This article explains how to insert a new element at any location using JavaScript’s insertBefore method . It also explains how to move existing elements .
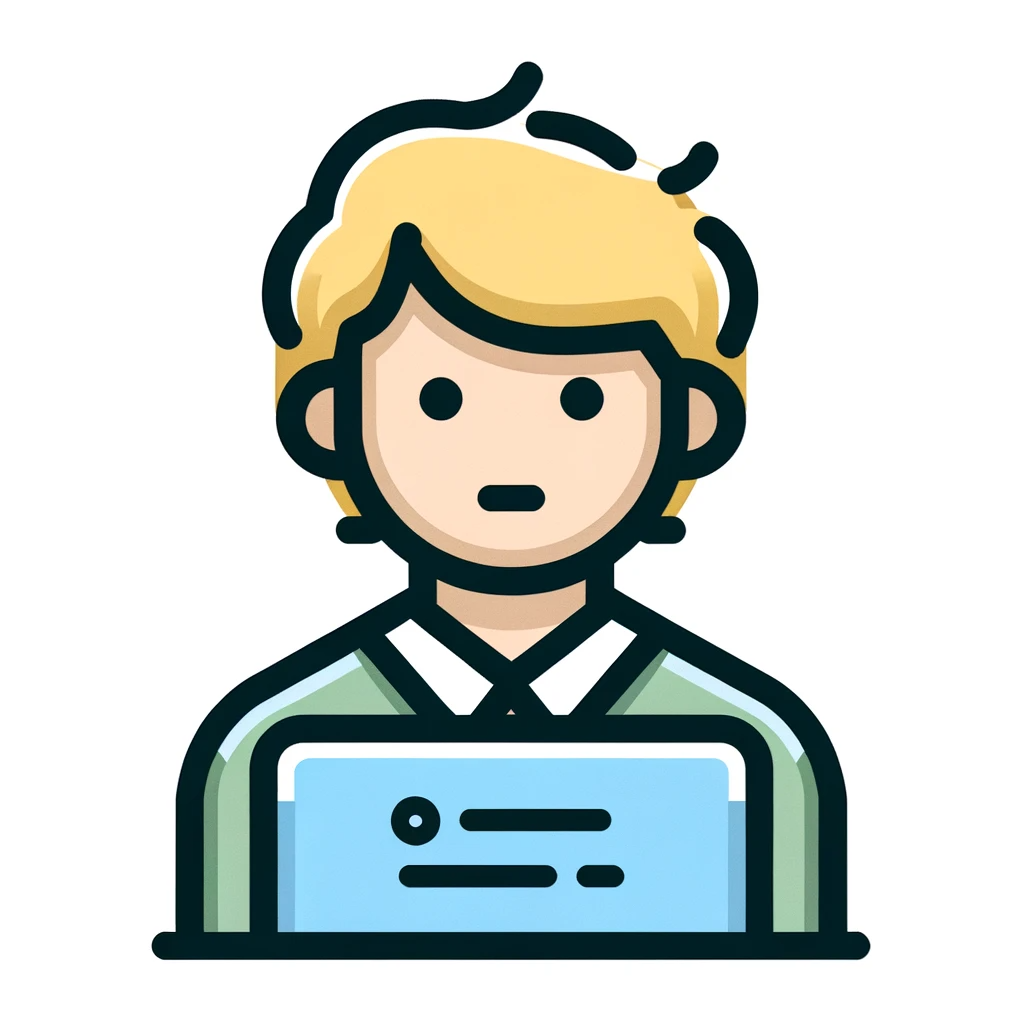
I’m looking into how to dynamically add elements with JavaScript, and it seems like there is a way to use the insertBefore method.
Please teach me how to use it!
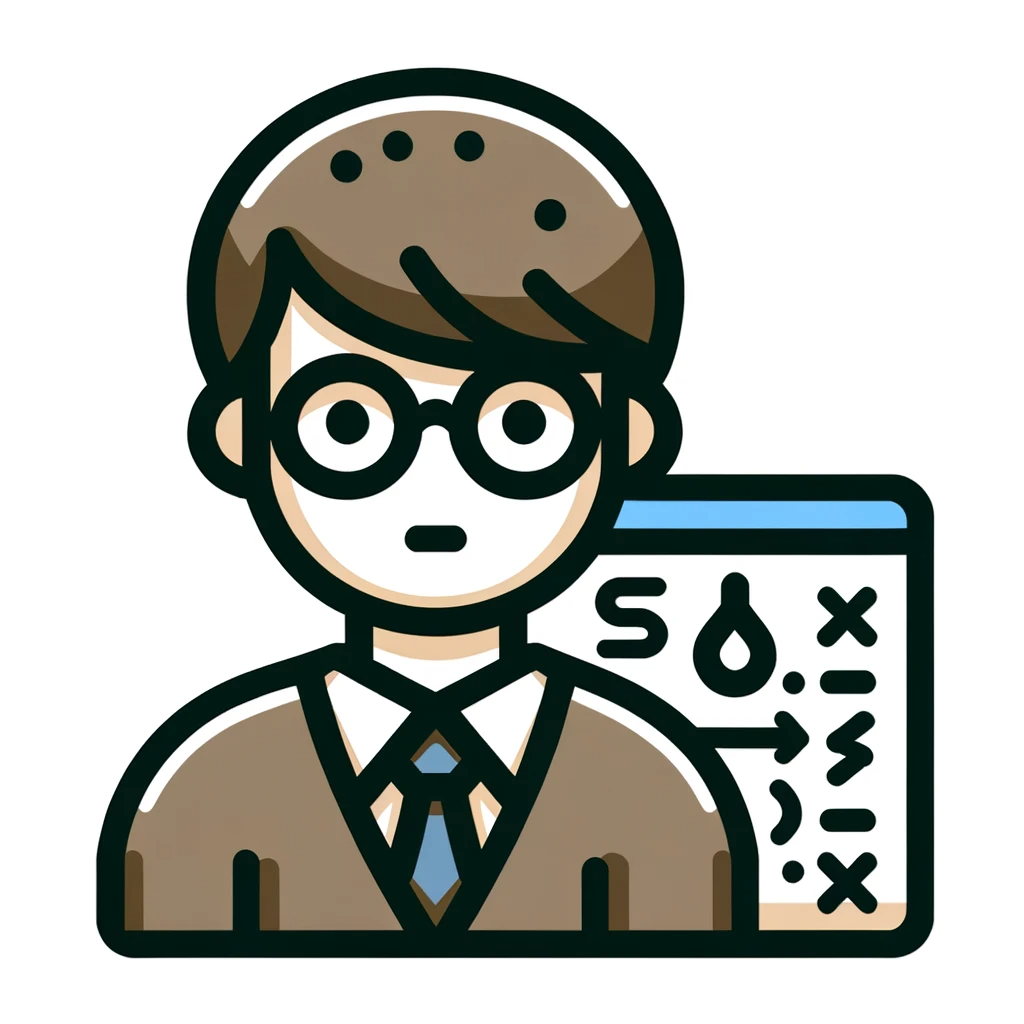
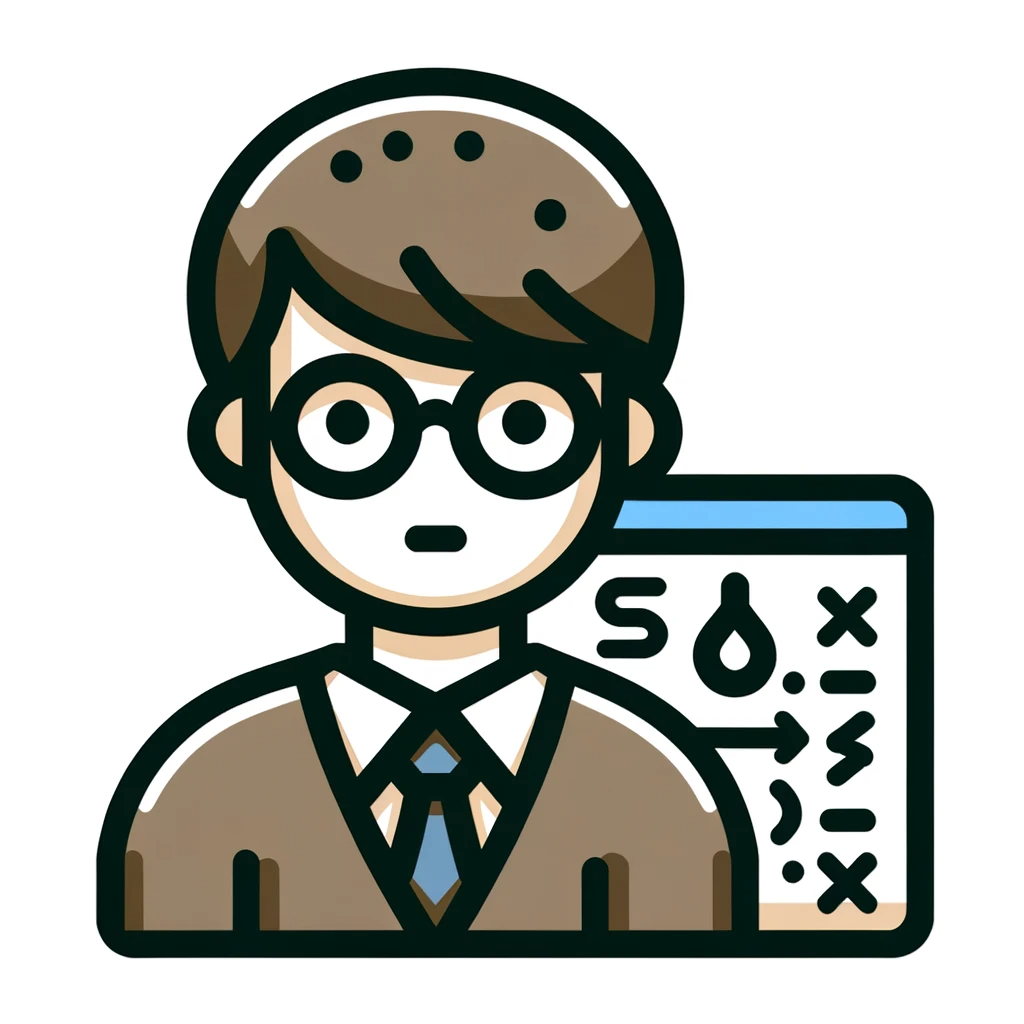
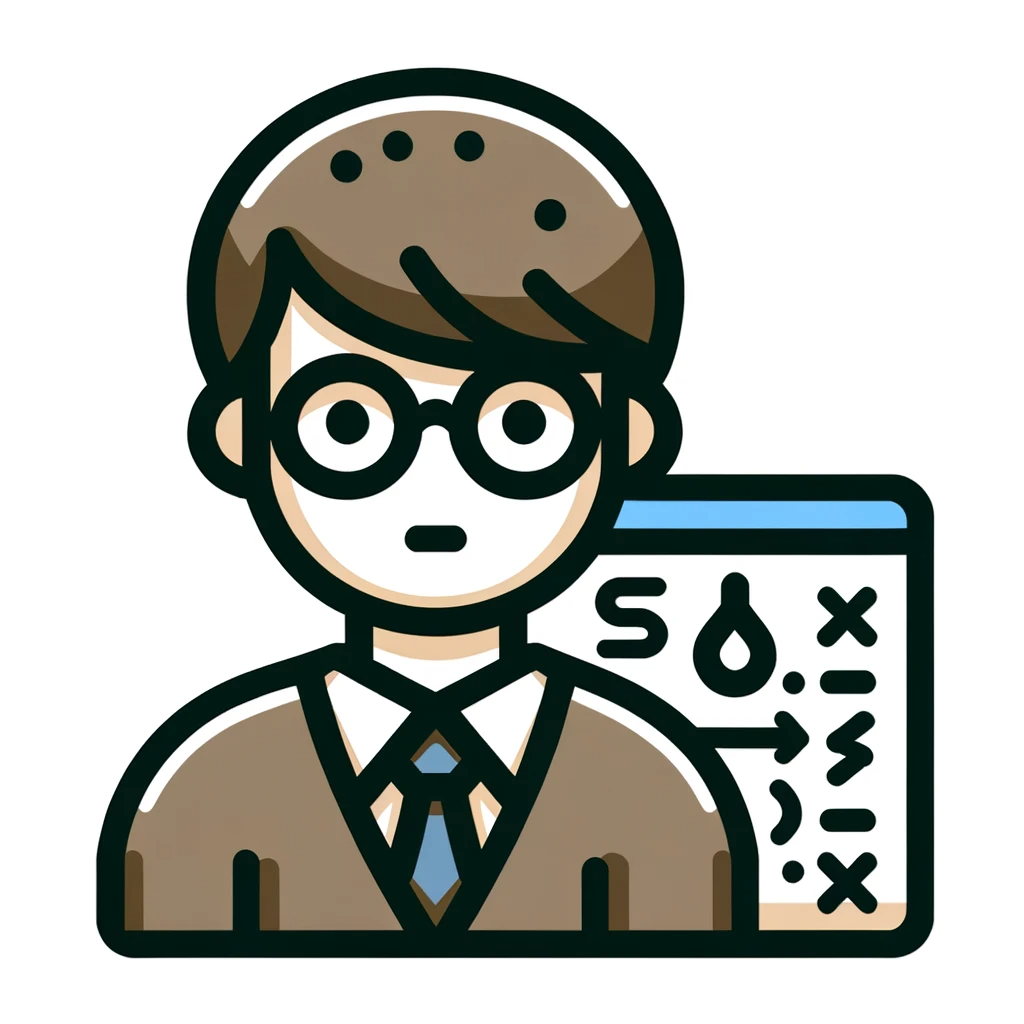
The insertBefore method is used to insert a new element into the parent element.
Specifically, inserts a new element before the specified element among the child elements of the parent element.
Detailed explanation of insertBefore method
Use the insertBefore method to insert an element anywhere .
Use the following format.
parentNode.insertBefore(newNode, referenceNode);
- parentNode: parent element to insert into
- newNode: new element to insert
- referenceNode: existing child element to insert into
For specific usage, the code is as follows.
const parent = document.getElementById('parent');
const newElement = document.createElement('div');
const referenceElement = document.getElementById('reference');
parent.insertBefore(newElement, referenceElement);
This adds a newElement element before the reference element within the parent element .
The sample below is a sample program that inserts a new list item when you click a button.
<button id="add-button">Add new item</button>
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
const addButton = document.getElementById('add-button');
const list = document.getElementById('list');
addButton.addEventListener('click', () => {
const newListItem = document.createElement('li');
newListItem.textContent = 'New item';
list.insertBefore(newListItem, list.children[1]);
});
</script>
In this code, when you click the button “Add new item”, a new list item “New item” is added before “Item 2”.
How to move existing elements with insertBefore
To move an existing element, you must first obtain the element and then obtain the position where you want to insert the element. You can move elements using the insertBefore method .
For example, consider moving the second child element of the ul element before the first child element as shown below.
<ul>
<li>apple</li>
<li>orange</li>
<li>grape</li>
</ul>
const ul = document.querySelector('ul');
const li = ul.getElementsByTagName('li')[1];
const firstChild = ul.firstChild;
ul.insertBefore(li, firstChild);
First, get the element to be moved (second child element). Use getElementsByTagName method to get the li element and item method to get the second child element , as below .
Then get the position to insert (before the 1st child element). Get the first child element (1st child element) of the ul element using the firstChild property as shown below .
Finally, use the insertBefore method to move the element . Insert the li element before firstChild using insertBefore method as below.
By doing this, the order of the child elements of the ul element will be changed from apple, orange, grape to orange, apple, grape.
Below is a sample program implementing the above example.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>How to move existing elements</title>
</head>
<body>
<ul>
<li>apple</li>
<li>orange</li>
<li>grape</li>
</ul>
<script>
const ul = document.querySelector('ul');
const li = ul.getElementsByTagName('li')[1];
const firstChild = ul.firstChild;
ul.insertBefore(li, firstChild);
</script>
</body>
</html>
Summary of this article
We explained how to insert a new element at any location using the insertBefore method .
We also explained how to move existing elements .
Summary of how to insert a new element at any location using the insertBefore method
- The insertBefore method inserts a new element before the specified position within the parent element.
- Use in the format parentNode.insertBefore(newNode, referenceNode)
[parentNode: parent element to insert into, newNode: new element to insert, referenceNode: specifies an existing child element to base the insertion on] - A new element is added at the specified position
Summary of how to move existing elements using the insertBefore method
- To move an existing element, get the element, get the insert position, and use the insertBefore method.
- To retrieve elements, use querySelector or getElementsByTagName method.
- To get the insertion position, get the element located before the target element.
- Specify the element you want to move in the first argument of the insertBefore method, and the element at the insertion position in the second argument.
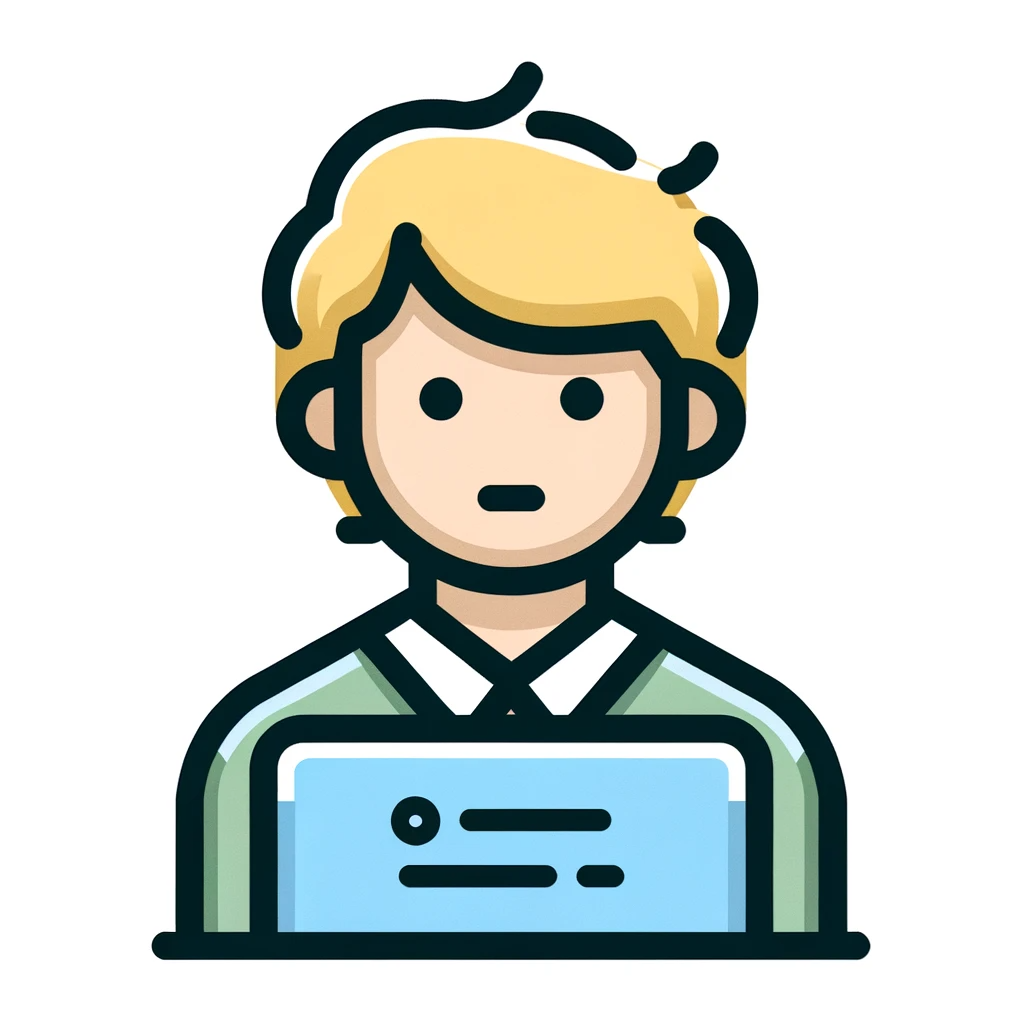
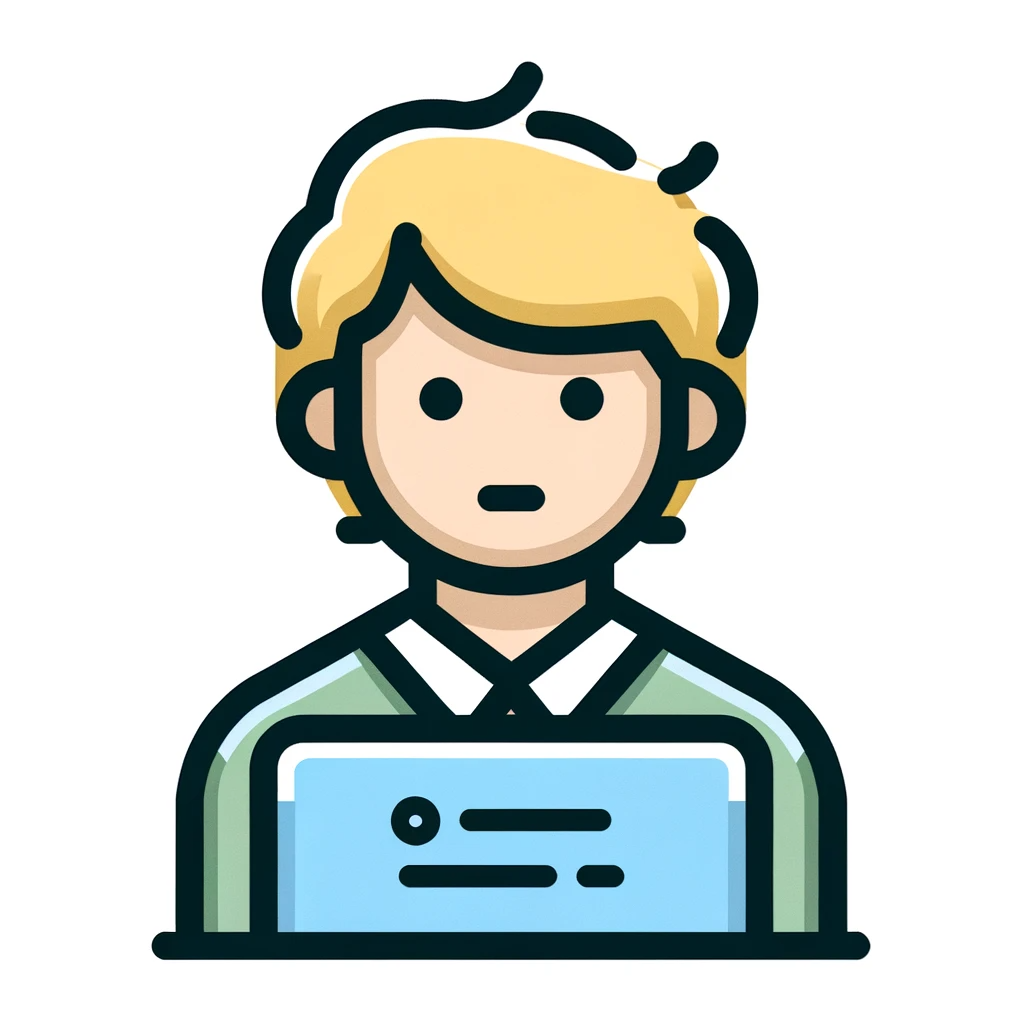
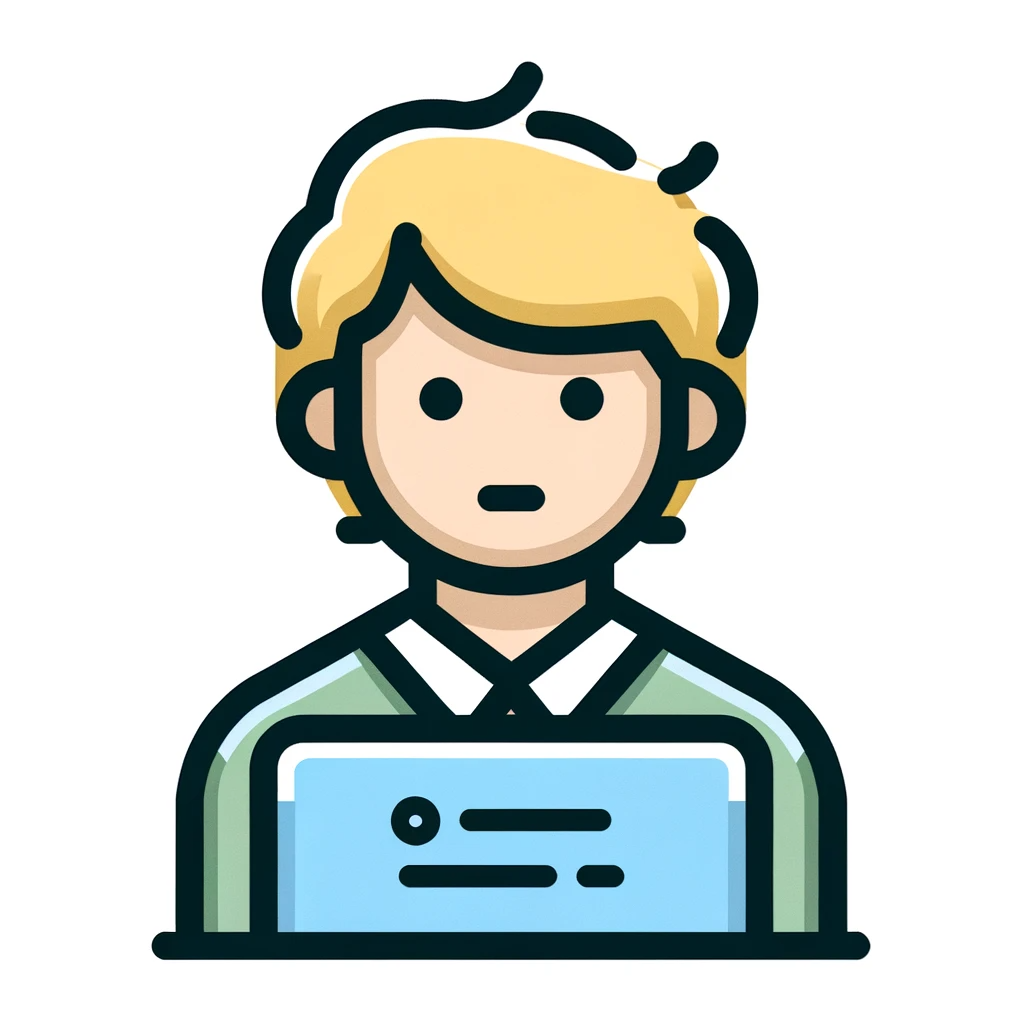
I see, you can dynamically insert elements using the insertBefore method.
Now you can create dynamic pages without any problems.
thank you!
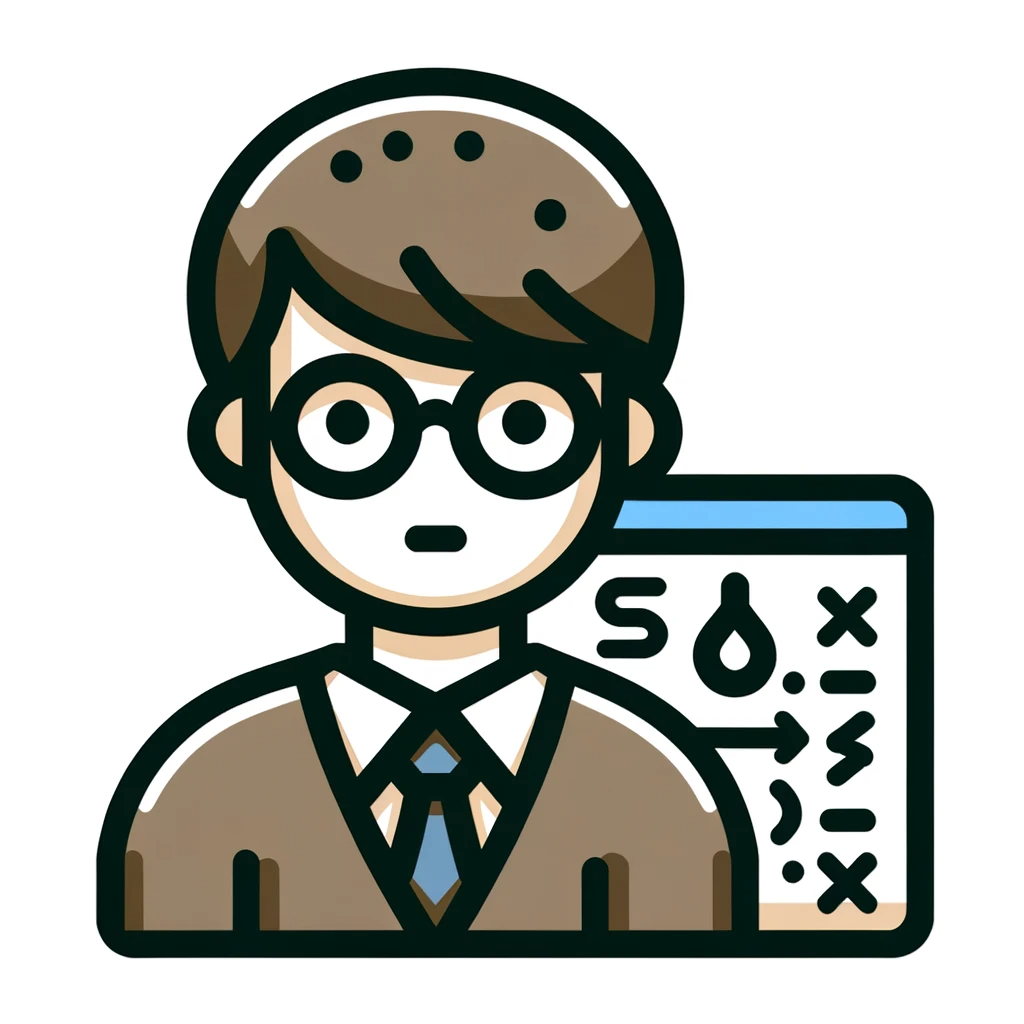
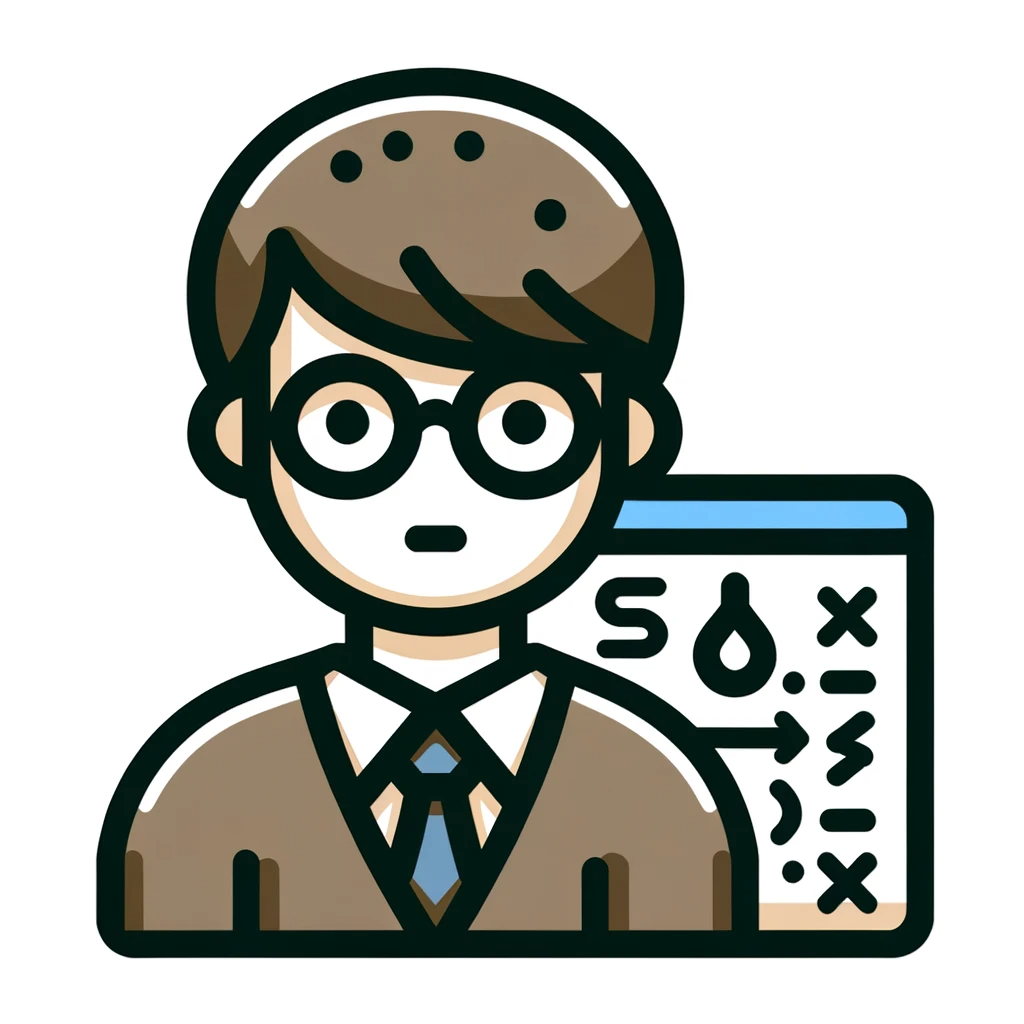
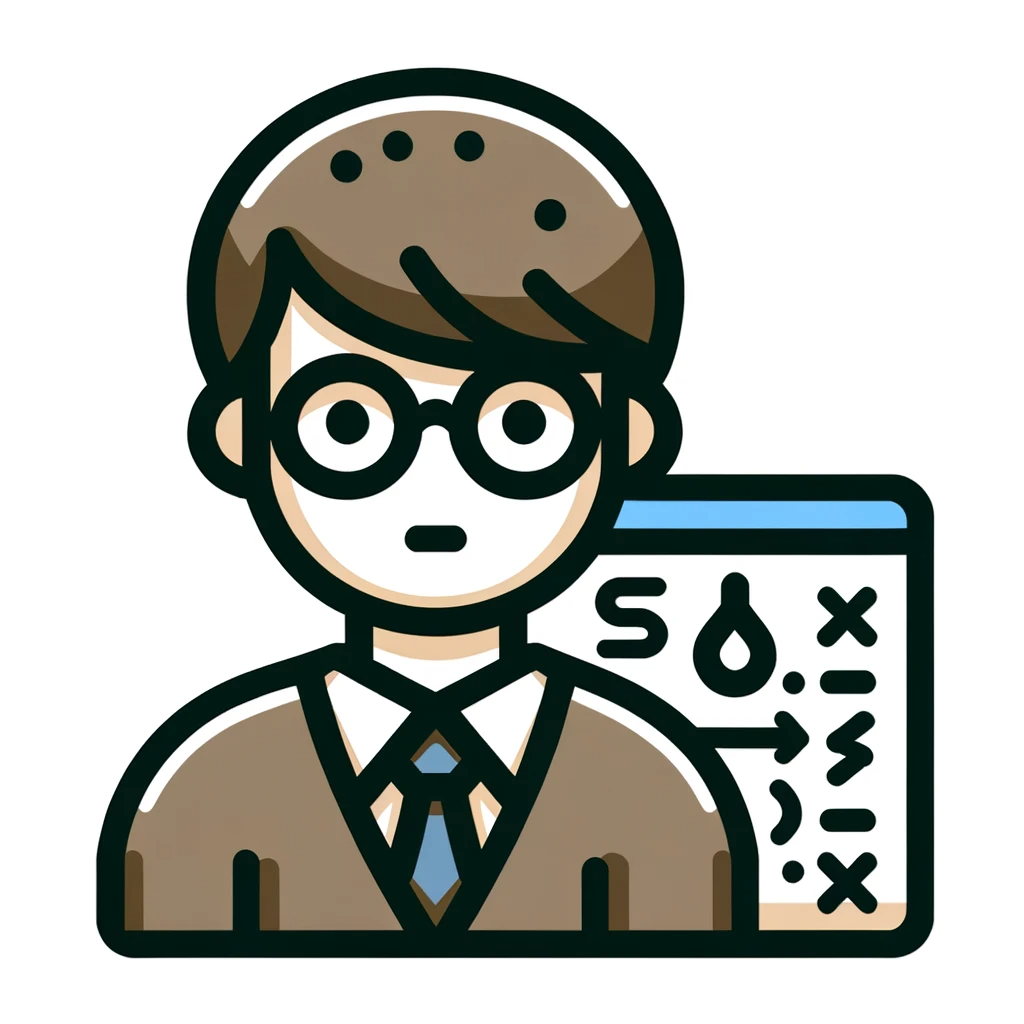
The insertBefore method is useful when adding elements dynamically.
It is important to specify exactly which existing child element to base the insertion on.
It is also convenient to use the document.createElement() method when creating new elements.
When adding dynamic elements, it is important to dynamically add them using JavaScript instead of relying on HTML code.
Comments