In JavaScript, an array is a data structure that can store multiple values. By using arrays, you can handle multiple values at the same time. Here you will learn how to define, initialize, copy, and combine JavaScript arrays.
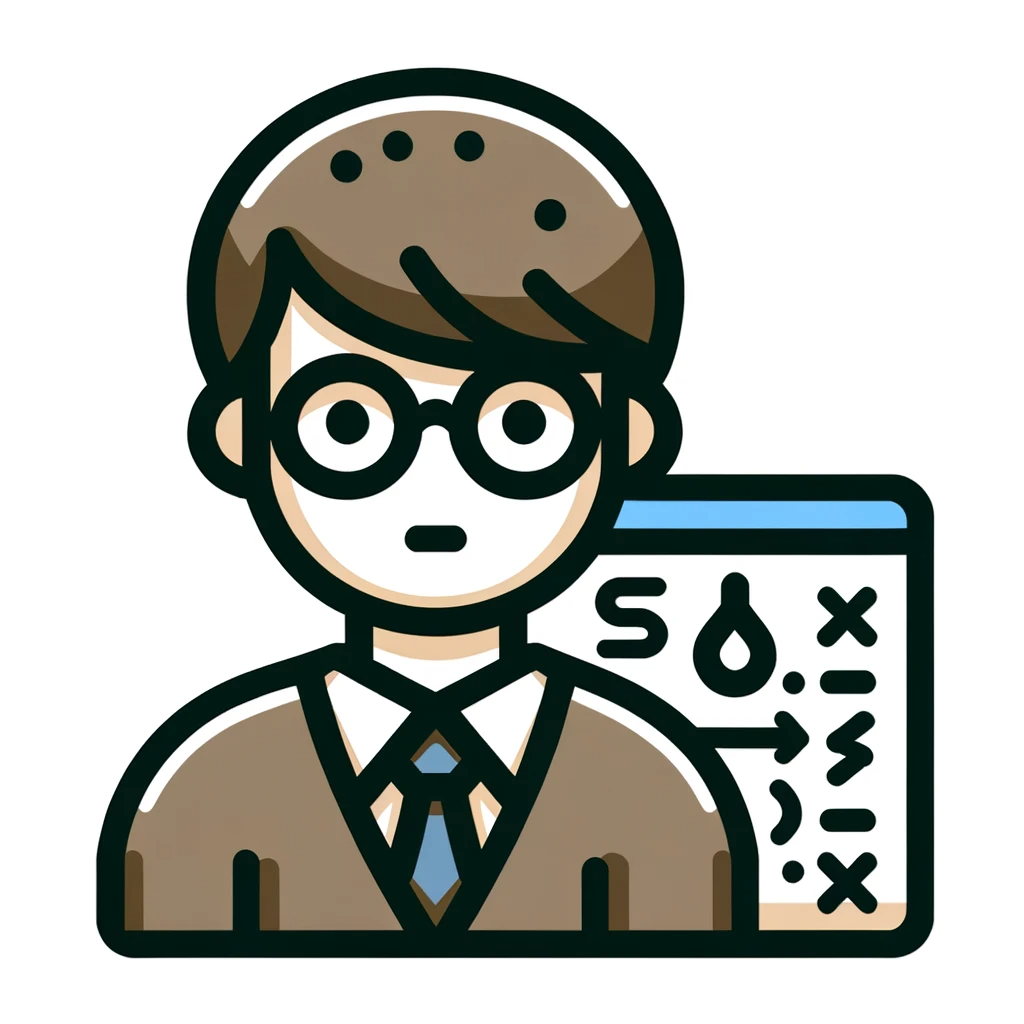
Let’s learn how to create arrays in JavaScript.
What is an array?
An array is a box that can store different data types.
Arrays allow you to handle multiple elements at once . Data can be stored side-by-side, so it can be processed sequentially.
To use arrays, you can define them directly using array literals, or you can create arrays using the Array constructor.
const array1 = [1, 2, 3];
const array2 = new Array(1, 2, 3);
How to write an array
There are two ways to create an array in JavaScript: you can directly define an array using an array literal , or you can create an array using the Array constructor .
Arrays allow you to store data side by side, allowing you to process the data sequentially.
Array definition
To define an array using an array literal , do the following:
const array = [1, 2, 3];
This method []
uses curly braces to define an array and stores comma-separated values as elements.
Also, how to create an array using the Array constructor is as follows.
const array = new Array(1, 2, 3);
This method uses new Array()
to create an array and store comma-separated values as elements.
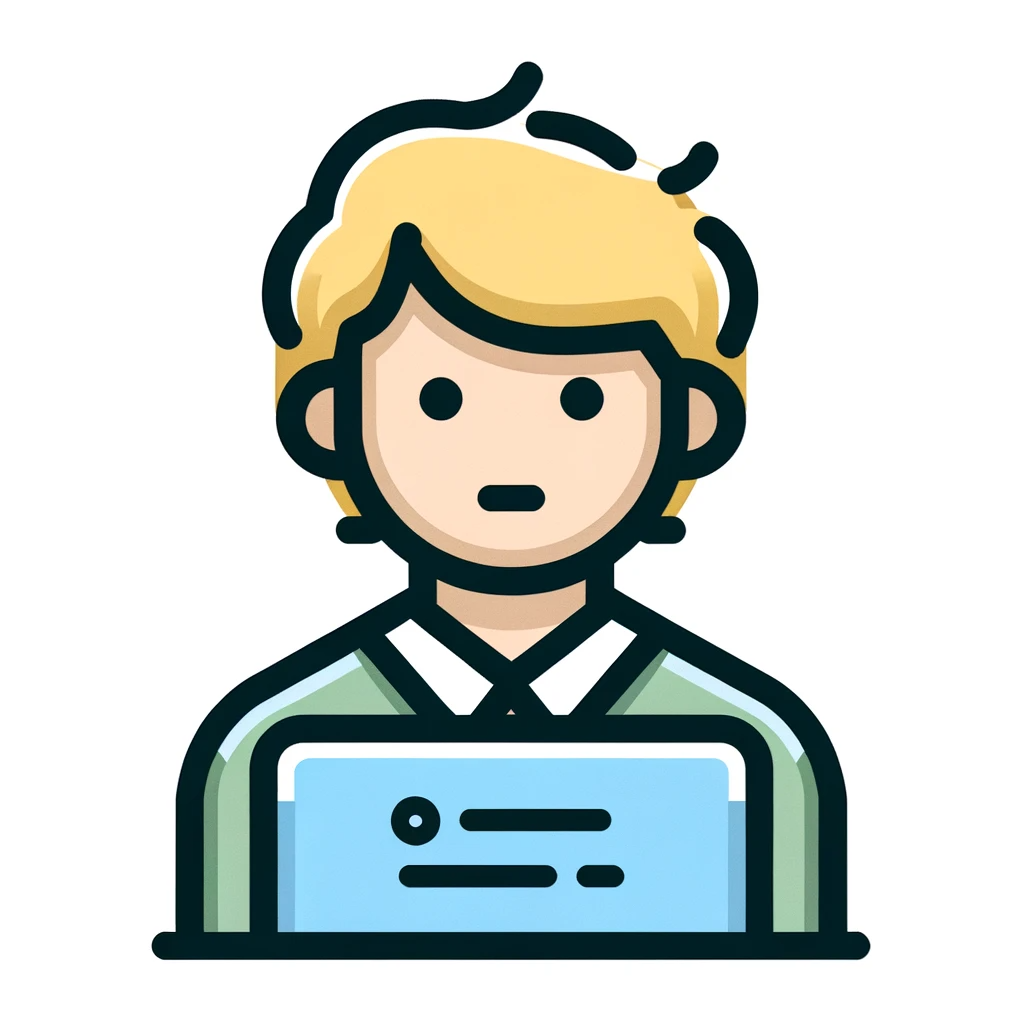
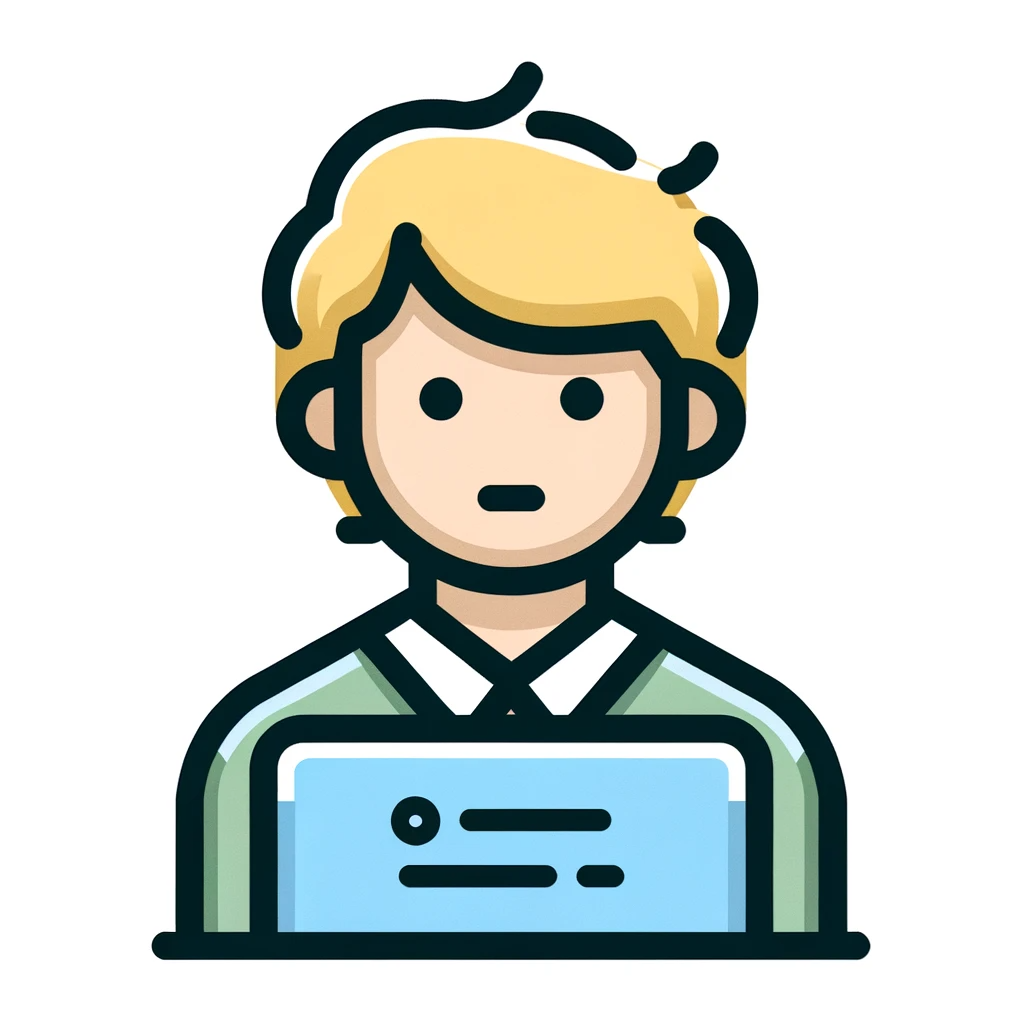
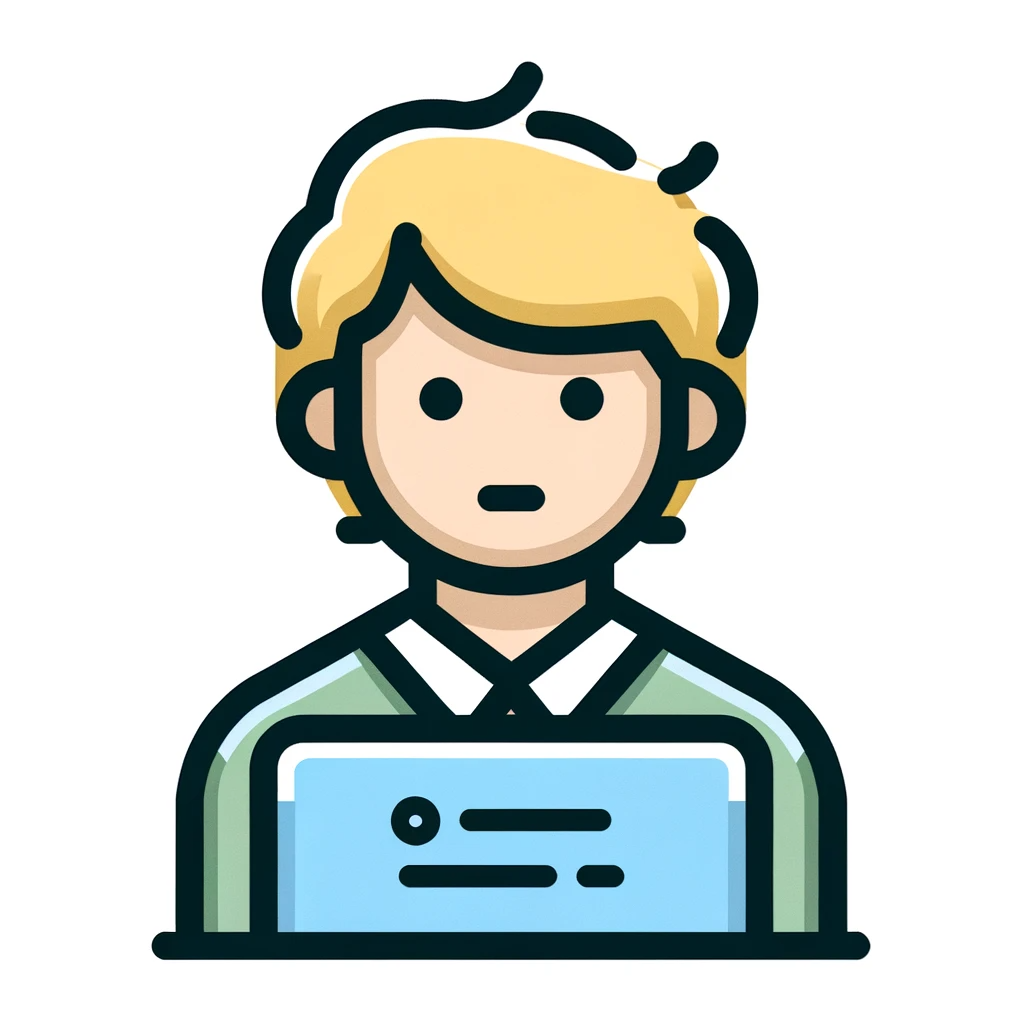
Array literals
seem
simpler and easier to use than Array constructors !
You can also use the Array constructor to create an array with a specified number of elements.
You can create an empty array with 10 elements by doing the following:
const array = new Array(10);
In JavaScript, you can declare an array using const; if you declare an array using const, you cannot reassign it, but you can manipulate its elements.
Array reference
Arrays can be referenced by specifying subscripts as shown below. The first element starts at 0.
console.log(array[1]); // 2
Generating nested arrays
JavaScript allows you to store other arrays as elements of an array. Such an array is called a ” nested array .”
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
console.log(matrix[0][1]); // 2
In JavaScript, the last element can end with a comma.
Initialize an array using a for statement
To initialize the elements of an array, you can also initialize the array using a for statement after creating the array .
const array = new Array(10);
for (let i = 0; i < array.length; i++) {
array[i] = 0;
}
By doing this, you can create an array with 10 elements whose values are all 0.
How to copy an array
You can copy an array using the slice method .
const array1 = [1, 2, 3];
const array2 = array1.slice();
You can also copy using the concat method.
const newArray = [].concat(arrayName);
How to concatenate arrays
Arrays can be concatenated using the concat method .
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const array3 = array1.concat(array2);
You can also combine using the spread operator.
const newArray = [...arrayName1, ...arrayName2];
About associative arrays with a structure similar to arrays
An “associative array” is an object that has key-value pairs, and is a type of JavaScript object.
Regular arrays use numbers as indexes to store values, whereas associative arrays use arbitrary values, such as strings or numbers, as keys to store their corresponding values.
summary
We explained how to define, initialize, copy, and join arrays.
- Arrays allow you to handle multiple elements at once.
- To use arrays, you can define them directly using array literals, or you can create arrays using the Array constructor.
- You can declare an array using const, but you cannot reassign the array, but you can manipulate the elements of the array.
- Another array can be stored as an element of an array.
- JavaScript provides various methods (slice, concat, etc.) to manipulate arrays.
By using arrays, you can handle multiple elements at once. You can also represent complex data structures by using nested arrays. Various methods are provided for manipulating arrays.
By mastering arrays, you can create more efficient programs.
Comments