This article explains “methods for concatenating multiple arrays” in JavaScript.
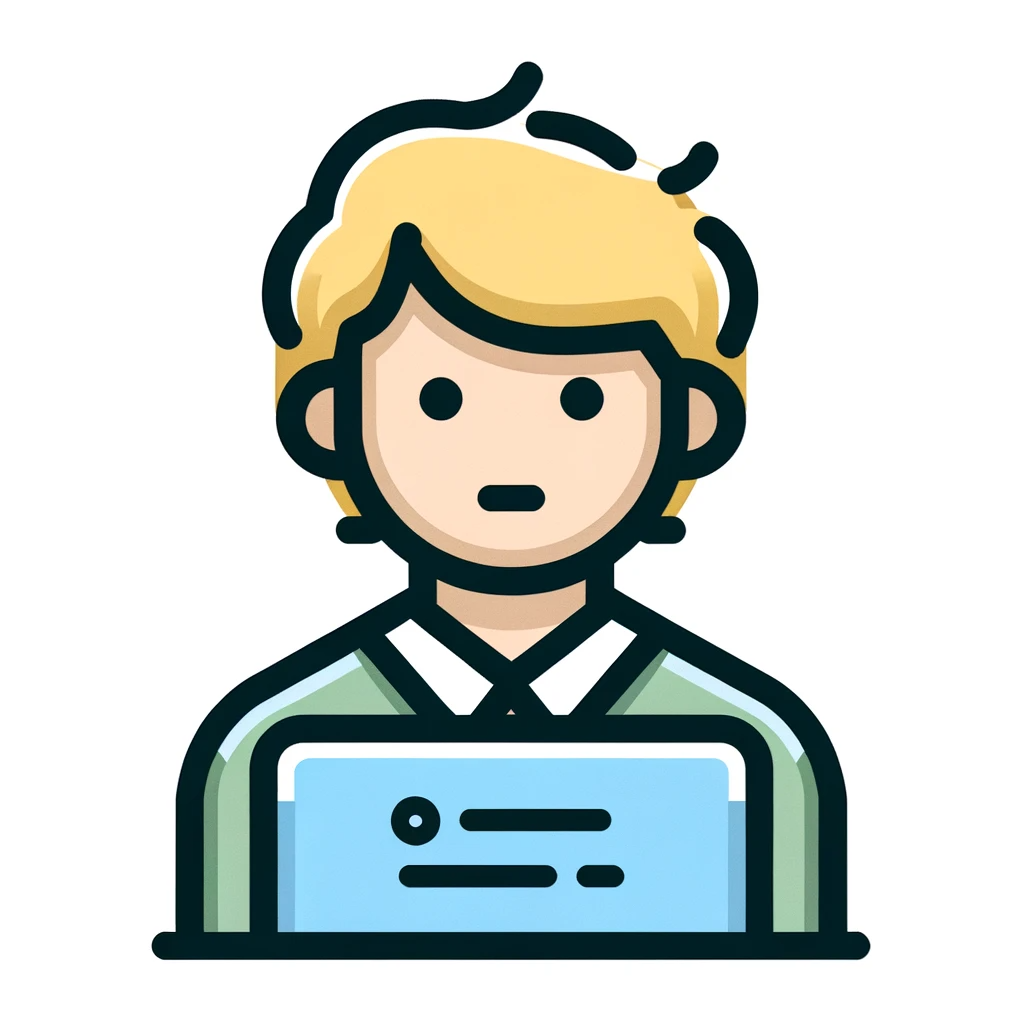
Is there a way to combine multiple arrays in JavaScript?
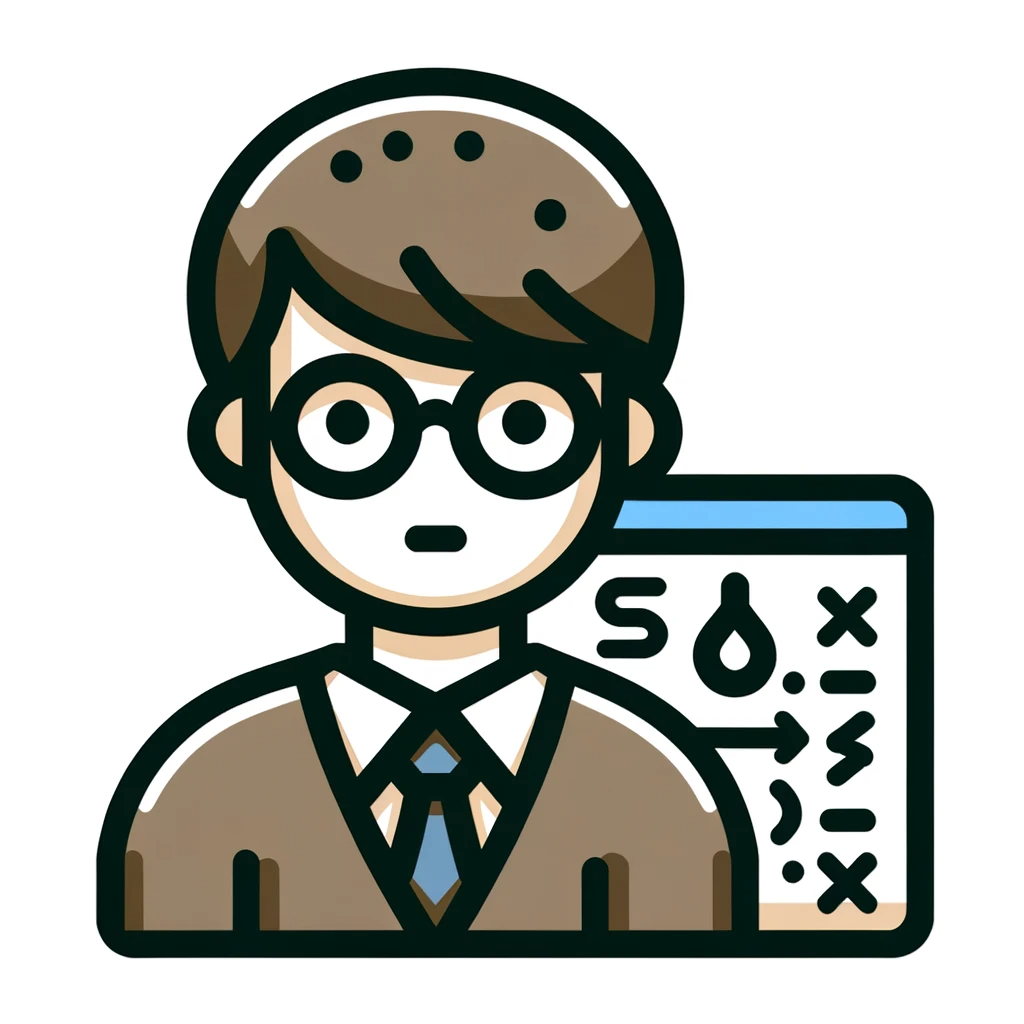
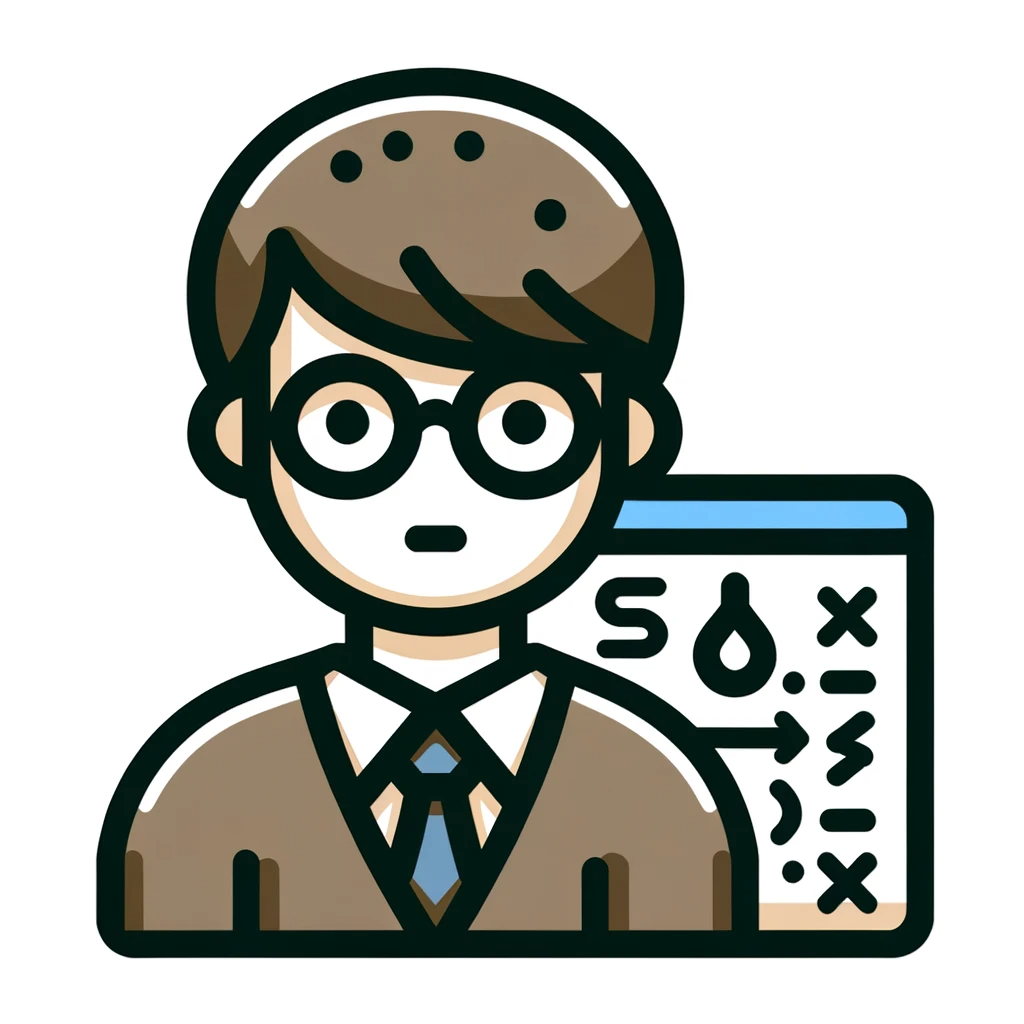
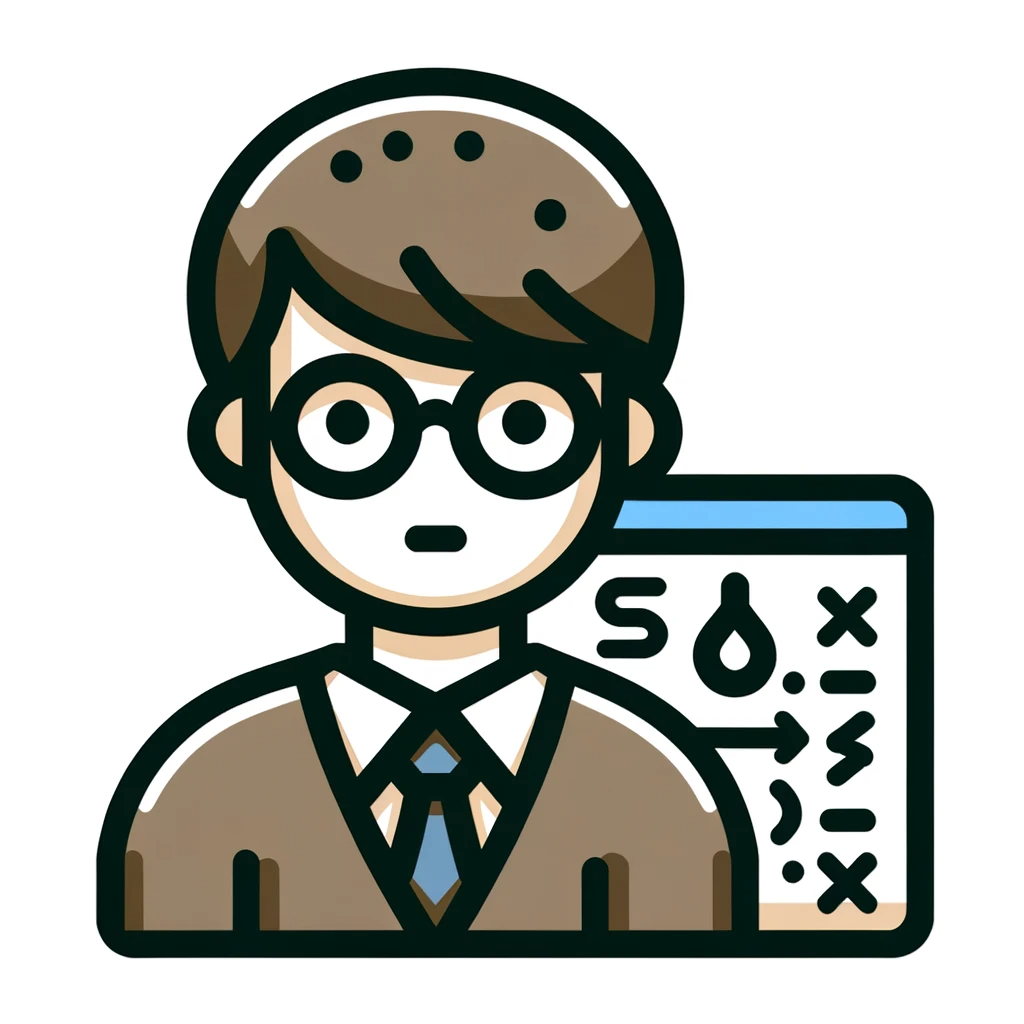
There are ways to combine arrays using the concat method or the spread operator.
“concat method” and “spread operator” to combine multiple arrays
To concatenate multiple arrays, you can use the concat method or the spread operator .
Combine multiple arrays using concat method
The concat method combines a new array or elements with an existing array and returns a new array . To use it, call the concat method on the array as shown below, and pass the array or elements you want to combine as arguments.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let array3 = [7, 8, 9];
let newArray = array1.concat(array2, array3);
console.log(newArray); // [1, 2, 3, 4, 5, 6, 7, 8, 9]
Combine multiple arrays using the spread operator
On the other hand, you can use the spread operator to expand the elements of an array and create a new array . To use it, enclose the array in curly braces ([]) and expand it using the spread operator (…) as shown below.
let array4 = [10, 11, 12];
let array5 = [13, 14, 15];
let newArray2 = [...array4, ...array5];
console.log(newArray2); // [10, 11, 12, 13, 14, 15]
By using the spread operator, you can directly expand and combine the elements of an array, which improves readability.
The choice of concat method and spread operator depends on implementation conditions and requirements. For example, if you need to convert the concatenated array back to the original array, it is better to use the concat method.
On the other hand, if the original array is not modified or readability is important, it is better to use the spread operator.
How to combine array elements with commas and spaces
To join arrays separated by commas, etc., use the join method .
array.join([delimiter]);
summary
We explained “methods for concatenating multiple arrays”.
- You can use the concat method to combine multiple arrays to create a new array.
- The spread operator allows you to expand the elements of an array to create a new array.
To concatenate multiple arrays in JavaScript, you can use the concat method and spread operator.
These allow you to easily combine multiple arrays to generate new arrays.
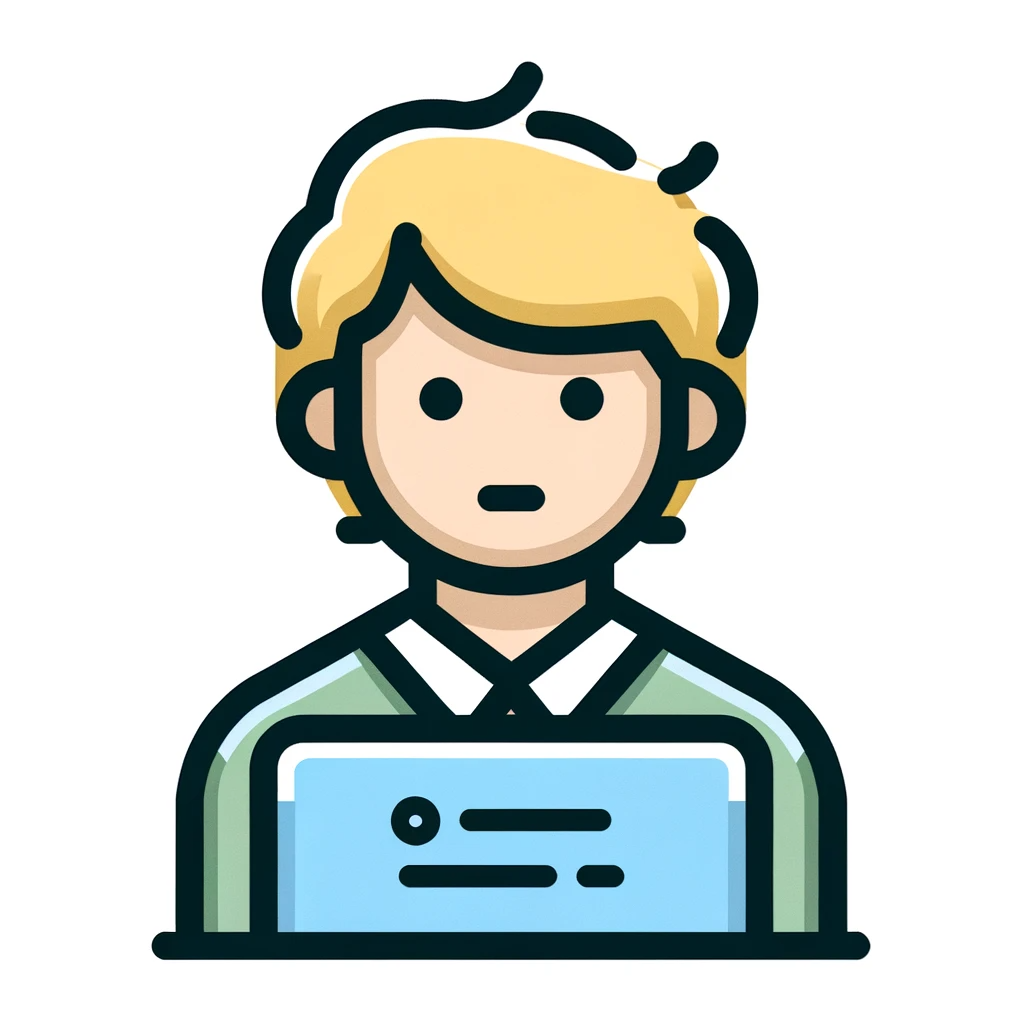
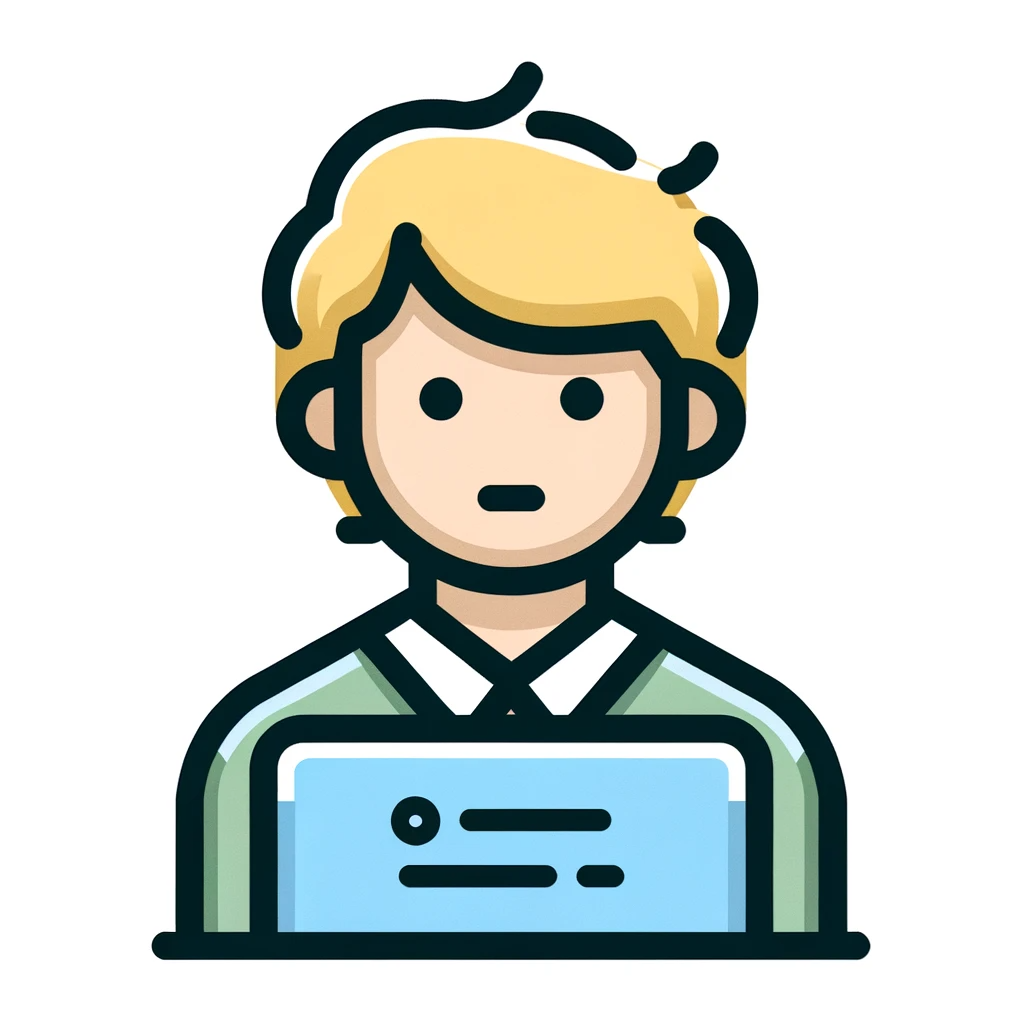
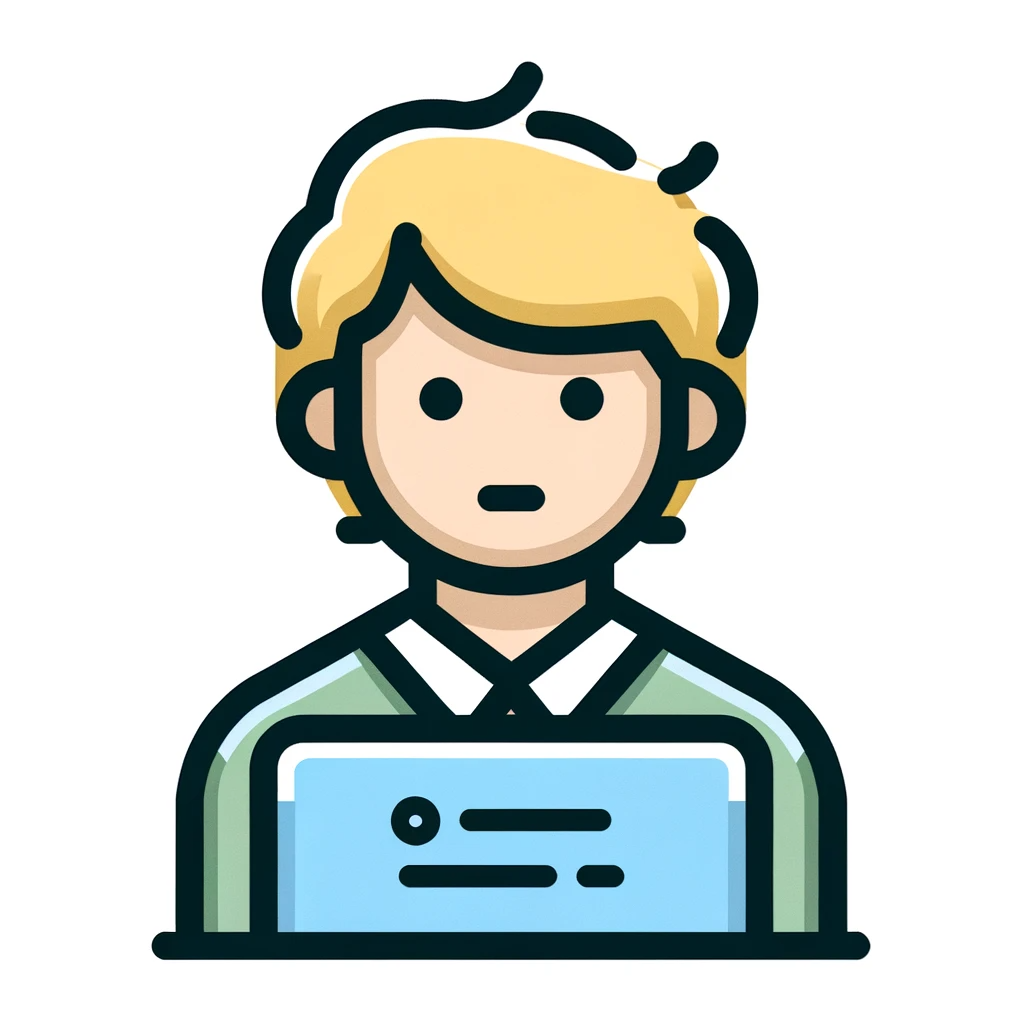
I was able to combine multiple arrays.
Comments