This section explains how to obtain XML format data using JavaScript.
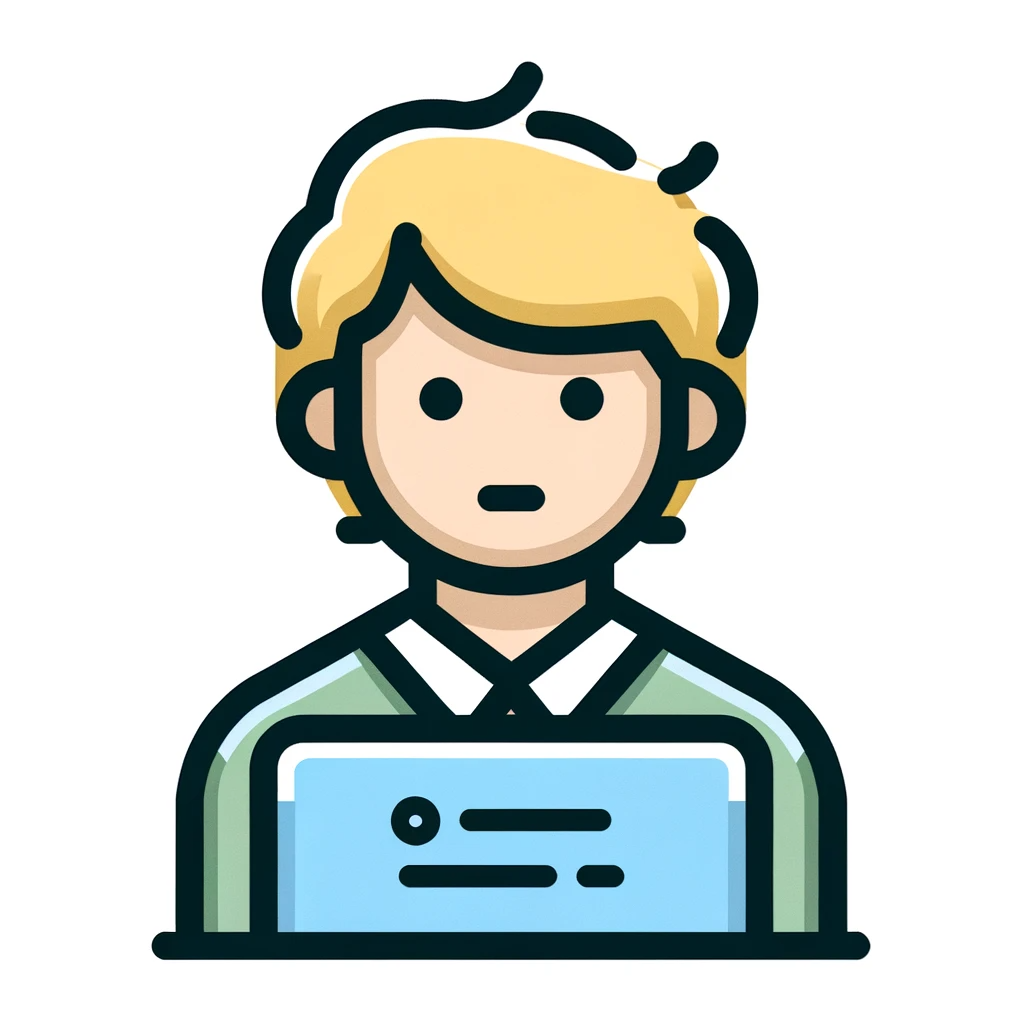
I would like to know how to obtain XML format data using JavaScript. How can I do this?
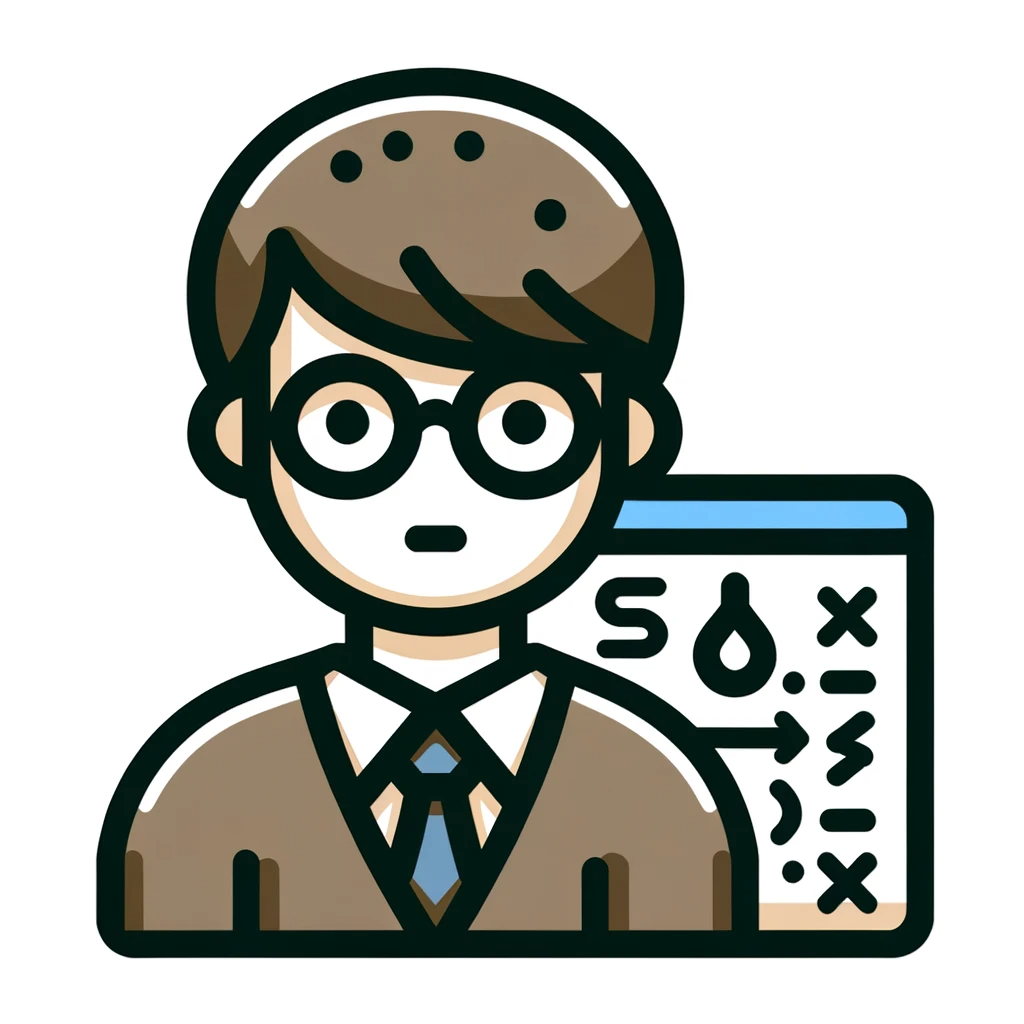
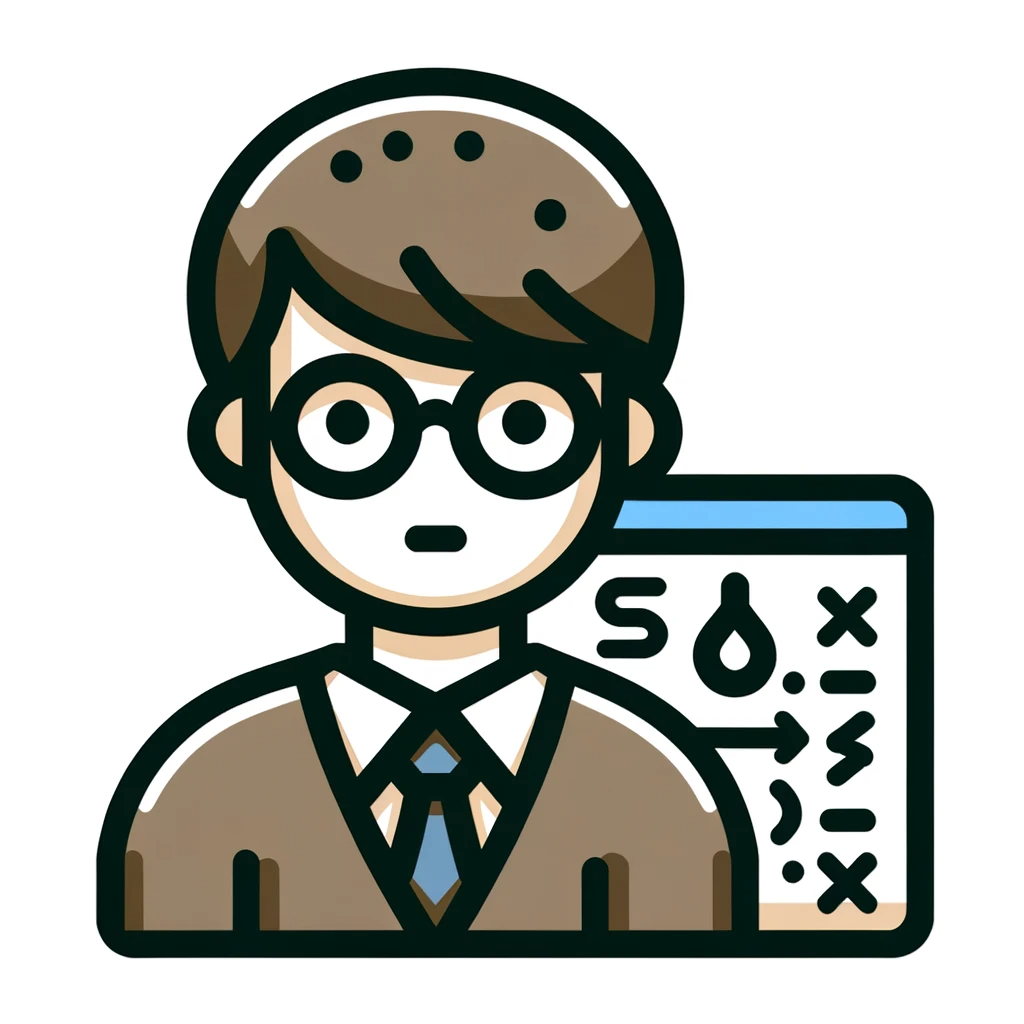
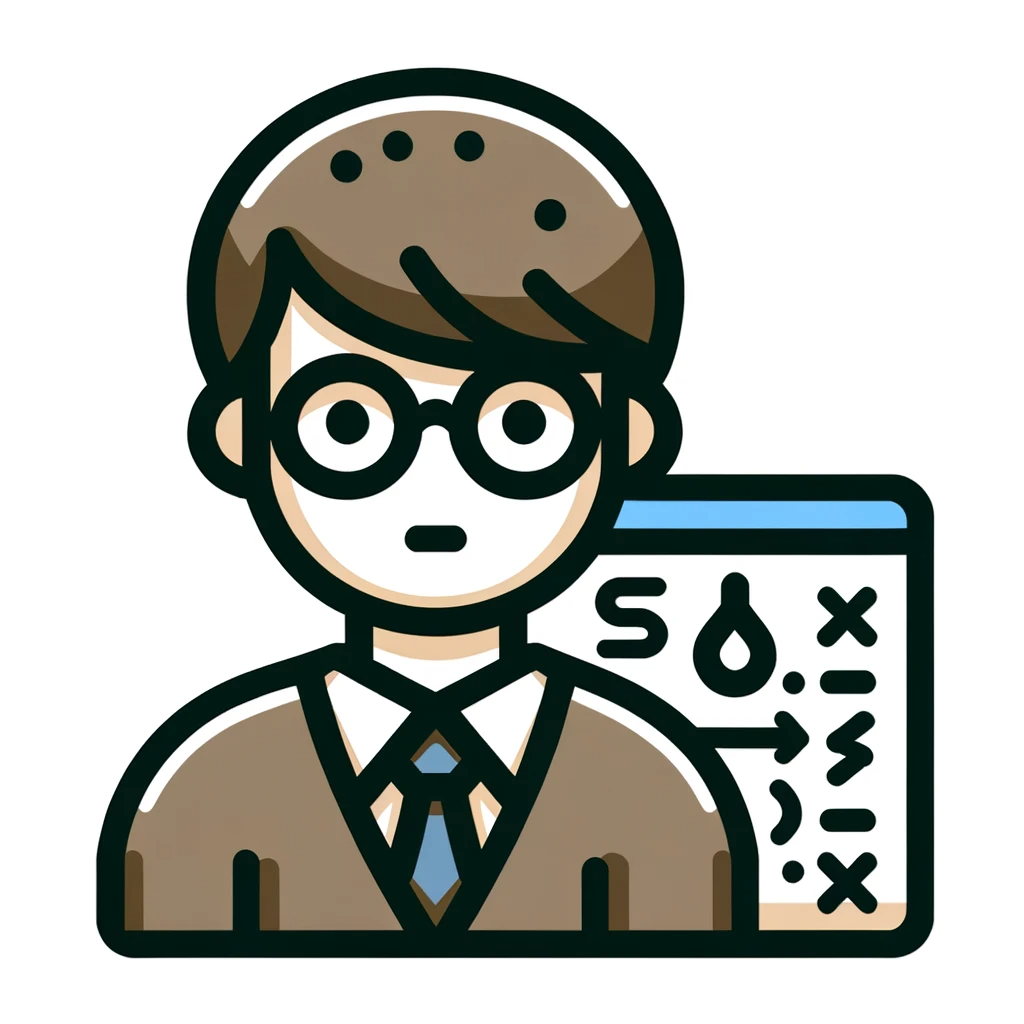
By using the XMLHttpRequest object, you can retrieve XML format data with JavaScript.
What is an XMLHttpRequest object?
The XMLHttpRequest object is a standard JavaScript API for exchanging data between a web page and a server . The XMLHttpRequest object allows you to send a request to a server and receive and process the response after a web page has loaded.
Specifically, it has the following functions.
- Ability to create requests and send them to the server.
- Request headers and response headers can be retrieved.
- The content of the response can be obtained and handled as a DOM object or JSON object.
- Can process multiple requests at the same time.
- You can manage the progress of requests, such as timeouts and progress monitoring.
The XMLHttpRequest object is one of the essential technologies for implementing web applications using asynchronous communication. On the other hand, for security reasons, there are restrictions on cross-domain communication, and CORS (Cross-Origin Resource Sharing) must be configured on the server side. You should also keep an eye on the latest development trends, as the XMLHttpRequest object may be replaced by new APIs such as the Fetch API.
Explanation using a sample program
By using the sample program below, you can use the XMLHttpRequest object to obtain XML format data.
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/sample.xml', true);
xhr.send();
xhr.onreadystatechange = function() {
if (this.readyState === 4 && this.status === 200) {
const responseXML = this.responseXML;
console.log(responseXML);
}
};
First, create an XMLHttpRequest object and use the xhr.open() method to specify the URL containing the XML-format data you wish to retrieve. xhr.send() method is called to send the request to the server. At this time, specify true as the third argument for asynchronous communication.
Next, set a function to the xhr.onreadystatechange property. This function will be called automatically when the request status changes. this.readyState === 4 indicates that the request is complete, and this.status === 200 indicates that a normal response has been returned from the server.
If this condition is satisfied, use the this.responseXML property to obtain the response’s data in XML format. By outputting the acquired data in XML format in console.log(), it can be confirmed that the data has been acquired correctly.
As described above, XML format data can be obtained using the XMLHttpRequest object. The obtained data in XML format can be retrieved using the responseXML property.
summary
We explained how to obtain XML format data using JavaScript.
- The XMLHttpRequest object allows JavaScript to retrieve data in XML format.
- To send a request, use xhr.open() and xhr.send().
- The response is received by xhr.onreadystatechange.
- The data in XML format of the response can be obtained with the responseXML property.
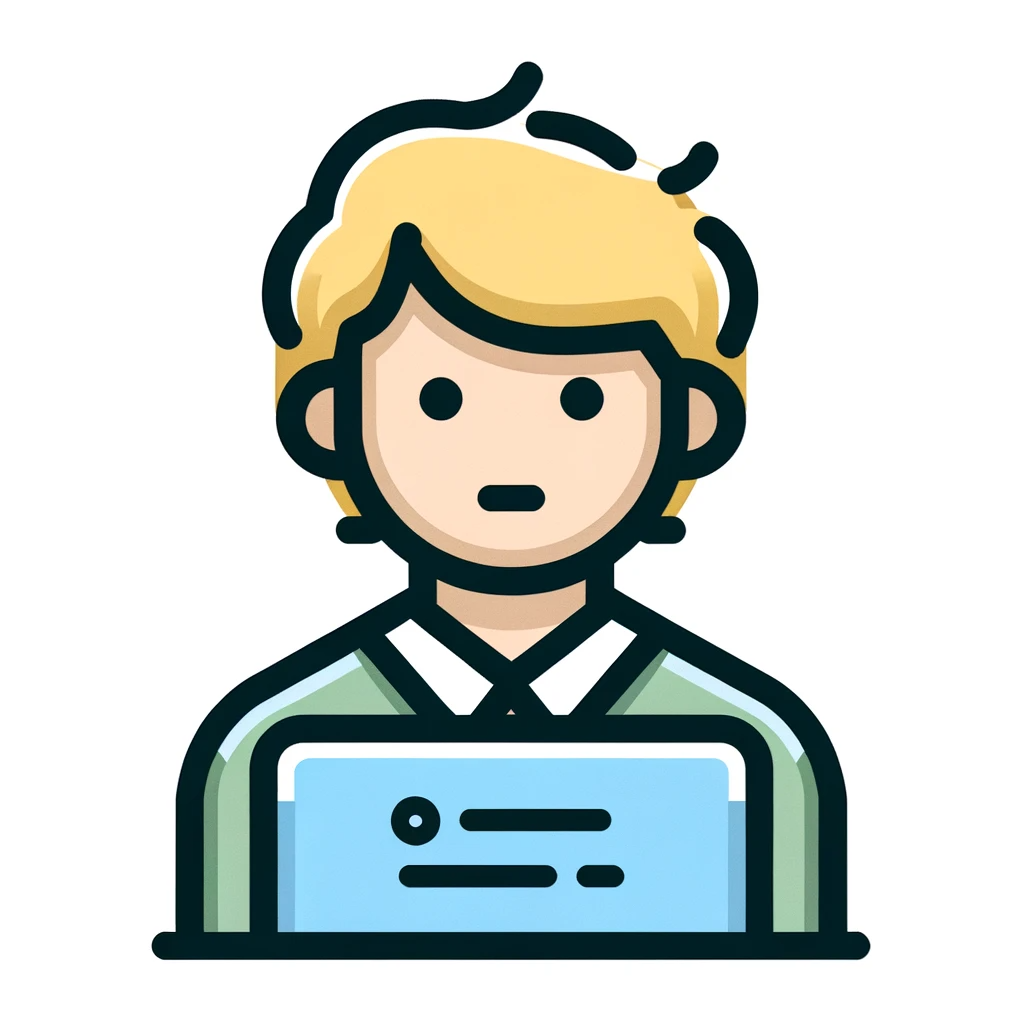
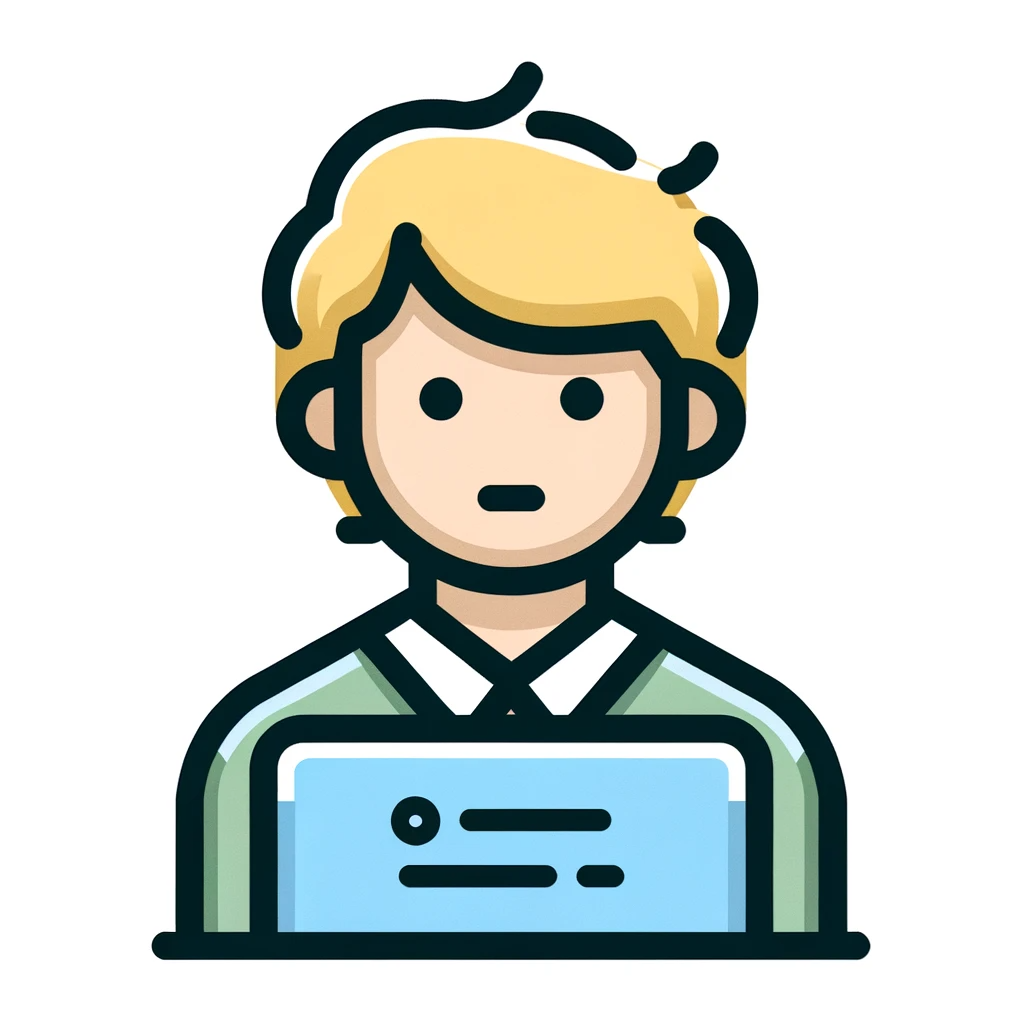
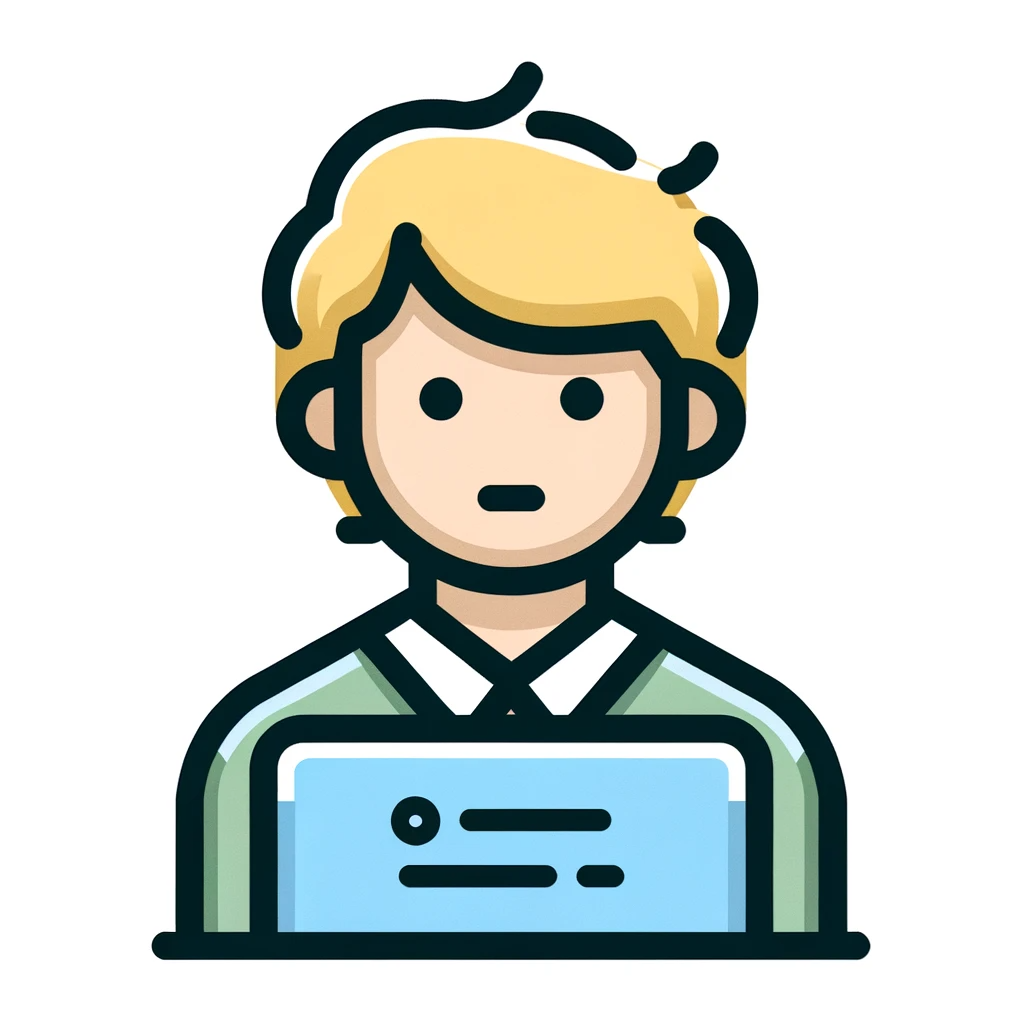
You can easily retrieve XML format data using the XMLHttpRequest object.
I learned a lot.
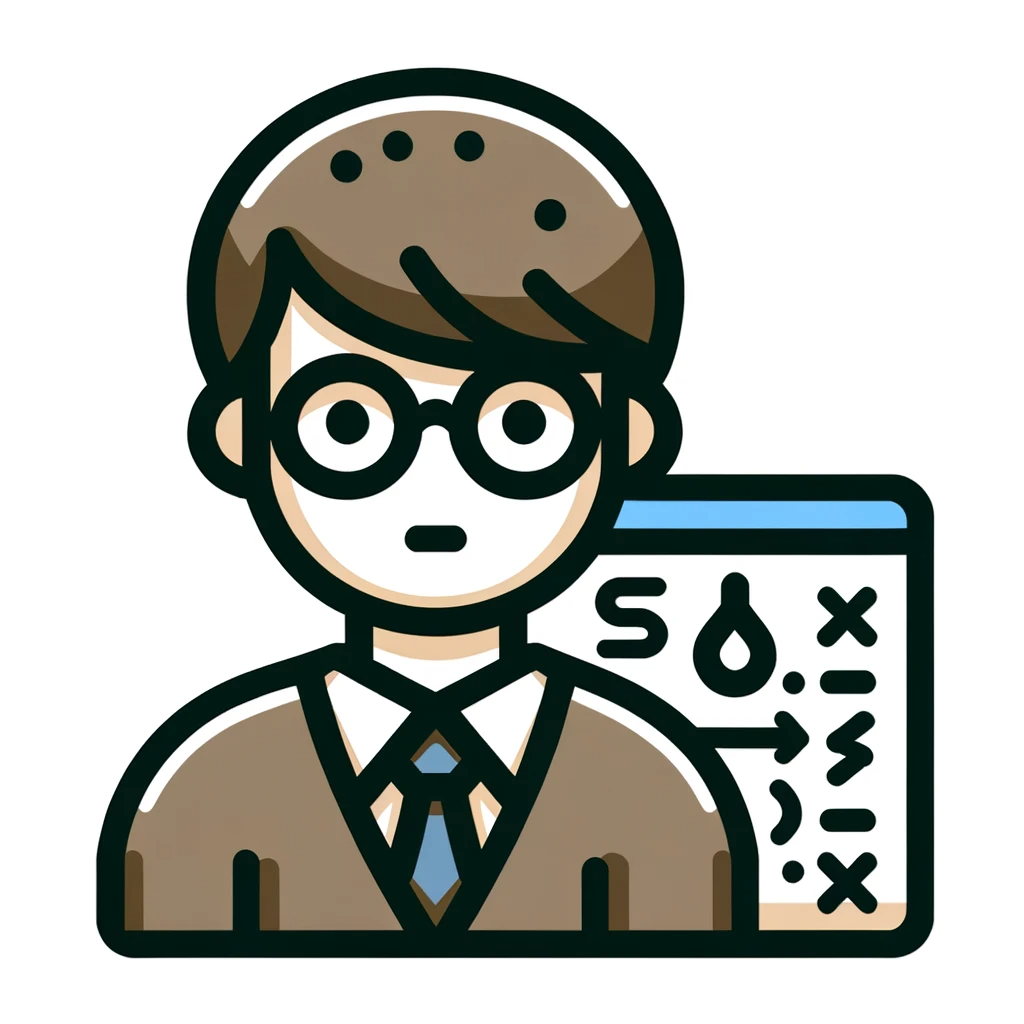
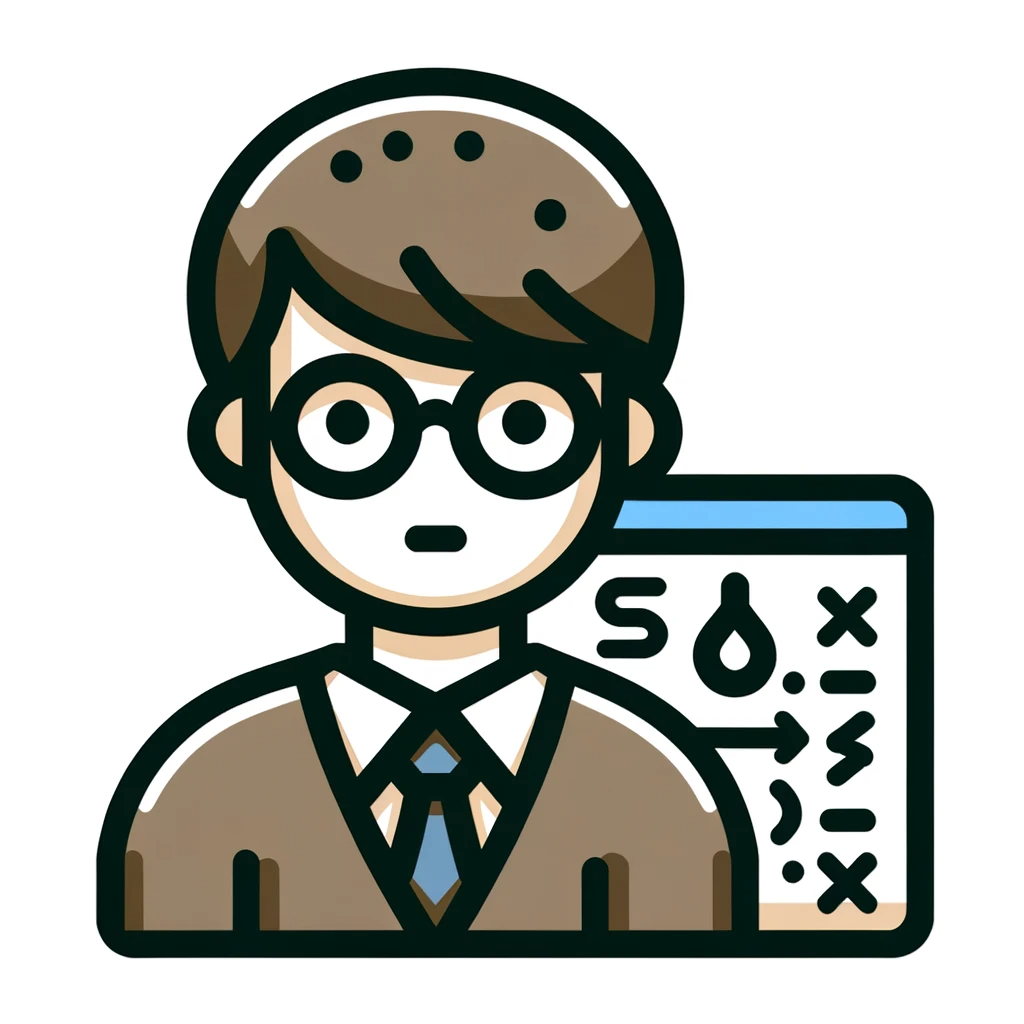
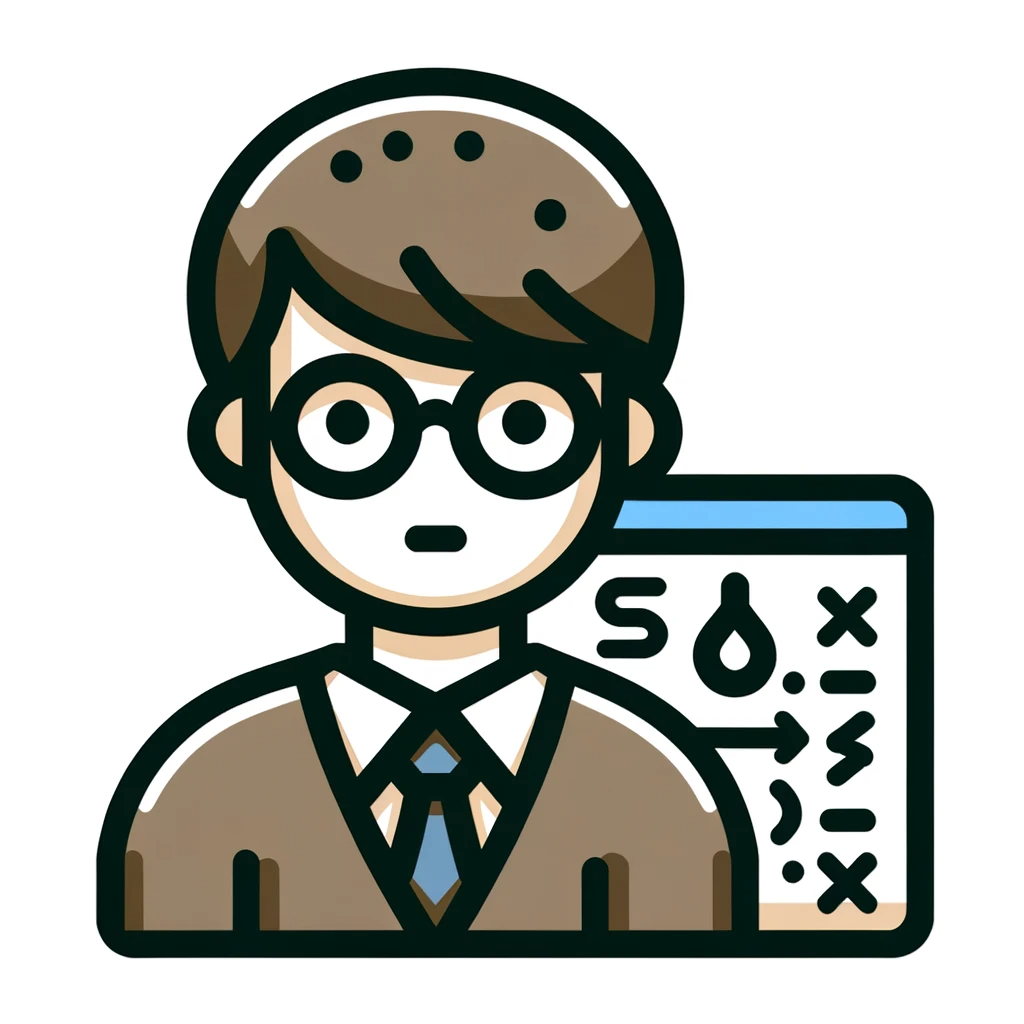
The XMLHttpRequest object allows you to send a request to a server and receive and process the response after a web page has loaded.
However, for security reasons, requests to different domains are restricted, so it is recommended to implement it within the same domain.
Comments