This article explains how to dynamically set and remove the style class of HTML elements using JavaScript .
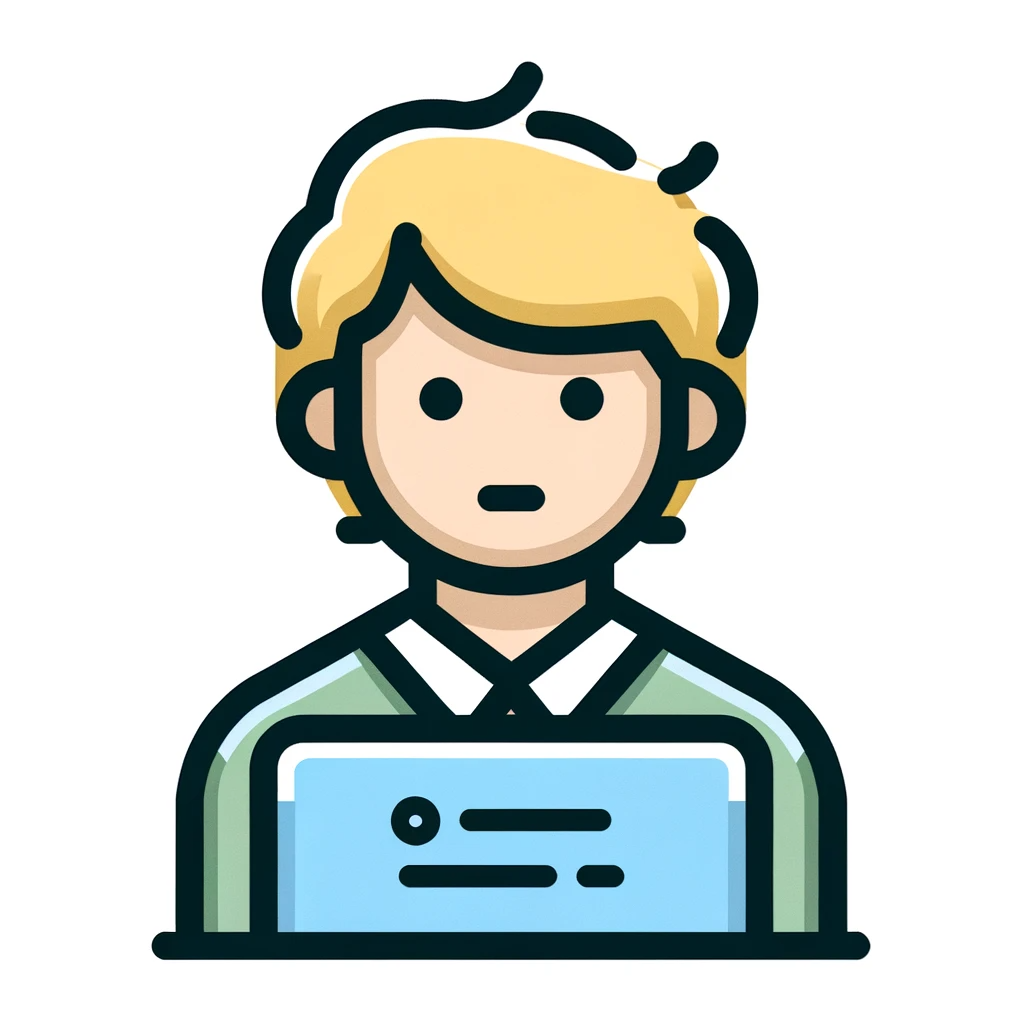
I want to dynamically set the style class of a web page, but how do I do it?
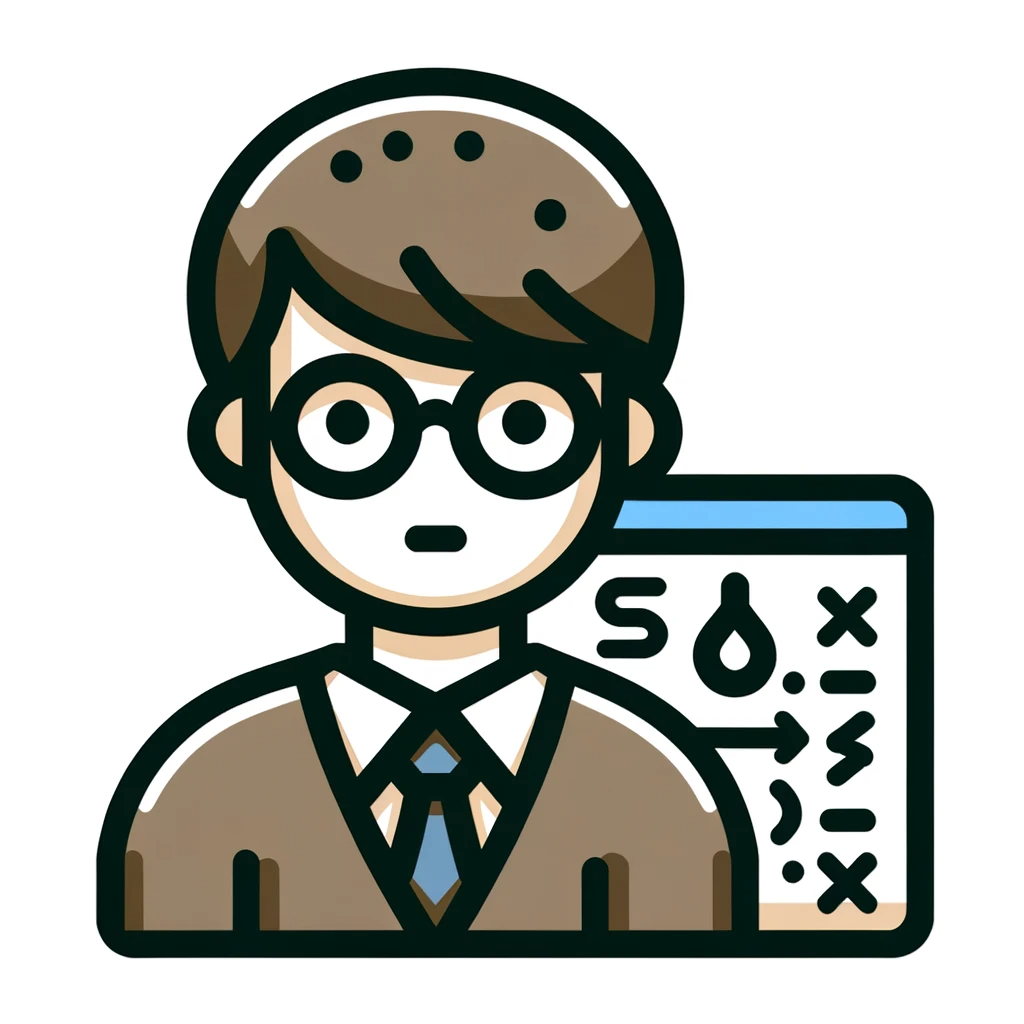
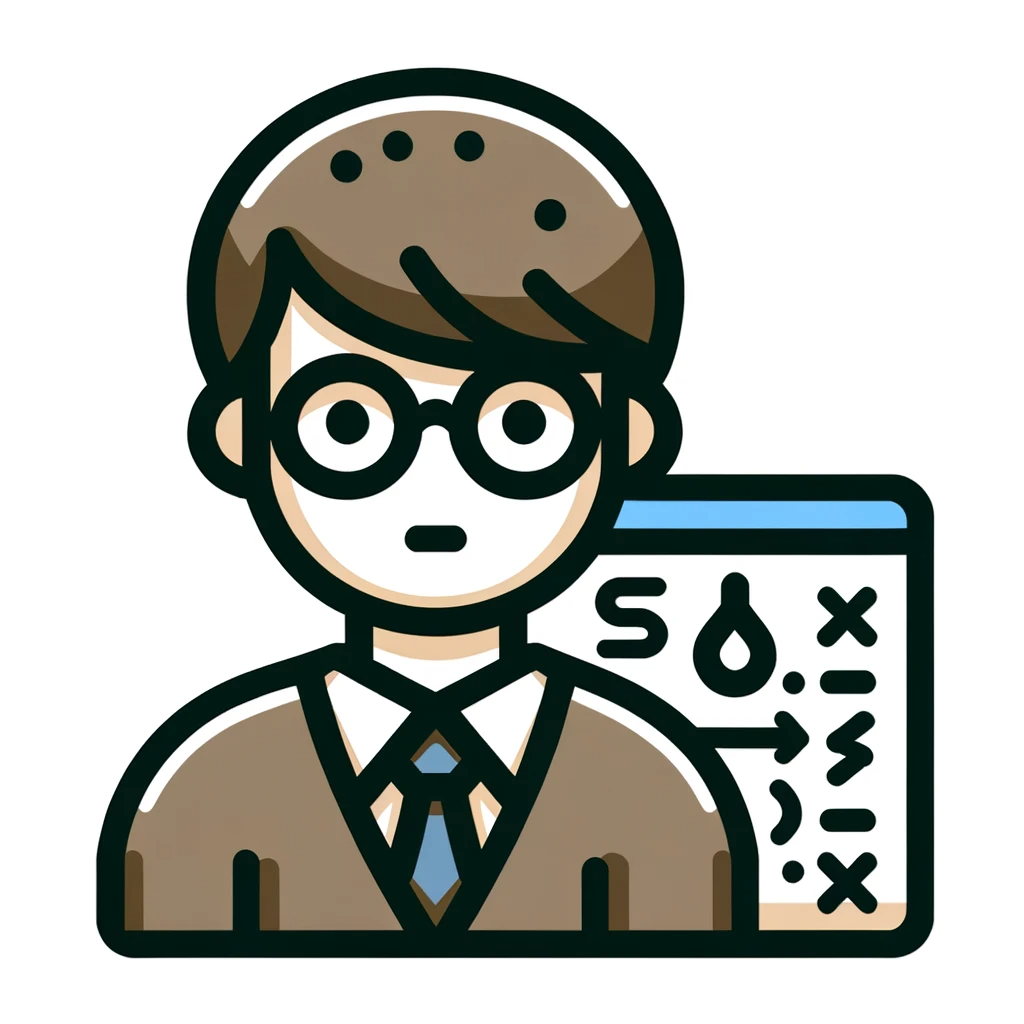
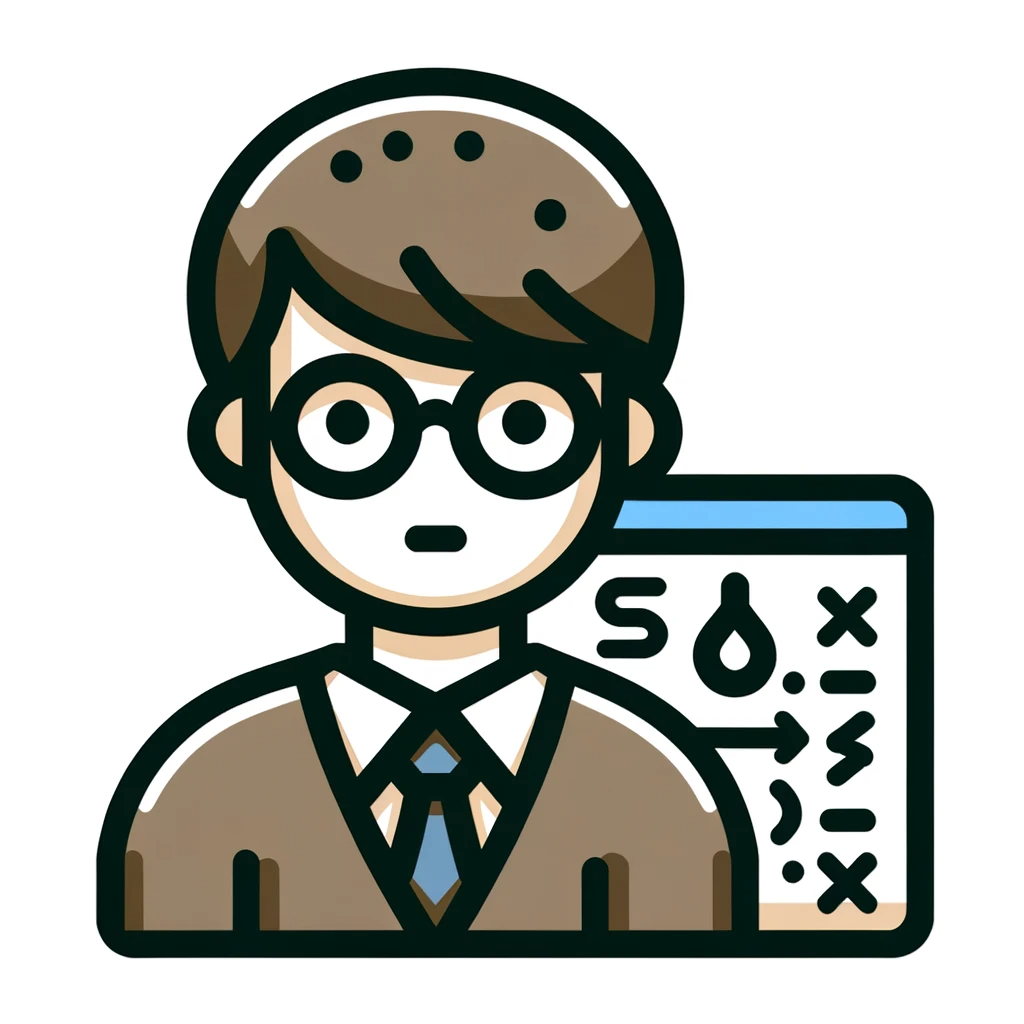
There are two ways to dynamically set style classes: the classList property and the className property.
How to use classList property
One way to dynamically set style classes is to use the classList property . The classList property allows you to add, remove, or toggle an element’s class list .
Below is an example of how to add a new class to an element using the classList property.
var element = document.getElementById("myDiv");
element.classList.add("myClass");
In this example, you can add a class called “myClass” to the element with id “myDiv”. In this way, you can easily apply styles to an element by adding a new class to the element using the classList property.
You can also delete classes using the classList property. Below is an example of how to remove it.
var element = document.getElementById("myDiv");
element.classList.remove("myClass");
In this example, you can remove the class “myClass” from the element with id “myDiv”.
You can also use the contains method of the classList property to check if a class exists . Below is an example of how to check if a class exists using the contains method.
var element = document.getElementById("myDiv");
if (element.classList.contains("myClass")) {
// Processing when "myClass" is included in the element's class list
} else {
// Processing when "myClass" is not included in the element's class list
}
In this example, you can check whether the element with id “myDiv” contains a class called “myClass” and perform processing according to the condition.
Sample program using classList property
Introducing a sample program that uses the classList property and its explanation.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>How to use the classList property</title>
<style>
.selected {
background-color: yellow;
}
</style>
</head>
<body>
<p id="sample">I am learning JavaScript.</p>
<button onclick="addStyle()">Add a style</button>
<button onclick="removeStyle()">Delete Style</button>
<script>
function addStyle() {
var element = document.getElementById("sample");
element.classList.add("selected");
}
function removeStyle() {
var element = document.getElementById("sample");
element.classList.remove("selected");
}
</script>
</body>
</html>
In the above sample, the addStyle() function first uses document.getElementById(“sample”) to obtain the element whose id attribute is sample. For the retrieved element, a selected class is added using classList.add(). This applies the .selected class defined for the element and changes the background color to yellow.
In the removeStyle() function, the element whose id attribute is sample is first retrieved using document.getElementById(“sample”). For the retrieved element, classList.remove() is used to remove the selected class. This removes the .selected class defined for the element and returns the background color to its original state.
How to use className property
Next, we will explain how to dynamically set and delete style classes using the className property .
To add a new class to an element, add the class name you want to add to the value of the className property and set it to the class attribute of that element.
In the example below, a class called “myClass” is added to the element with id “myDiv”.
<div id="myDiv">Hello World!</div>
<button onclick="addClass()">Add Class</button>
<script>
function addClass() {
var element = document.getElementById("myDiv");
element.className += " myClass";
}
</script>
This code first defines a div element that contains the text “Hello World!”. Below that there is a button called “Add Class”. When the button is clicked, the above JavaScript code is called and adds a class called “myClass” to the element with id “myDiv”.
Next, to remove a class from an element, remove the class name you want to remove from the value of the className property and set it in the class attribute of that element. In the example below, the class “myClass” is removed from the element with id “myDiv”.
<div id="myDiv" class="myClass">Hello World!</div>
<button onclick="removeClass()">Remove Class</button>
<script>
function removeClass() {
var element = document.getElementById("myDiv");
var classes = element.className.split(" ");
var index = classes.indexOf("myClass");
if (index !== -1) {
classes.splice(index, 1);
element.className = classes.join(" ");
}
}
</script>
This code first defines a div element that contains the text “Hello World!”. Below that there is a button called “Remove Class”. When the button is clicked, the above JavaScript code is called and the class called “myClass” is removed from the element with id “myDiv”.
Sample program using className property
I will introduce a sample program that uses the className property.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>How to use the className property</title>
<style>
.selected {
background-color: yellow;
}
</style>
</head>
<body>
<p id="sample">I am learning JavaScript.</p>
<button onclick="addStyle()">Add a style</button>
<button onclick="removeStyle()">Delete Style</button>
<script>
function addStyle() {
var element = document.getElementById("sample");
element.className = "selected";
}
function removeStyle() {
var element = document.getElementById("sample");
element.className = "";
}
</script>
</body>
</html>
In the above sample, the addStyle() function first uses document.getElementById(“sample”) to obtain a element whose id attribute is sample. For the retrieved element, the className property is assigned to “selected”. This applies the .selected class defined for the element and changes the background color to yellow.
In the removeStyle() function, the element whose id attribute is sample is first obtained using document.getElementById(“sample”). For the retrieved element, an empty string is assigned to the className property. This deletes the .selected class defined for the element and returns the background color to its original state.
summary
We explained how to dynamically set and delete the style class of HTML elements.
- There are two ways to dynamically set style classes: using the classList property and using the className property.
- You can add new classes to an element or remove existing classes by using the classList property.
- You can also add a new class to an element or remove an existing class by using the className property.
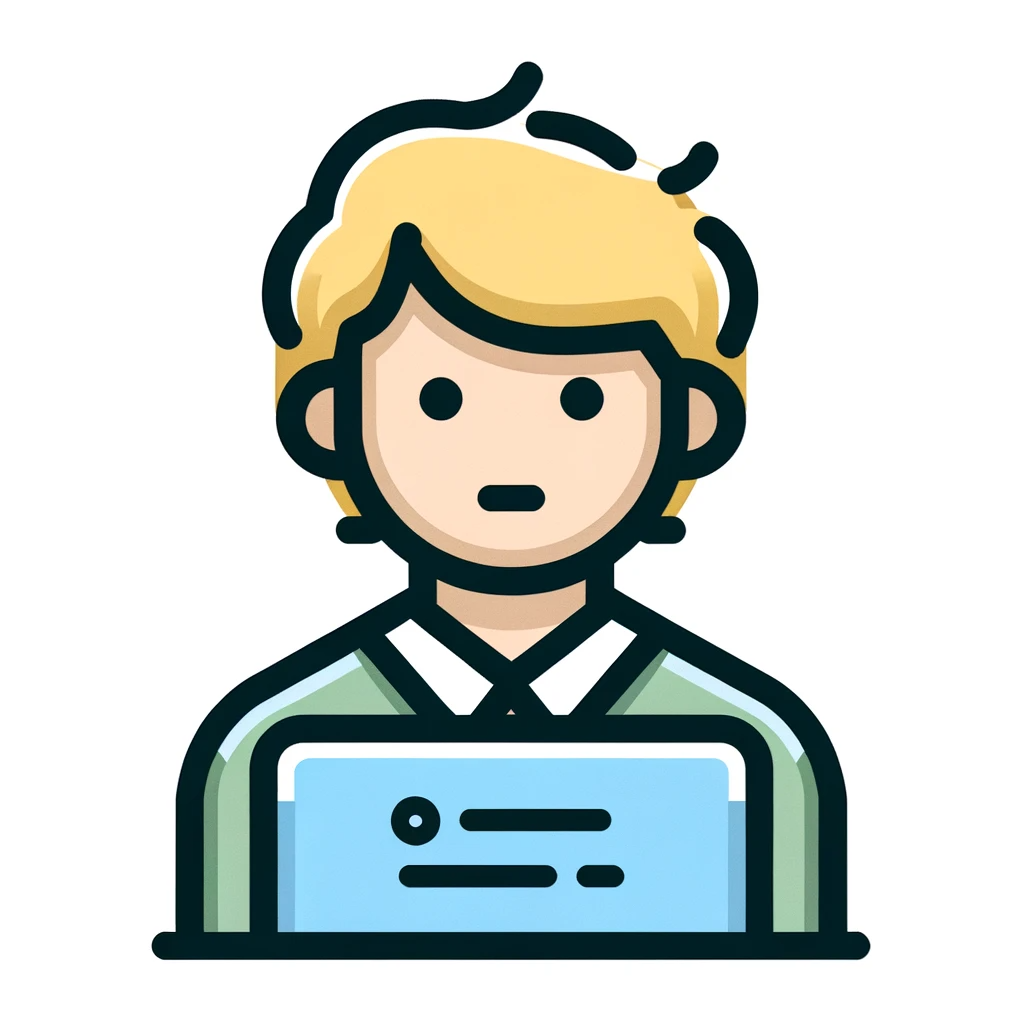
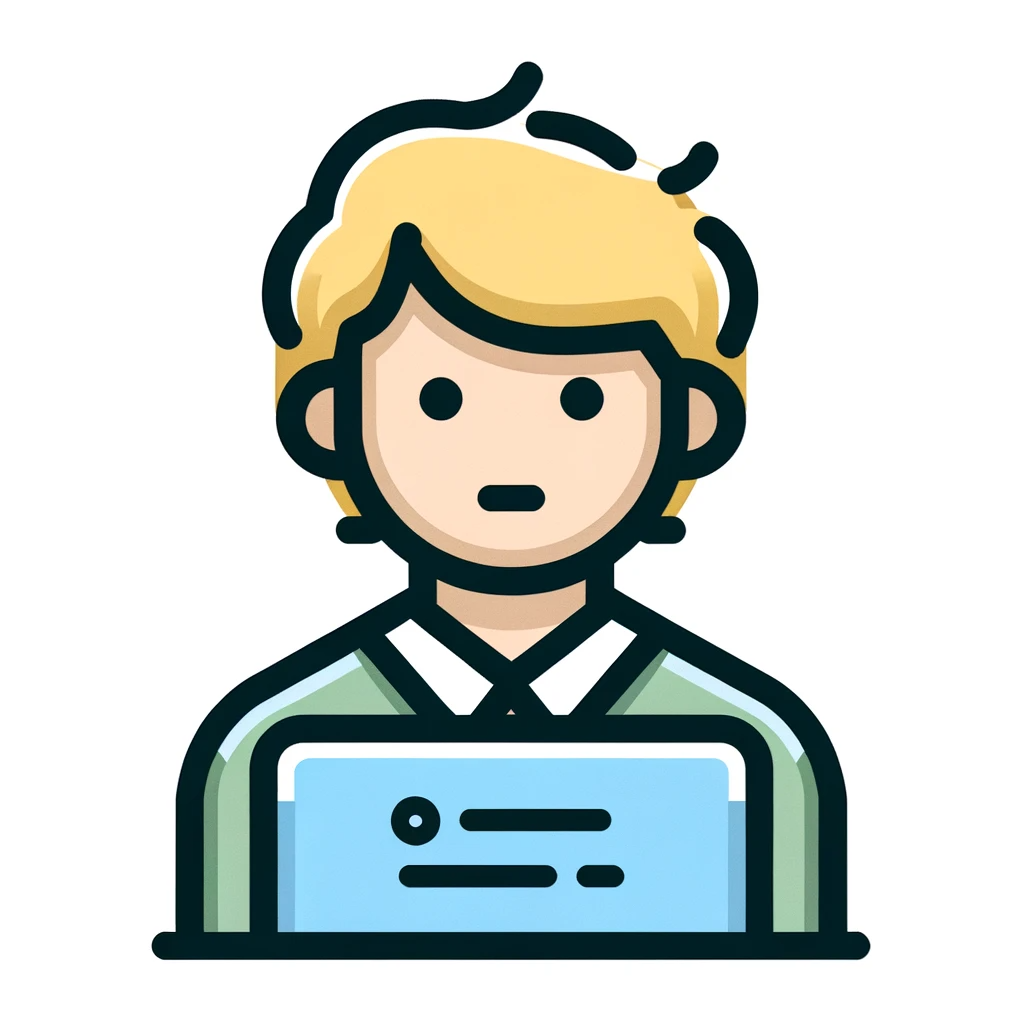
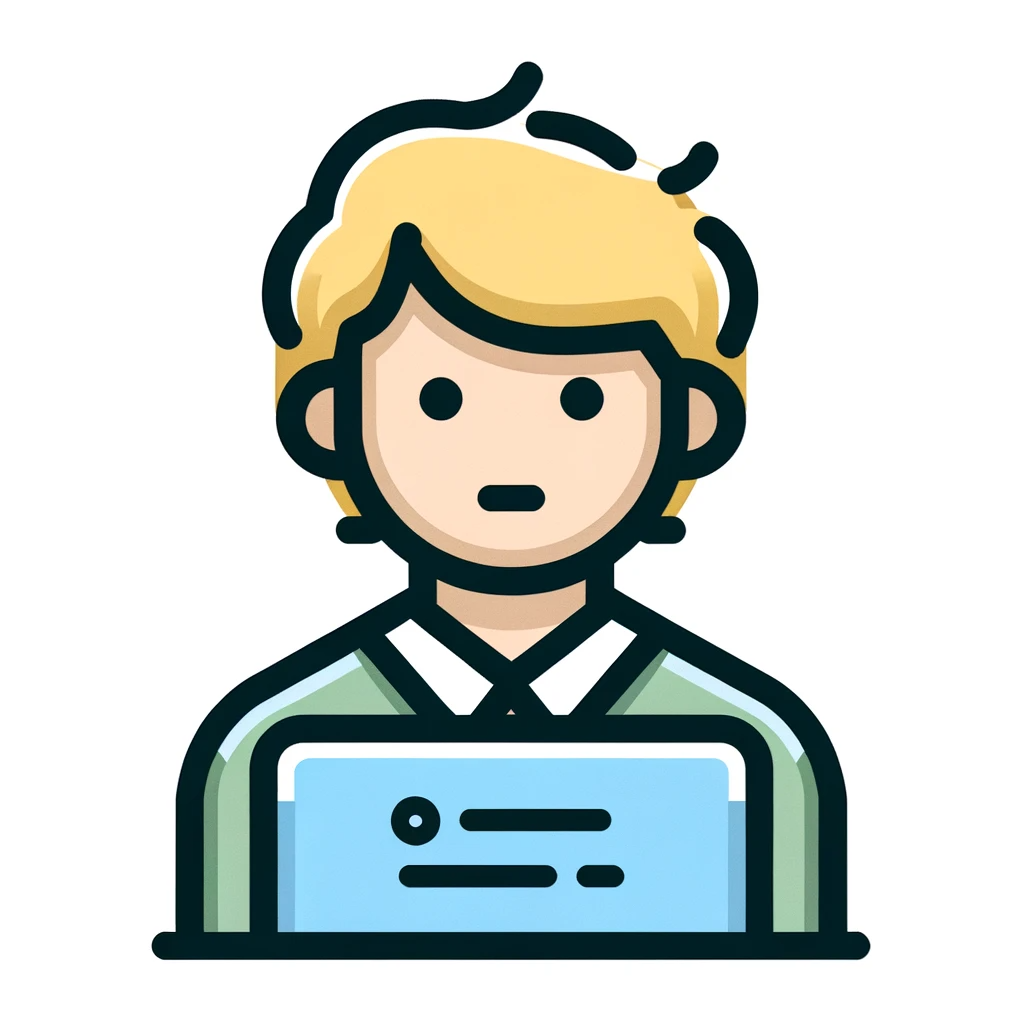
Style classes can now be set and removed dynamically!
By using the classList property, you can easily apply styles, which is very convenient.
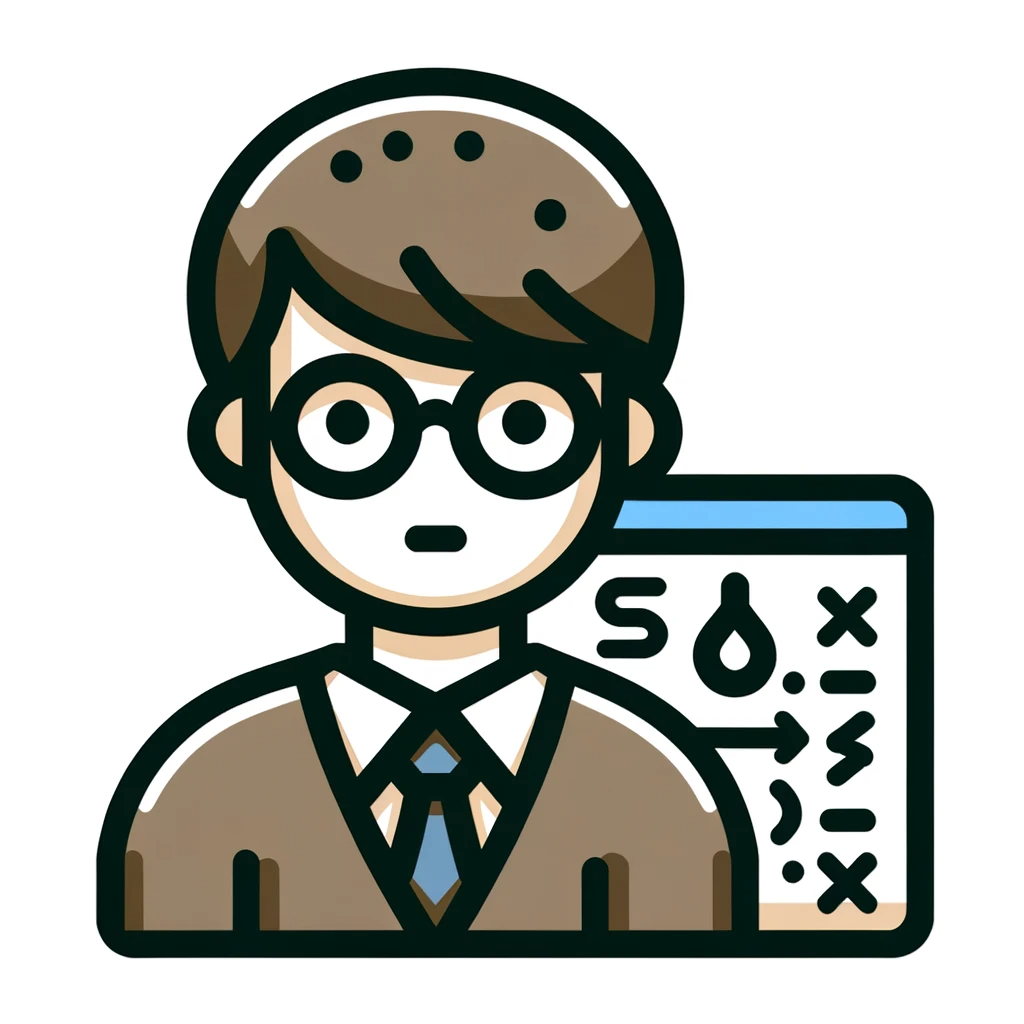
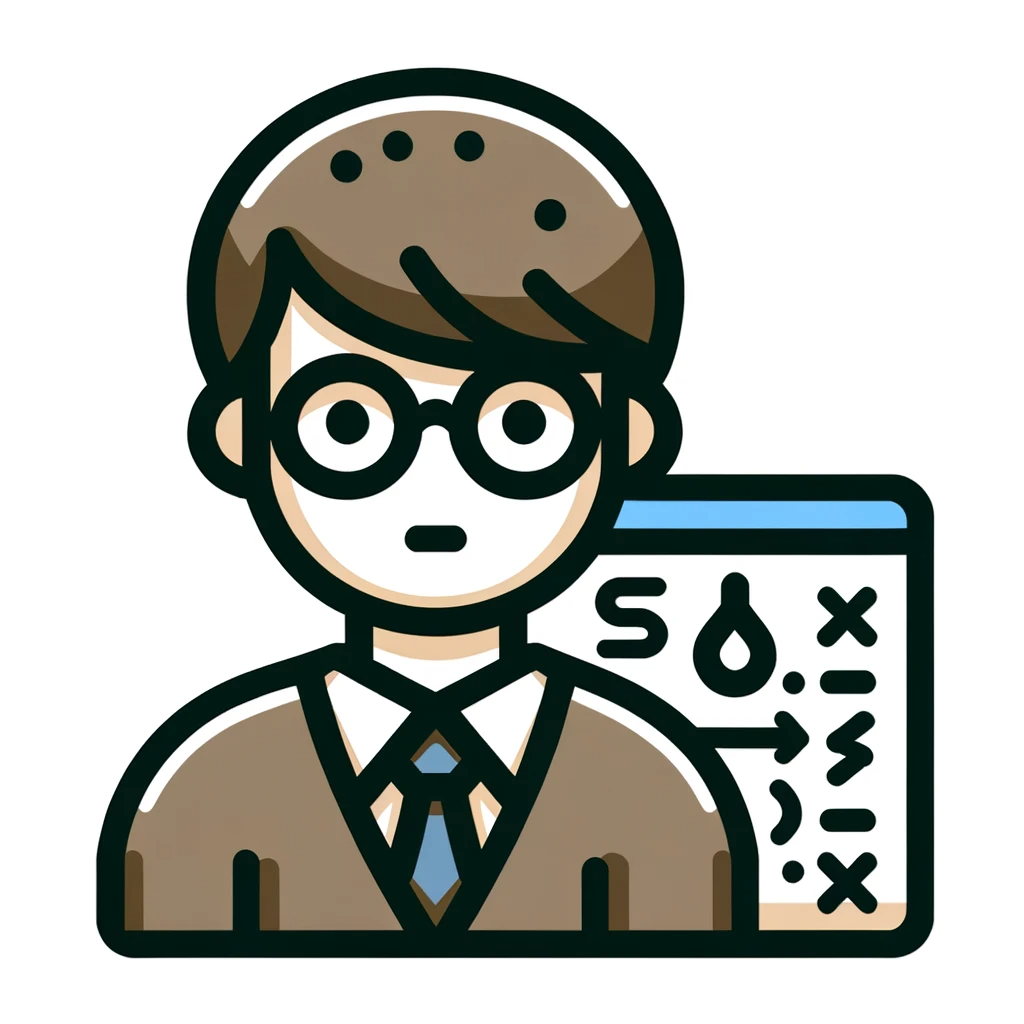
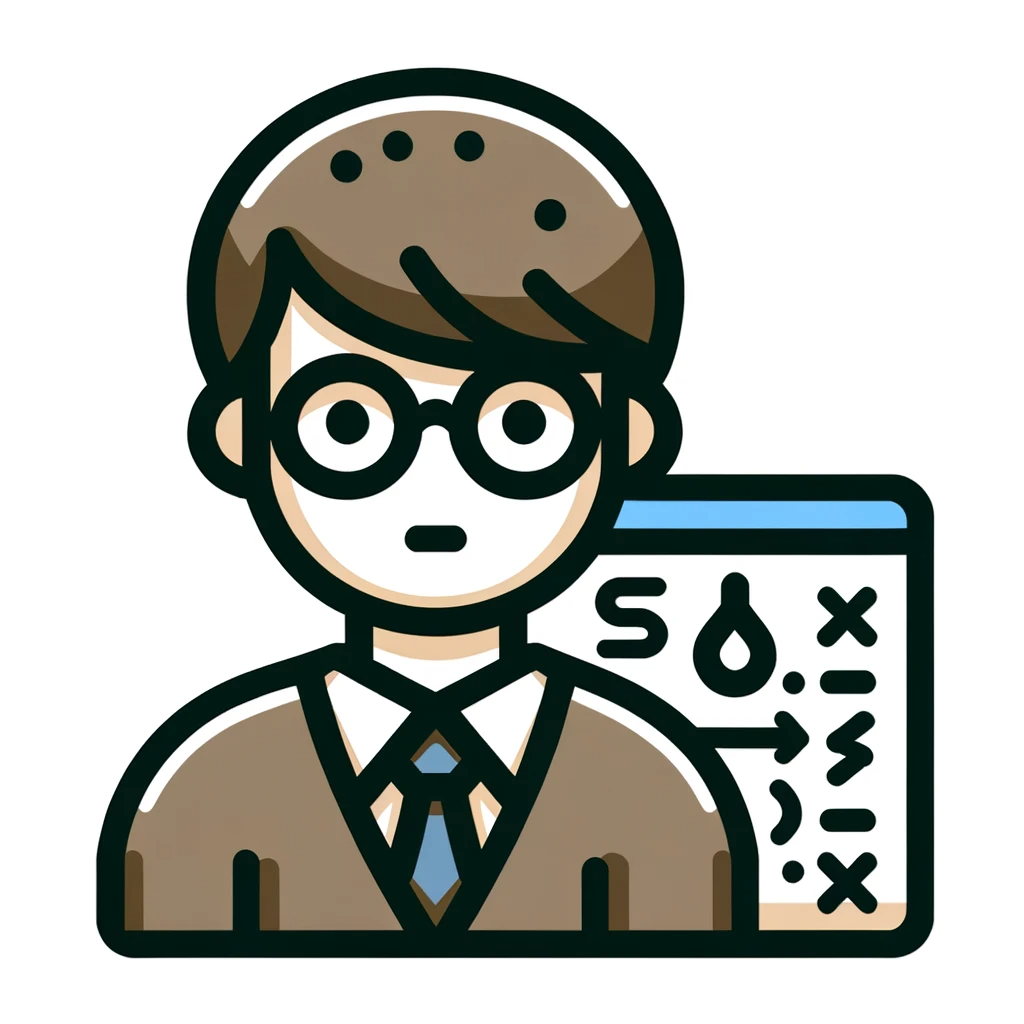
By dynamically setting style classes, you can use JavaScript to create more flexible web pages.
However, use them sparingly, as excessive style changes can disrupt layouts and slow loading speeds.
Comments