We will explain ” How to find the absolute value, minimum value, and maximum value ” using JavaScript.
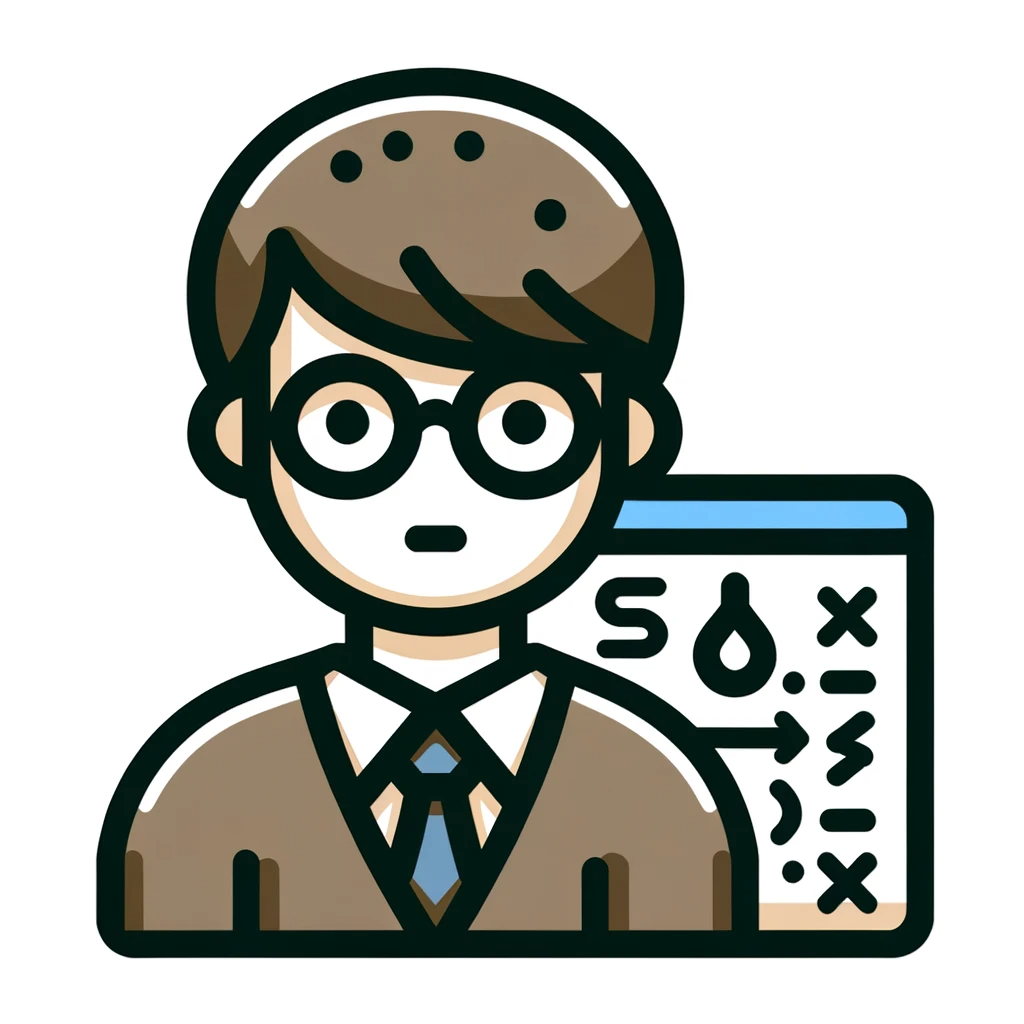
JavaScript provides functions for finding absolute values, minimum values, and maximum values.
This article details how to use those functions.
How to find the absolute value
To find the absolute value, use the abs() method of the Math object.
This method returns the absolute value of the given number.
Math.abs(number);
For example, the code below finds the absolute value of the number stored in variable x.
var x = -10;
var absX = Math.abs(x);
console.log(absX); // 10
How to find the minimum value
One way to find the minimum value is to use the min() method of the Math object.
The Math object’s min() method returns the minimum value from among multiple numbers passed as arguments.
Math.min( number1,number2… );
The example below uses the min() method to find the minimum value of a number.
var a = 3;
var b = 7;
var c = 1;
var d = 9;
var e = 2;
var min = Math.min(a, b, c, d, e);
console.log(min); // 1
How to find the maximum value
One way to find the maximum value is to use the max() method of the Math object.
The max() method of the Math object returns the maximum value among multiple numbers passed as arguments.
Math.max(number1,number2…);
The example below uses the max() method to find the maximum value of a number.
var a = 3;
var b = 7;
var c = 1;
var d = 9;
var e = 2;
var max = Math.max(a, b, c, d, e);
console.log(max); // 9
In this way, you can use the max() method to find the maximum value.
Other ways to find maximum and minimum values
How to find the maximum and minimum values with a for loop
You can also use a for loop to find the maximum or minimum value.
The example below shows how to use a for loop to compare the elements of an array in order and find the maximum value.
var a = [3, 7, 1, 9, 2];
var max = a[0];
for (var i = 1; i < a.length; i++) {
if (a[i] > max) {
max = a[i];
}
}
console.log(max); // 9
How to get maximum and minimum values using apply() method
You can also use the apply() method to get the maximum and minimum values from an array of numbers. The apply() method allows you to call a function while changing the this keyword.
In the example below, the max() method of the Math object is used along with the apply() method to get the maximum value of an array.
var a = [3, 7, 1, 9, 2];
var max = Math.max.apply(null, a);
console.log(max); // 9
summary
The table below summarizes ” methods for finding absolute values, small values, and large values .”
method | explanation |
---|---|
Math.abs(x) | returns the absolute value of x |
Math.min(a, b, c, …) | Returns the smallest value among 0 or more numbers |
Math.max(a, b, c, …) | Returns the largest value among 0 or more numbers |
Using these methods, you can easily perform calculations. In particular, calculations such as comparing numbers and finding absolute values can be easily implemented using these methods.
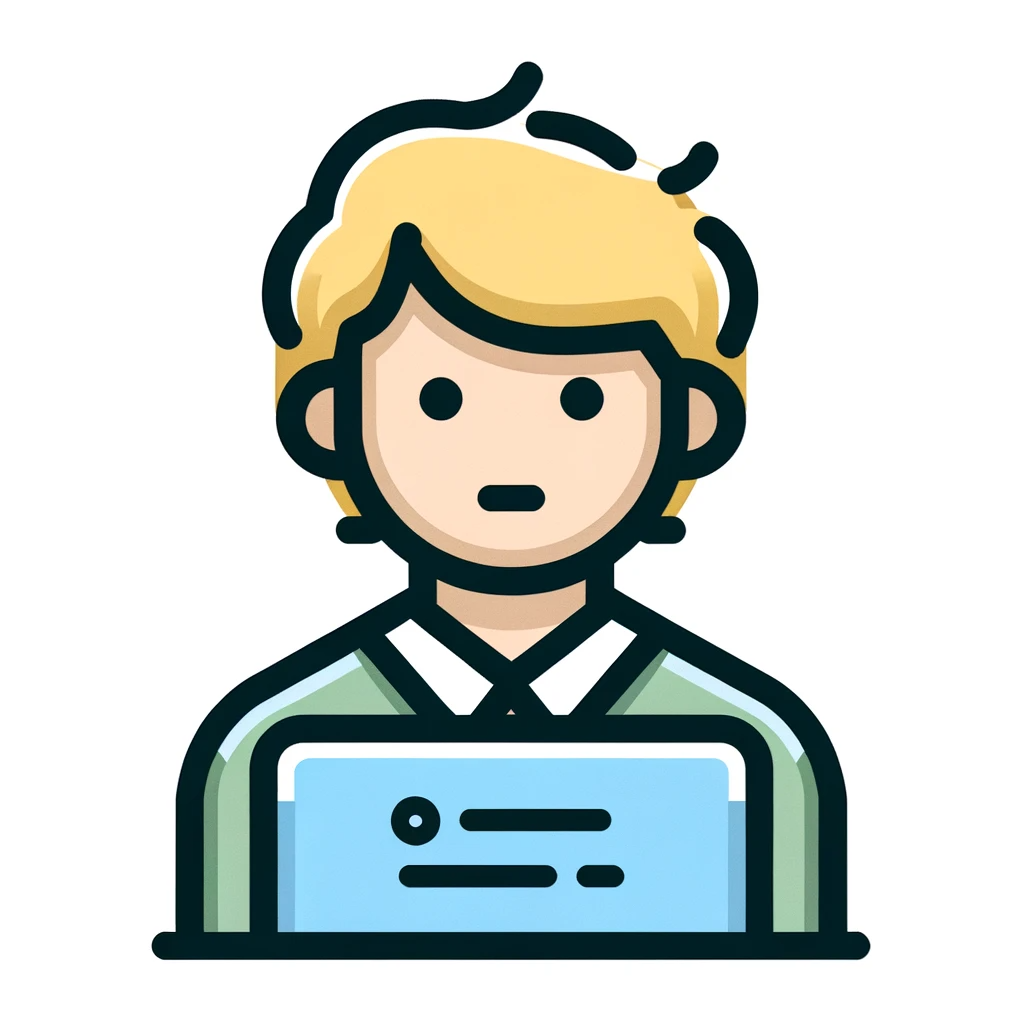
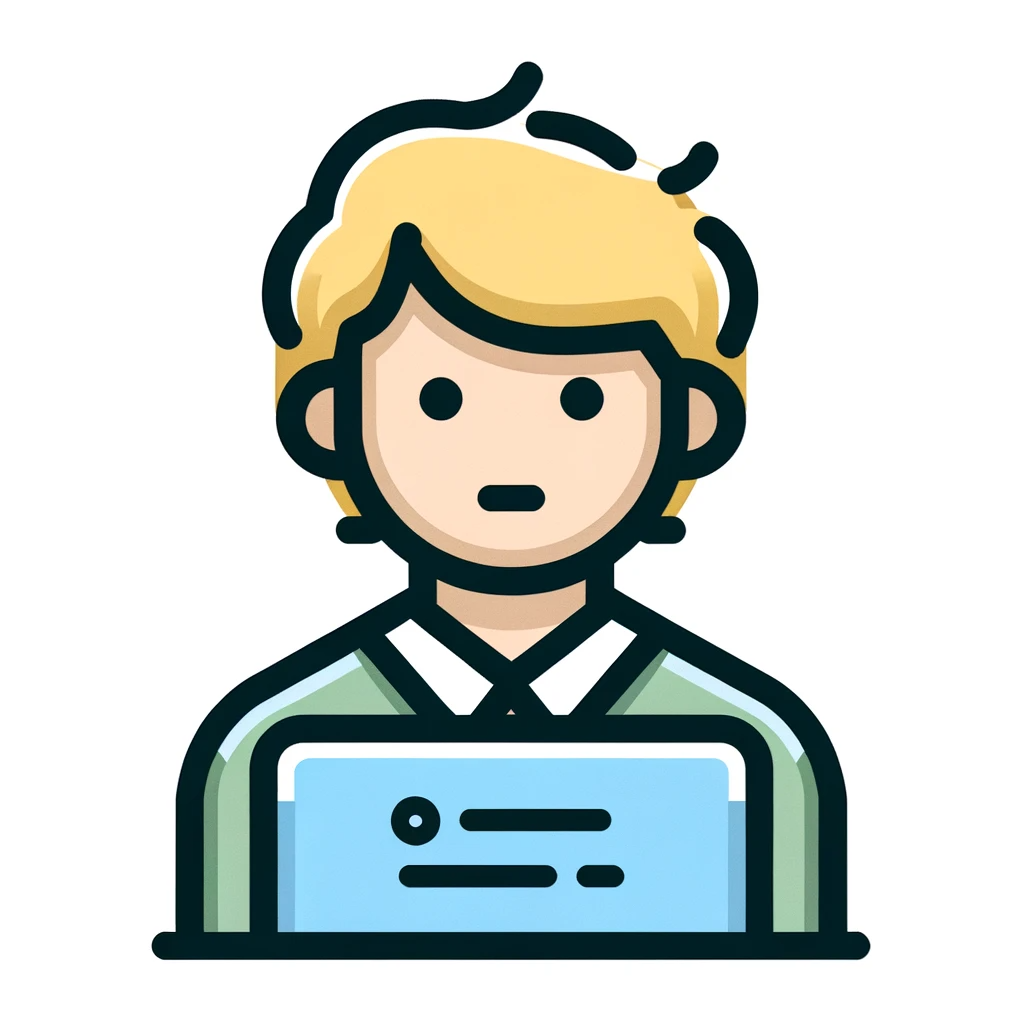
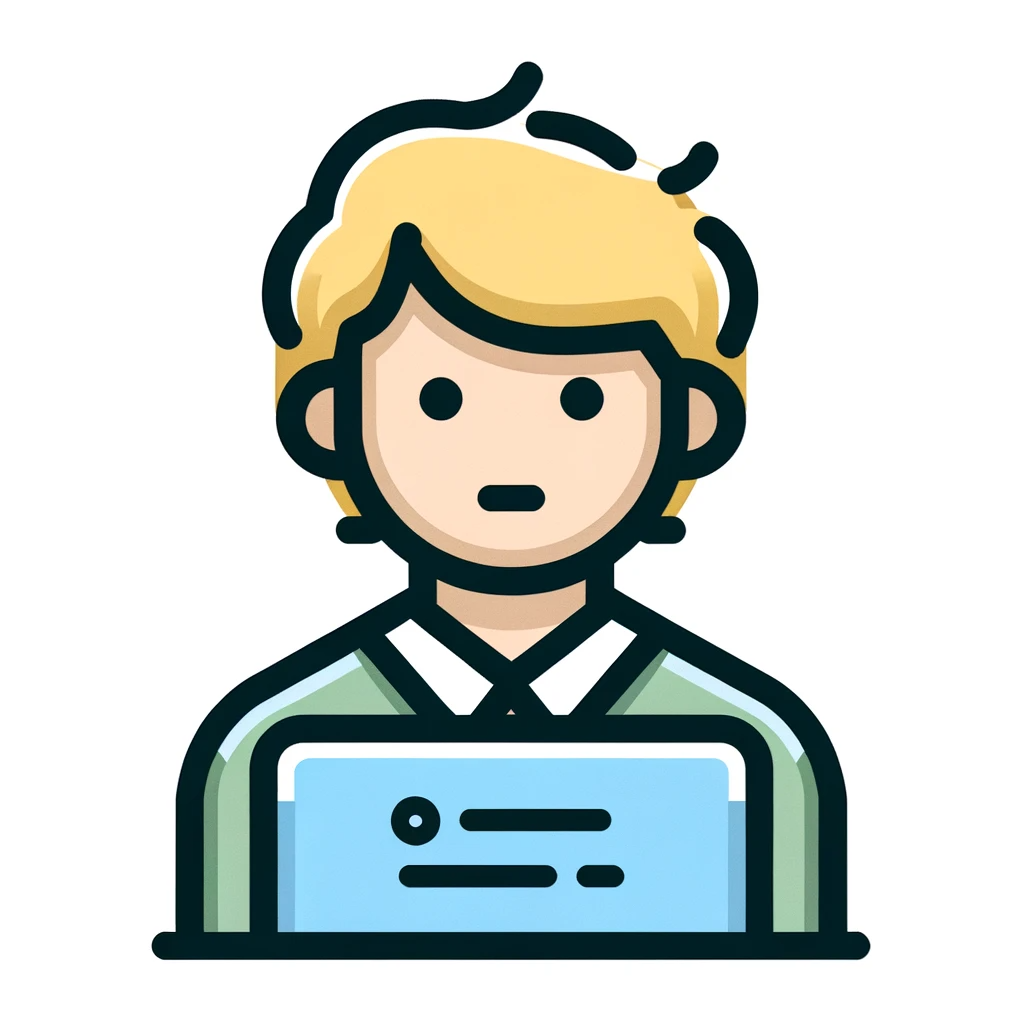
It turns out that there are useful functions for finding the absolute value, minimum value, and maximum value.
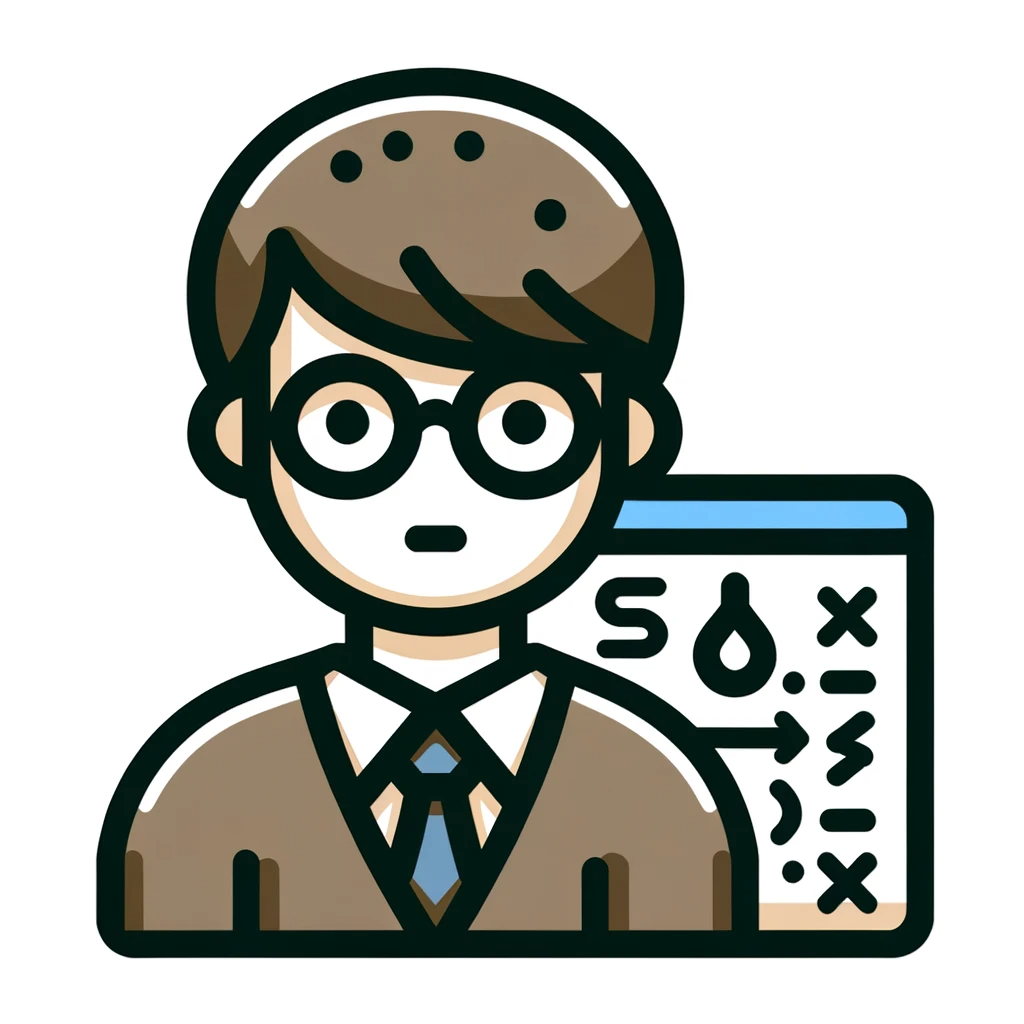
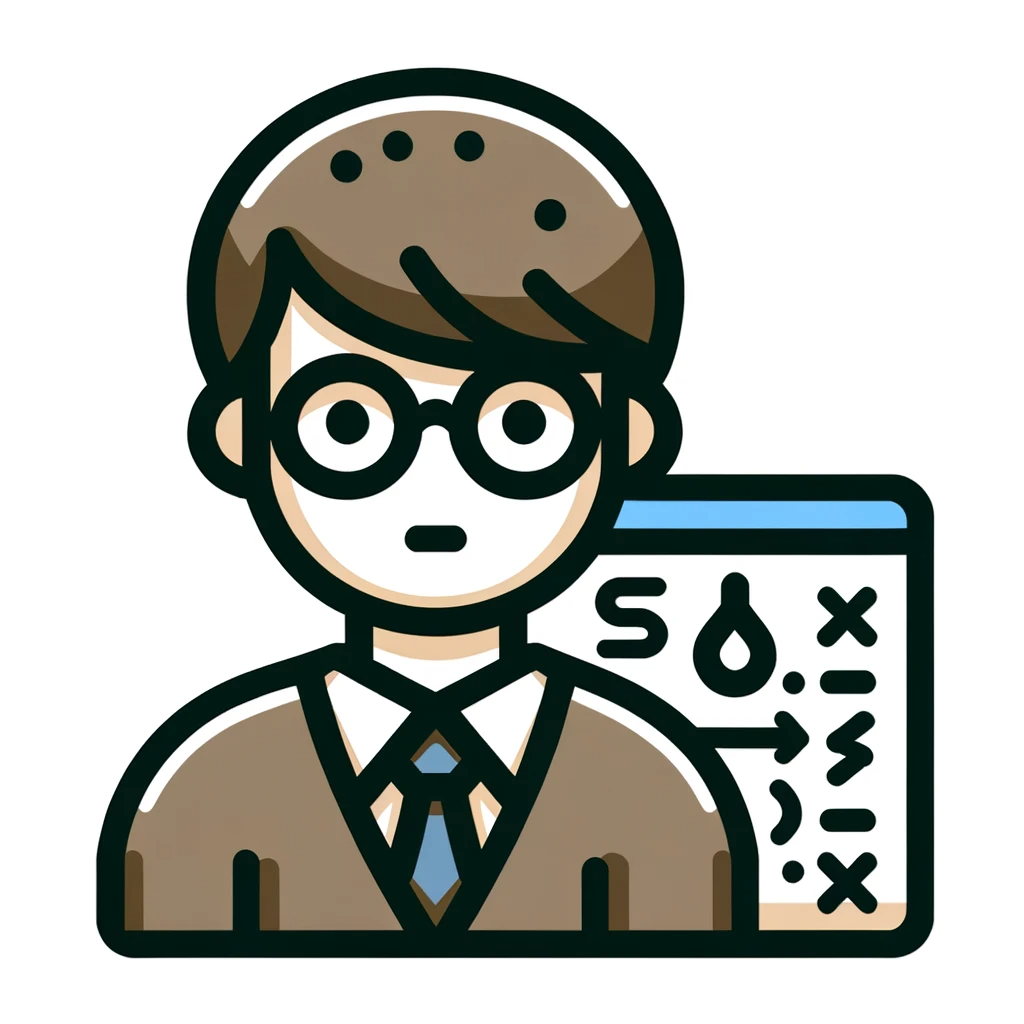
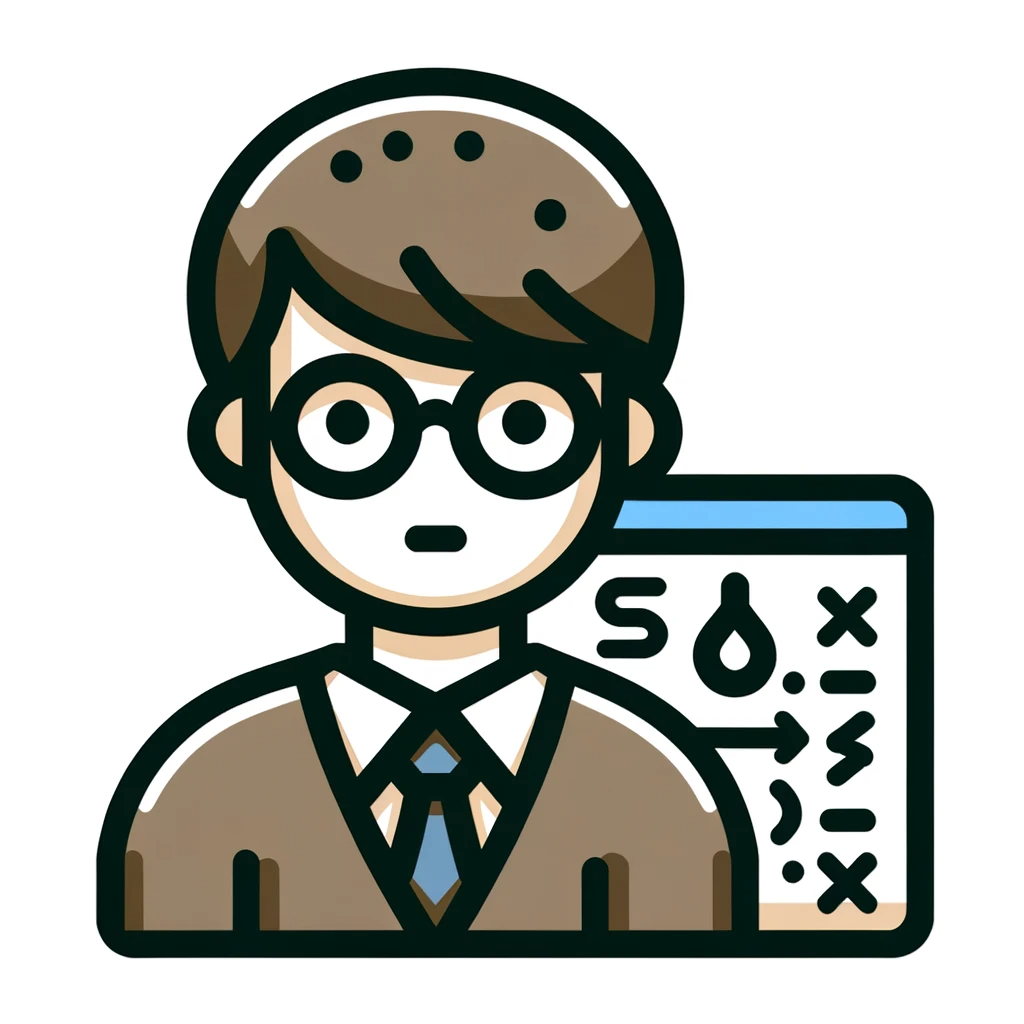
Learning how to use functions will make your programming smoother.
Comments