This article explains how to clone HTML elements using JavaScript’s cloneNode method.
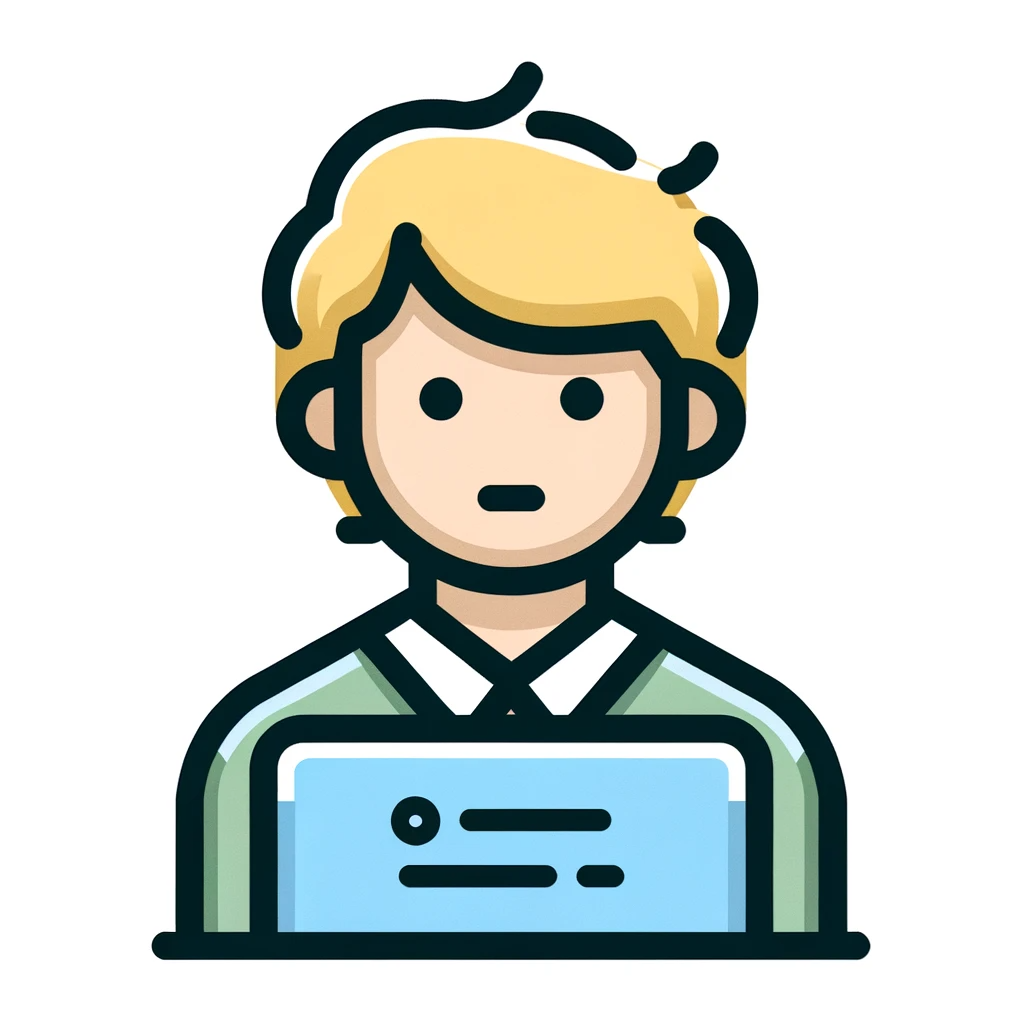
Is there a way to duplicate HTML elements?
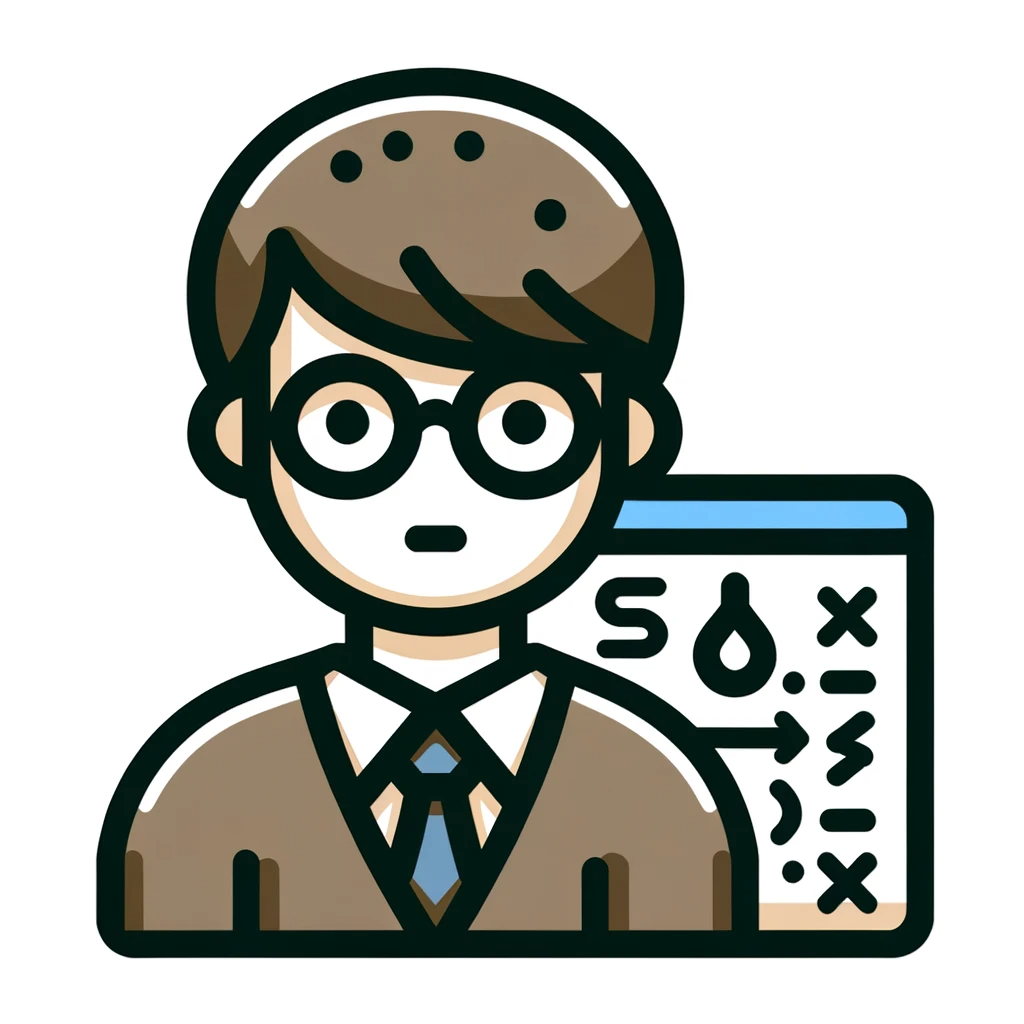
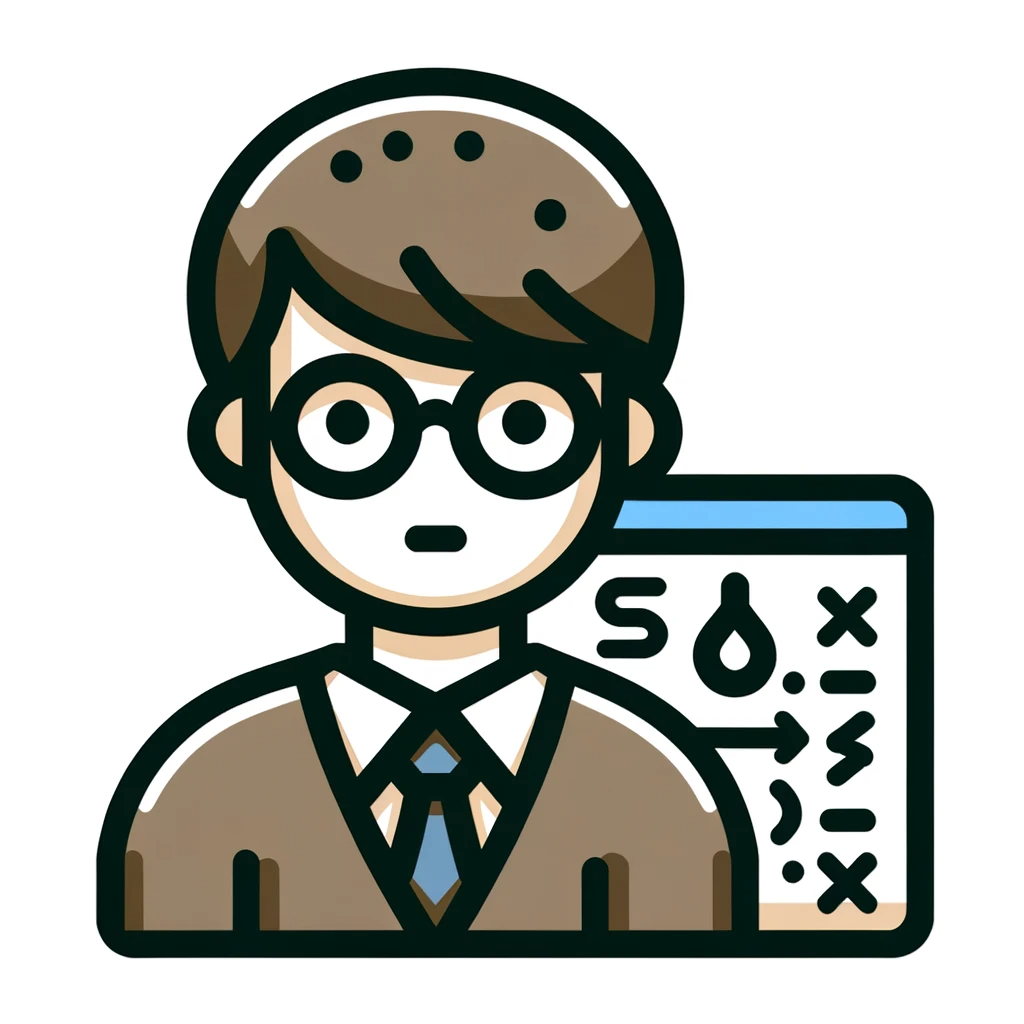
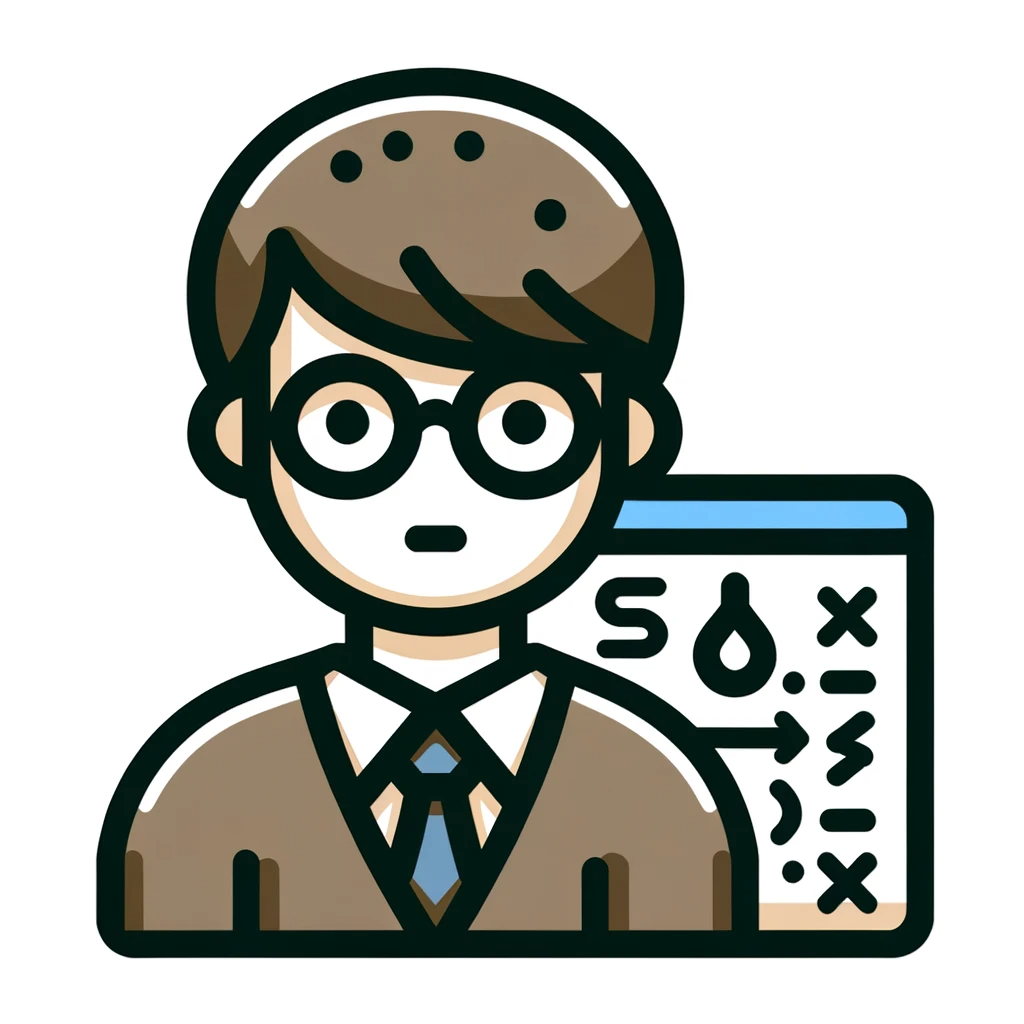
You can clone an element by using
the cloneNode method .
What is the cloneNode method?
The cloneNode method creates a clone of the specified element .
This method clones the entire tree structure of the specified element.
node.cloneNode(deep)
deep: whether to replicate up to descendant nodes
node is a node object that specifies the element to duplicate, and deep is a boolean value that specifies the hierarchy to duplicate.
If this boolean value is true, the source element and all its descendant elements will be duplicated. If false, only the original element will be duplicated. If deep is omitted, the default is false.
How to use the cloneNode method
Use the cloneNode method as follows.
Suppose you have an HTML element like the one below.
<div id="example">
<p>Hello, world!</p>
</div>
To duplicate this element:
const original = document.getElementById('example');
const cloned = original.cloneNode(true);
In this case, the div element with id “example” will be cloned, and all elements contained below it will also be cloned. By specifying the true parameter, the entire element will be duplicated, including child elements.
On the other hand, if you specify false as shown below, only the original element will be duplicated.
const original = document.getElementById('example');
const cloned = original.cloneNode(false);
In this case, only the div element with id “example” will be cloned, and the p elements contained below it will not be cloned.
Elements cloned using the cloneNode method have the same attributes as the original element, but state such as event listeners and data are not cloned. If you want to append the duplicated element to another element, you should use a method such as appendChild to append it to another element in the document.
Explanation using cloneNode sample program
The sample program below clones the div element with id “example” and adds a new element to the body element.
<div id="example">
<p>Hello, world!</p>
</div>
const original = document.getElementById('example');
const cloned = original.cloneNode(true);
document.body.appendChild(cloned);
Running this code will generate HTML code like the one below.
<body>
<div id="example">
<p>Hello, world!</p>
</div>
</body>
In this example, the original element had an id attribute, so the cloned element also has the same id attribute. Therefore, if the duplicated element is added elsewhere in the document, it will not behave differently from the original element.
summary
We explained how to clone HTML elements using the cloneNode method.
- The cloneNode method is used to clone an element.
- The cloneNode method has a boolean value that specifies whether to clone the source element and all its descendant elements, and if omitted, it defaults to false.
- The cloned element has the same attributes as the original, but state such as event listeners and data are not duplicated.
- If you want to append the duplicated element to another element, you should use a method such as appendChild to append it to another element in the document.
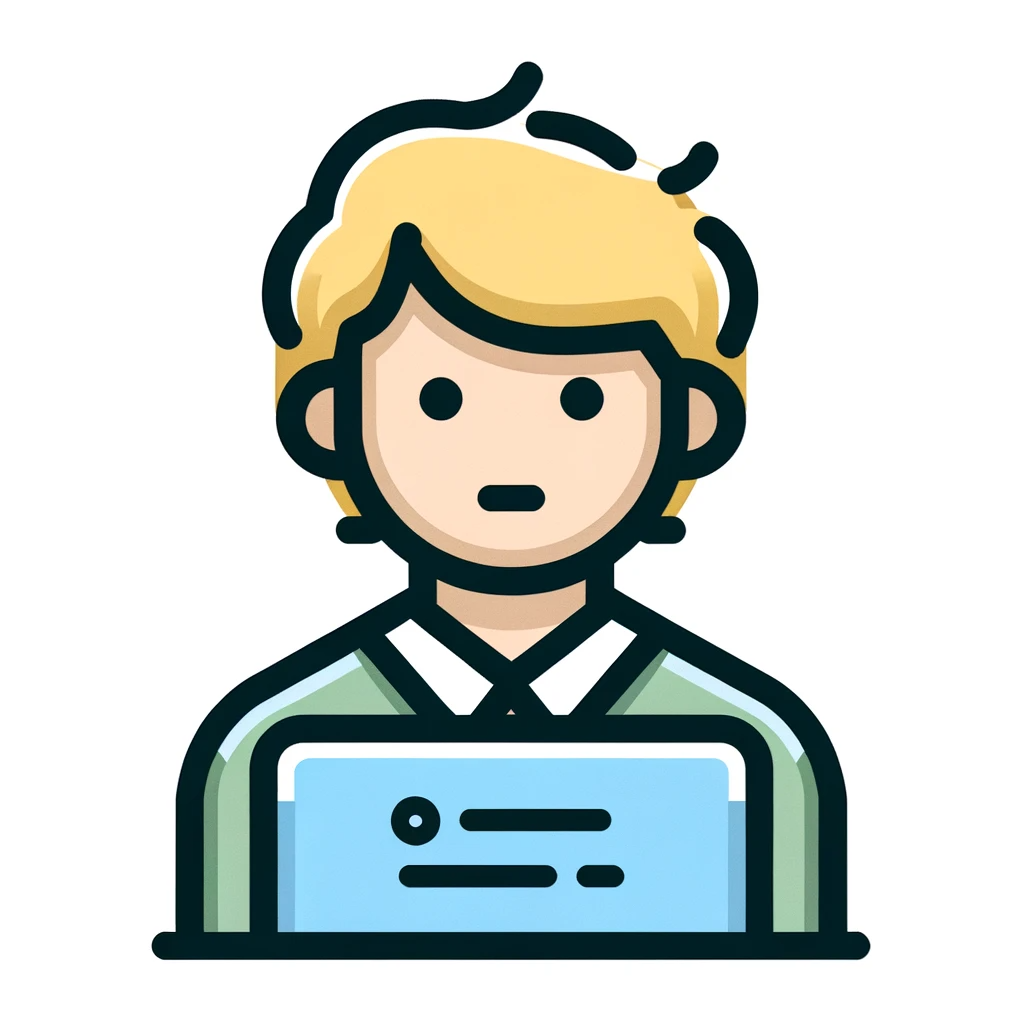
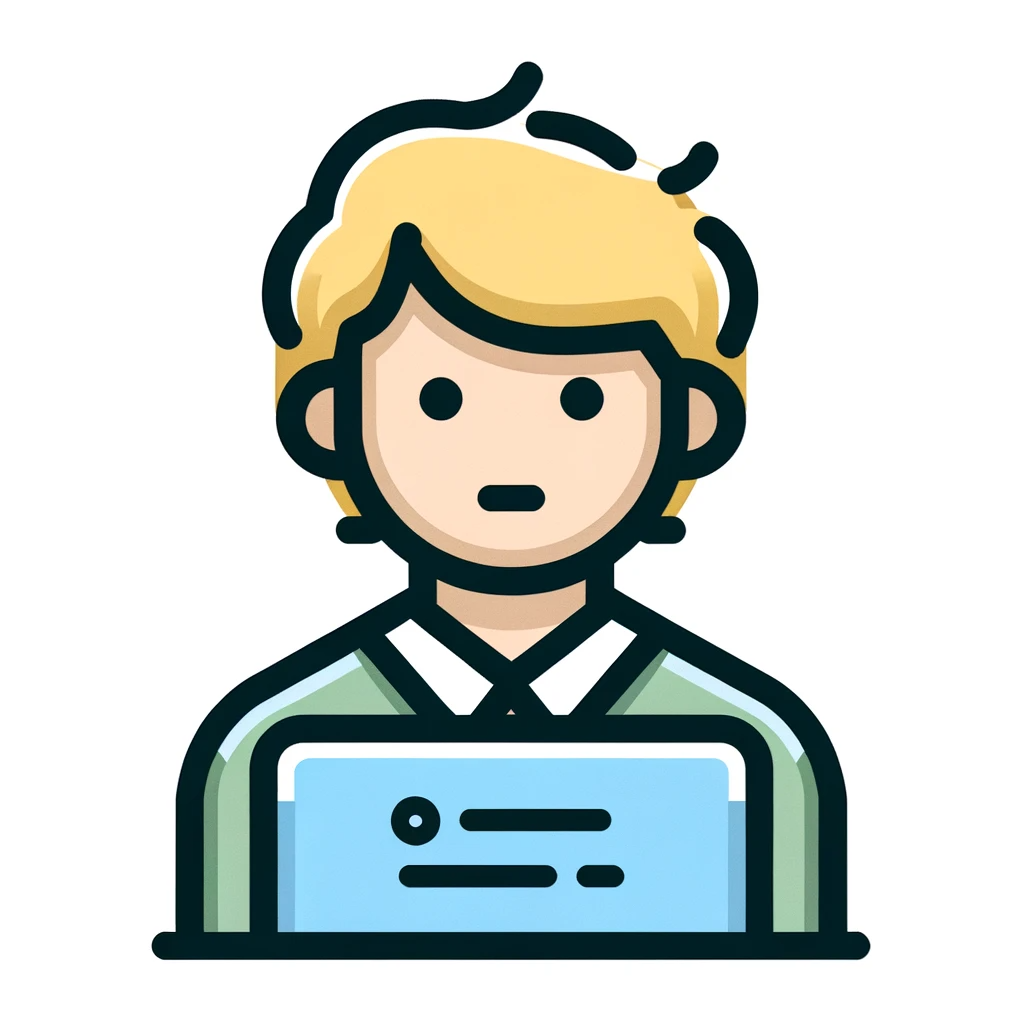
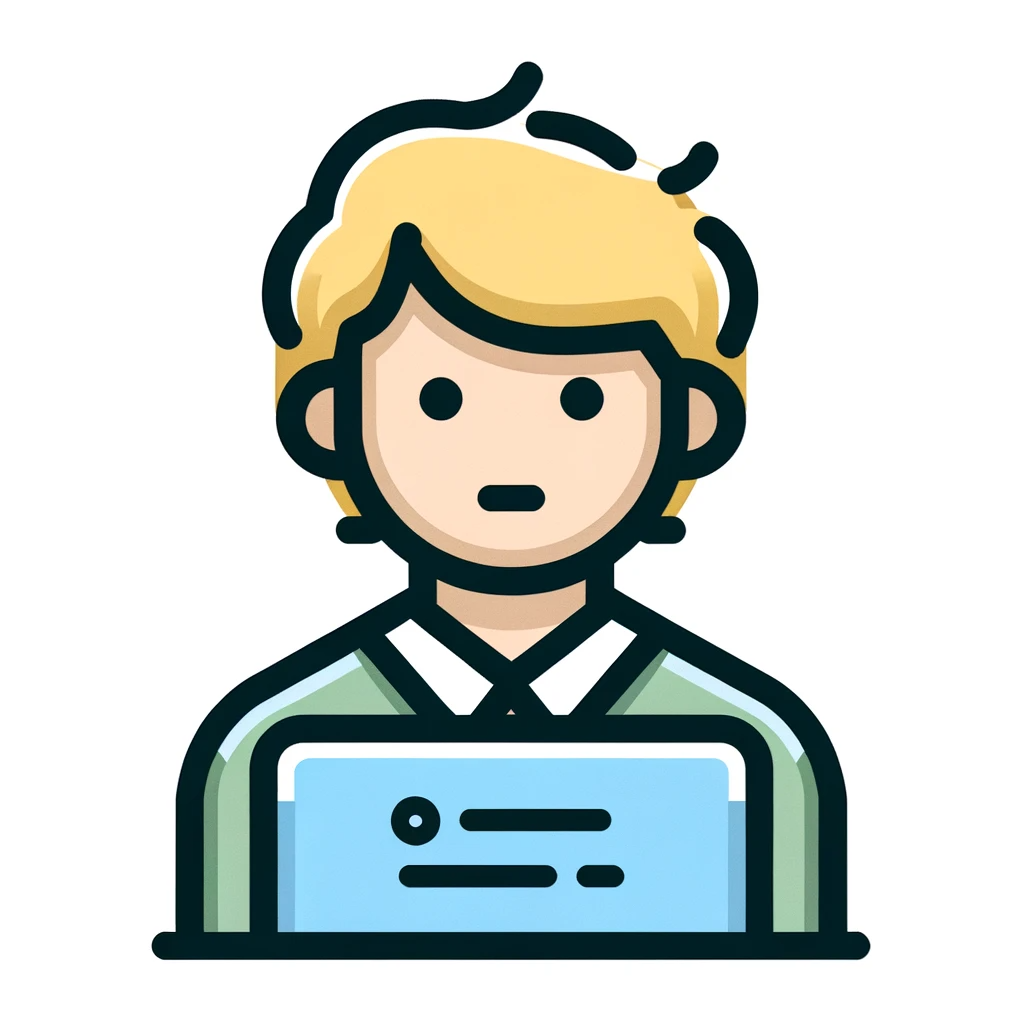
The cloneNode method seems to be useful when duplicating elements.
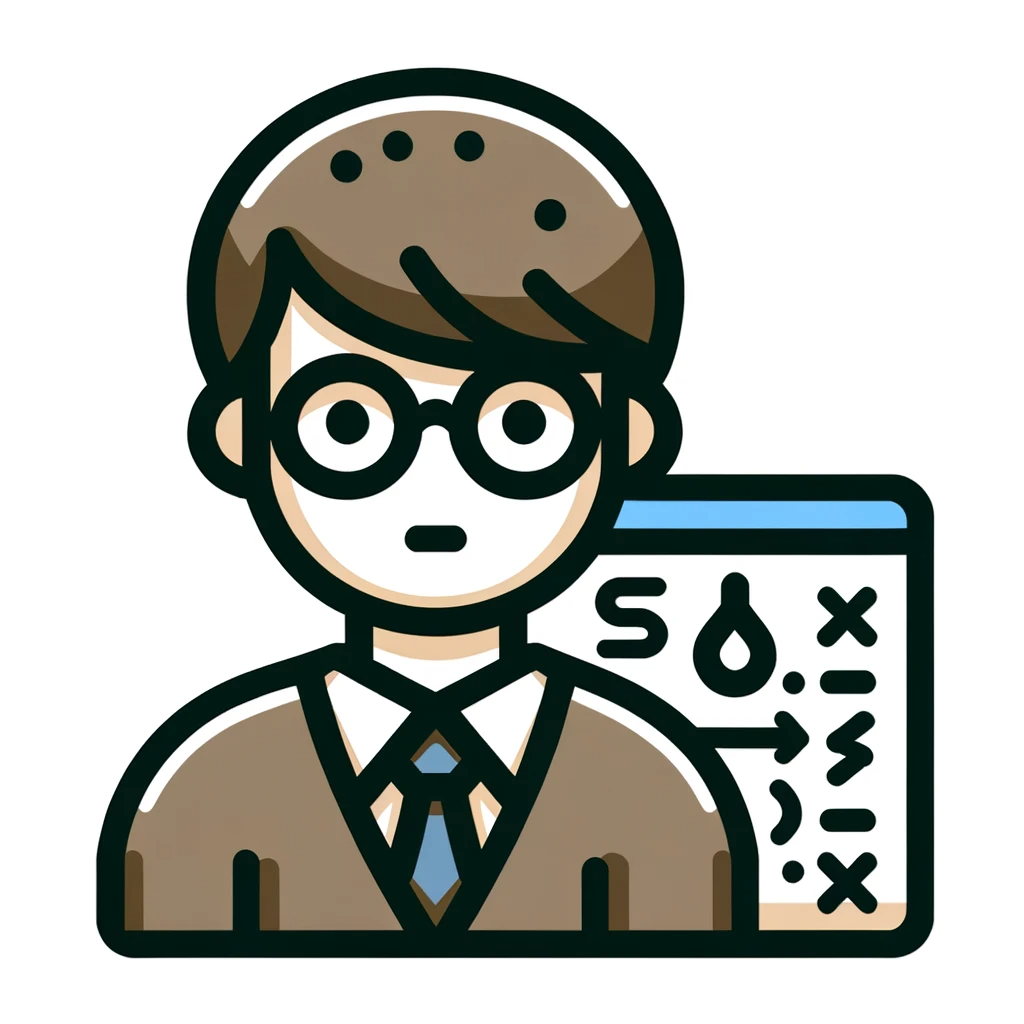
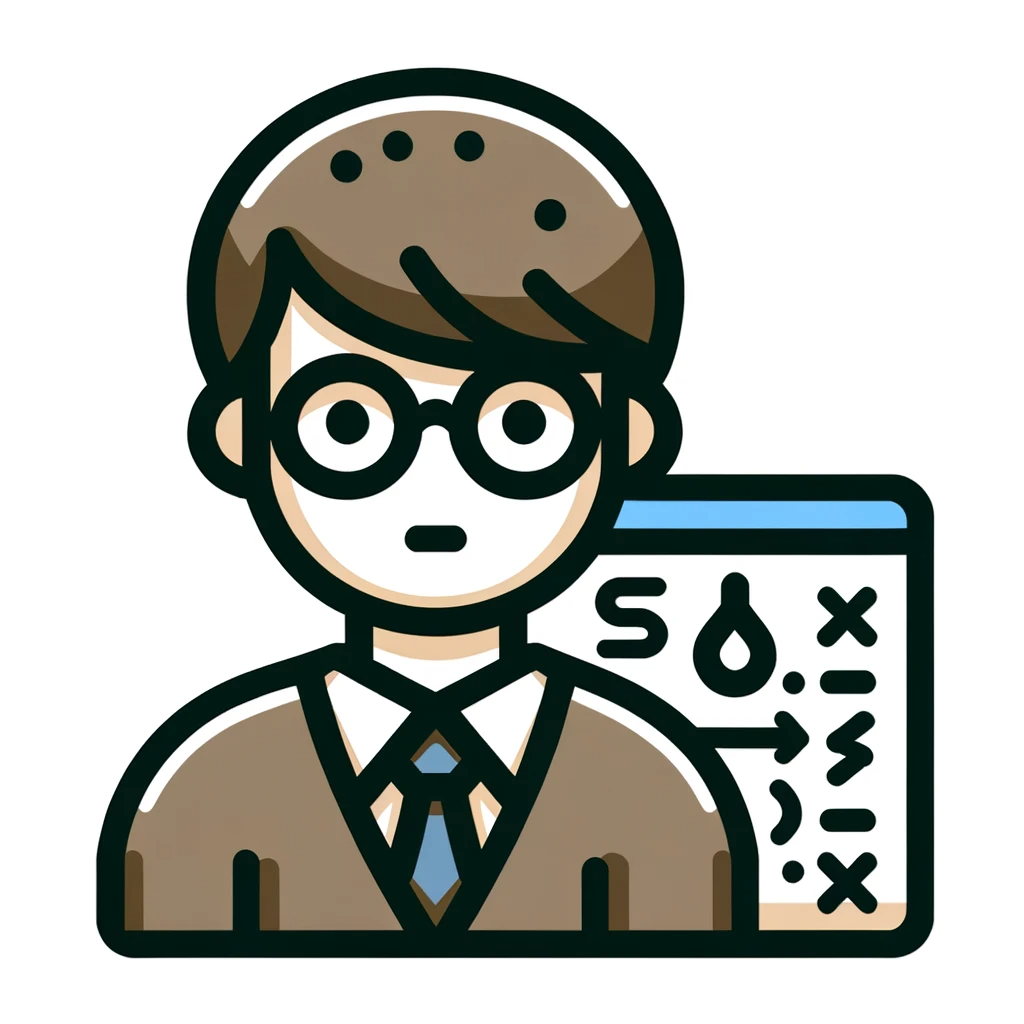
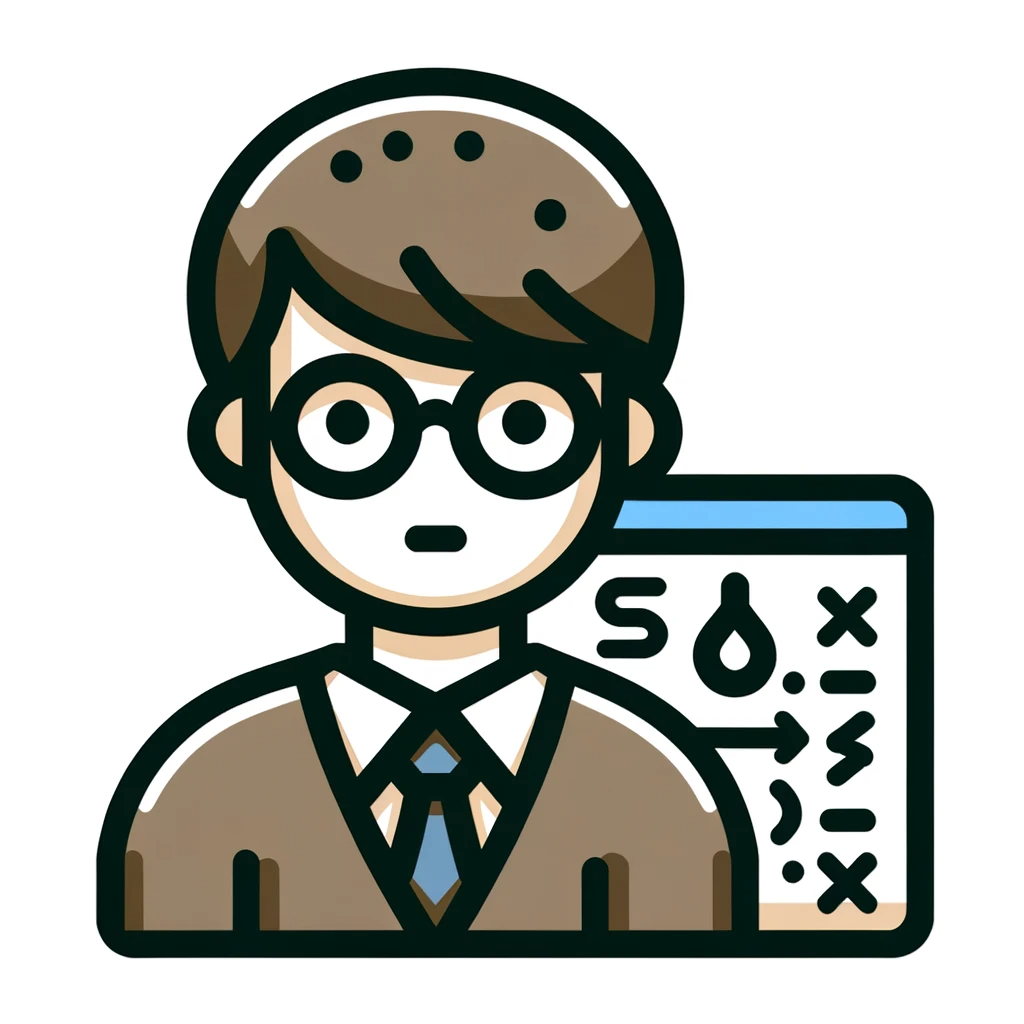
The cloneNode method is one of the basic methods for duplicating elements.
Duplicating an element prevents the original element from being changed by user interaction.
Additionally, by using the cloneNode method, you can reuse elements and improve the readability and maintainability of your code.
Comments