This section explains how to process Promise asynchronous processing synchronously in JavaScript .
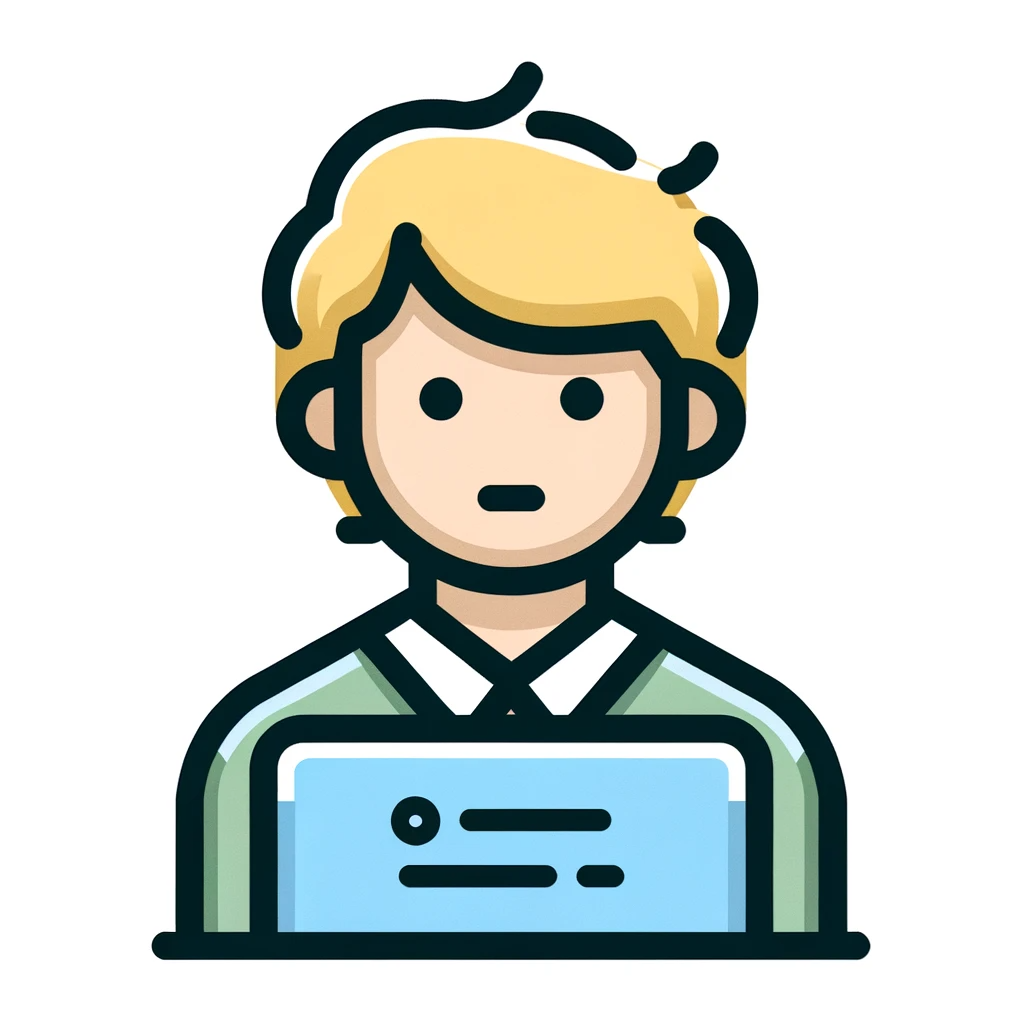
Is there a way to synchronously process asynchronous processing using promises?
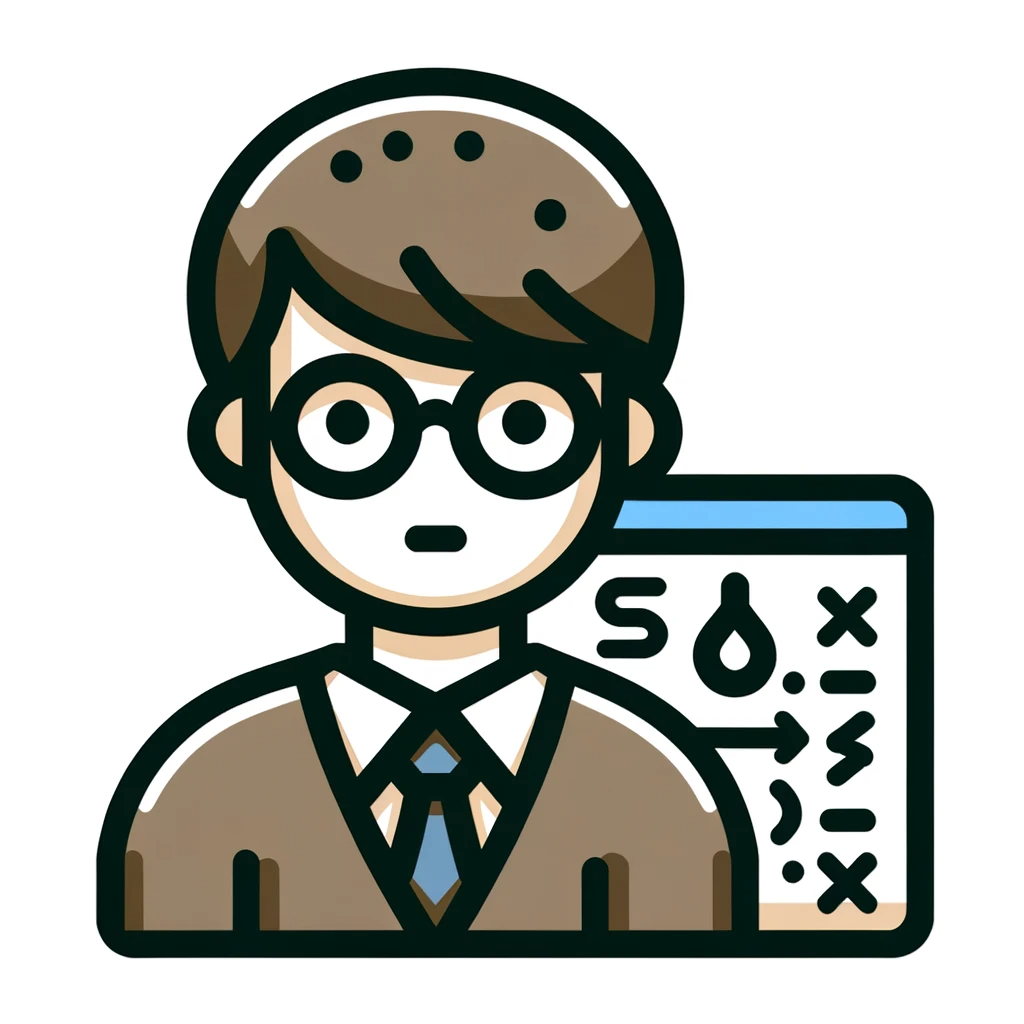
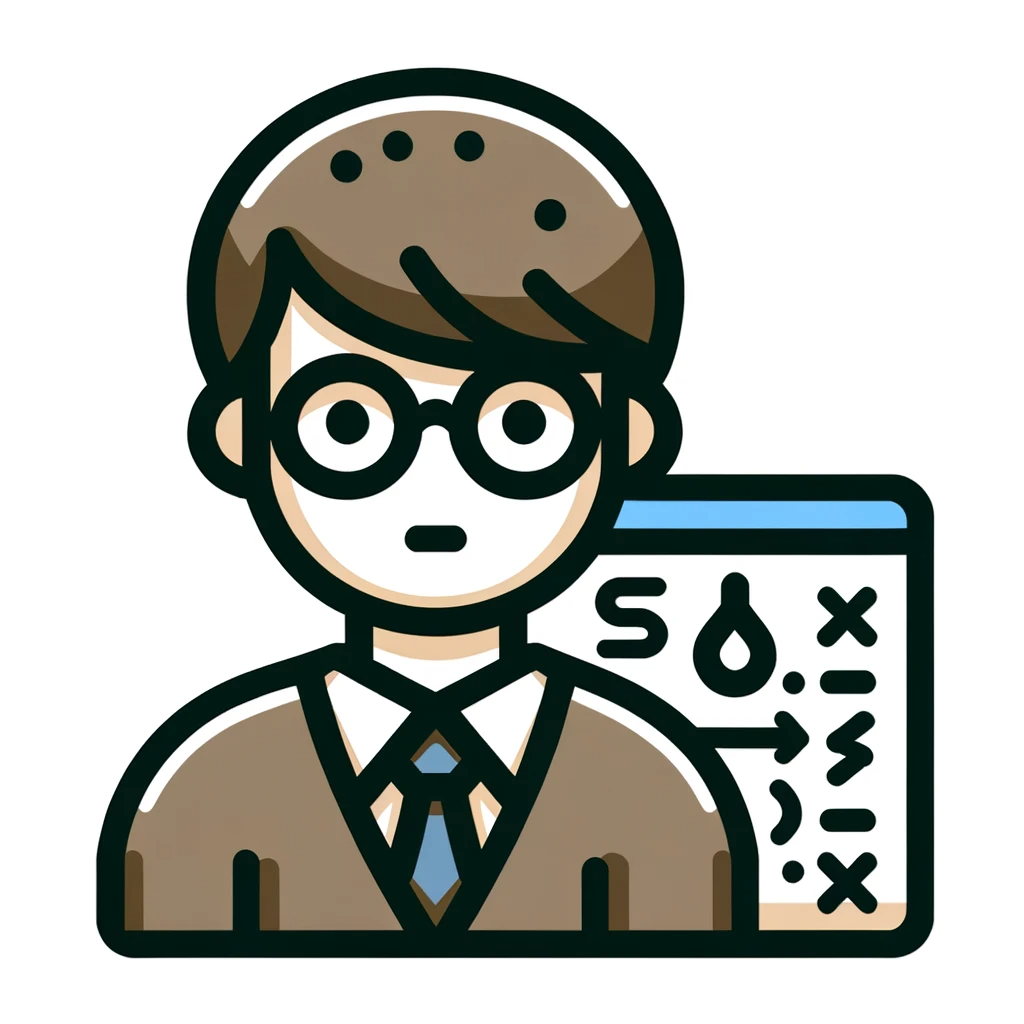
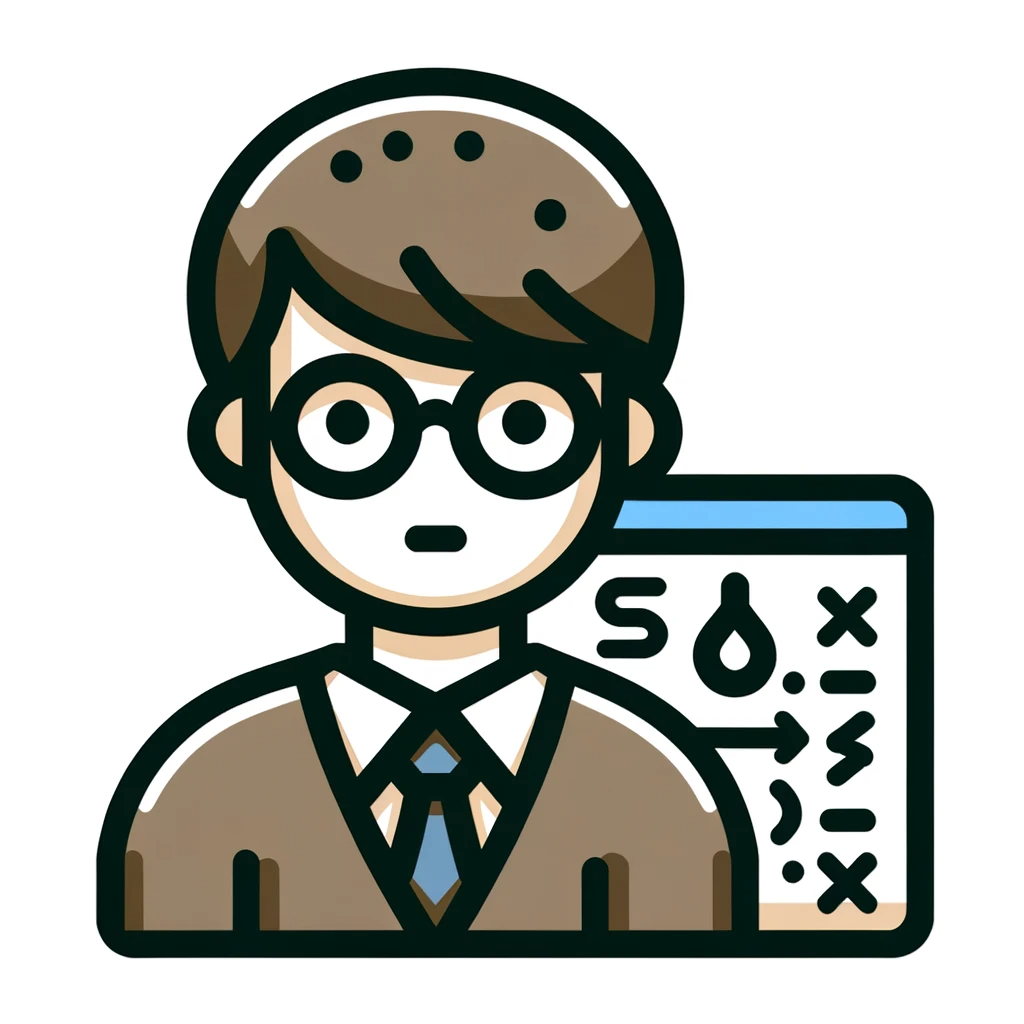
There is a way to process promises synchronously using async/await.
Processing promises synchronously – using async/await
Promise is an object for handling the results of asynchronous processing. Promises are often used when performing asynchronous processing.
However, there are times when you want to treat asynchronous processing like synchronous processing. In such cases, you can use async/await to treat it like synchronous processing.
async/await are keywords for writing asynchronous processing like synchronous processing . By prefixing a function to a function, you can handle asynchronous processing synchronously within that function.
async/await is a new feature introduced in ECMAScript 2017. Therefore, it may not be usable in older browsers or environments.
Also, async/await can only be used in functions that return promises. Therefore, if a function that performs asynchronous processing does not return a Promise, you need to convert it to a Promise using Promise.resolve() or Promise.reject().
Below is a sample program using async/await.
// Functions for asynchronous processing
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('data fetched');
}, 2000);
});
}
// Synchronous processing using async/await
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.log(error);
}
}
getData();
The sample program above shows the fetchData function that performs asynchronous processing and the getData function that performs synchronous processing.
- The fetchData function uses the setTimeout function to return a Promise that returns ‘resolved’ after 2 seconds.
- The getData function
async
is defined with . Thisawait
will allow you to use within this function. - The fetchData function is called within the getData function and the result is assigned to data.
await
By using , you can wait until the fetchData function finishes and receive the results. - For exception handling,
try-catch
I am using. This allows you to handle errors that occur within the fetchData function.
When executed, “data fetched” will be displayed after 2 seconds. This confirms that asynchronous processing is treated like synchronous processing.
Summary
The following is a summary of “How to synchronously process Promise asynchronous processing.”
- To process Promises synchronously in JavaScript, you can use async/await.
- Using async/await, you can treat asynchronous processing like synchronous processing.
- By prefixing a function to a function, you can handle asynchronous processing synchronously within that function.
- By using await, you can wait until an asynchronous process finishes.
- async/await can only be used in functions that return promises.
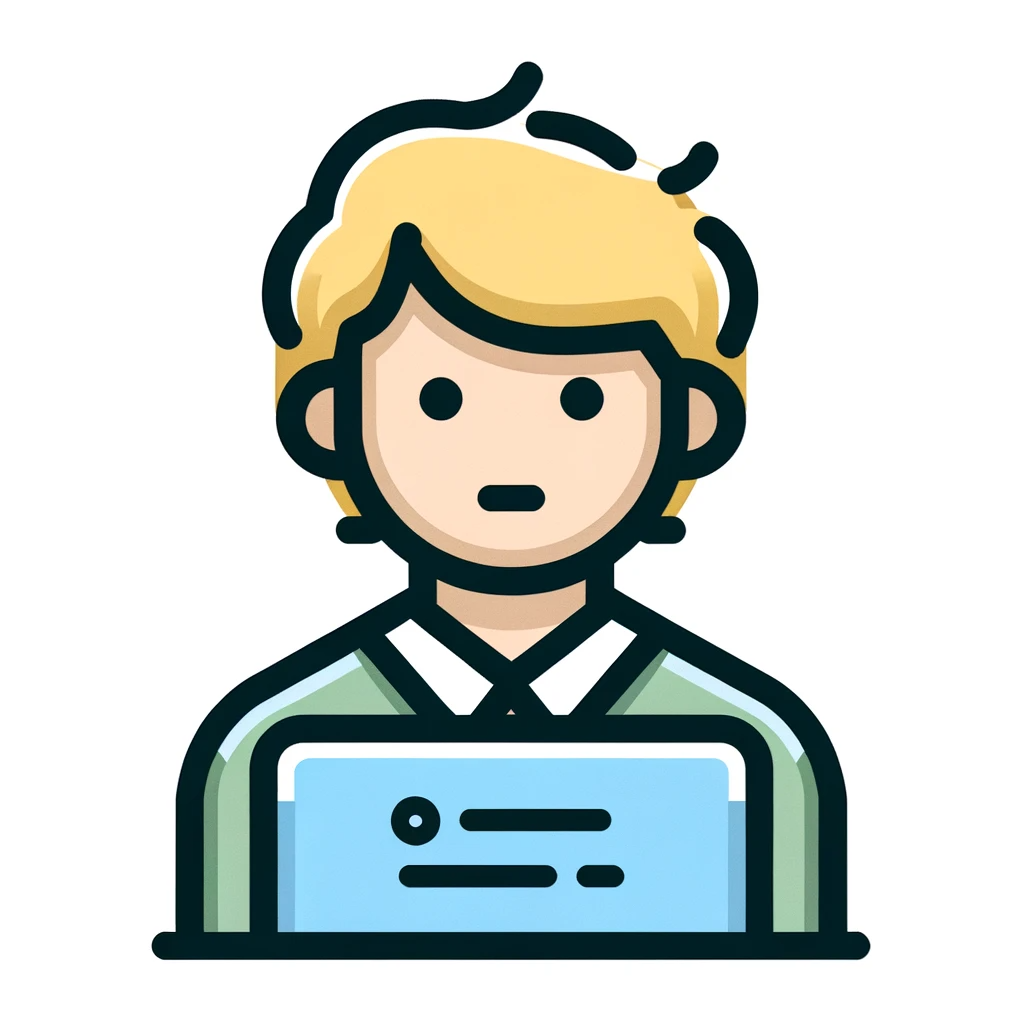
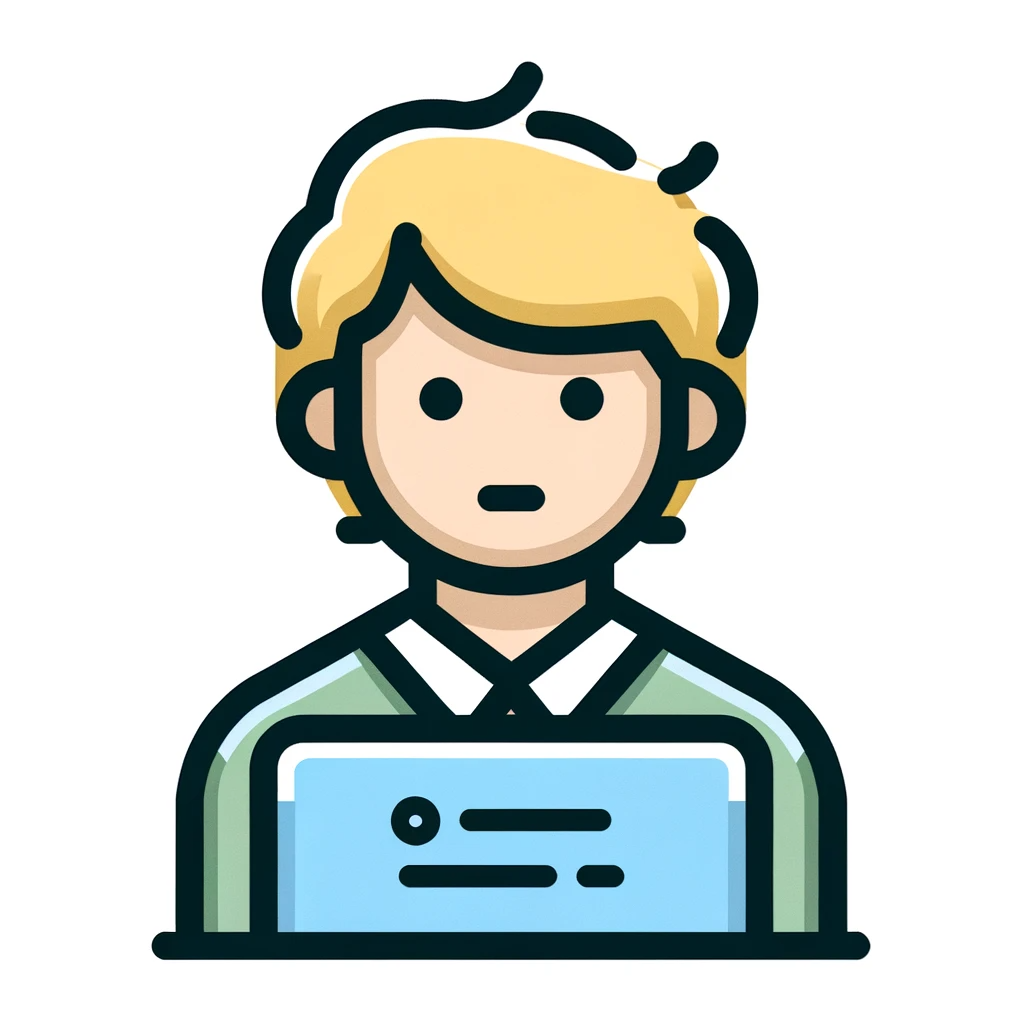
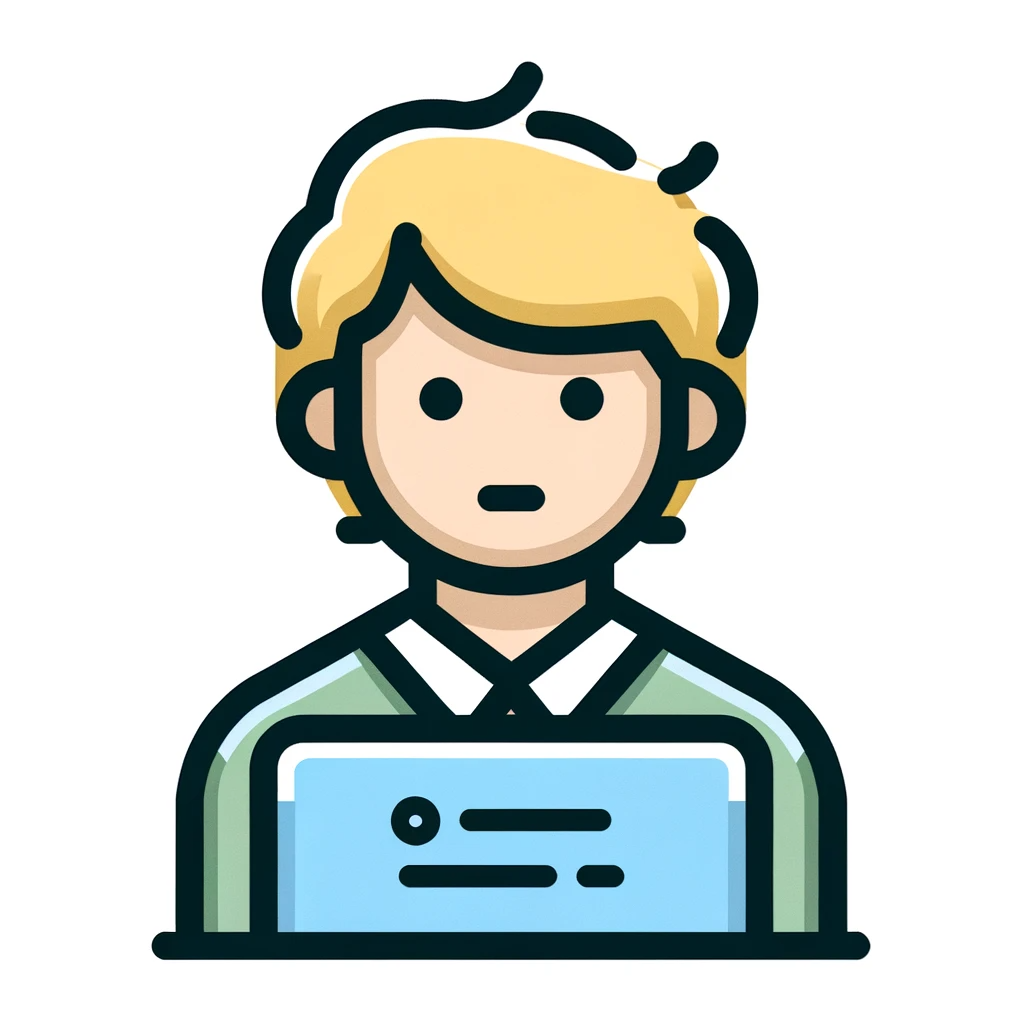
We were able to perform synchronous processing using async/await!
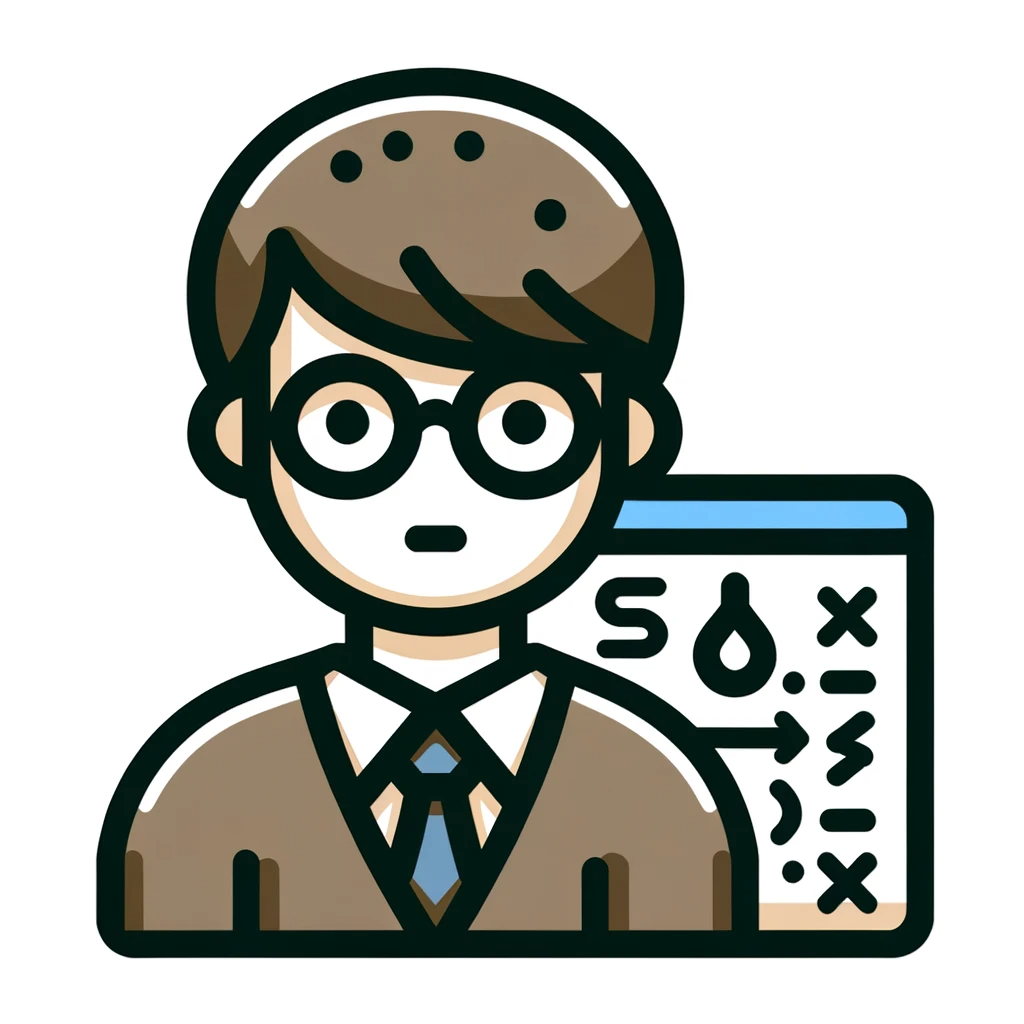
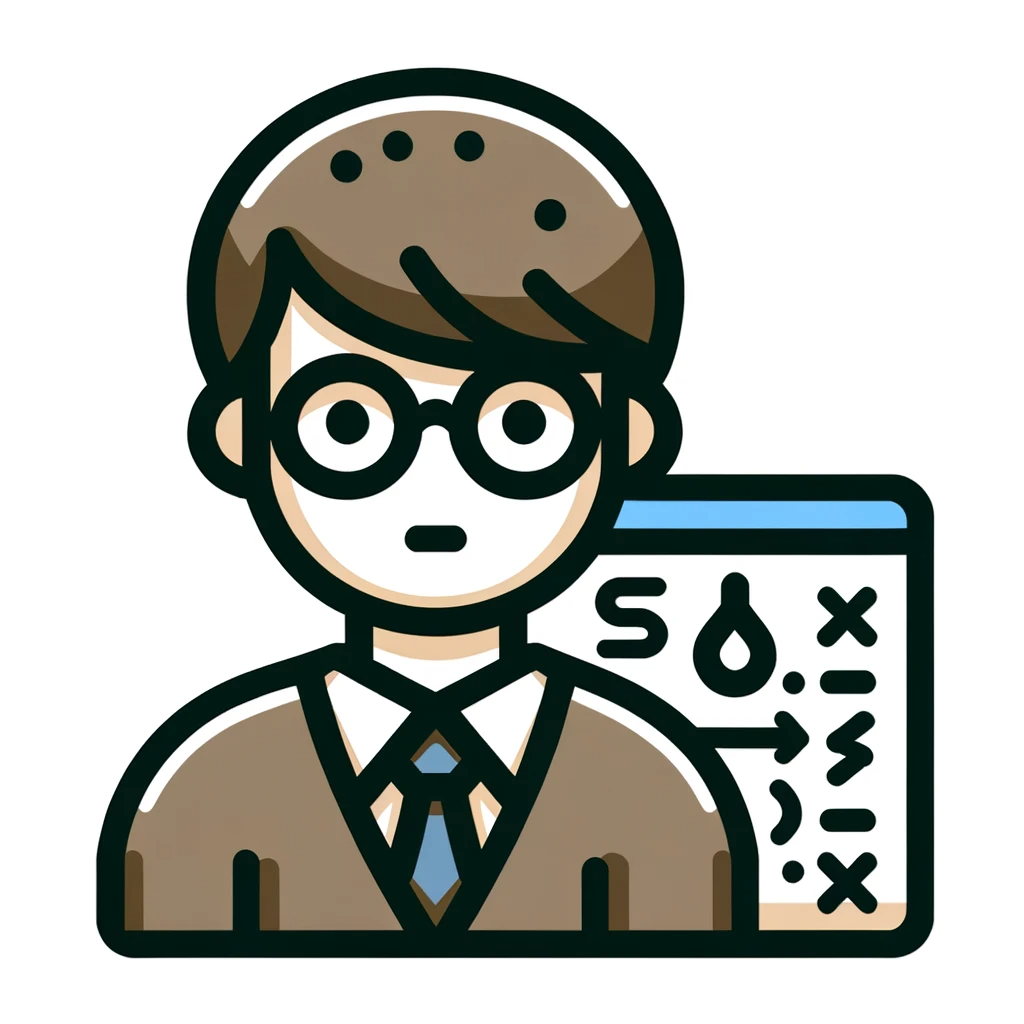
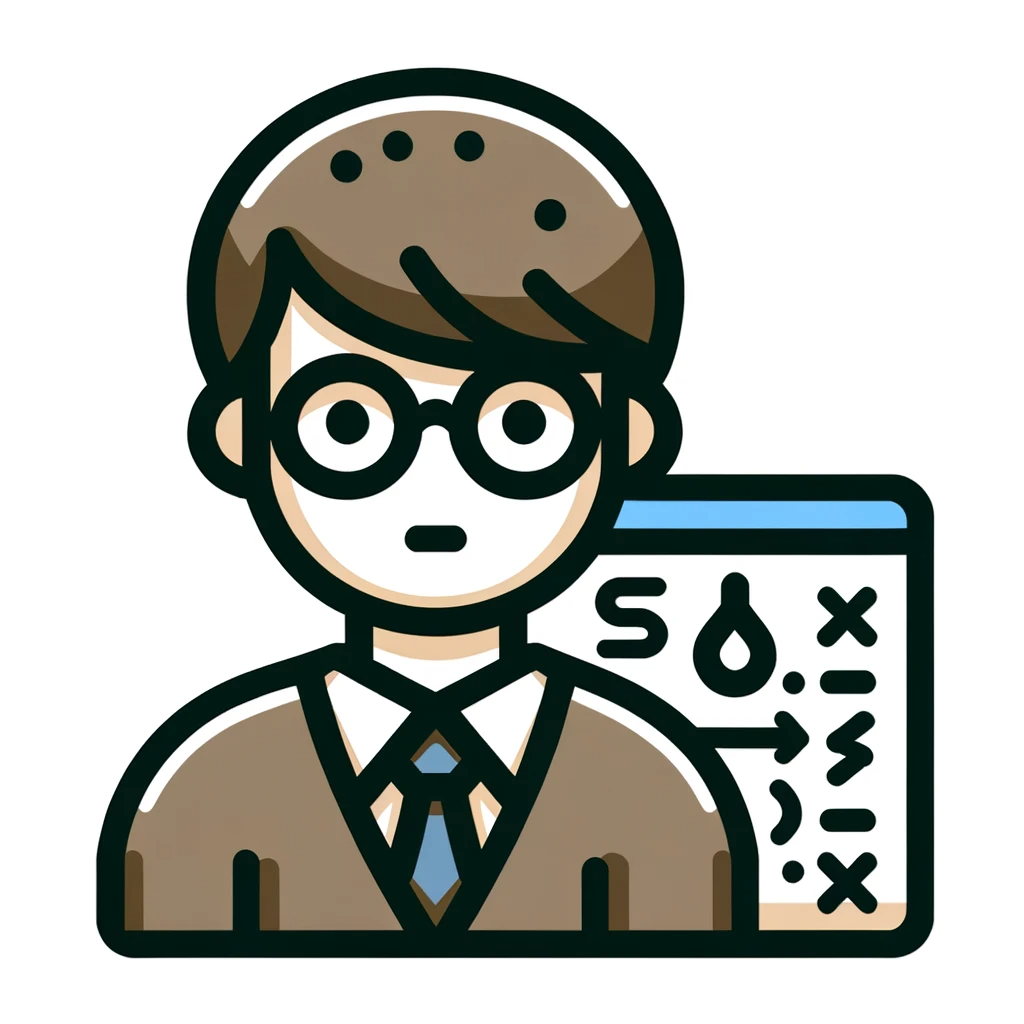
This time, we introduced a method using async/await to synchronously process Promises in JavaScript.
By using async/await, asynchronous processing can be treated like synchronous processing.
Please try it out.
Comments