}
In the above example, if an error occurs, it will print the stack property of the error object to the log. This allows you to print a stack trace.
Count the number of times for a specific label
The console.count() method is a method that allows you to count the number of times a specific label is associated. This method allows you to count how many times a particular code is called. The following explains how to use the console.count() method.
Count the number of times for a specific label: console.count()
You can count the number of times a specific label is used by using the console.count() method . You can use it like this:
console.count('label');
In the above example, we count the number of times for the label label. You can count the number of times by calling this method multiple times.
If no label is specified
If you call the console.count() method without specifying a label, “default” will be used as the label by default. You can use it like this:
console.count();
console.count();
console.count();
In the above example, we will count the number of times the label “default” is associated.
Reset count
The results counted using the console.count() method can be reset by reloading the browser or by calling the console.countReset() method. You can use it like this:
console.countReset('label');
The above example resets the count for the label label. If no label is specified, the default label “default” will be reset.
Using these methods, you can count the number of times a particular piece of code is called. You can use the console.count() method to find out which parts are called frequently.
Output grouped logs
The console.group() method is a method that can output grouped logs. By using this method, you can group and display logs hierarchically. The following explains how to use the console.group() method.
Create a group: console.group()
Groups can be created using the console.group() method. You can use it like this:
console.group('label');
The above example creates a group with the label label. After calling this method, if you output a log using the console.log() method, it will be displayed as a log included in the group.
nesting groups
Groups can be created hierarchically by nesting the console.group() method . You can use it like this:
console.group('parent');
console.log('parent log');
console.group('child');
console.log('child log');
console.groupEnd();
console.groupEnd();
In the above example, a group is created with the label parent, and a log called parent log is output to it. Next, create a group with the label child and output a log called child log in it. Finally, close the group by calling the console.groupEnd() method twice.
Collapse groups
You can collapse a group using the console.groupCollapsed() method . You can use it like this:
console.groupCollapsed('label');
The above example creates a group with the label label and displays it collapsed. You can expand a group by clicking on it.
These methods allow you to group and display logs hierarchically. You can use the console.group() method to clearly display the flow of code execution.
summary
I explained debugging using the console, which is one of the debugging methods in JavaScript.
- Logs can be output to the console using the console.log() method.
- Objects can be displayed on the console using the console.dir() method.
- You can measure the execution time of your code using the console.time() and console.timeEnd() methods.
- You can use the console.assert() method to output logs only when the condition is false.
- You can output the execution stack trace using the console.trace() method.
- You can use the console.count() method to count the number of times a particular block of code is executed.
- Logs can be grouped using the console.group() and console.groupEnd() methods.
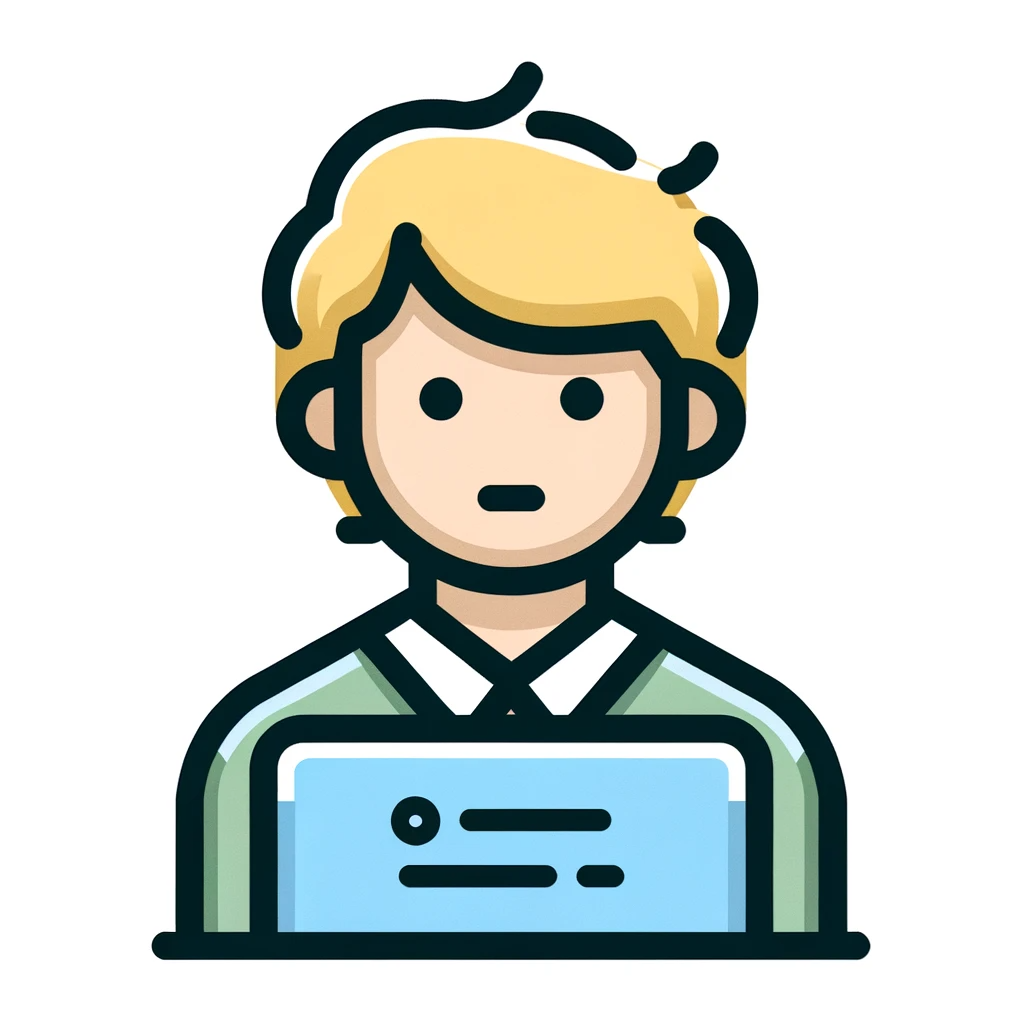
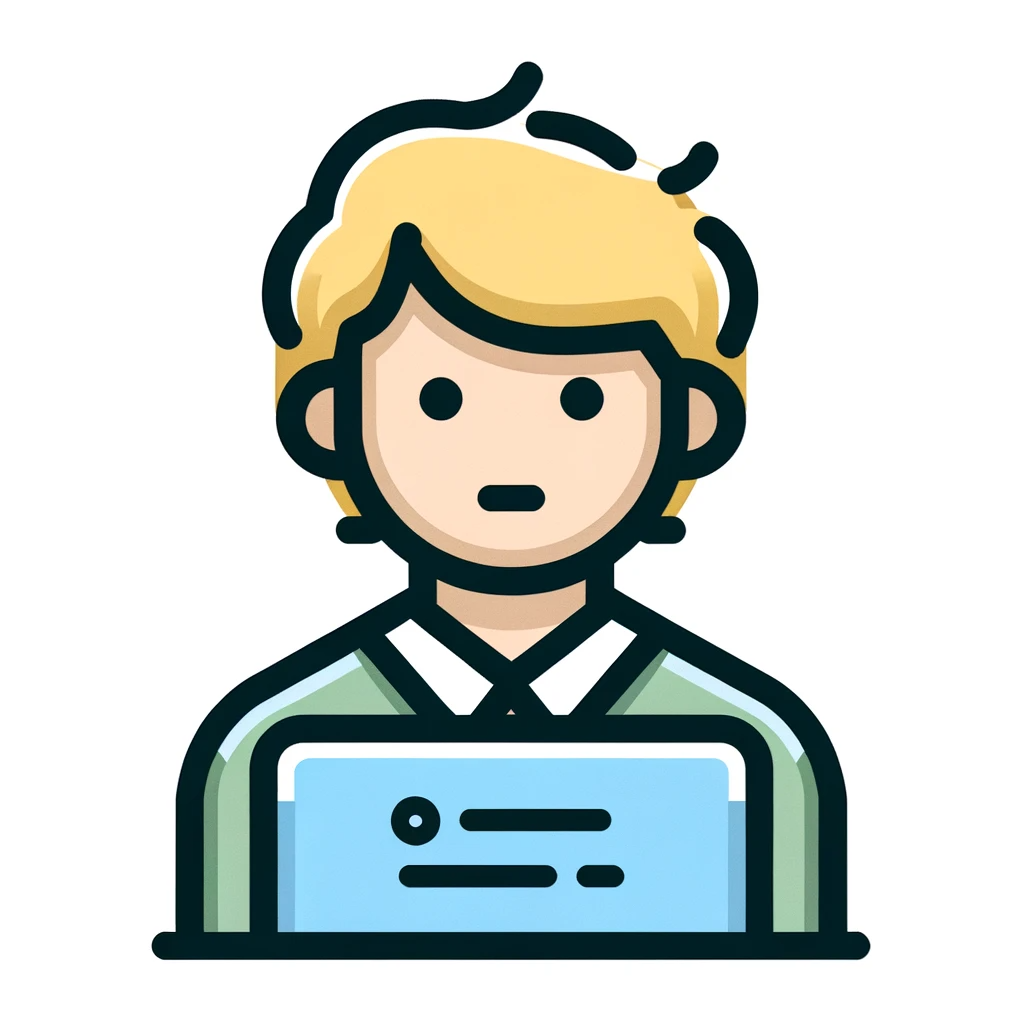
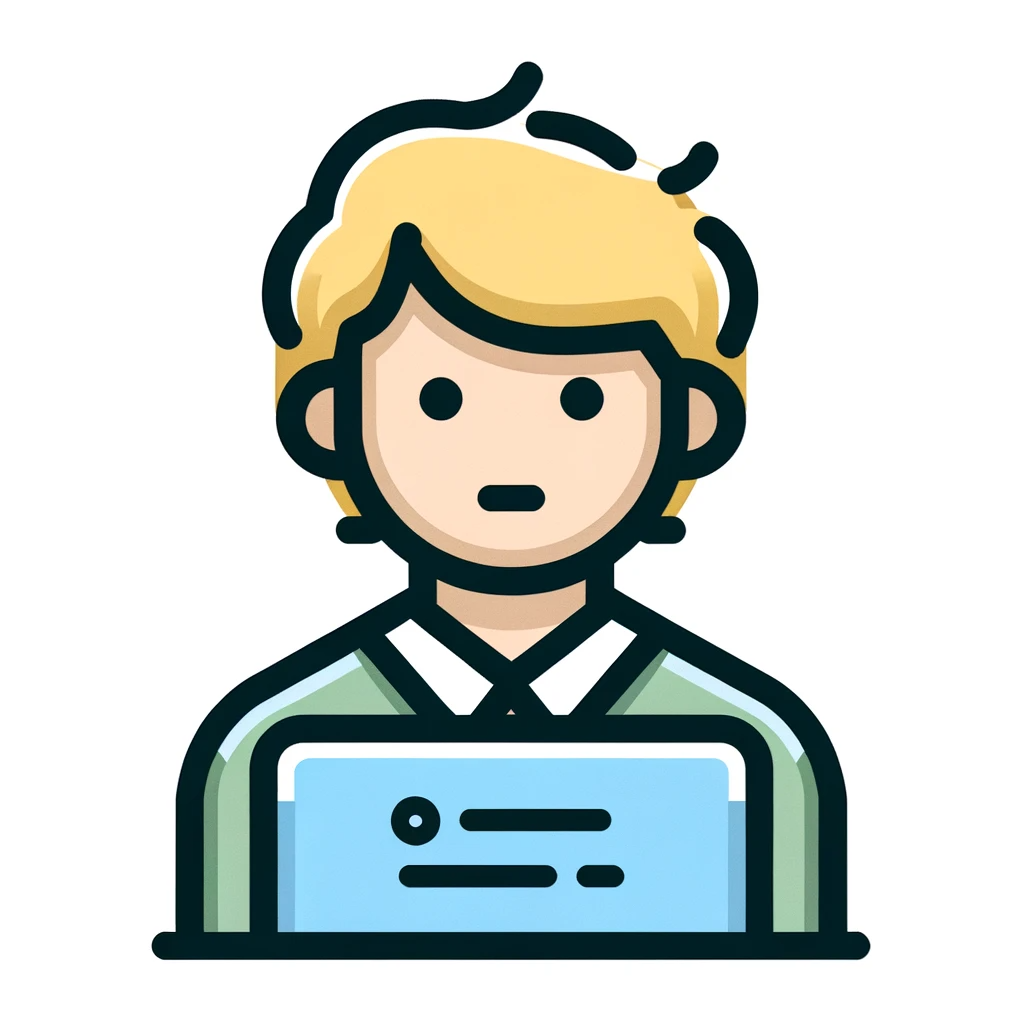
In addition to outputting logs, it is convenient to be able to obtain various information from the console, such as object and code execution time, conditional logs, and execution stack traces!
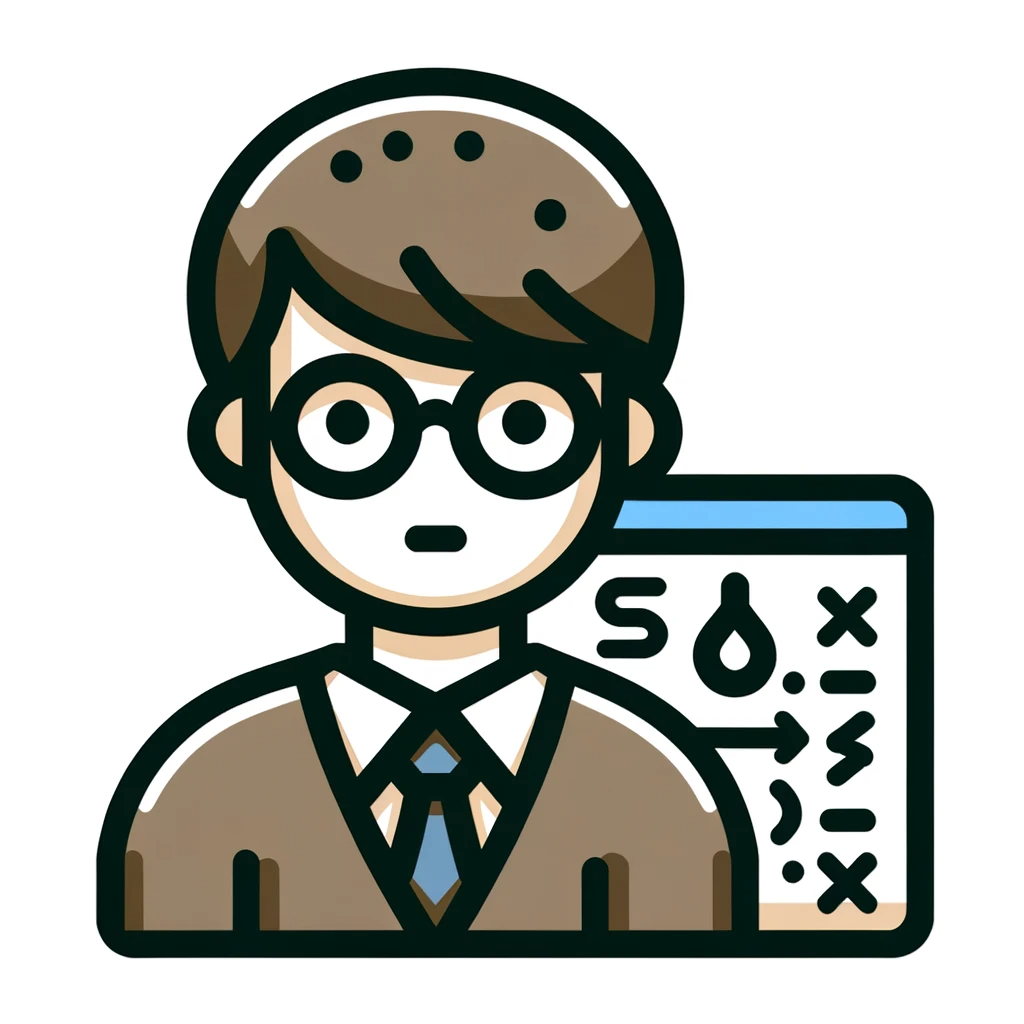
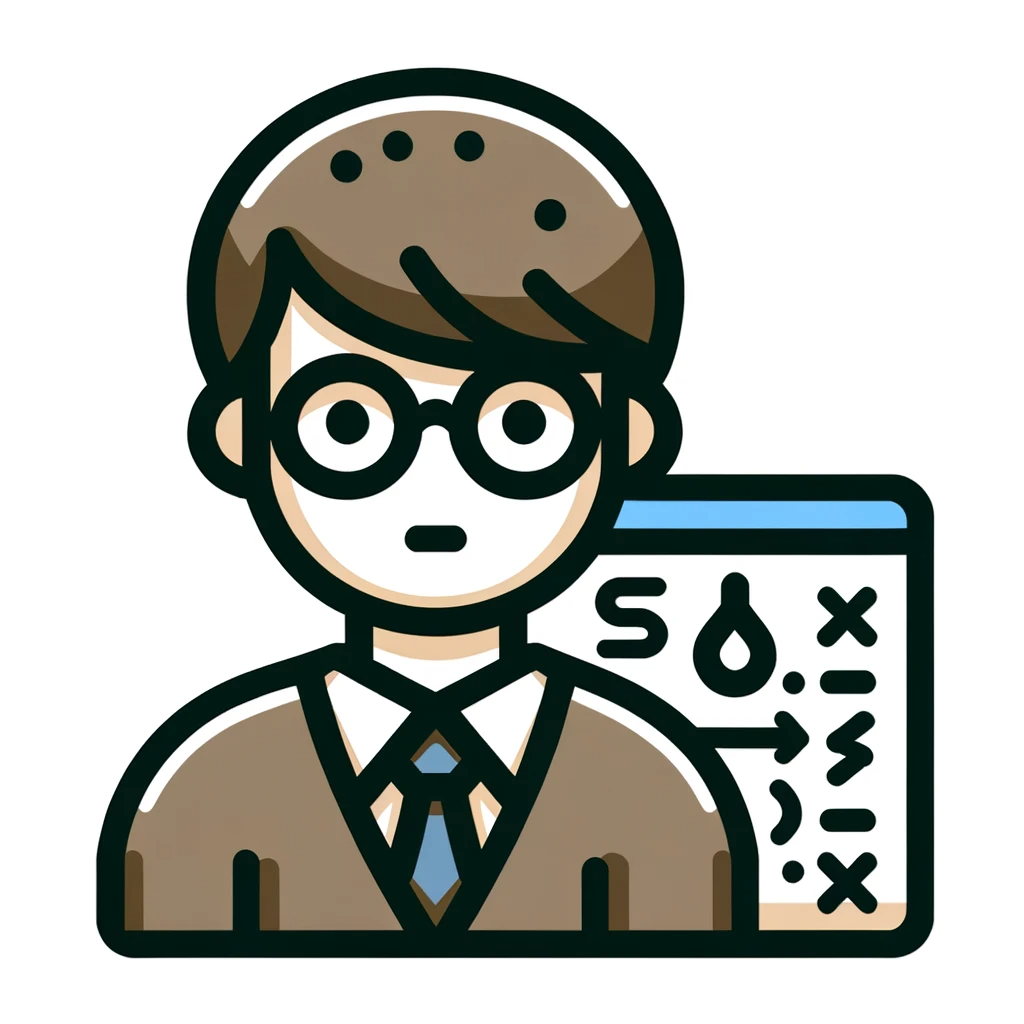
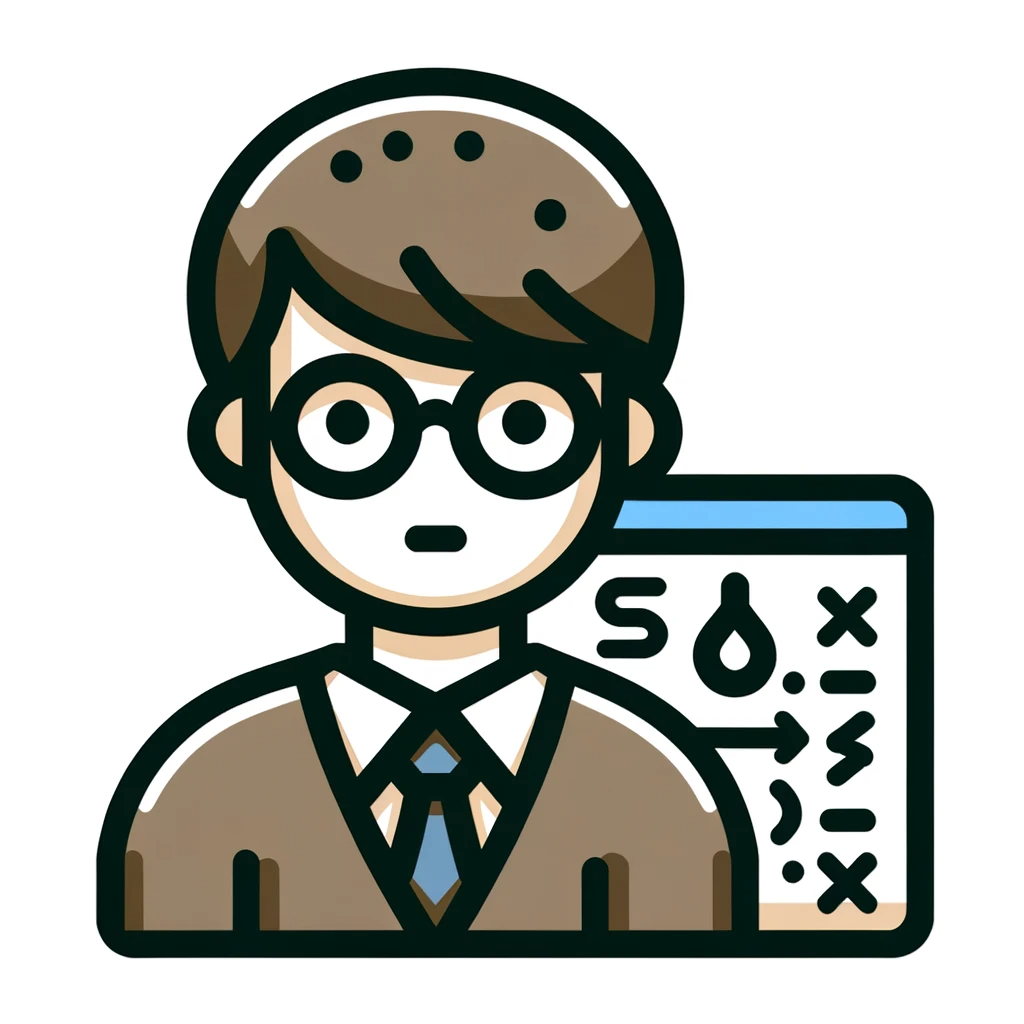
Debugging JavaScript becomes easier by leveraging the console.
Output logs on the console, display objects, measure code execution time, output logs according to conditions, output execution stack traces, count, and group logs. can do.
Make good use of these features for efficient debugging.
Comments