This section explains in detail how to remove an element attribute using JavaScript’s removeAttribute .
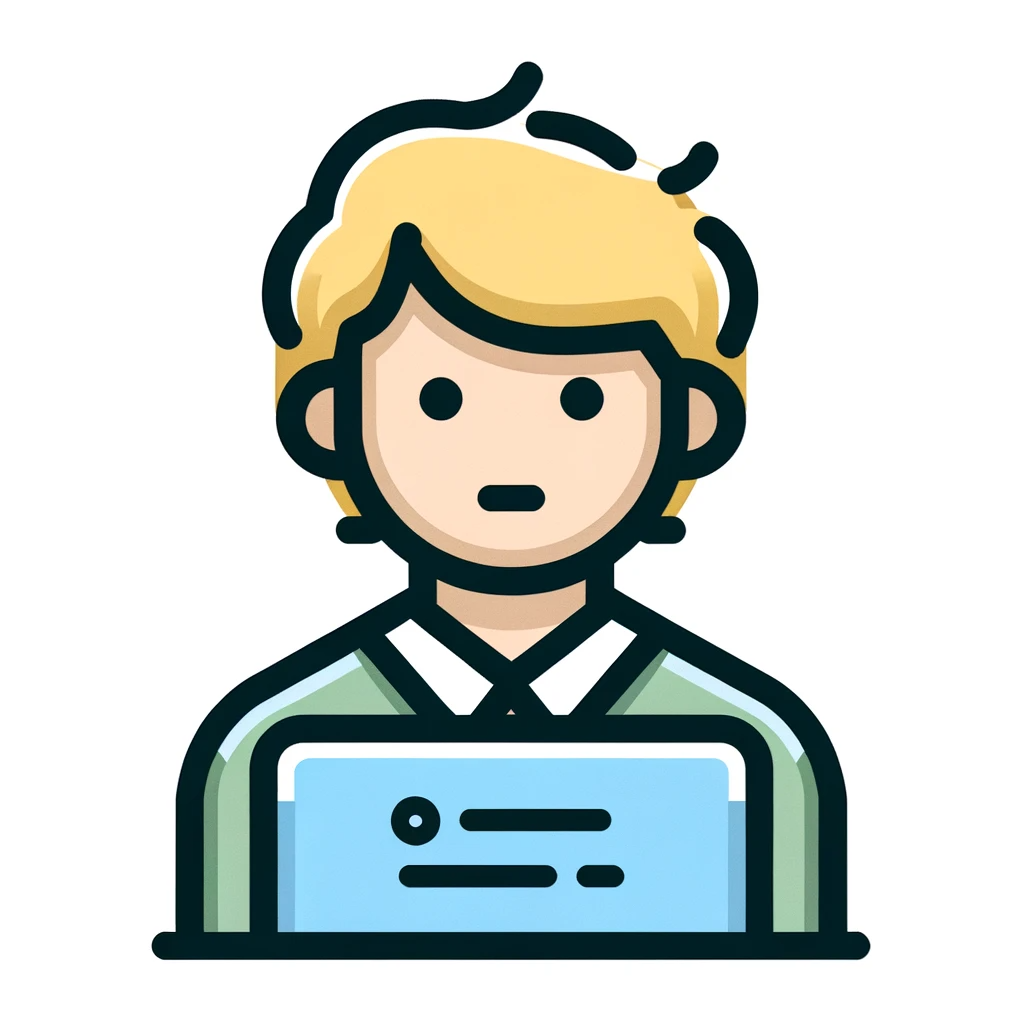
How do I remove attributes of an element in JavaScript?
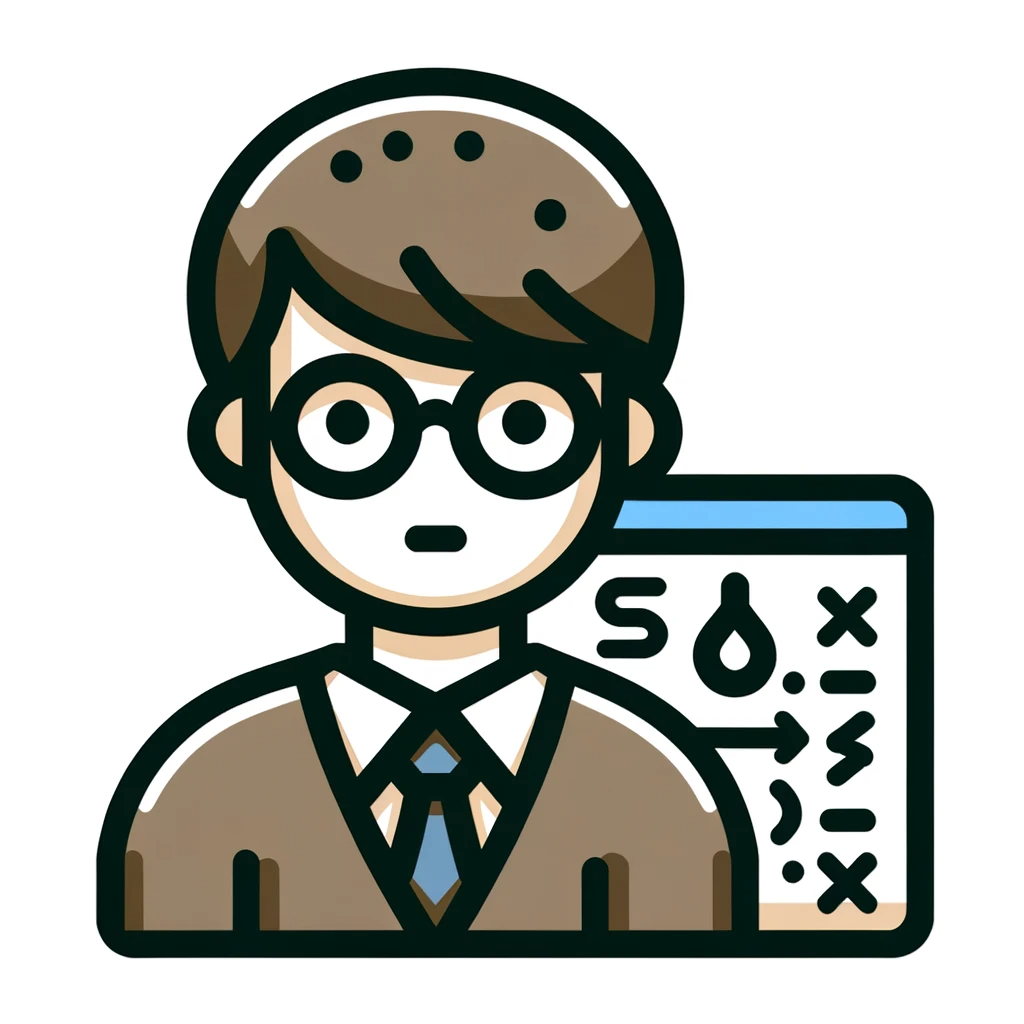
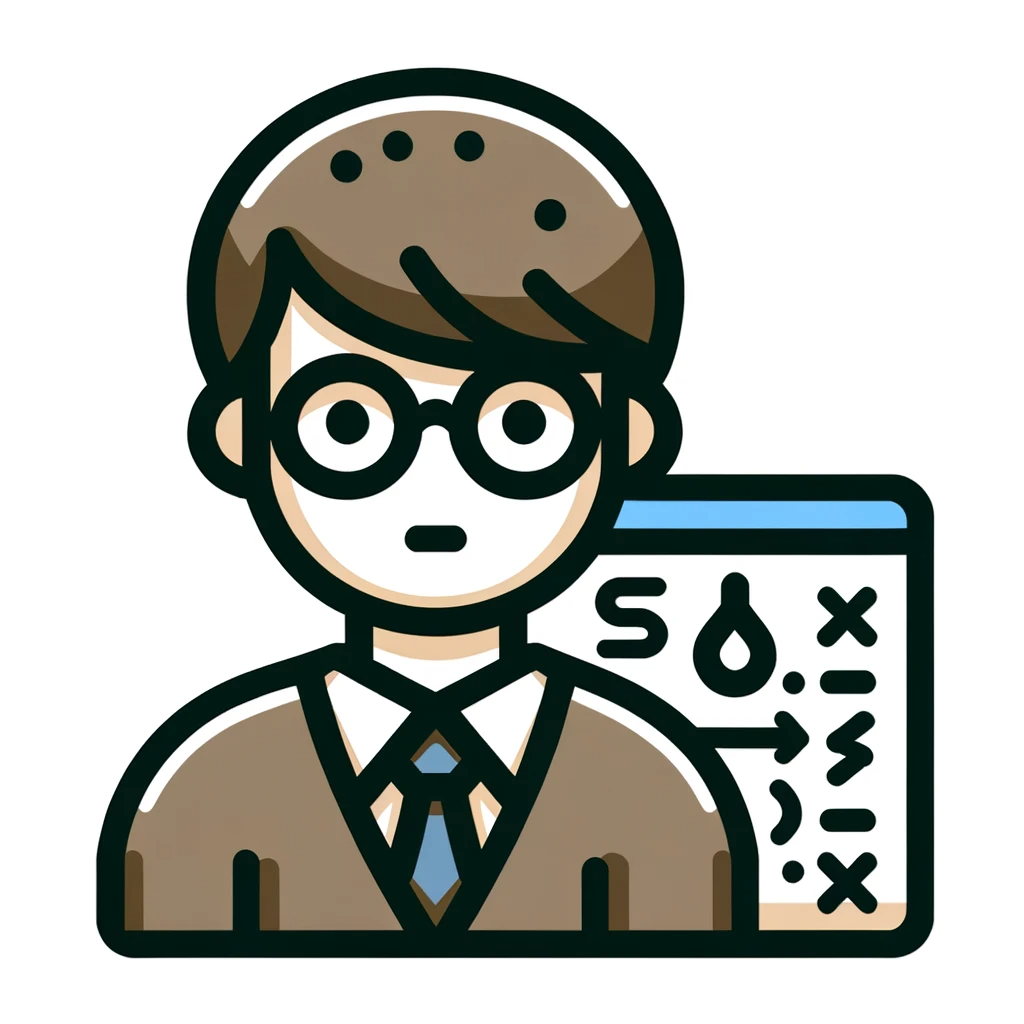
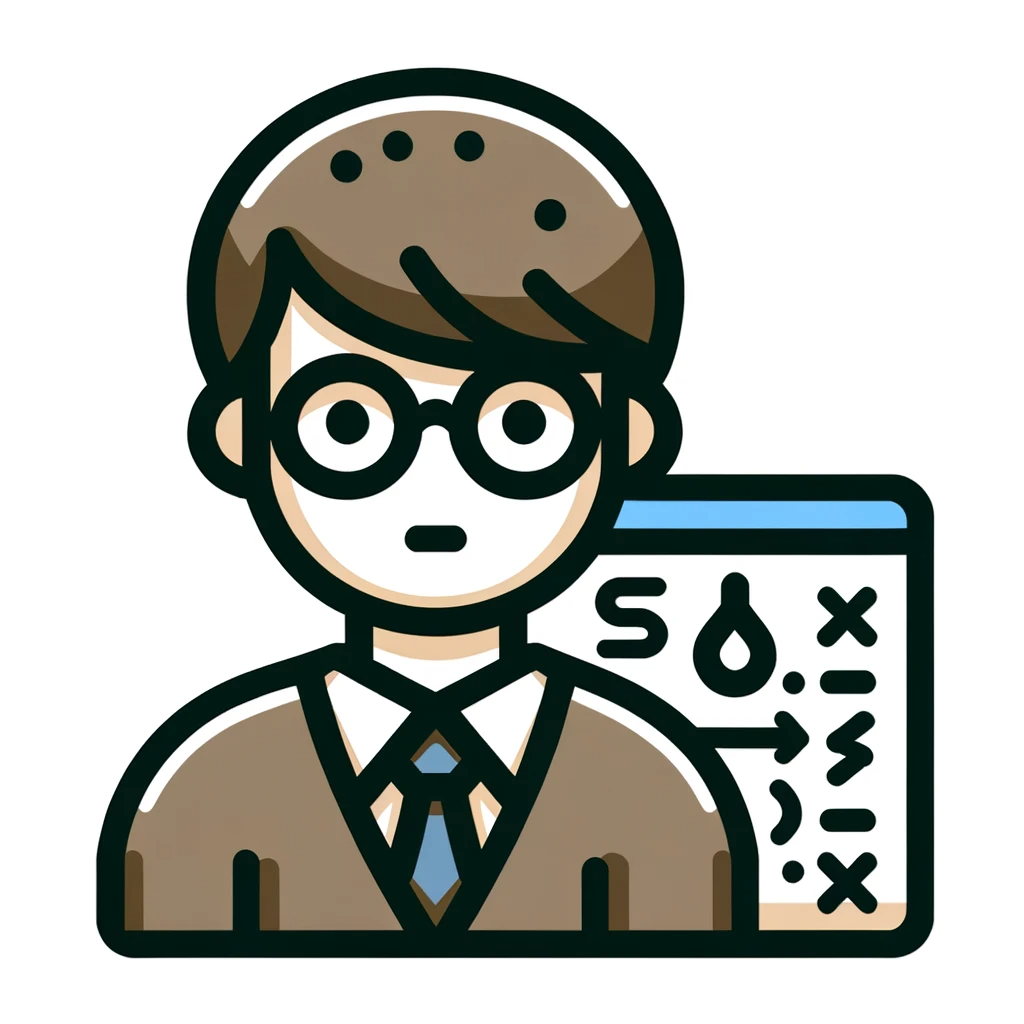
You can remove attributes of an element using
removeAttribute .
What is removeAttribute?
removeAttribute allows you to remove a specific attribute from an HTML element.
This method allows you to change the layout and behavior of a web page by removing the attributes defined for the element.
removeAttribute must be executed after retrieving the HTML element from JavaScript. You must also specify the attribute name to be deleted. By using this method, you can remove unnecessary attributes from an element or dynamically change attribute values.
// Get HTML Elements
let element = document.getElementById("myDiv");
// Delete attribute
element.removeAttribute("class");
How to use removeAttribute to remove an attribute of an element
To remove an attribute from an HTML element, you can use the removeAttribute method.
You can use this method to remove attributes defined on an element.
Rough usage instructions
- Get HTML elements from JavaScript.
- Use removeAttribute method.
- Specify the attribute name to be deleted.
How to use removeAttribute
“removeAttribute” is a method that allows you to remove attributes from HTML elements using JavaScript. How to use it is as follows.
How to use removeAttribute
- Get HTML elements from JavaScript. For this, you can use methods such as “document.getElementById” and “document.querySelector”.
- Use the “removeAttribute” method on the retrieved element. This method can remove attributes defined on an element.
- Specify the attribute name to be deleted. The “removeAttribute” method requires the name of the attribute to be removed as an argument.
Below is an explanation using a sample program of removeAttribute using HTML and JavaScript.
<div id="myDiv" class="box">This is a box</div>
let element = document.getElementById("myDiv");
element.removeAttribute("class");
This sample program removes the “class” attribute from the HTML “div” element.
Get the “div” element from JavaScript using the “document.getElementById” method. Specify the argument “myDiv” to retrieve the “div” element with the ID “myDiv”.
Use the “removeAttribute” method on the obtained “div” element. You can remove the “class” attribute by specifying the argument “class”.
In this way, by combining HTML and JavaScript, you can remove an attribute from an element using the “removeAttribute” method.
Summary of this article
We explained how to remove an attribute of an element using removeAttribute .
- The removeAttribute method is a method for removing attributes from HTML elements.
- You can retrieve the HTML element using the document.getElementById method and remove the attribute using the removeAttribute method.
- By combining HTML and JavaScript, you can remove attributes from elements.
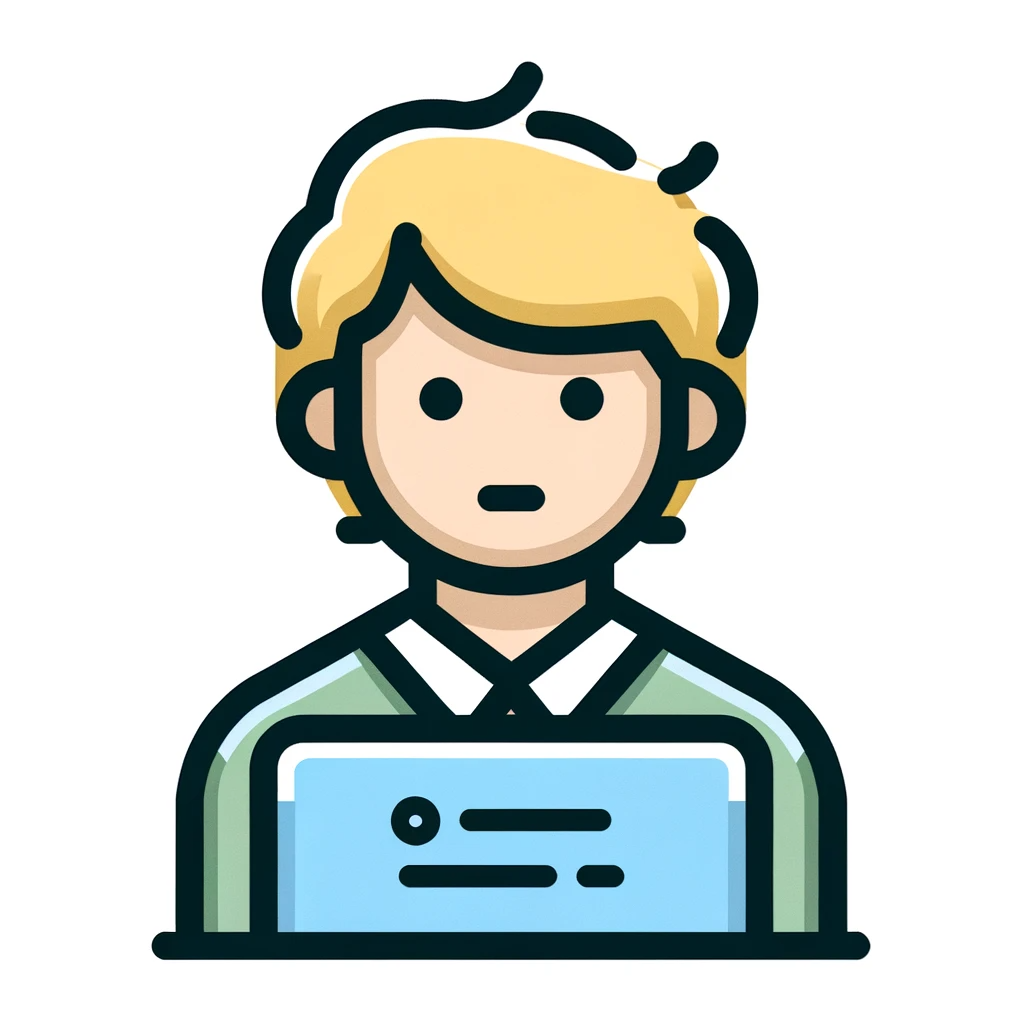
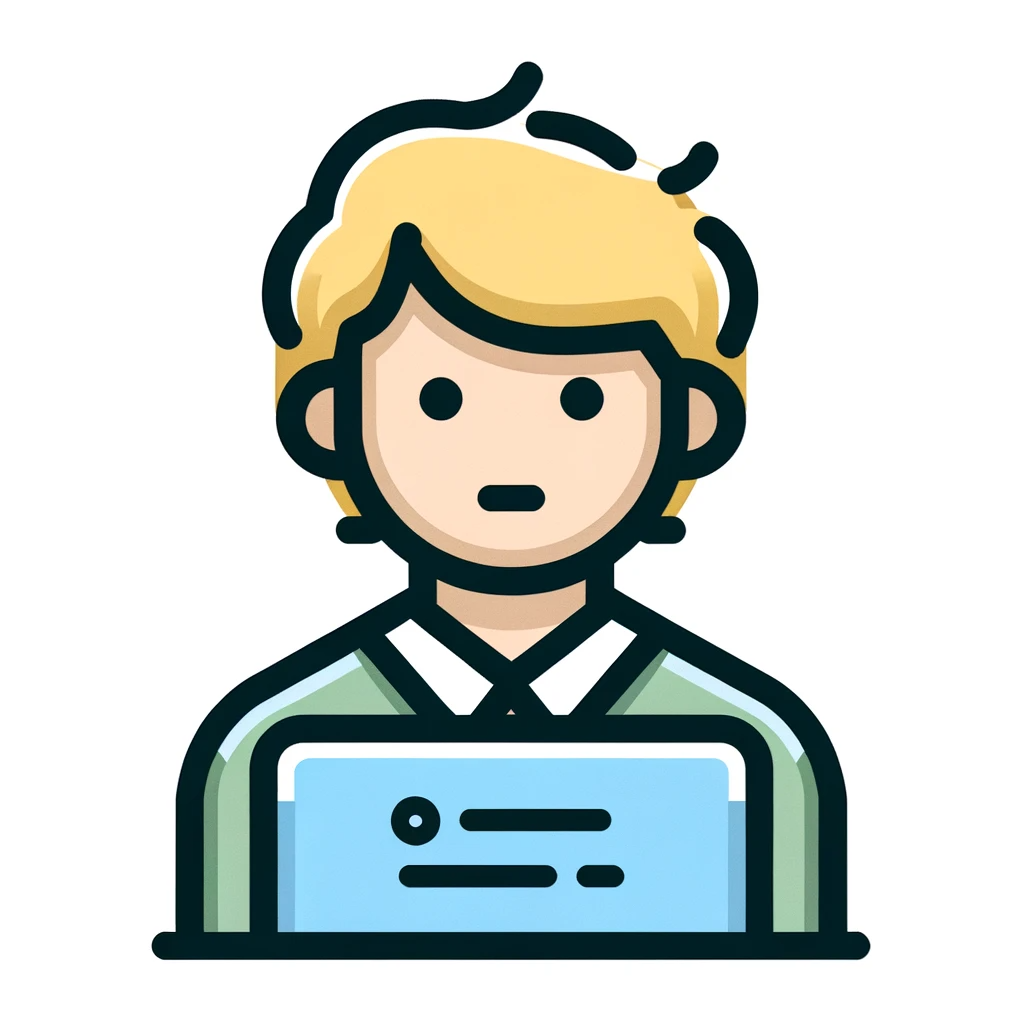
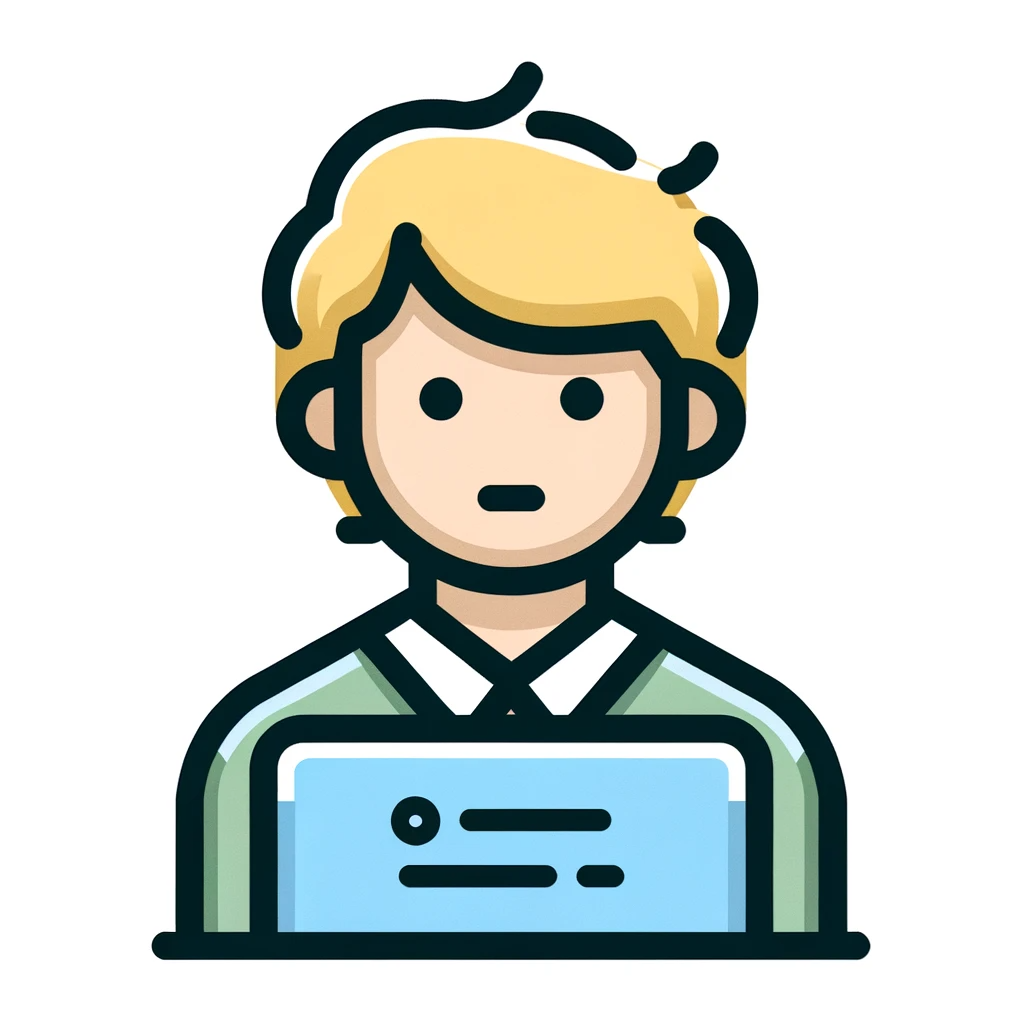
It turns out that the removeAttribute method is very easy to use and allows you to remove attributes from HTML elements!
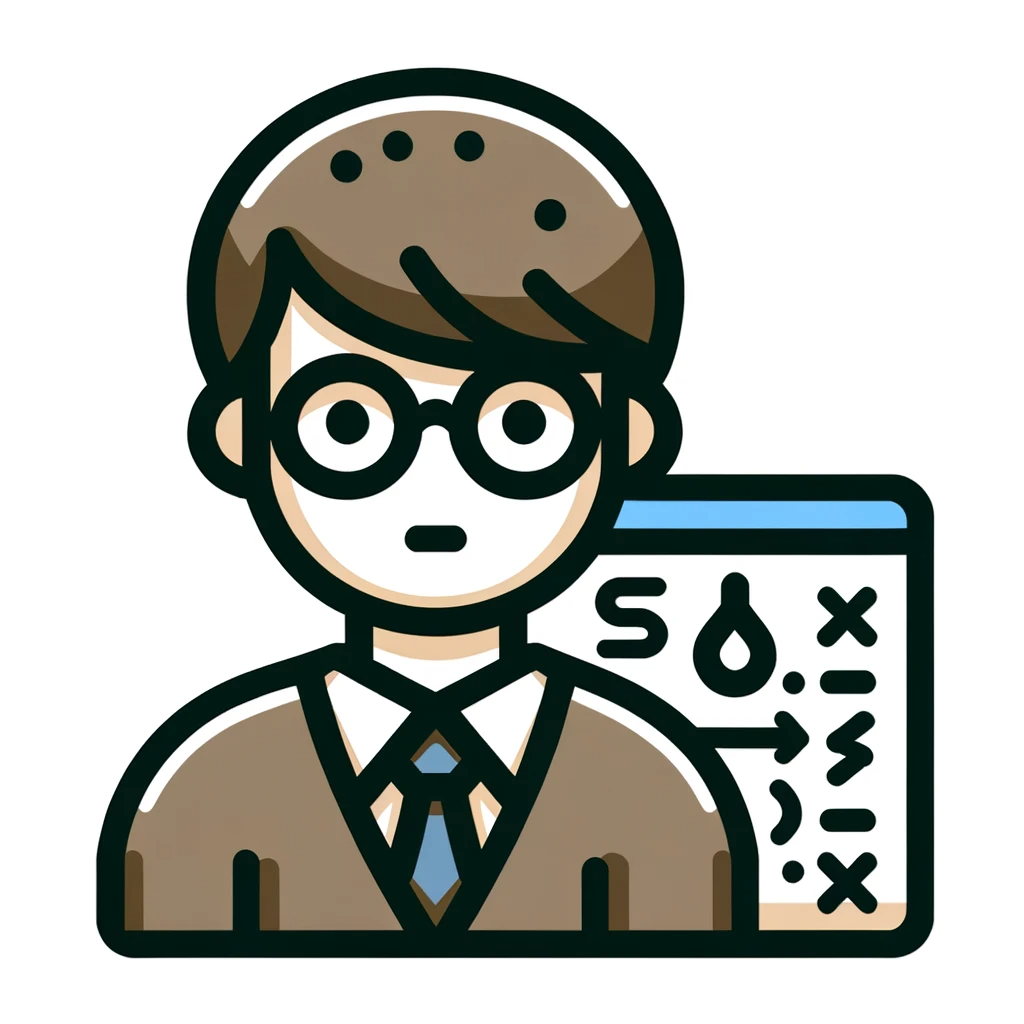
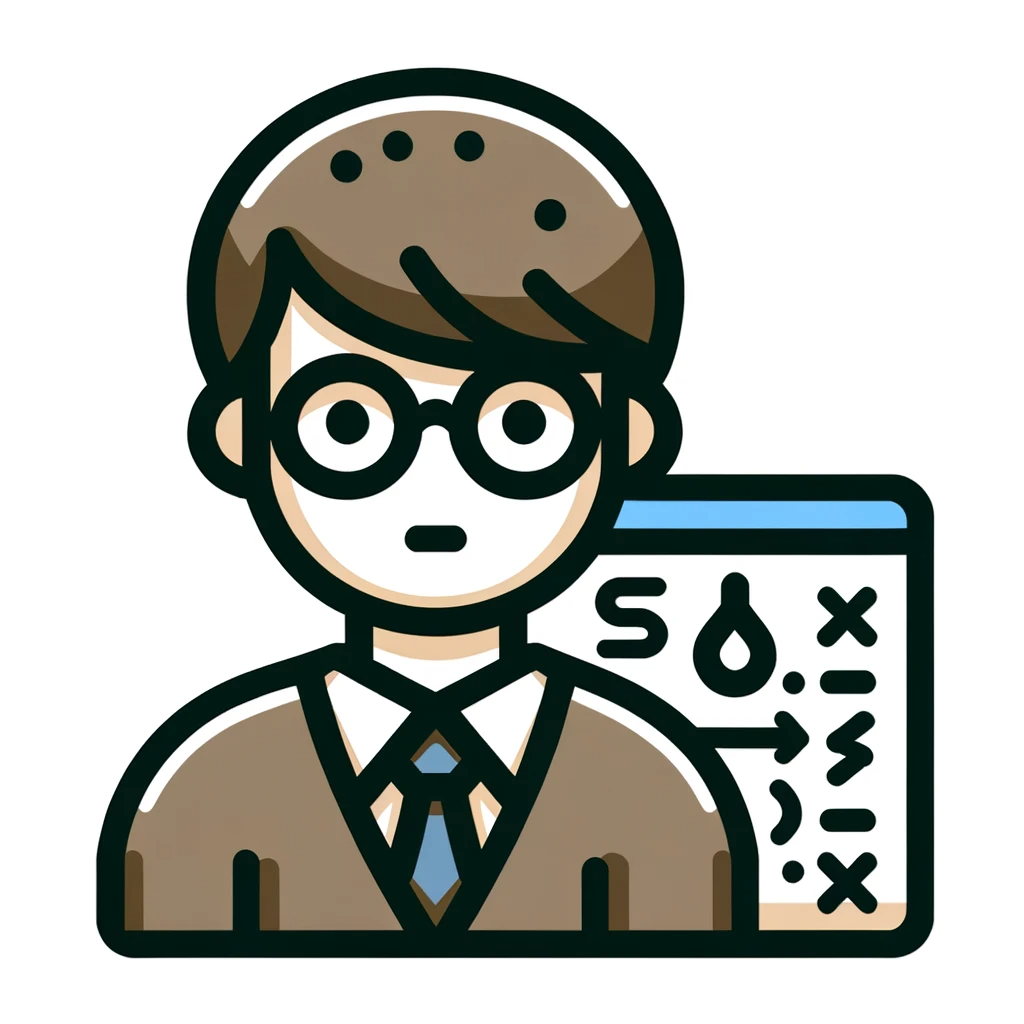
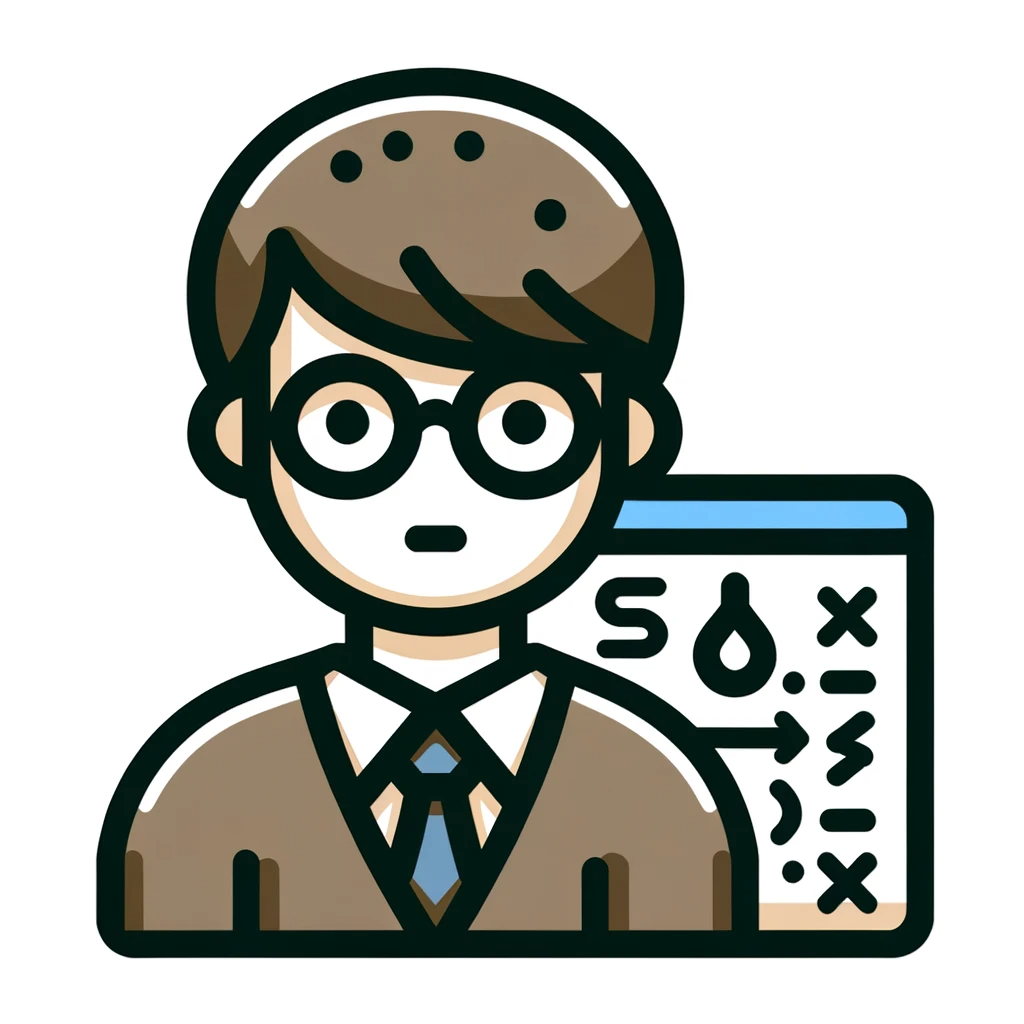
You can easily remove attributes from HTML elements by using JavaScript’s removeAttribute.
If you can master this function, you can create smarter web pages.
Comments