In JavaScript, we will explain how to implement exception handling when an error occurs in API communication.
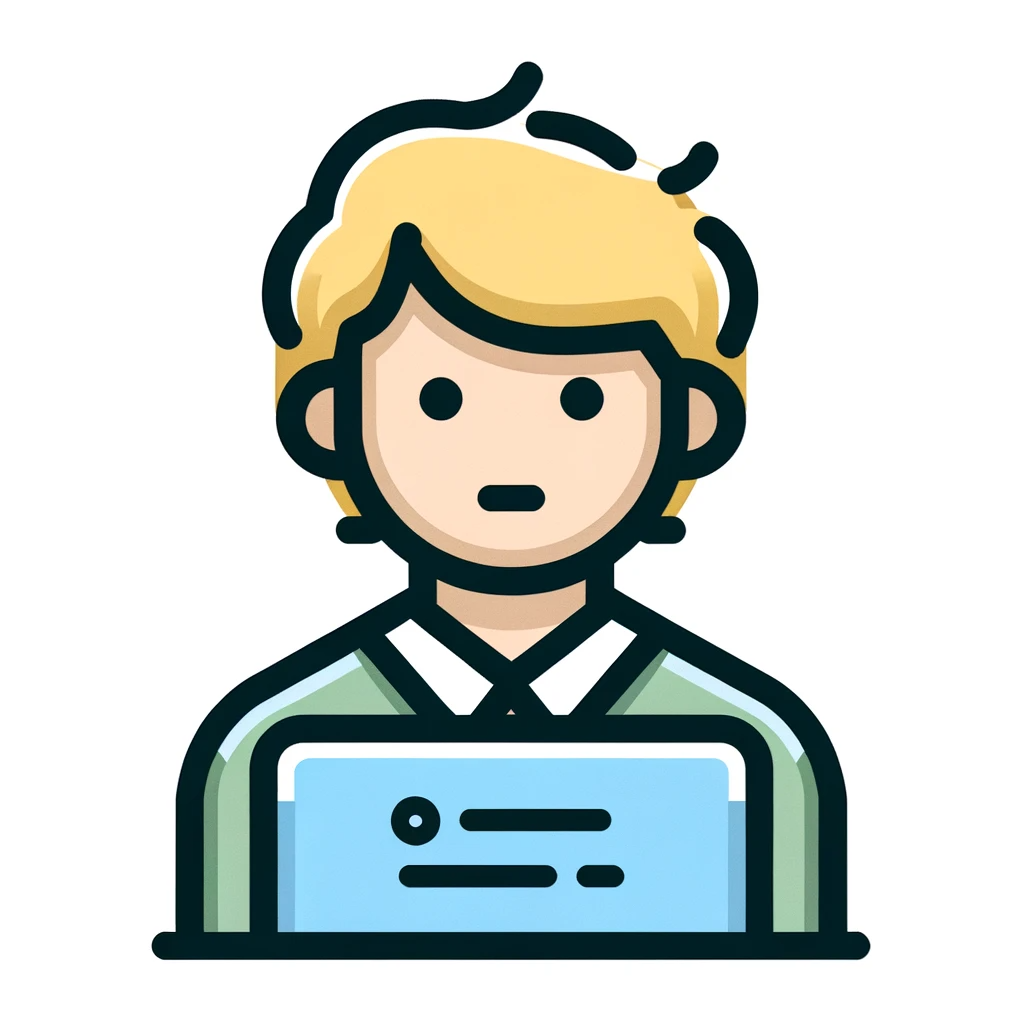
If an error occurs during API communication with JavaScript, how should I implement exception handling?
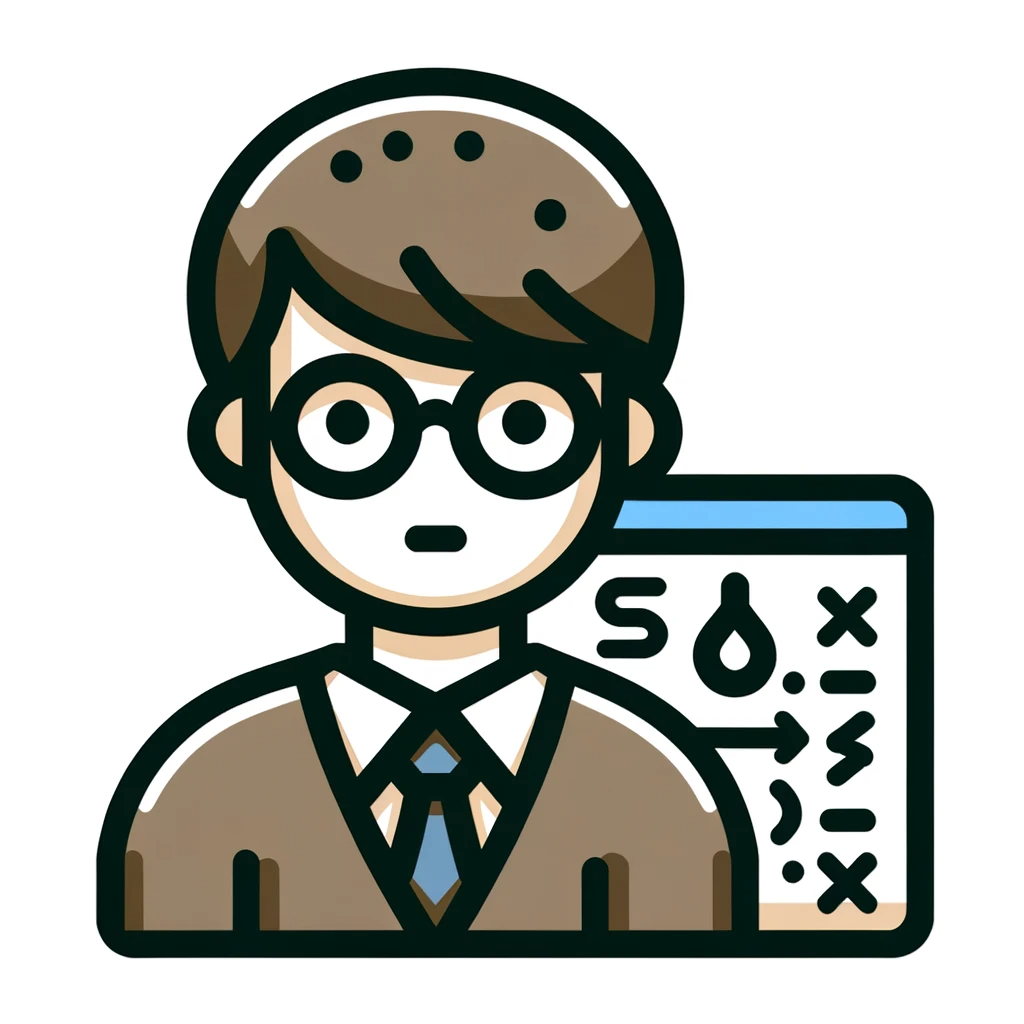
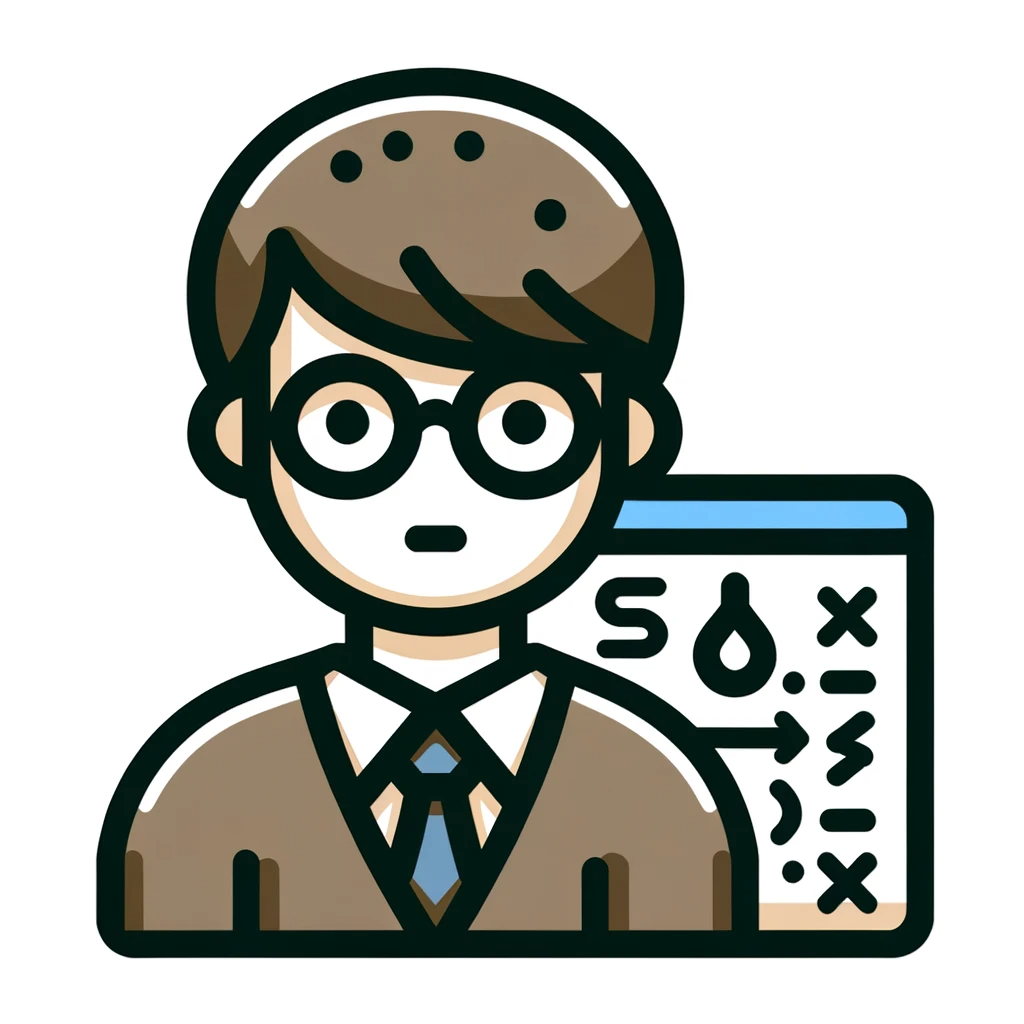
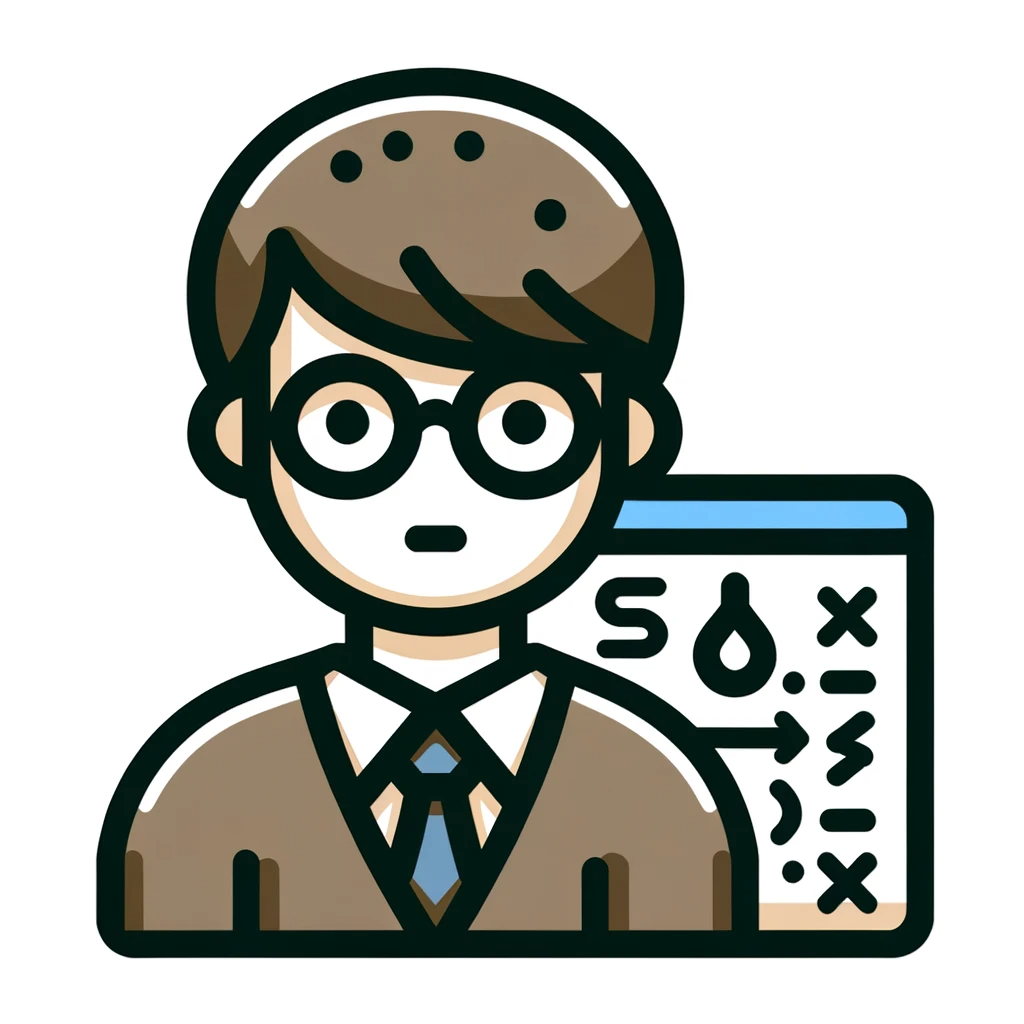
Let’s explain how to handle exceptions using try-catch when API communication errors occur.
Importance of exception handling
If an error occurs in API communication, leaving it unattended may cause serious problems such as system outage. Therefore, it is important to implement exception handling.
Implementing exception handling is especially important for API communication . If a communication error or server error occurs and the program stops, the reliability of the system will decrease for users as well. Therefore, even in API communication, it is necessary to implement exception handling using try-catch statements and output appropriate error messages.
Exception handling is an important effort to improve system reliability and stability. System quality can be improved by responding to unexpected errors. Therefore, it is necessary to implement exception handling appropriately in the program.
How to implement exception handling in API communication
In API communication, errors may occur, so it is important to implement exception handling. If a communication error occurs, the program will stop, so appropriate exception handling is required.
The way to implement exception handling in API communication is to use try-catch statements. The try block describes the communication process, and the catch block describes the process to be executed when a communication error occurs.
Explanation using a sample program
The following is a sample program that uses the fetch method to perform API communication and outputs an error message if an error occurs.
try {
const response = await fetch('https://example.com/api/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('An error occurred in API communication.', error);
}
Inside the try block, we use the fetch method to communicate with the API. If the communication completes successfully, process the data obtained in the then block. On the other hand, if a communication error occurs, control is transferred to the catch block. A catch block prevents the program from stopping when an error occurs by outputting an error message.
Note that exception handling can also be implemented using try-catch statements in API communications other than the fetch method as well. For example, if you use XMLHttpRequest, write the XMLHttpRequest processing in a try block and perform error handling in a catch block.
While it is important to perform normal communication processing, it is also necessary to properly implement error handling in order to increase the reliability and stability of the system.
summary
We explained how to implement exception handling when an error occurs in API communication.
- In API communication, it is important to implement exception handling.
- You can use the try-catch statement to implement processing when an error occurs.
- Describe the API communication process in the try block, and describe the process when an error occurs in the catch block.
- It is necessary to perform appropriate error handling such as outputting error messages.
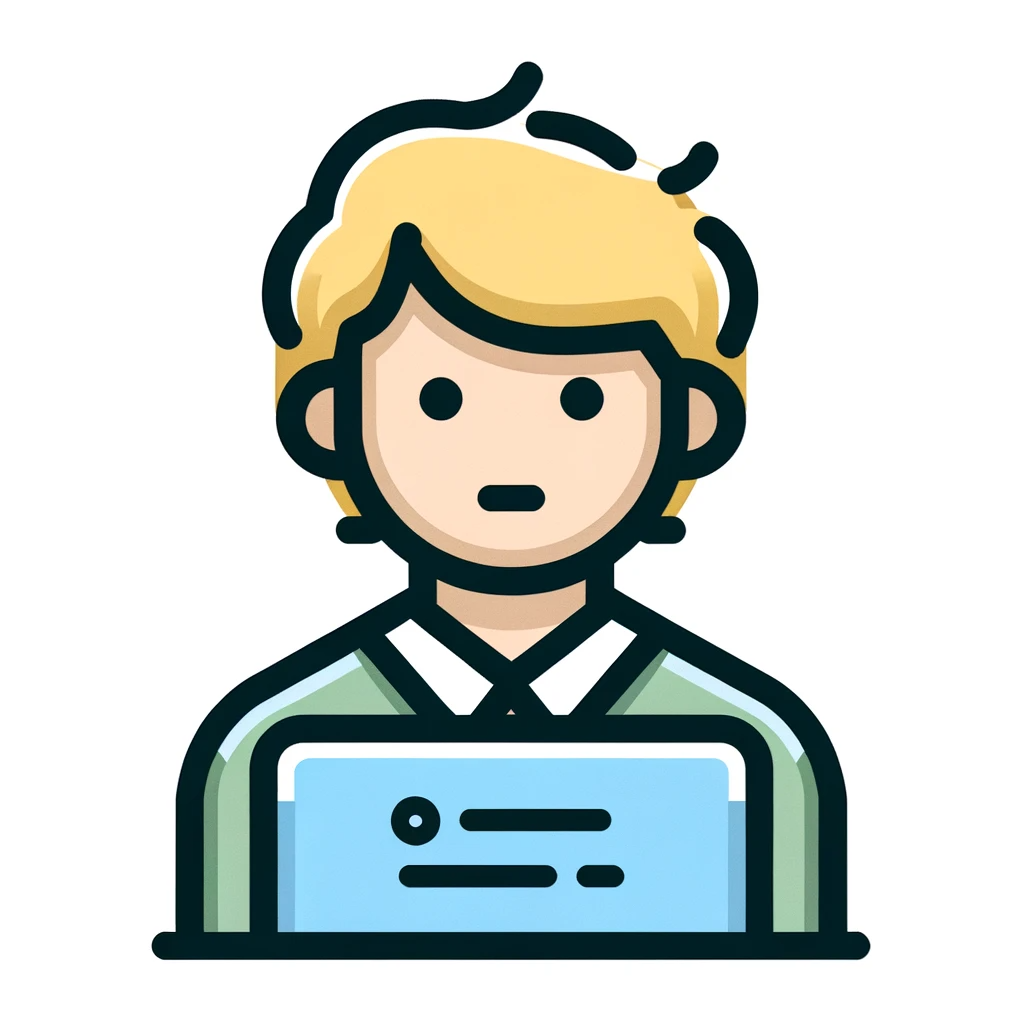
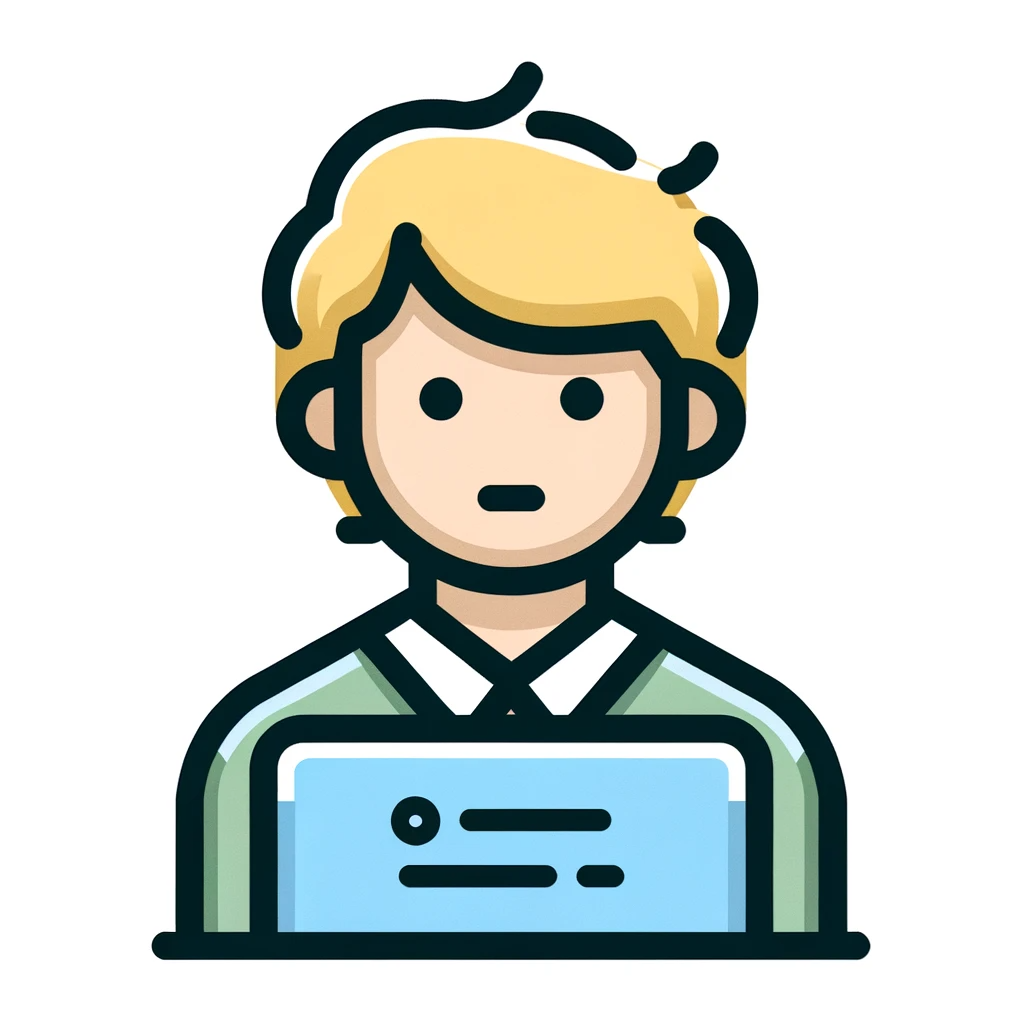
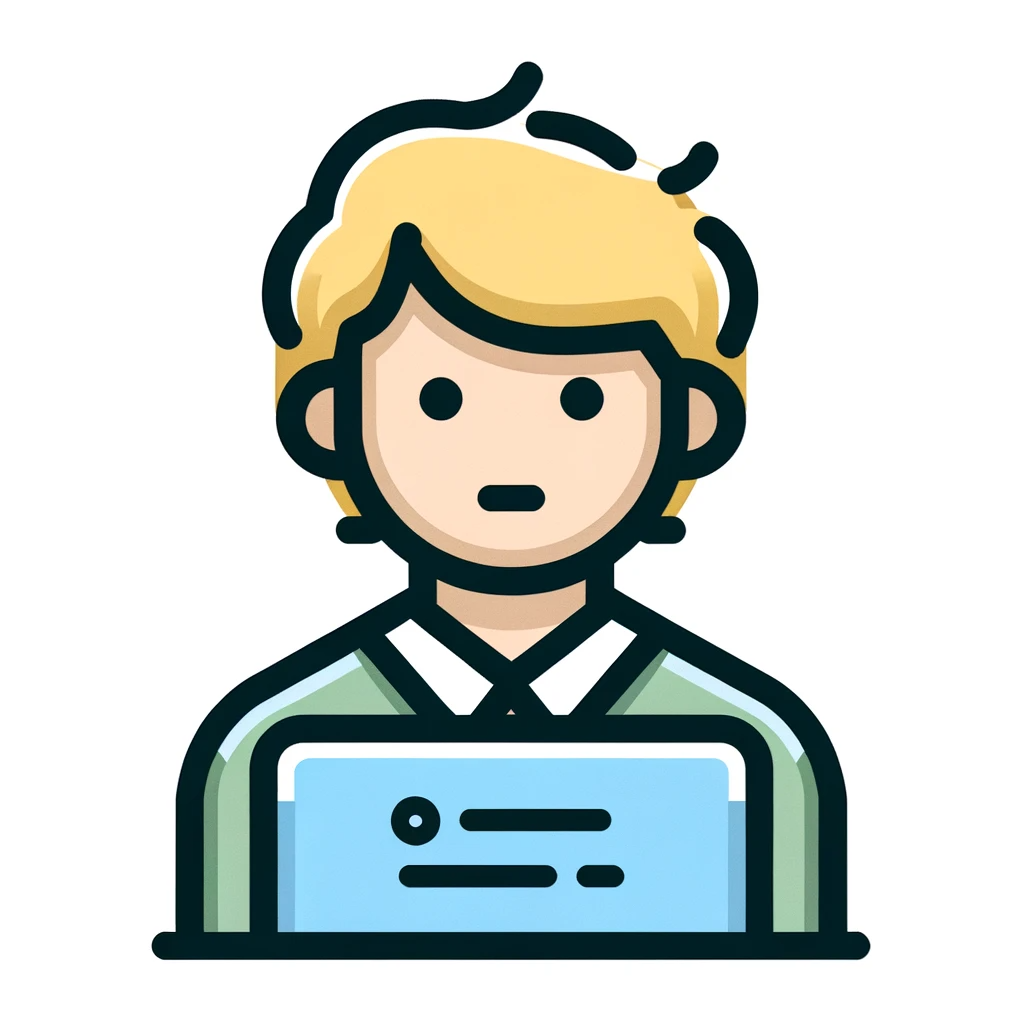
I learned that it is essential to implement exception handling when communicating with APIs using JavaScript.
I felt that making good use of try-catch statements and outputting appropriate error messages is important for increasing the reliability and stability of programs.
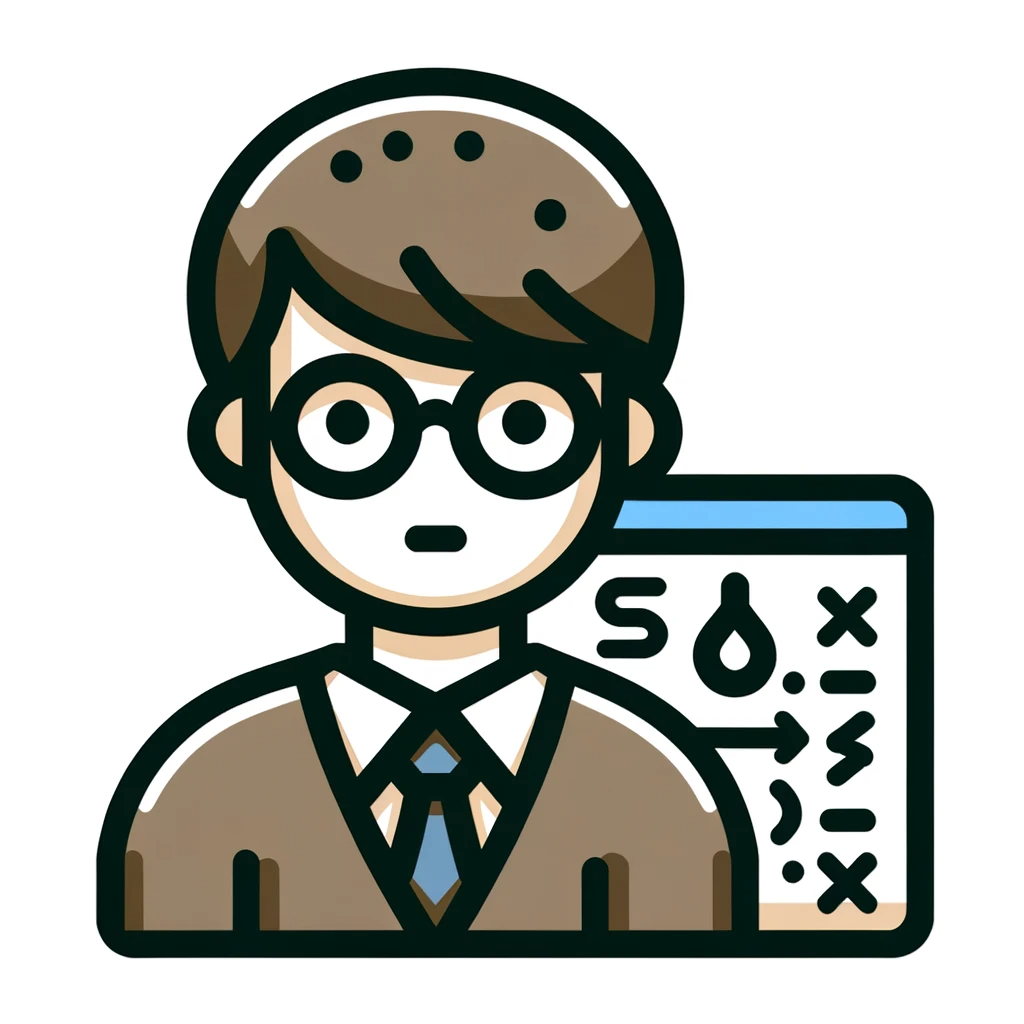
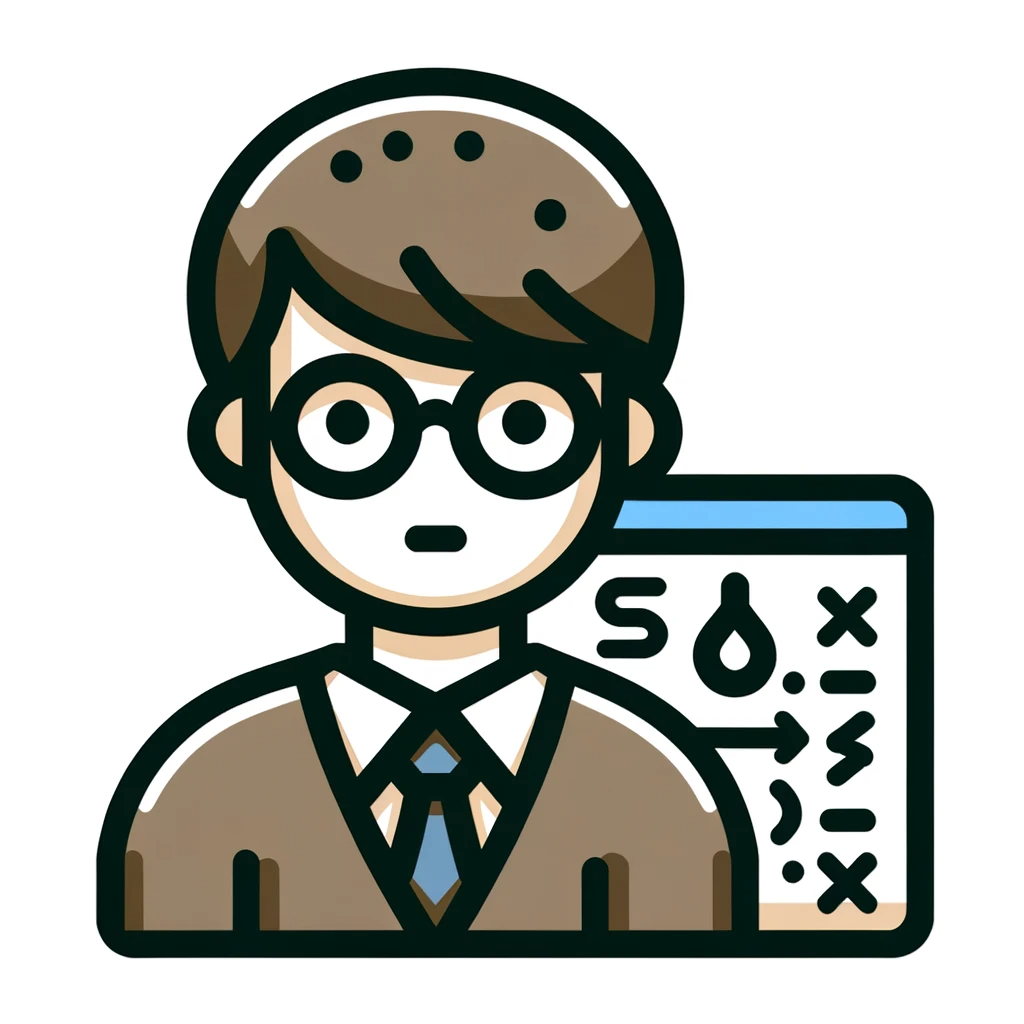
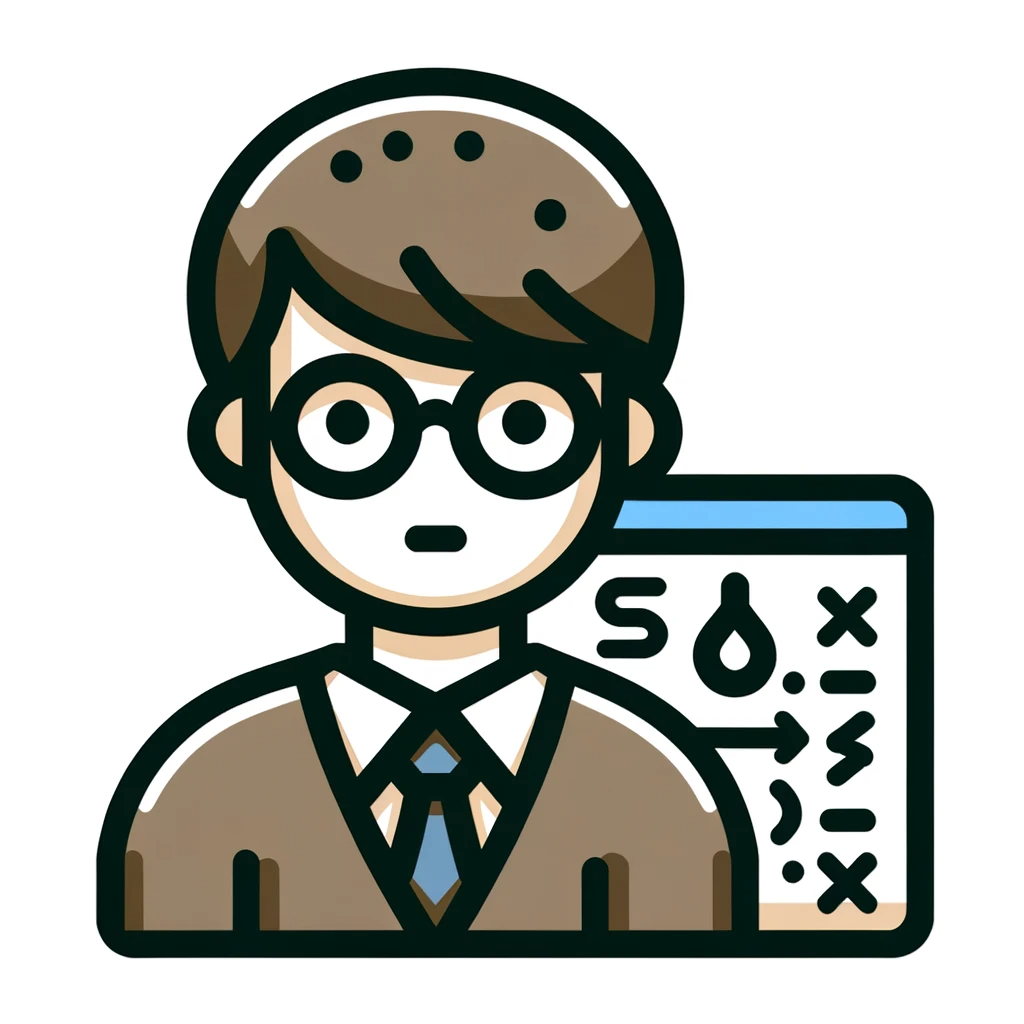
Implementing exception handling in API communication is a necessary effort to improve system reliability and stability.
You can improve the quality of your program by making good use of try-catch statements and outputting appropriate error messages.
It is also important to handle not only communication errors but also error responses from the API appropriately.
Don’t forget to read the API specifications carefully and implement the necessary error response processing.
Comments