We will show you how to use JavaScript pseudo-classes to switch styles depending on the result of form validation and how to customize messages during validation.
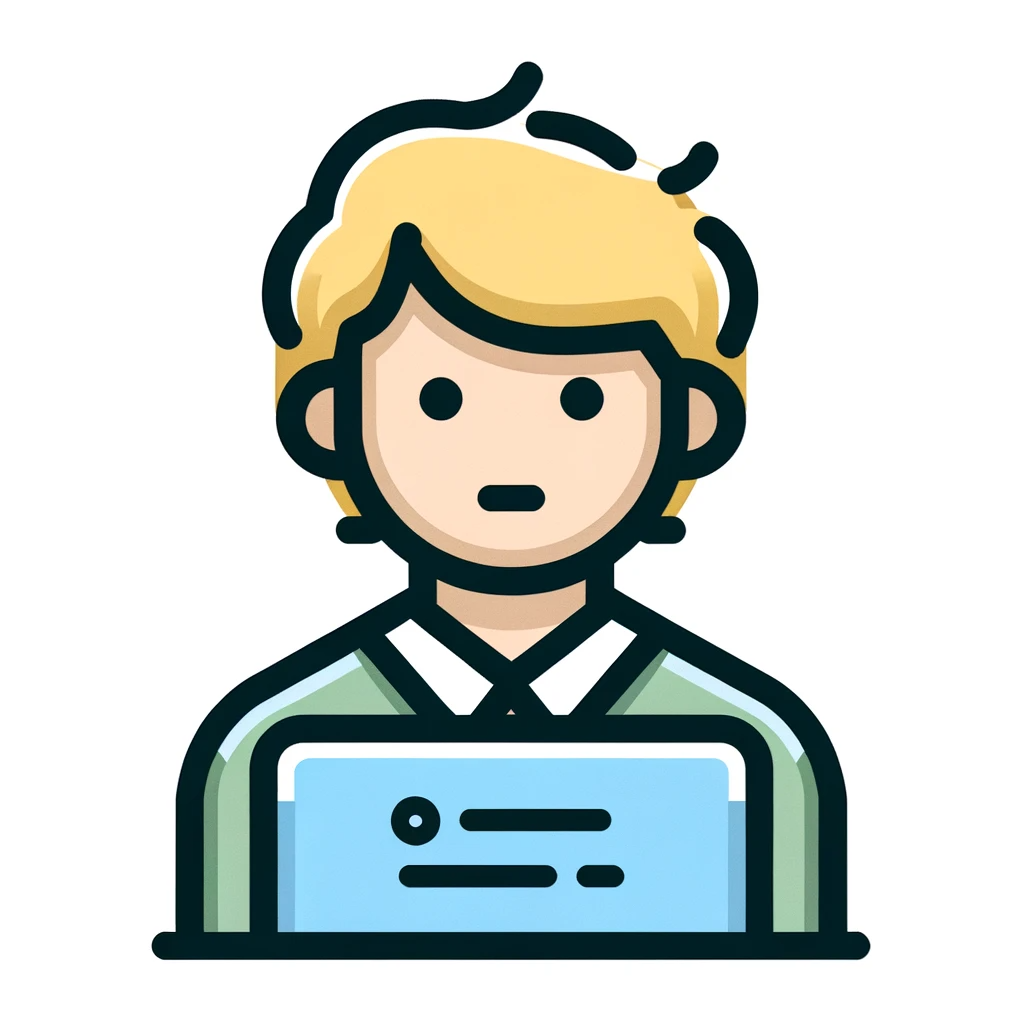
I would like to know how to switch styles depending on the result of form validation.
What should I do?
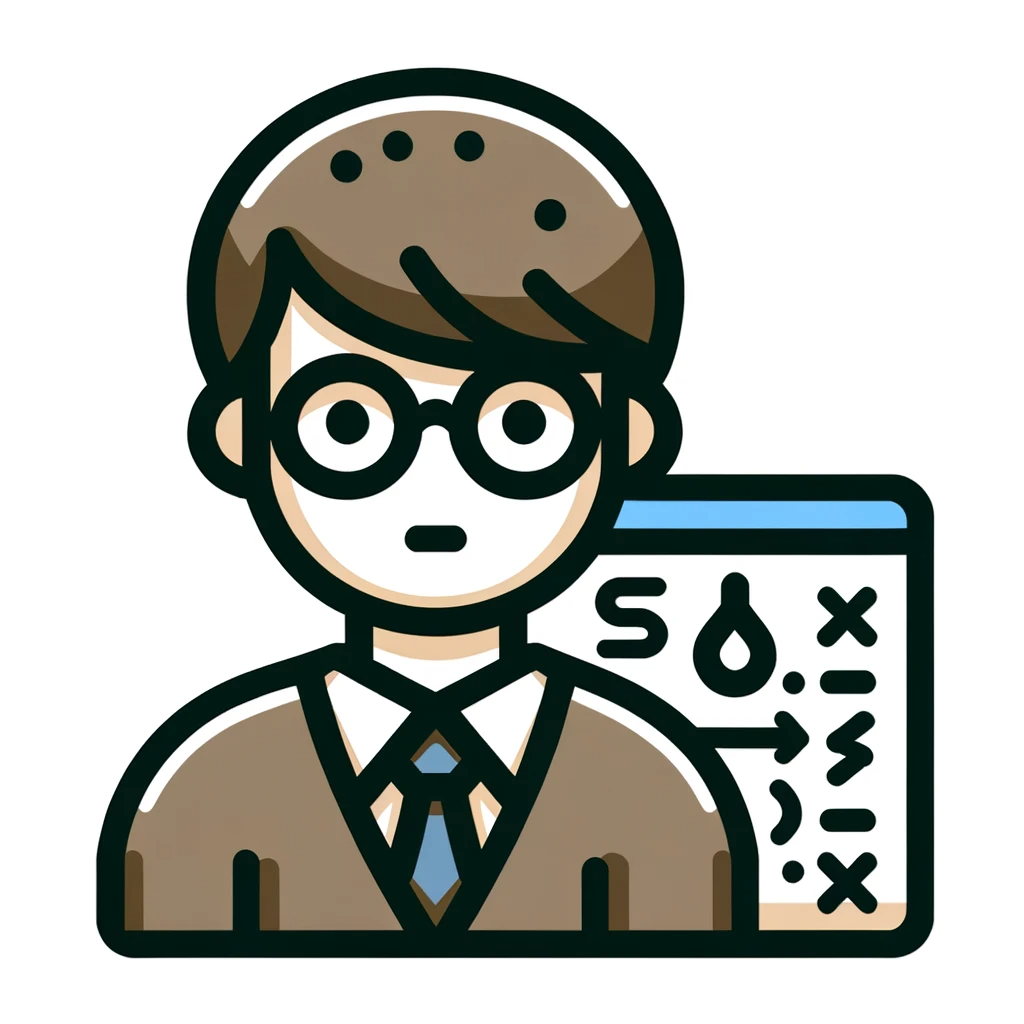
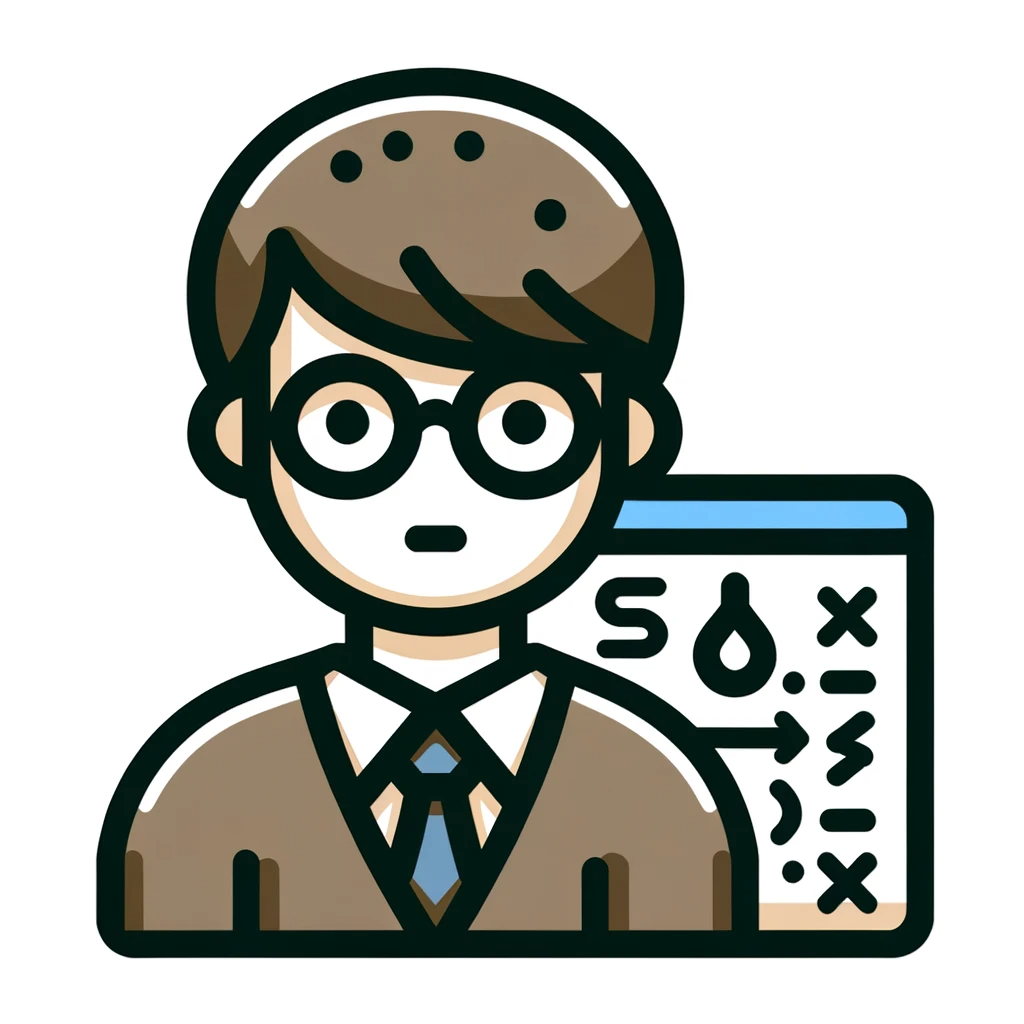
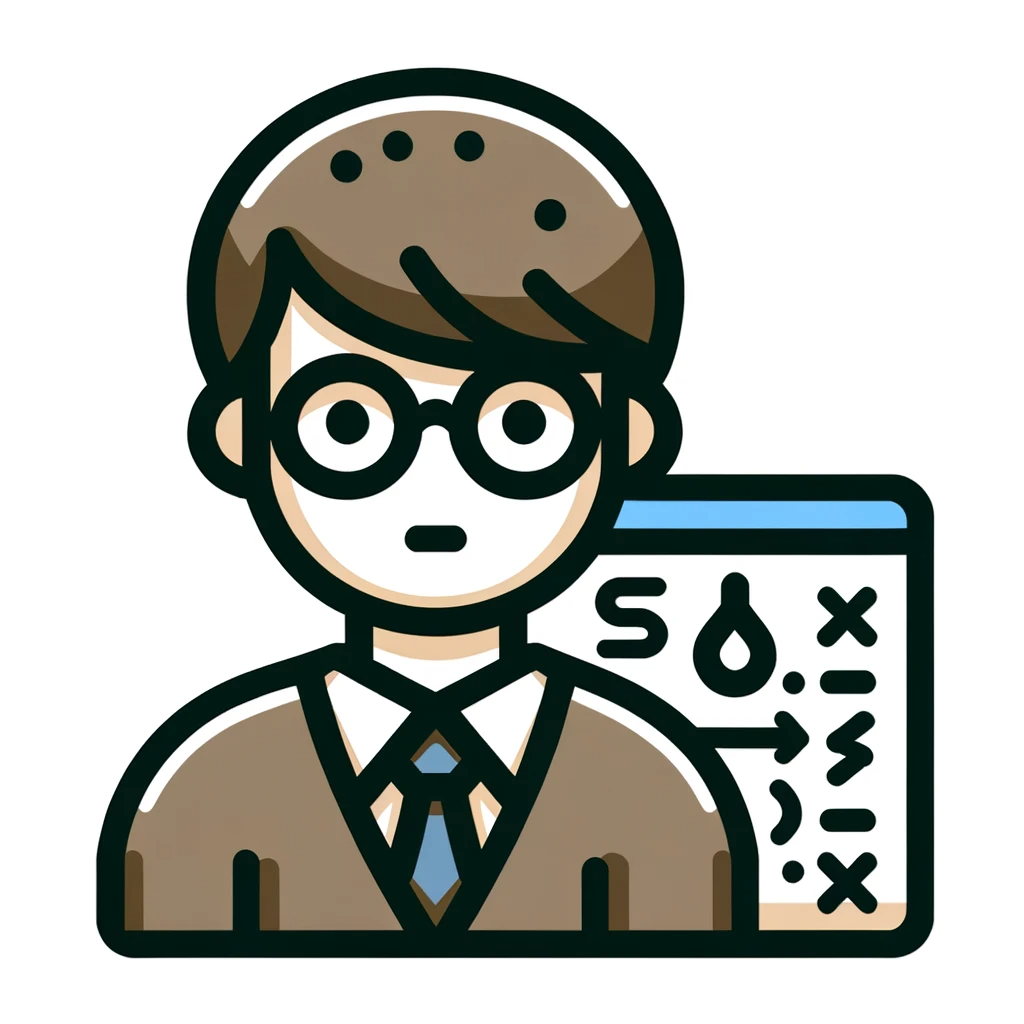
By using pseudo-classes, you can apply a green border to elements that pass form validation, and a red border to elements that fail.
Use :valid and :invalid pseudo-classes.
How to switch styles using classes during input judgment
To determine the validation status of input judgment, use the following pseudo-class .
pseudo class | meaning |
---|---|
:valid | Matches elements that pass form validation. |
:invalid | Matches elements that fail form validation. |
:required | Matches elements that have the required attribute set. |
:optional | Matches elements that do not have the required attribute set. |
:in-range | Matches elements with values within the range of min and max attributes. |
:out-of-range | Matches elements with values outside the range of min and max attributes. |
:valid + label | Matches the label as the next sibling element of an element that passes form validation. |
:invalid + label | Matches the label as the next sibling element of an element that fails form validation. |
These pseudo-classes can be used to apply styles to elements depending on the results of form validation.
For example, you can use the :valid pseudo-class to mark elements that pass form validation with a green border. You can
also use the :invalid pseudo-class to add a red border to elements that fail form validation .
A sample that changes the style depending on the verification result using a pseudo class
The sample program below shows how to apply styles to elements depending on the results of form validation. Use a pseudo-class to mark elements that pass form validation with a green border and elements that fail with a red border.
<!DOCTYPE html>
<html>
<head>
<style>
input:valid {
border: 2px solid green;
}
input:invalid {
border: 2px solid red;
}
</style>
</head>
<body>
<form>
<label for="username">username:</label>
<input type="text" id="username" name="username" required>
<label for="password">password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
</body>
</html>
This example uses the :valid pseudo-class to display a green border around the required username and password elements if they have input. It also uses the :invalid pseudo-class to display a red border around the username and password elements when input is required but none is entered.
In this sample program, :valid and :invalid pseudo-classes are used for input elements. The :valid pseudo-class matches elements that pass form validation, and the :invalid pseudo-class matches elements that fail form validation. These pseudo-classes allow you to easily apply styles to elements.
How to customize validation messages
Form validation displays a default error message. However, it is also possible to customize these messages. Here’s how.
Configuring messages using the required attribute
In HTML5, using the required or pattern attributes automatically displays a standard error message. To customize these messages, write them in the HTML as shown below.
<input type="text" id="email" name="email" required
oninvalid="this.setCustomValidity('Enter your email address')"
onchange="this.setCustomValidity('')">
In the example above, a custom message will be displayed if you do not enter an email address. setCustomValidity()
You can display your own messages using methods.
Configuring messages using ValidityState objects
The ValidityState object represents the validation state of an input element, and is referenced by the input element’s validity property after validation has been performed. You can use this to customize messages using JavaScript.
Below are the main properties of the ValidityState object and their descriptions.
properties | explanation |
---|---|
valueMissing | Requires an input value but returns true if no value is provided |
typeMismatch | Returns true if the input value is incompatible with the specified type attribute |
patternMismatch | Returns true if the input value does not match the specified regular expression pattern |
tooLong | Returns true if the input value exceeds the number of characters specified in the maxlength attribute |
tooShort | Returns true if the input value is less than the number of characters specified in the minlength attribute |
rangeUnderflow | Returns true if the input value is less than the value specified in the min attribute |
rangeOverflow | Returns true if the input value is greater than the value specified in the max attribute |
stepMismatch | Returns true if the input value is not divisible by the value specified in the step attribute |
customError | Returns true if a custom error message is set |
valid | Returns true if the input value passes validation |
These properties represent validation states specific to each input element. Input element validation states can be used in conjunction with other objects and properties available in JavaScript. For example, you can use the valueMissing property of the ValidityState object to display a message if a required field is missing.
The valid property will be true if all the above properties are false. In other words, it returns true if there is nothing wrong with the input value. The valid property can be used to determine whether an input element passes validation.
Below patternMismatch
is an example code that I converted in JavaScript to display a custom error message when
const emailInput = document.getElementById('email');
emailInput.addEventListener('input', function() {
if (emailInput.validity.patternMismatch) {
emailInput.setCustomValidity('Email address format is incorrect');
} else {
emailInput.setCustomValidity('');
}
});
In this example, we register an event listener for the first event email
. input
And input
when the event occurs, the entered value is patternMismatch
checked by the property.
If the input value does not match the regular expression pattern, setCustomValidity()
use the method to set a custom error message. On the other hand, if the input value matches the regular expression pattern, setCustomValidity()
use the method to clear the error message.
In this way, ValidityState
you can set custom error messages on input elements using object properties.
Summary of this article
We explained how to use pseudo-classes to switch styles depending on the result of form validation and how to customize messages during validation.
- The :valid pseudo-class is applied to elements that pass form validation, and the :invalid pseudo-class is applied to elements that fail form validation.
- Pseudo-classes allow you to easily apply styles to elements.
- To use pseudo-classes, you need to add the required attribute to the HTML element.
ValidityState
You can set custom error messages on input elements using object properties.setCustomValidity()
You can use methods to set custom error messages on input elements.
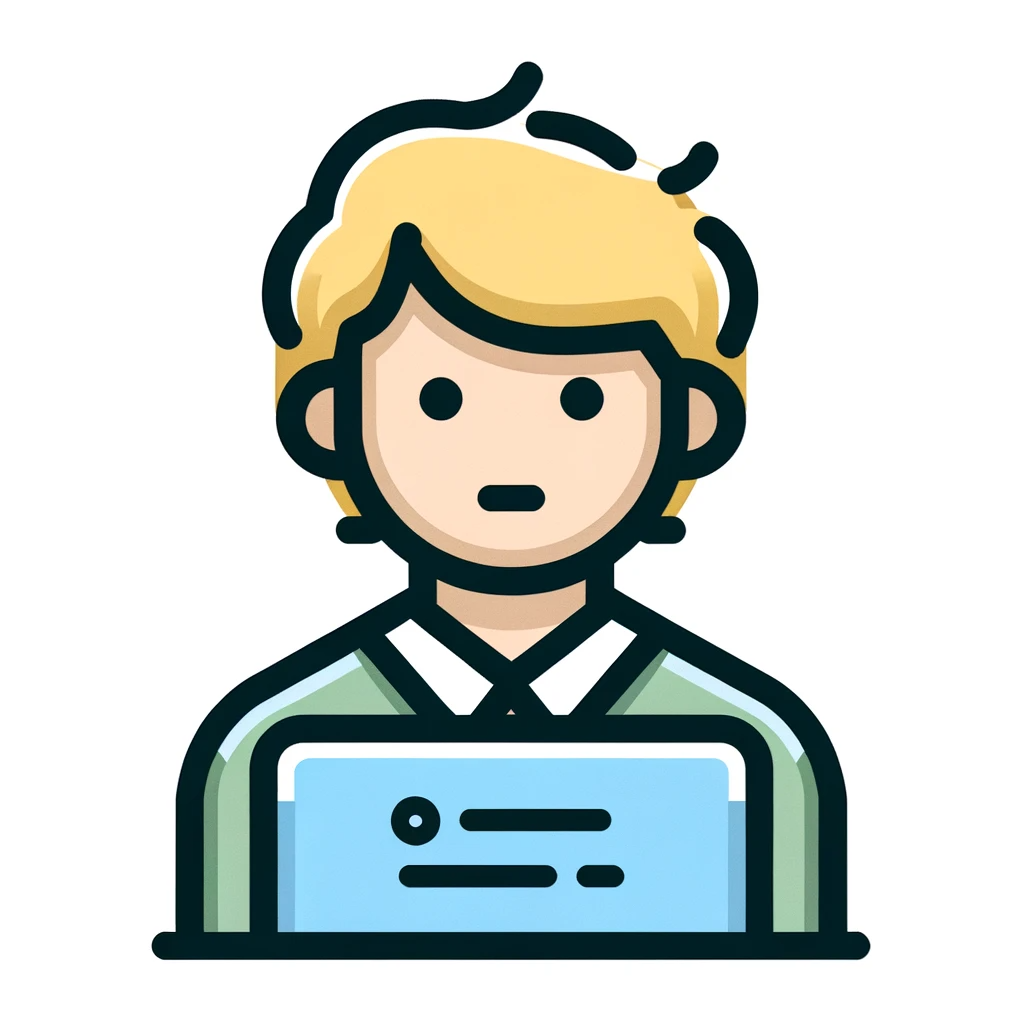
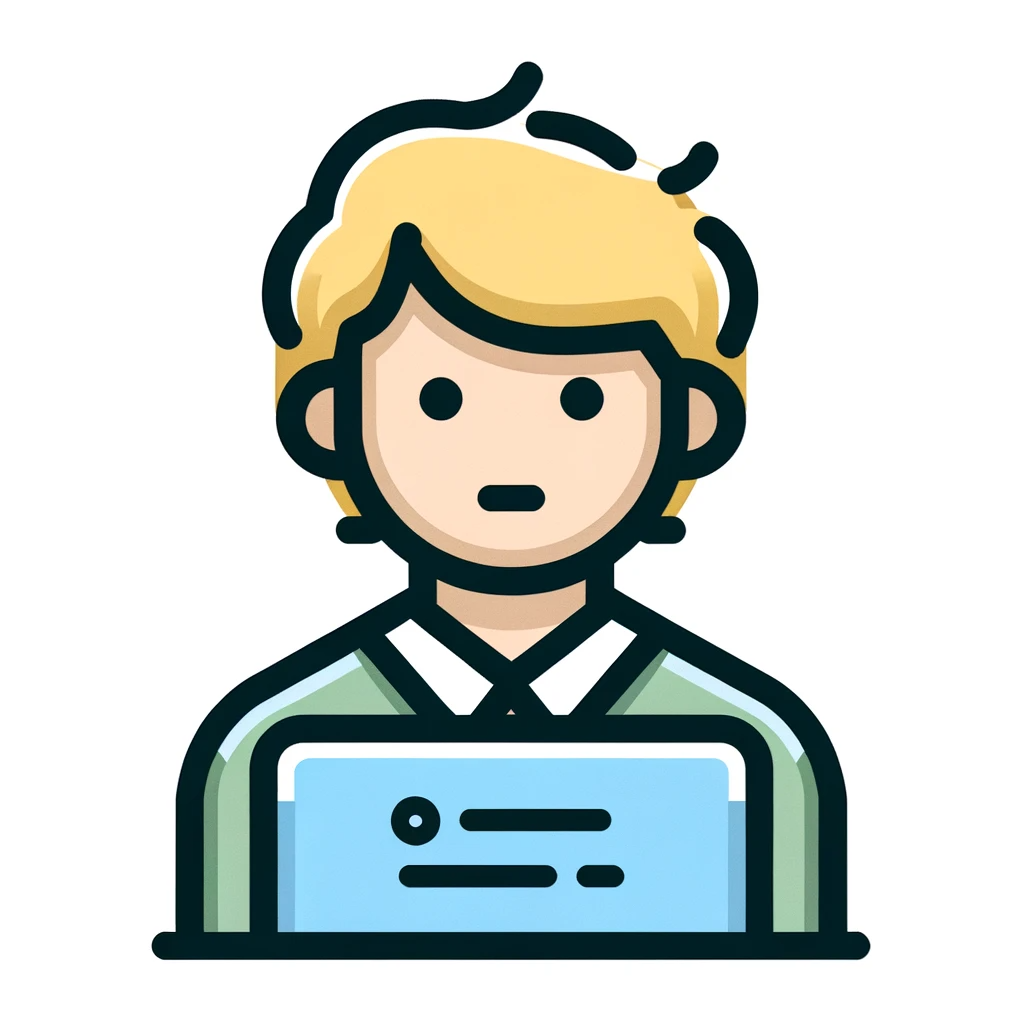
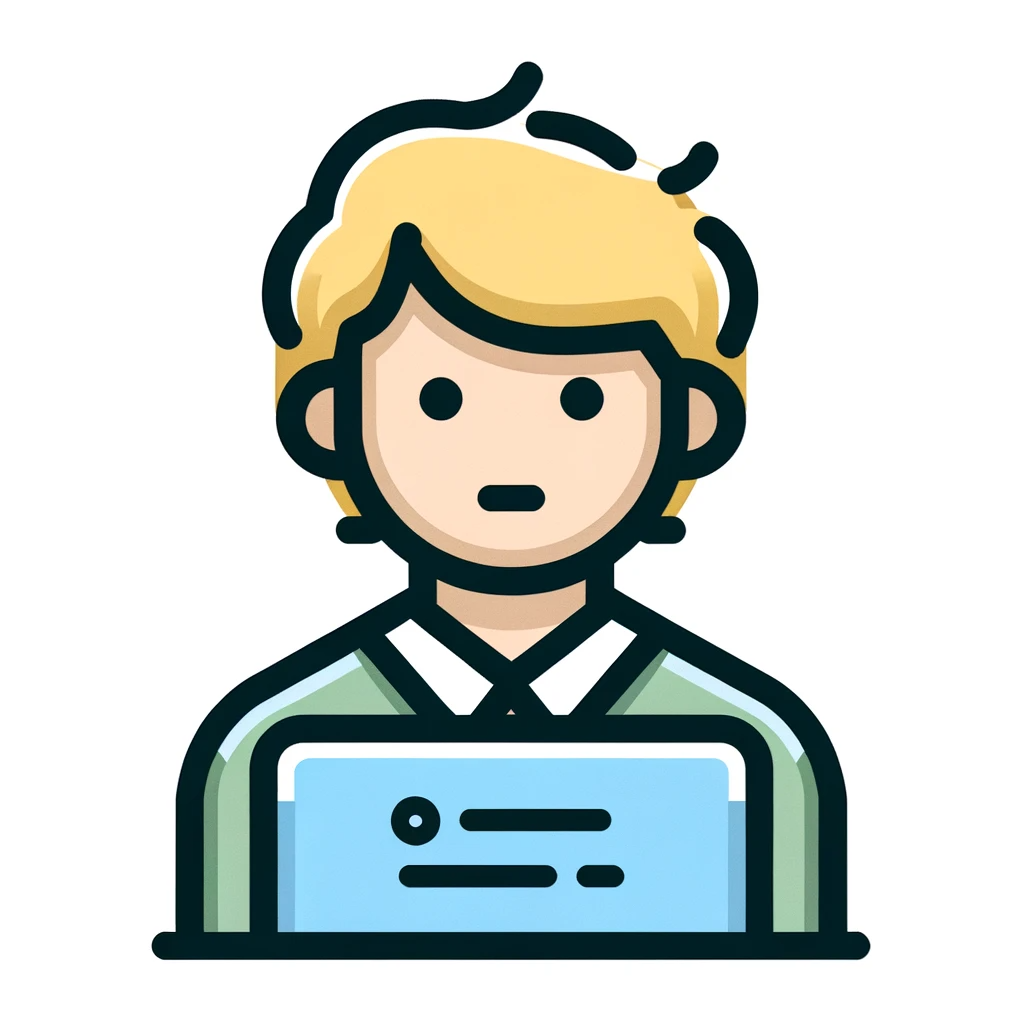
I was surprised at how easy it was to switch styles according to the results of form validation just by using pseudo-classes.
I also wanted to know how to customize form validation messages, so that was very helpful!
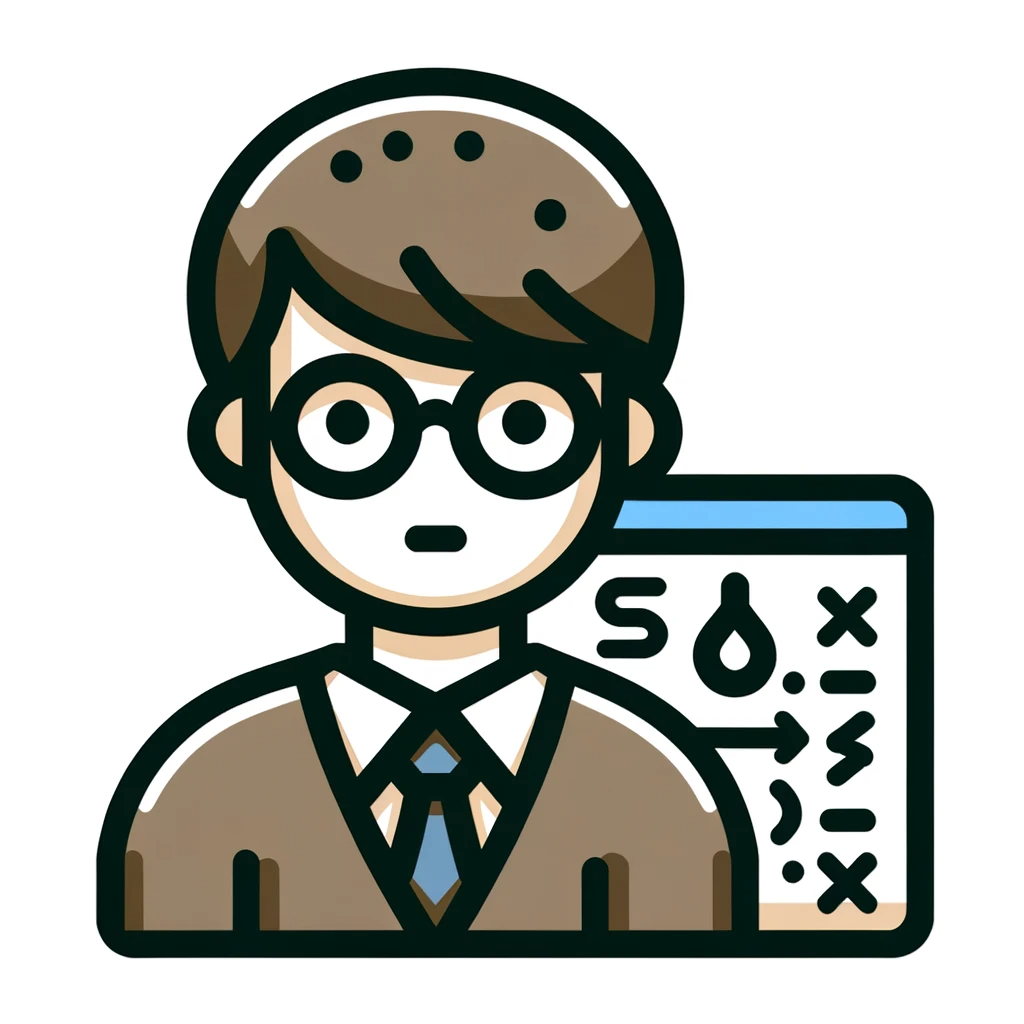
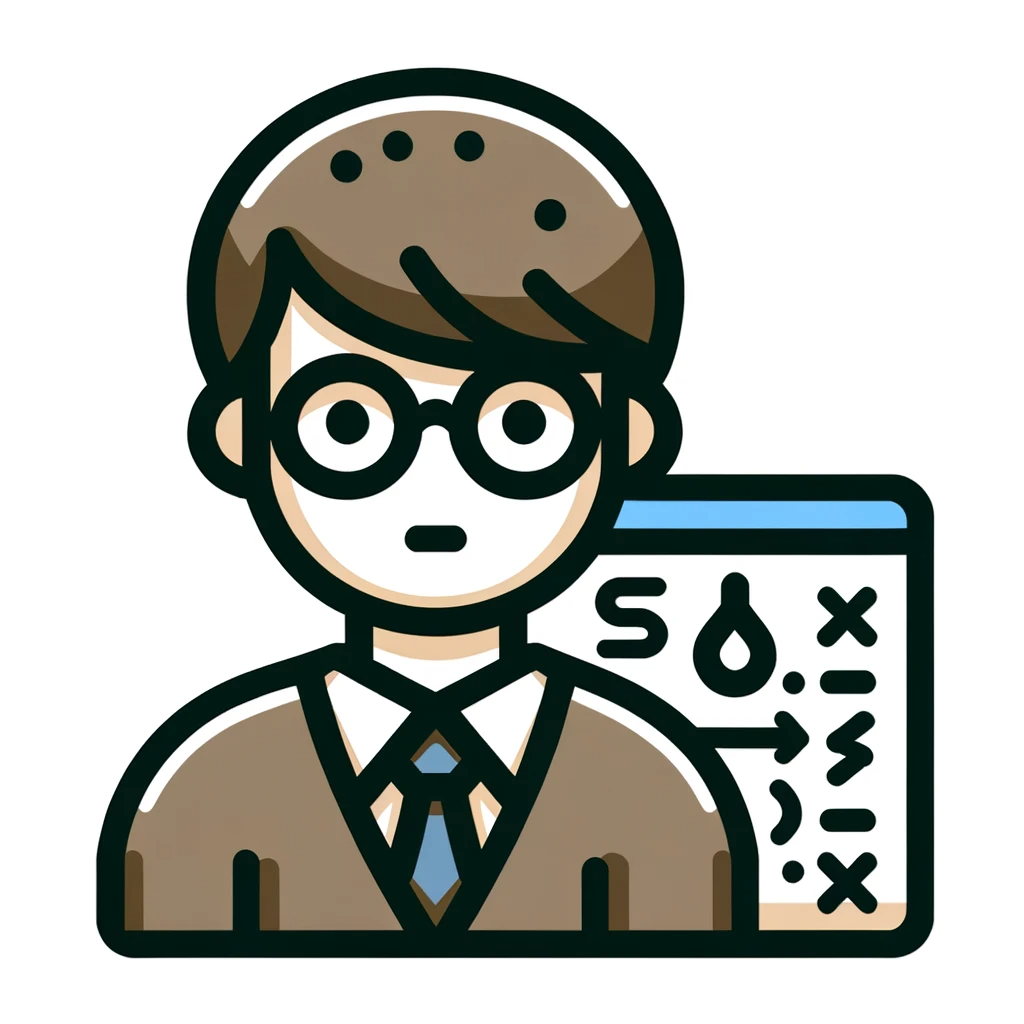
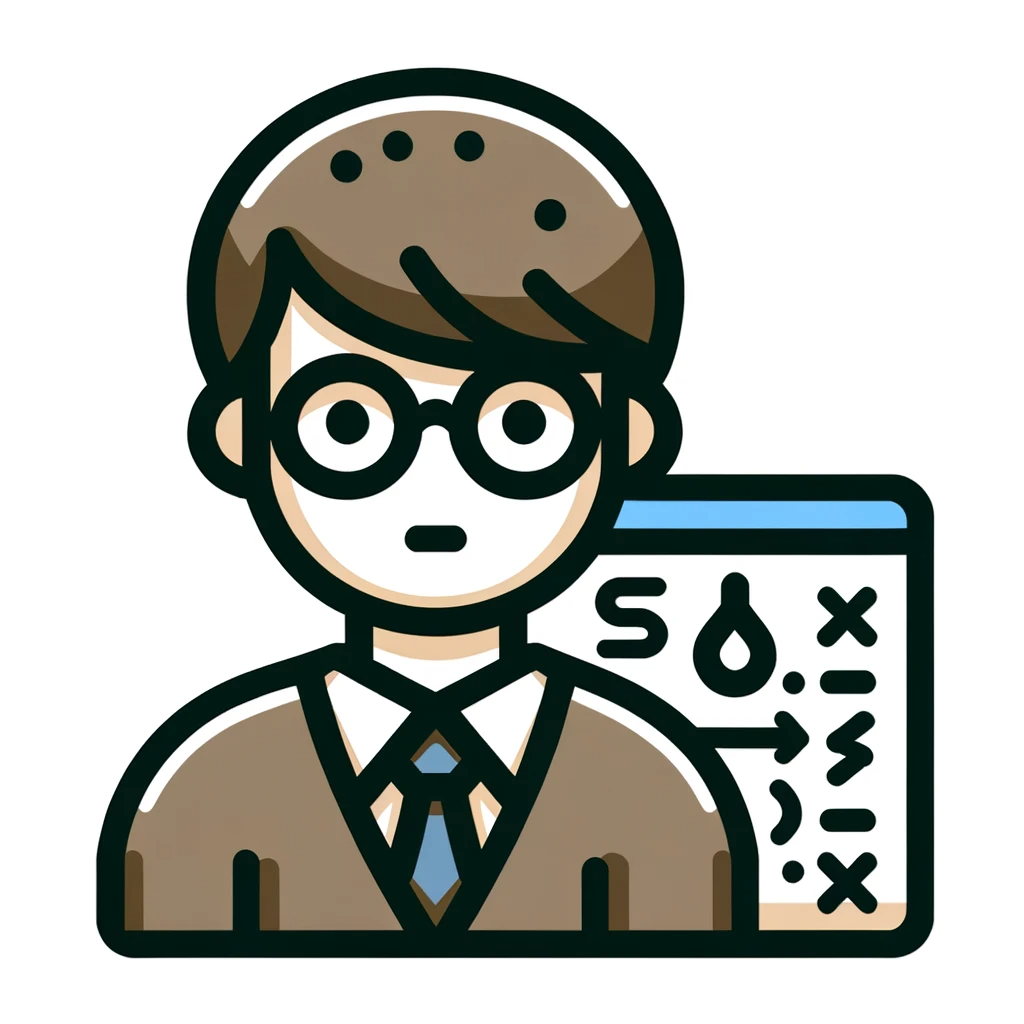
By using pseudo-classes, you can switch styles depending on the result of form validation without using JavaScript.
There are many ways to validate your forms, but using JavaScript is the most flexible method, allowing you to set custom error messages, implement dynamic validation, and more.
However, validation on the client side does not necessarily guarantee security, so it is important to check input values on the server side as well.
Comments