We will explain how to scroll a page using JavaScript by dividing it into scrolling to a specified position, scrolling by a specified amount, and scrolling to a specific element.
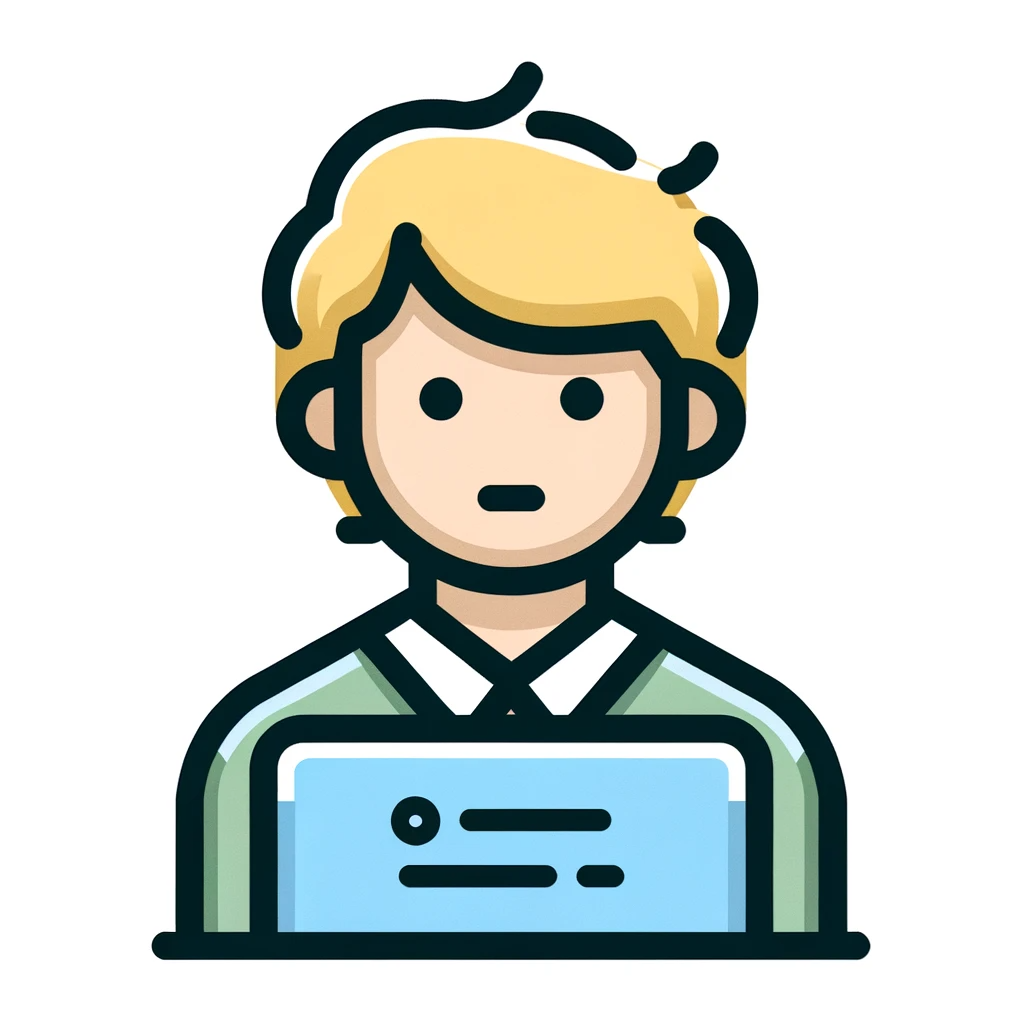
I would like to implement page scrolling using JavaScript. Could you please tell me the specific method?
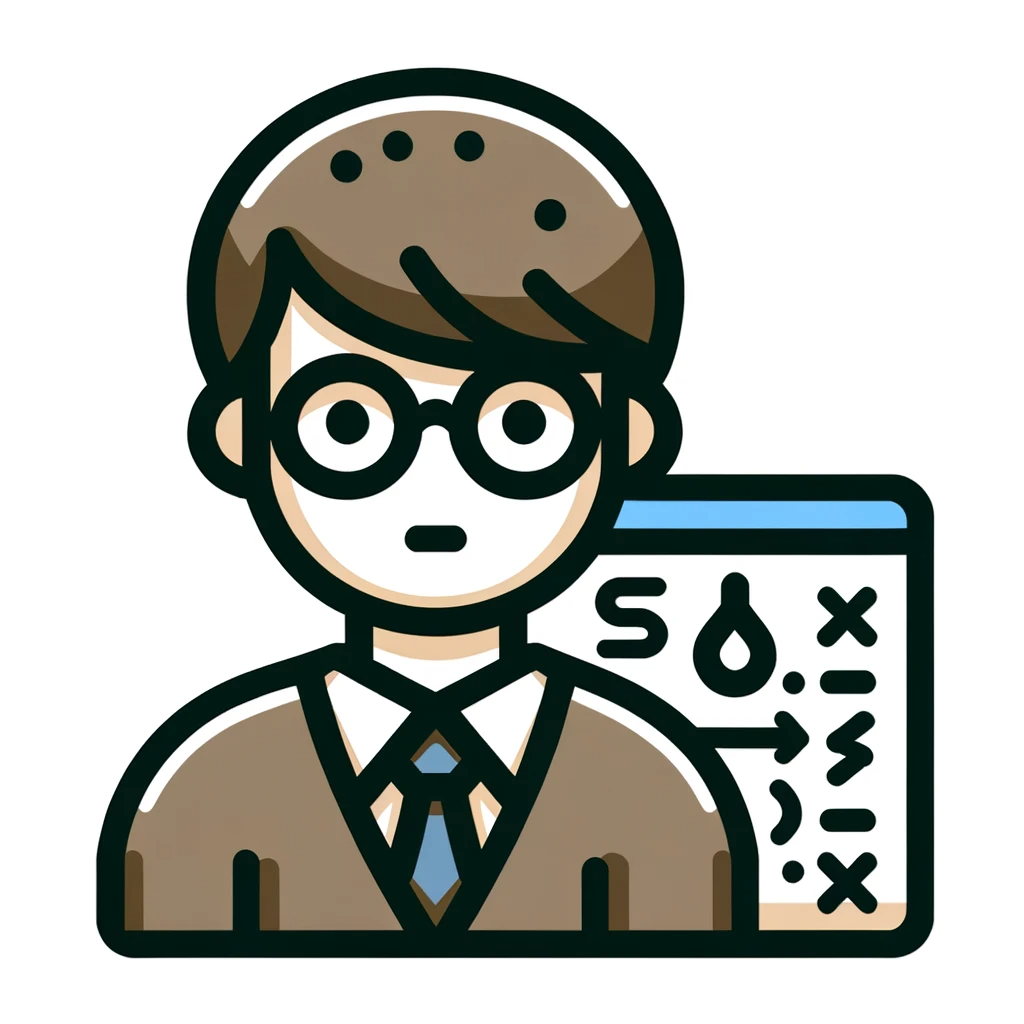
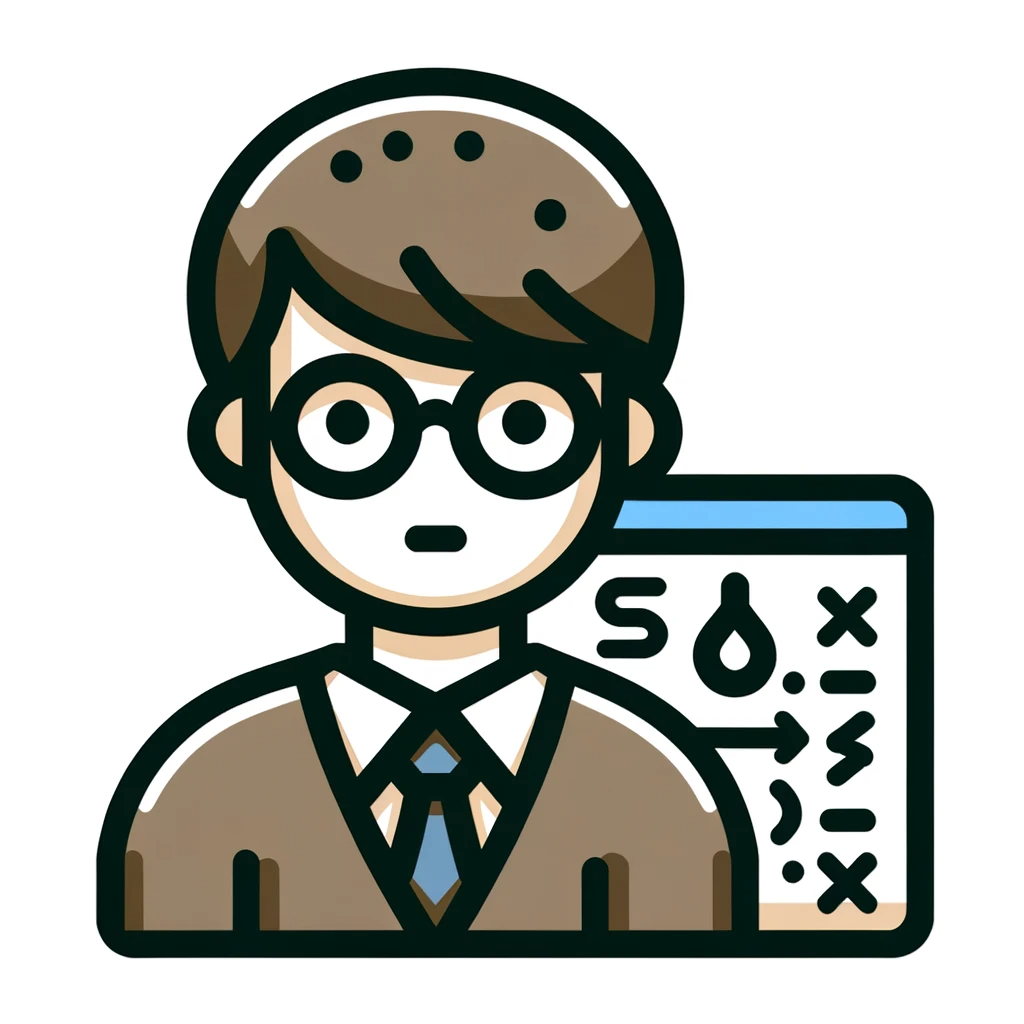
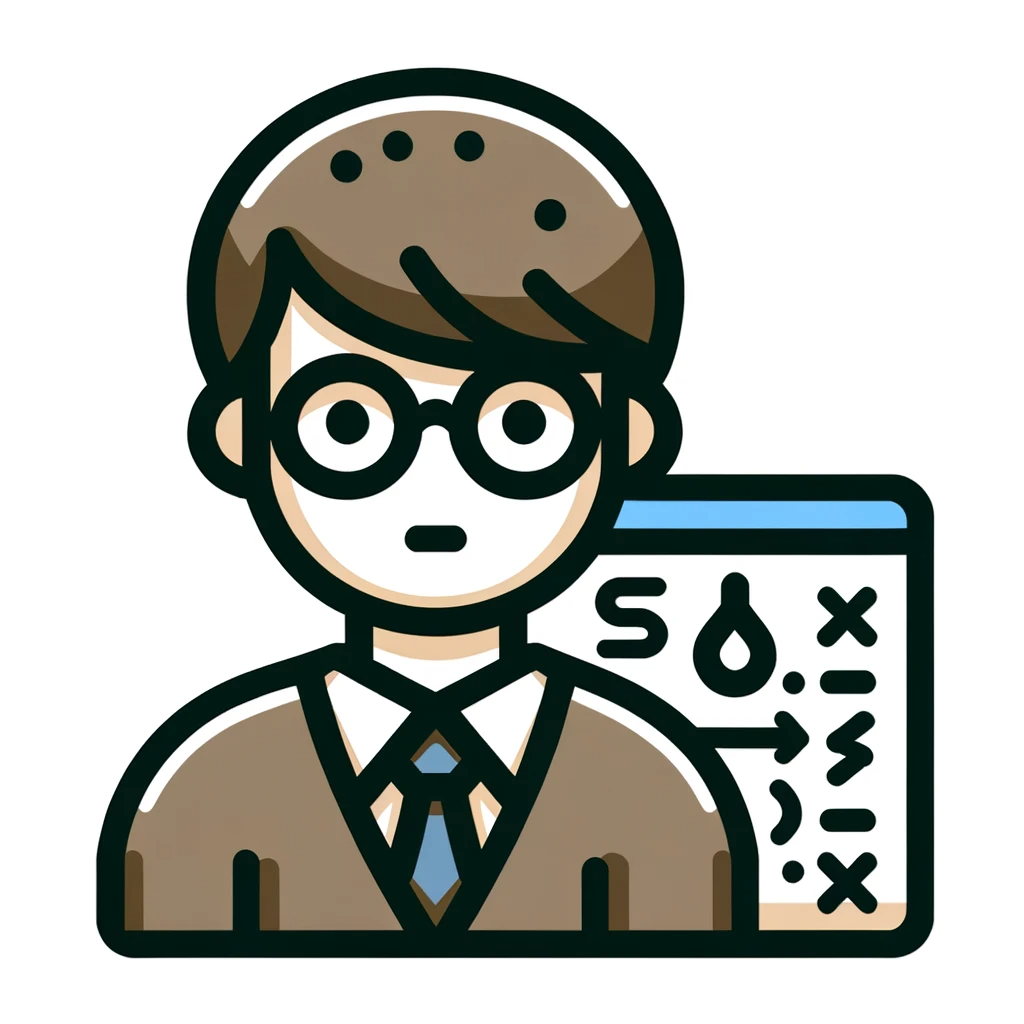
There are several ways to achieve page scrolling, but this time I will mainly explain the following three methods.
・Scroll to a specified position
・Scroll by a specified amount
・Scroll to a specific element
How to scroll to a specified position
Use the scrollTo method to scroll the page to a specified position . The scrollTo method scrolls to the position specified by the x and y coordinates passed as arguments.
Specifically, write it as follows.
// Scroll to x-coordinate 0, y-coordinate 500px
window.scrollTo(0, 500);
In the above example, the x coordinate is specified as 0 and the y coordinate is specified as 500. In this case, it will be scrolled to a position 500px from the top of the page.
You can also specify an object by passing it as an argument. Write it as follows.
// Scroll to x-coordinate 0, y-coordinate 500px
window.scrollTo({
top: 500,
left: 0,
behavior: 'smooth'
});
In the above example, 500 is specified for the top property and 0 is specified for the left property. Furthermore, by specifying ‘smooth’ in the behavior property, scrolling can be performed smoothly.
Explanation using a sample program
In the sample program below, clicking the button will scroll to the bottom of the page. I am using the scrollTo method to scroll to the bottom of the page when a click event occurs.
<!DOCTYPE html>
<html>
<head>
<title>How to scroll to the specified position</title>
</head>
<body>
<button onclick="scrollToBottom()">Scroll to the bottom of the page.</button>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<p>...</p>
<script>
function scrollToBottom() {
window.scrollTo({
top: document.body.scrollHeight,
left: 0,
behavior: 'smooth'
});
}
</script>
</body>
</html>
How to scroll by a specified amount
If you want to scroll by a specified amount, use the scrollBy method . The scrollBy method allows you to scroll a specified amount from the current scroll position.
The following is an example code that specifies the scroll amount as 200px and scrolls 200px from the current scroll position.
window.scrollBy(0, 200);
In this way, the first argument of the scrollBy method specifies the horizontal scroll amount, and the second argument specifies the vertical scroll amount. In the above example, 0 is specified for the first argument, so no horizontal scrolling will occur.
Also, when scrolling by the specified amount, it is scrolled relative to the current scroll position, so you can get the same result no matter where you call it.
Explanation using a sample program
Below is a sample code that specifies the scroll amount and scrolls when the button is clicked.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>ScrollBy Sample</title>
</head>
<body>
<button id="scrollBtn">Scroll Down</button>
<script>
const scrollBtn = document.getElementById('scrollBtn');
scrollBtn.addEventListener('click', () => {
window.scrollBy(0, 200);
});
</script>
</body>
</html>
In the above code, when the button is clicked, we call the window object’s scrollBy method to scroll 200px vertically.
How to scroll a page to a specific element
If you want to scroll to a specific element, use the scrollIntoView method . The scrollIntoView method allows you to scroll the specified element into view.
Below is an example of code that scrolls to the element with id “target”.
const targetElement = document.getElementById("target");
targetElement.scrollIntoView();
In this way, by calling the scrollIntoView method, the specified element will be scrolled into view. If the argument is omitted, the element specified in the argument will be scrolled so that it is displayed in the center of the window.
const targetElement = document.getElementById("target");
targetElement.scrollIntoView({ behavior: "smooth" });
The scrollIntoView method can specify two behavior modes: “auto” or “smooth” defined by the scroll-behavior property. By specifying “smooth”, scrolling becomes smooth.
Explanation using a sample program
Below is a sample code that scrolls to a specific element when a link is clicked.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>ScrollIntoView Sample</title>
<style>
#target {
height: 1000px;
background-color: red;
}
</style>
</head>
<body>
<a href="#" id="scrollLink">Scroll to Target</a>
<div id="target"></div>
<script>
const scrollLink = document.getElementById('scrollLink');
const targetElement = document.getElementById('target');
scrollLink.addEventListener('click', (event) => {
event.preventDefault();
targetElement.scrollIntoView({ behavior: 'smooth' });
});
</script>
</body>
</html>
In the above code, when the link is clicked, it scrolls to the element with id “target”. The argument of the scrollIntoView method specifies smooth scrolling.
About CSS scroll-behavior property
”Scroll-behavior” is a CSS property used to specify the scrolling behavior of an element.
The scroll-behavior property has three values.
- auto: Uses default scrolling behavior.
- smooth: Performs smooth scrolling.
- initial: Return to initial value.
The scroll-behavior property can be used alone, but it is also useful when implementing scrolling behavior in JavaScript. For example, to achieve smooth scrolling when using the scrollTo method, you can set the CSS scroll-behavior property as shown below.
html {
scroll-behavior: smooth;
}
With this setting, even if you use JavaScript’s scrollTo method to scroll to a specified position, the scroll will be performed with smooth animation.
However, the scroll-behavior property is not fully supported by all browsers. Therefore, when implementing scrolling behavior, you need to be aware of the differences between browsers. It is also important to set the speed appropriately, as smooth scrolling that is too fast can be annoying for users.
summary
We explained how to scroll a page using JavaScript by dividing it into scrolling to a specified position, scrolling by a specified amount, and scrolling to a specific element.
- You can use JavaScript to control scrolling within a page.
- There are several methods for scrolling: scrolling to a specified position, scrolling by a specified amount, and scrolling to a specific element.
- To scroll to a specified position, use the scrollTo method; to scroll by a specified amount, use the scrollBy method.
- If you want to scroll to a specific element, use the scrollIntoView method on that element.
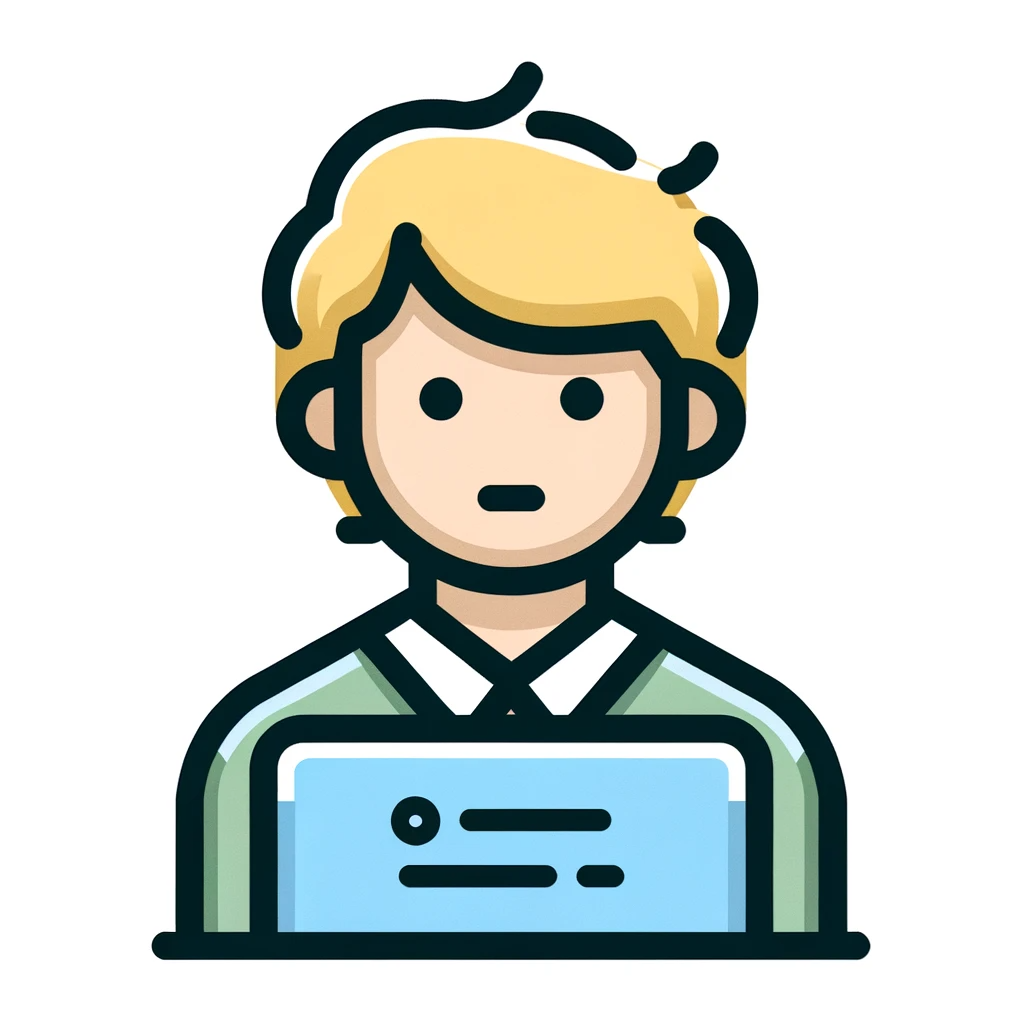
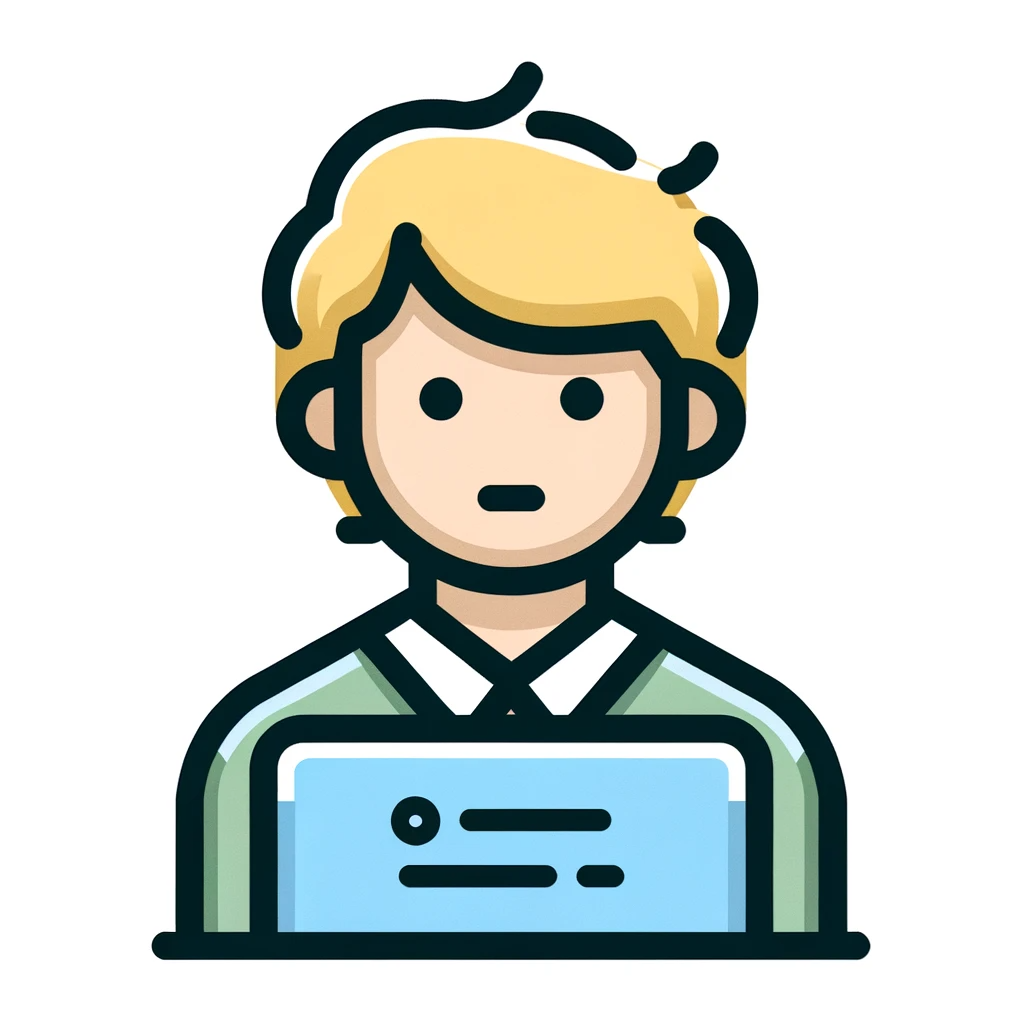
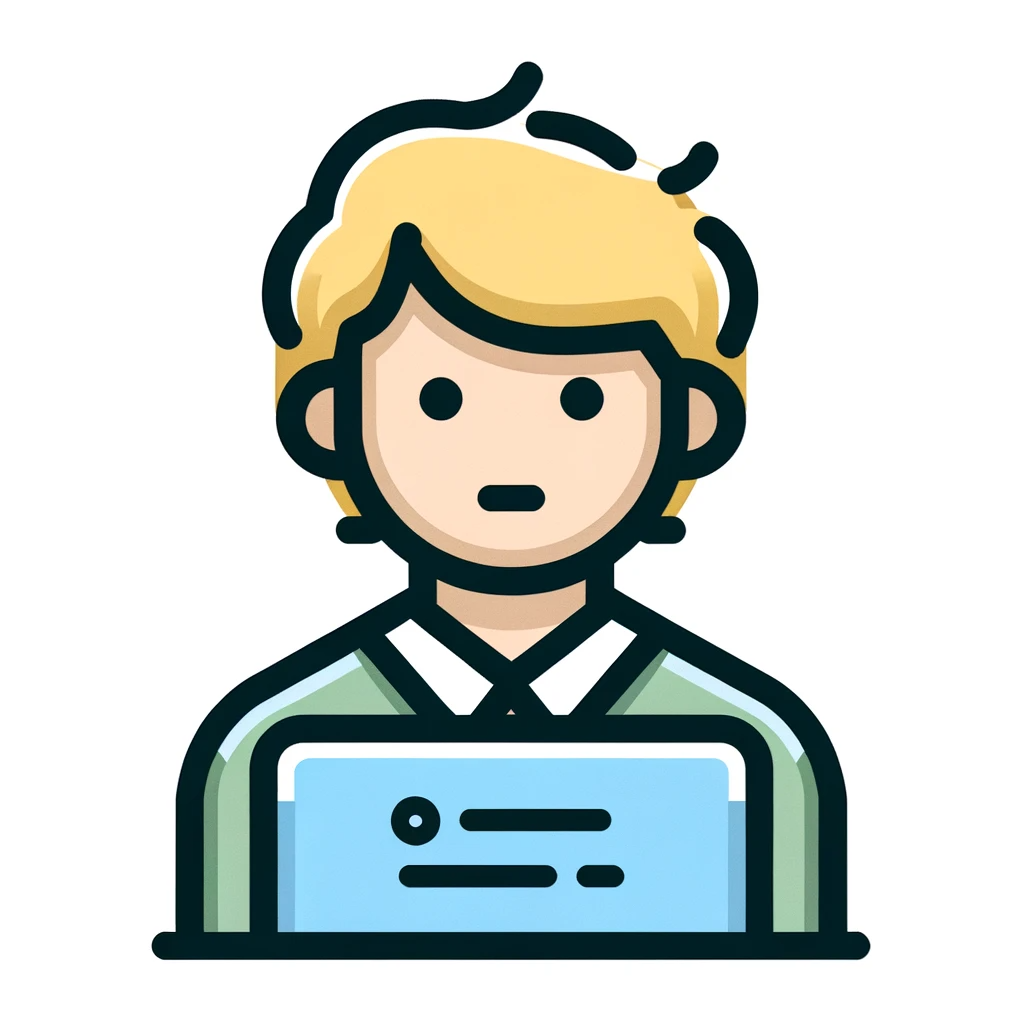
You can use these scroll controls to make the elements on your page easier to use!
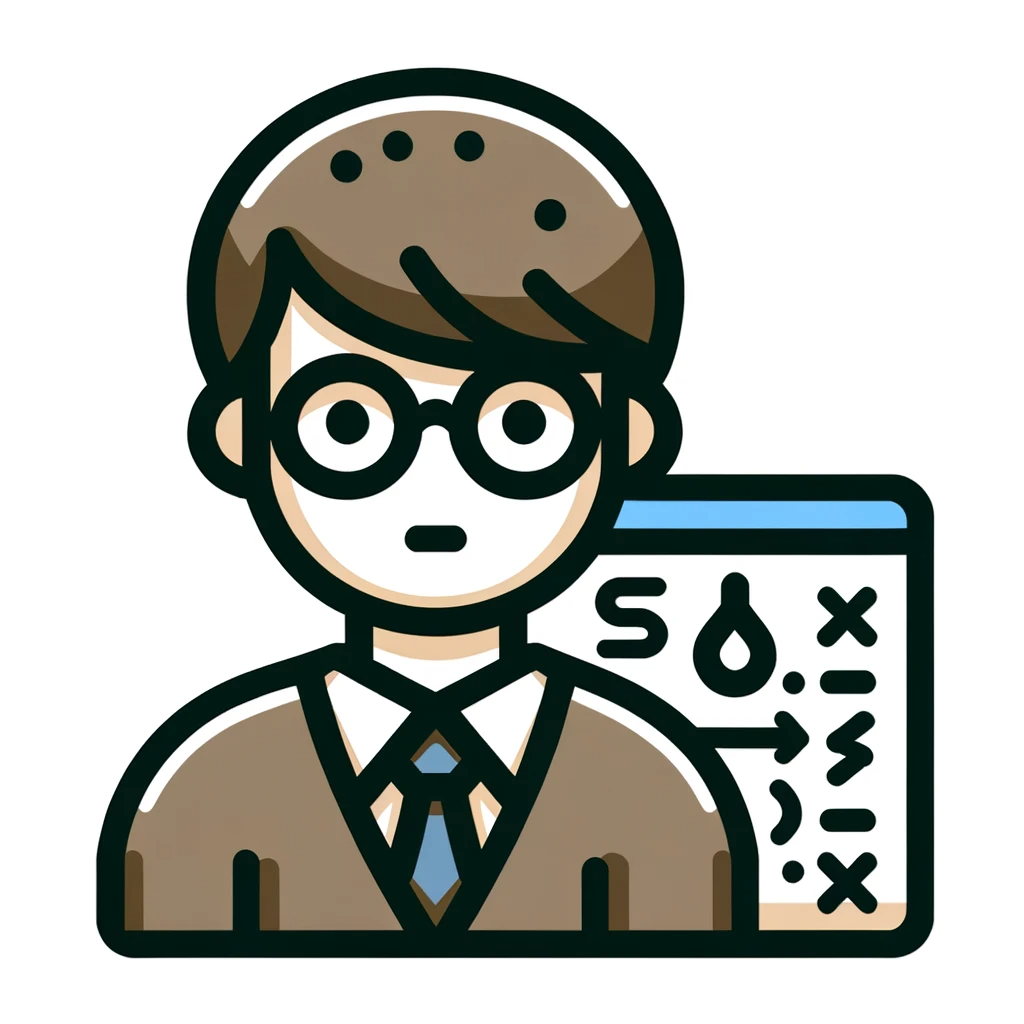
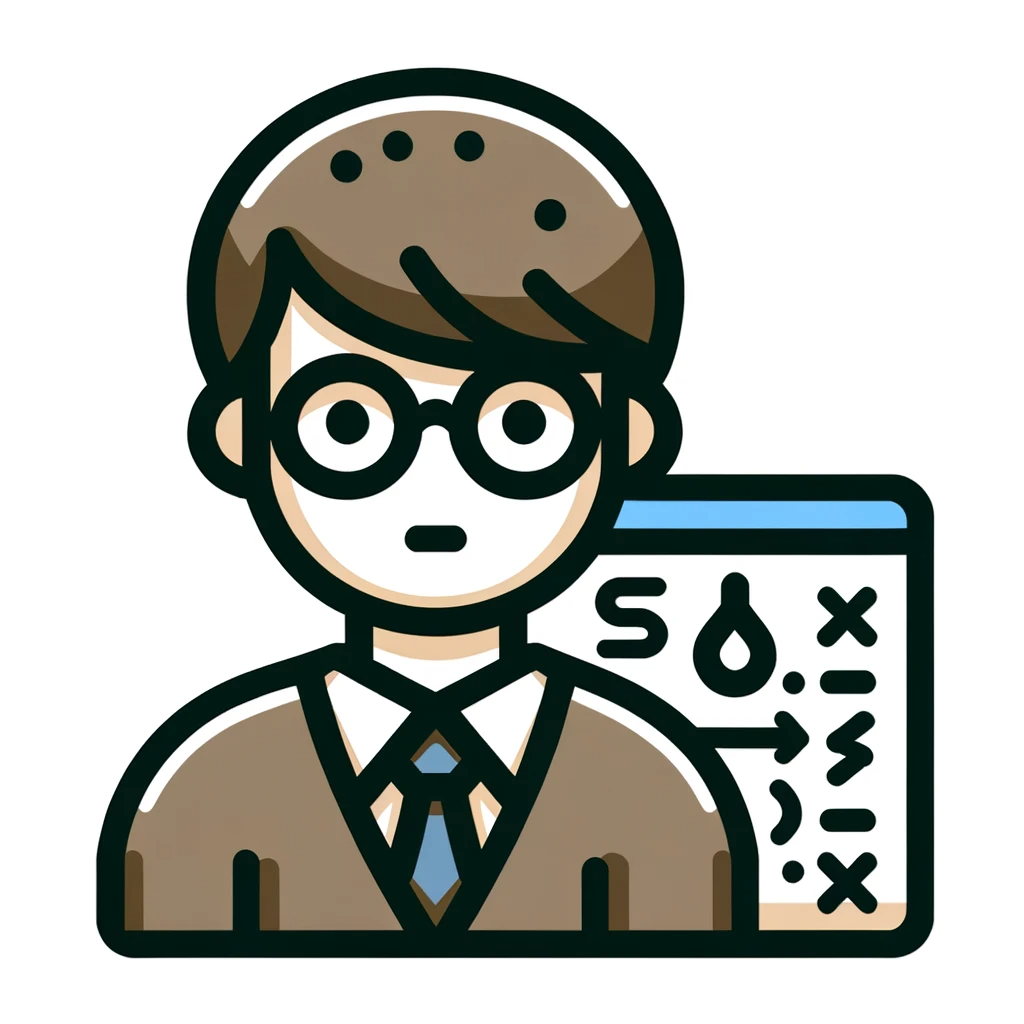
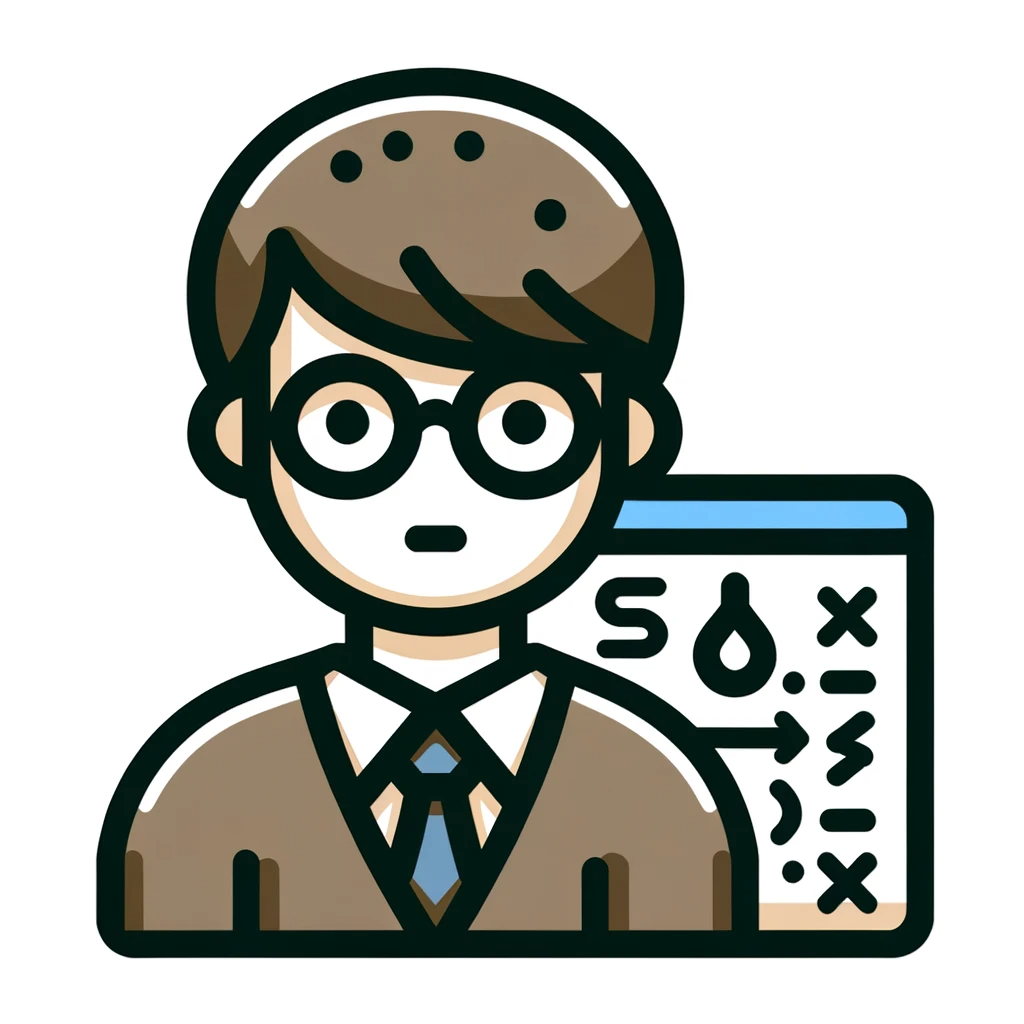
Scroll controls have a significant impact on the user experience, so care must be taken when implementing them.
Especially in order to achieve smooth scrolling, it is important to combine it with the CSS scroll-behavior property.
Additionally, when implementing scrolling behavior, we recommend testing the behavior under various scenarios.
By taking these measures, you can create pages that are comfortable and easy to use for users.
Comments