This article explains how to use JavaScript’s “getElementById” to quickly retrieve an HTML element using its id value as a key.
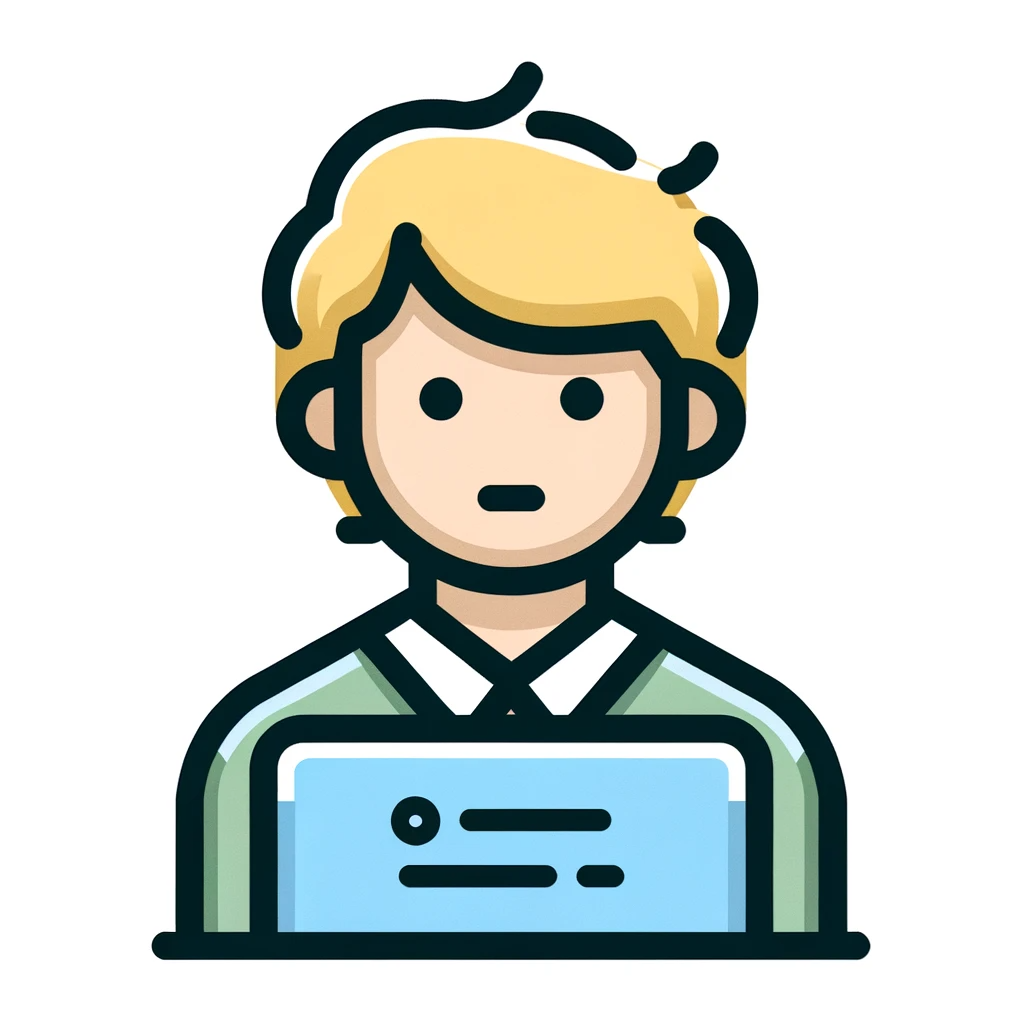
Is it possible to get an HTML element by its id value?
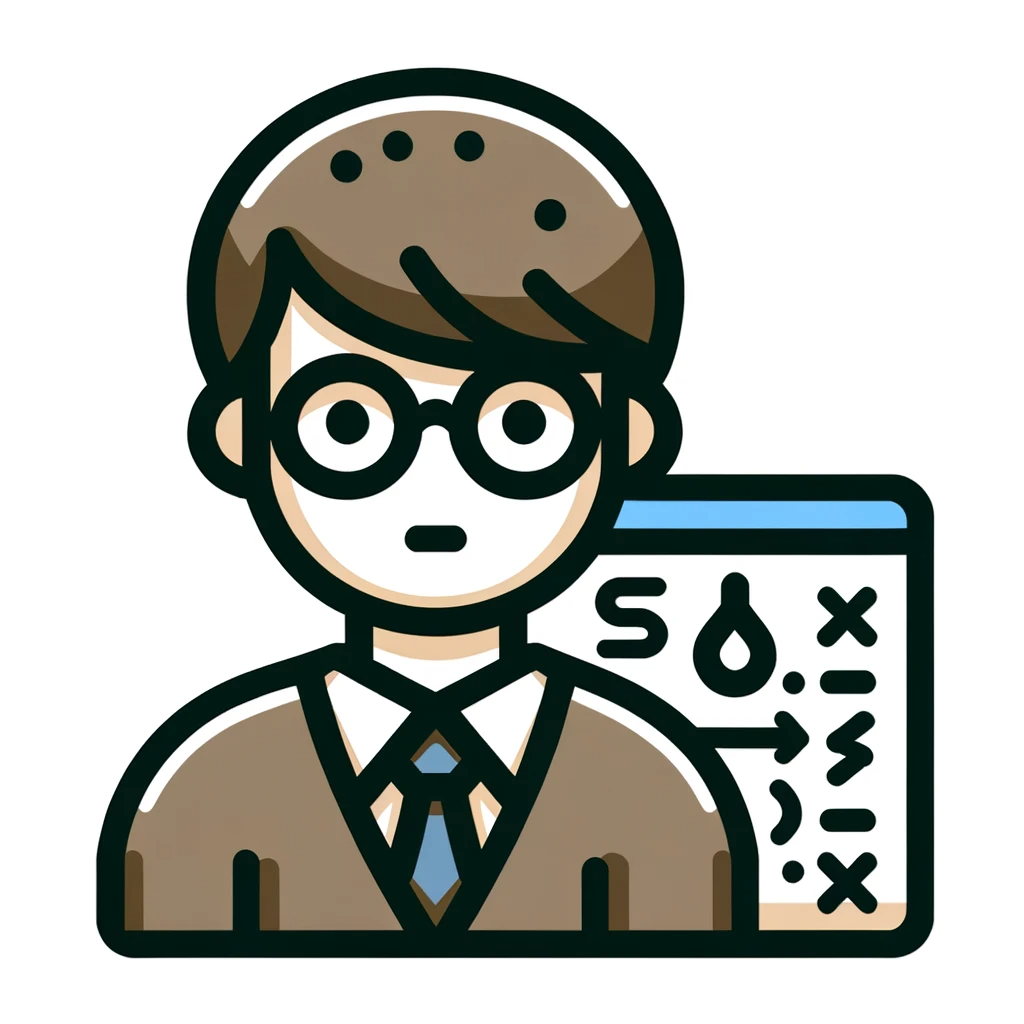
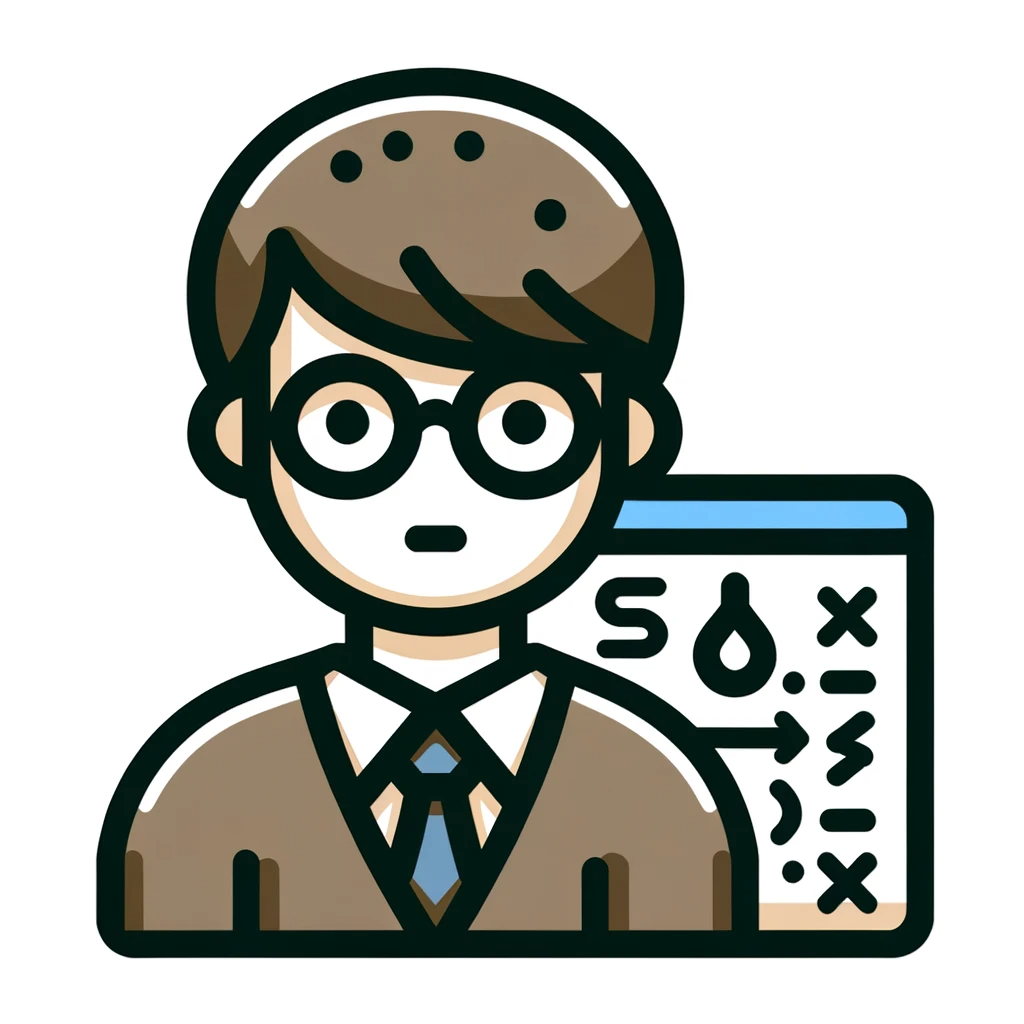
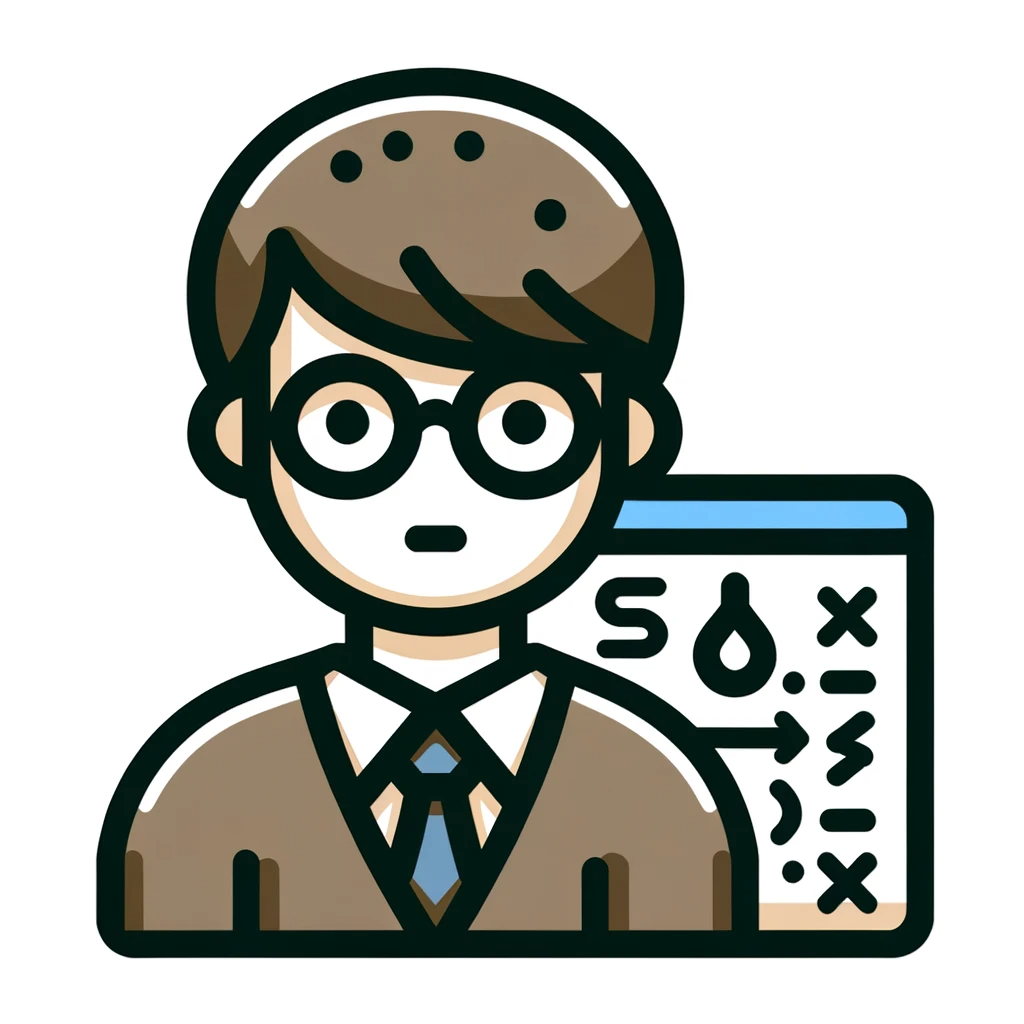
If you use “getElementById”, you can quickly retrieve an HTML element using the id value as a key.
How to use getElementById
” getElementById ” is a method to search and retrieve a specific element from an HTML document. This method searches for an element in an HTML document using the specified “id” attribute value. The first element found as a search result is the return value.
By using this method, you can manipulate elements with id values defined in HTML from JavaScript. To use “getElementById”, write as follows.
var element = document.getElementById(“id name”);
For “id name”, specify the id value defined in HTML. This allows you to retrieve the element whose id value is “id name”.
Additionally, the getElementById method returns null if the specified ID value is not found.
You can see how “getElementById” is used by looking at the following sample program.
<div id="target">Text will appear here.</div>
const target = document.getElementById("target");
target.innerHTML = "New Text";
In the above sample, we searched for and retrieved a “div” element with an “id” attribute value of “target”. The new text is then set in the “innerHTML” property of the retrieved element.
“getElementById” assumes that the “id” attribute value is unique. If there are duplicate “id” attribute values, only the first element found will be retrieved. Therefore, care must be taken to set the “id” attribute value to be unique.
This updates the text displayed in the “div” element with an “id” attribute value of “target” in the HTML document.
Sample program using getElementById
A sample program using “getElementById” is shown below. When the button is clicked, the string is assigned to the retrieved element.
<!DOCTYPE html>
<html>
<head>
<script src="test.js"></script>
</head>
<body>
<div id="myDiv">Hello!</div>
<button id="myButton">Change Element</button>
</body>
</html>
// Get element
var div = document.getElementById("myDiv");
var button = document.getElementById("myButton");
// Processing when a button is clicked
button.addEventListener("click", function() {
div.innerHTML = "Hello, World!";
});
In this sample, the HTML has a div element with an id value of “myDiv” and a button element with an id value of “myButton”.
In JavaScript (test.js), we use “getElementById” to retrieve these elements and change the contents of “myDiv” when the button is clicked.
Summary of this article
We explained how to use “getElementById” to retrieve an HTML element using its id value as a key.
- The getElementById method allows you to retrieve the element with a specified ID value in an HTML document.
- The getElementById method returns null if the specified ID value is not found.
- The getElementById method allows you to easily retrieve and manipulate a specified element in an HTML document.
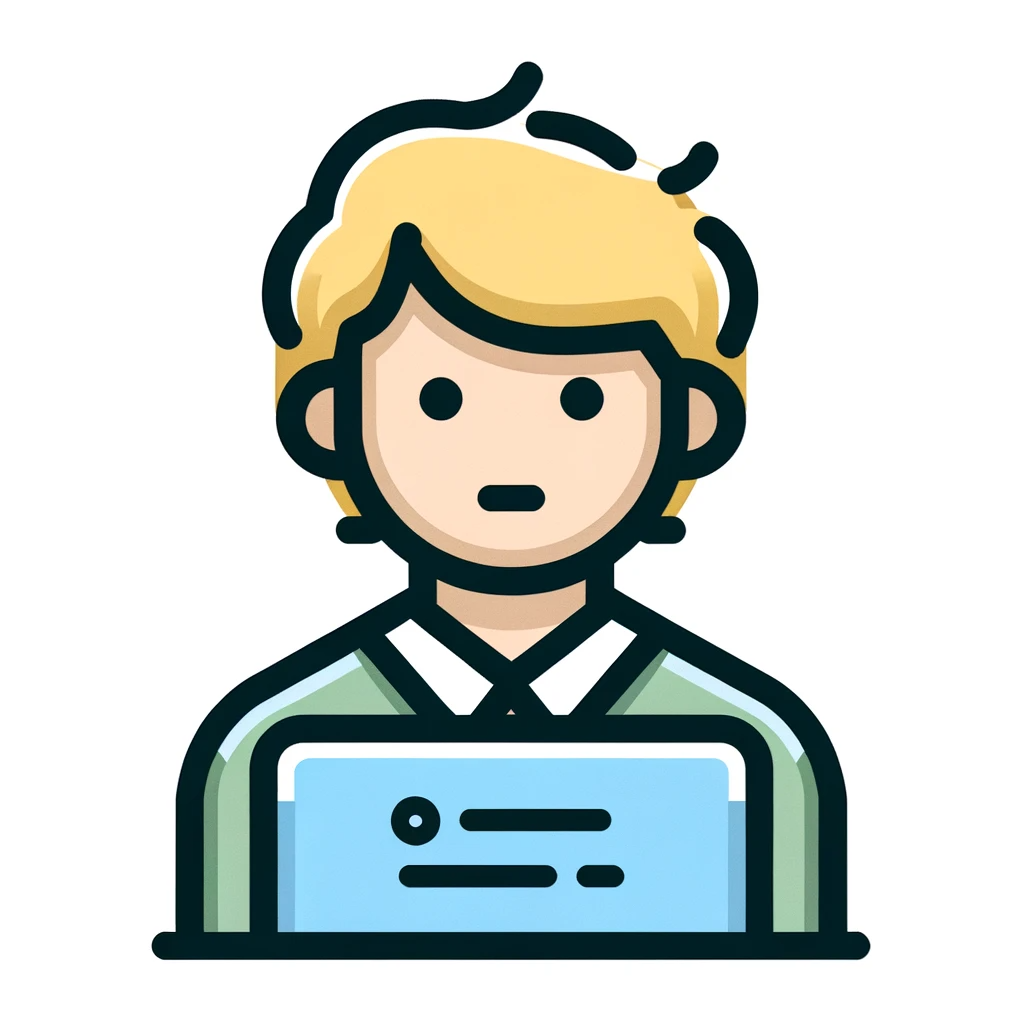
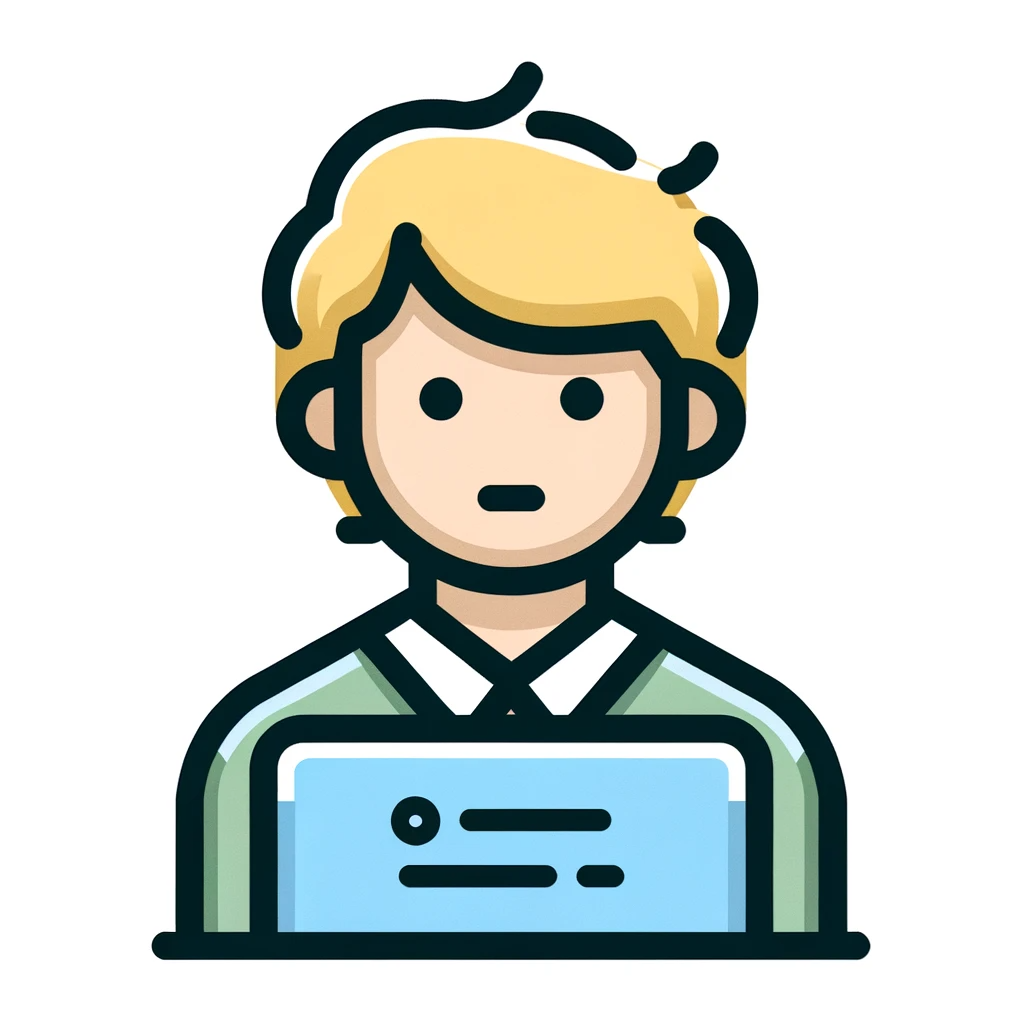
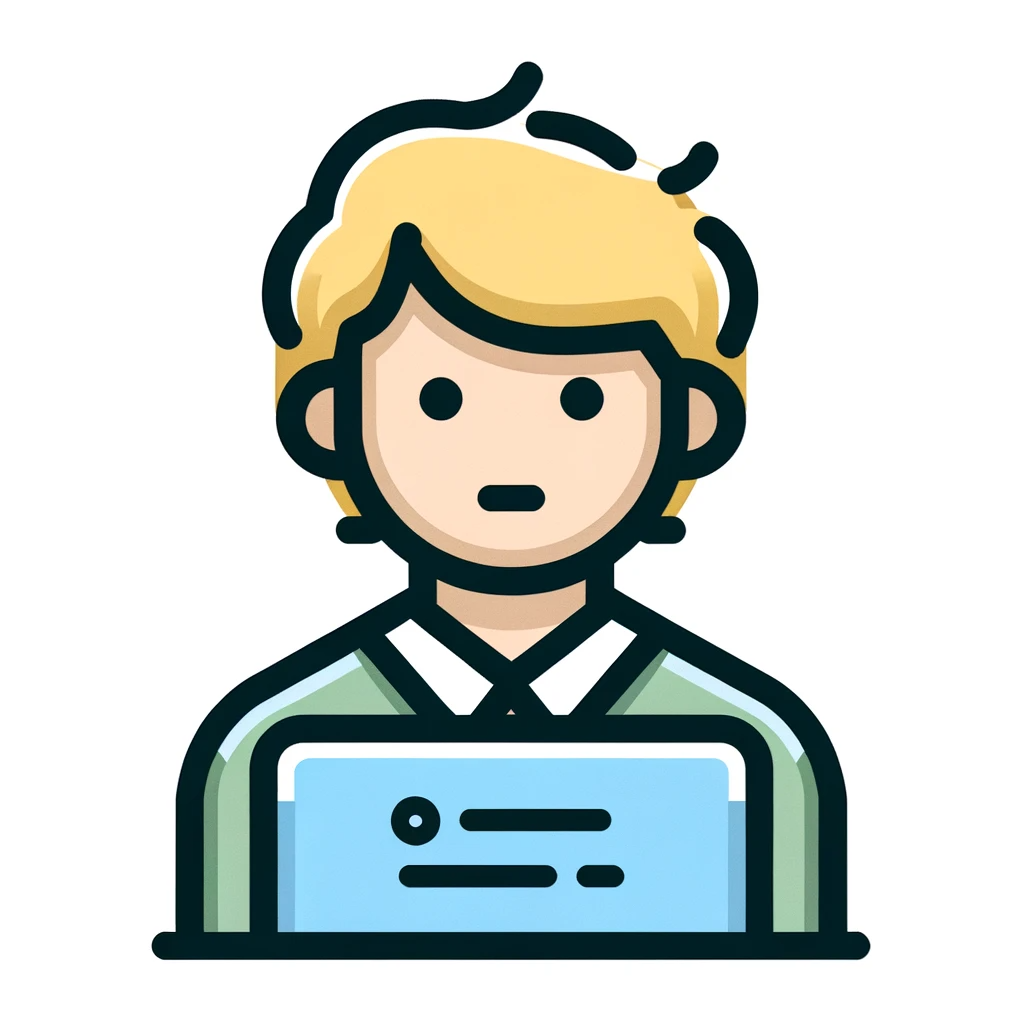
The getElementById method is a very useful method for manipulating HTML documents with JavaScript!
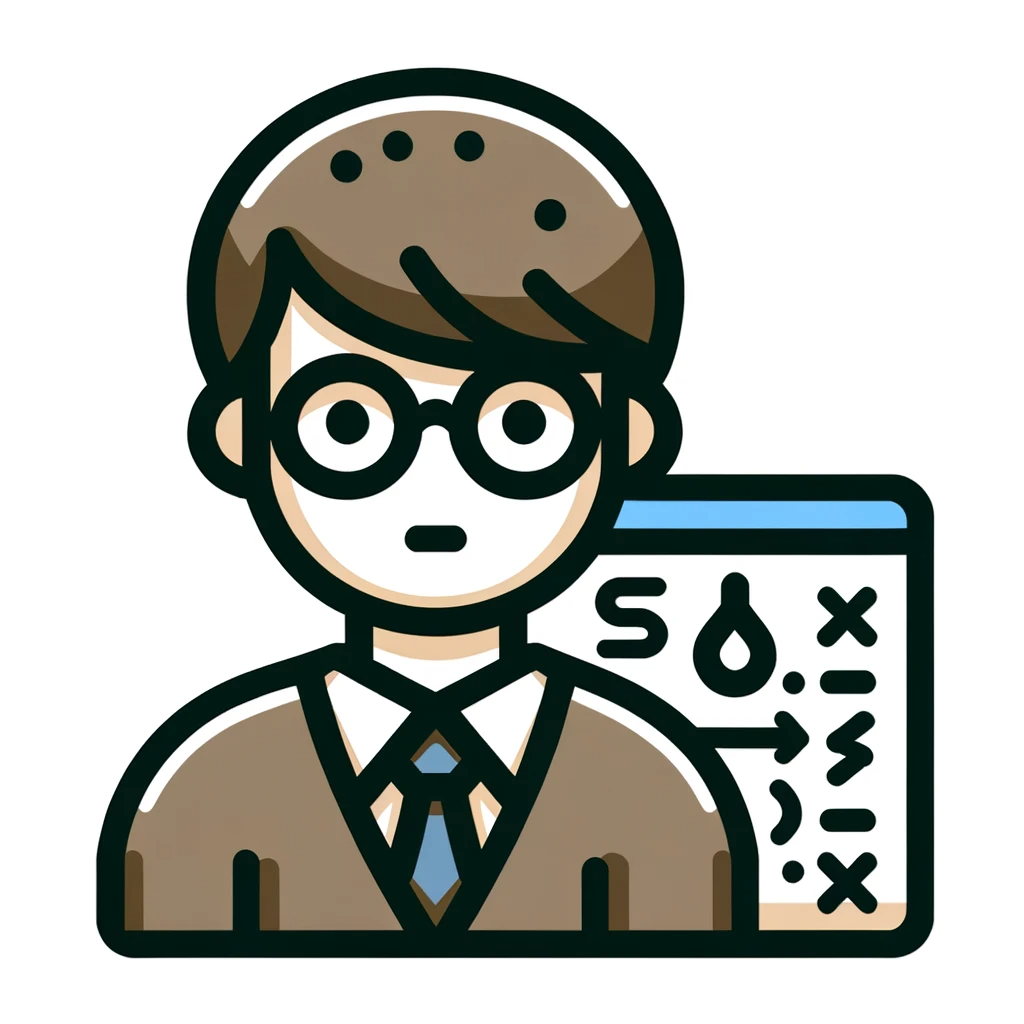
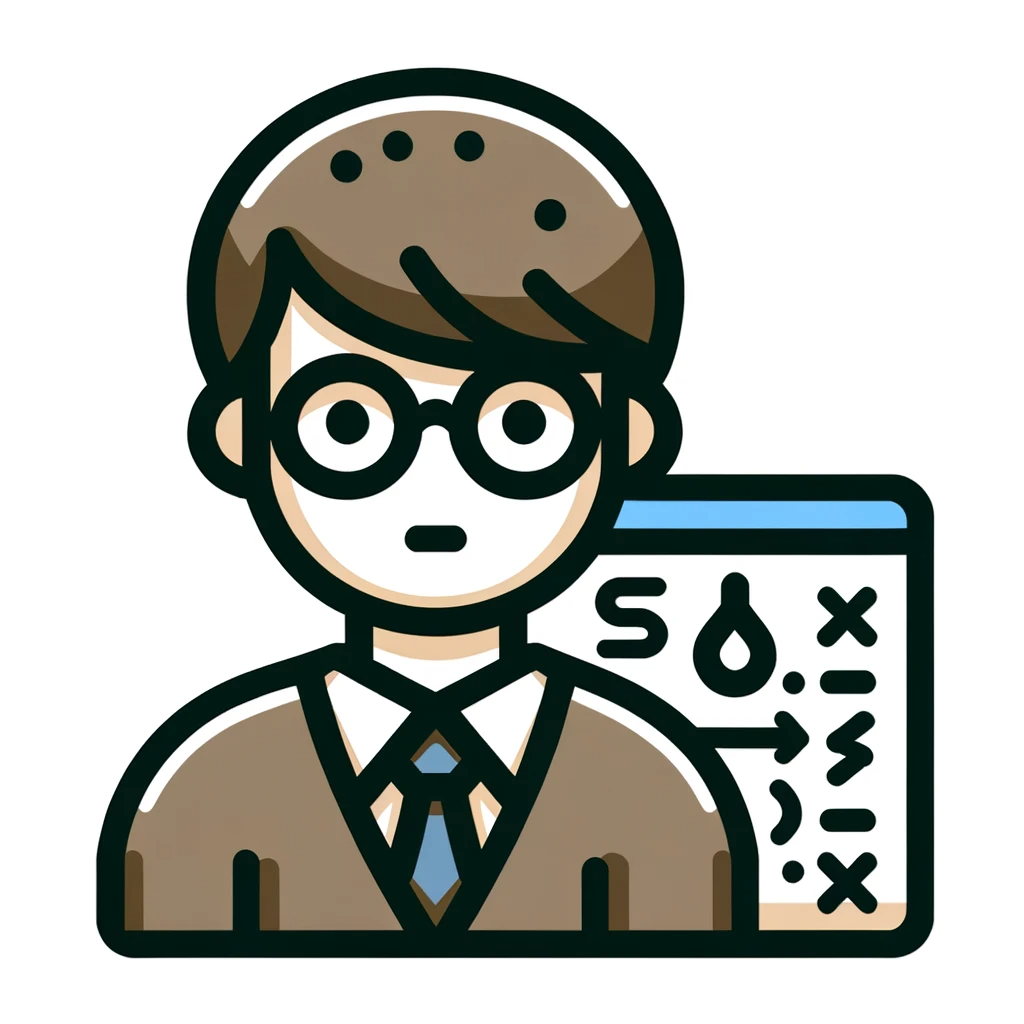
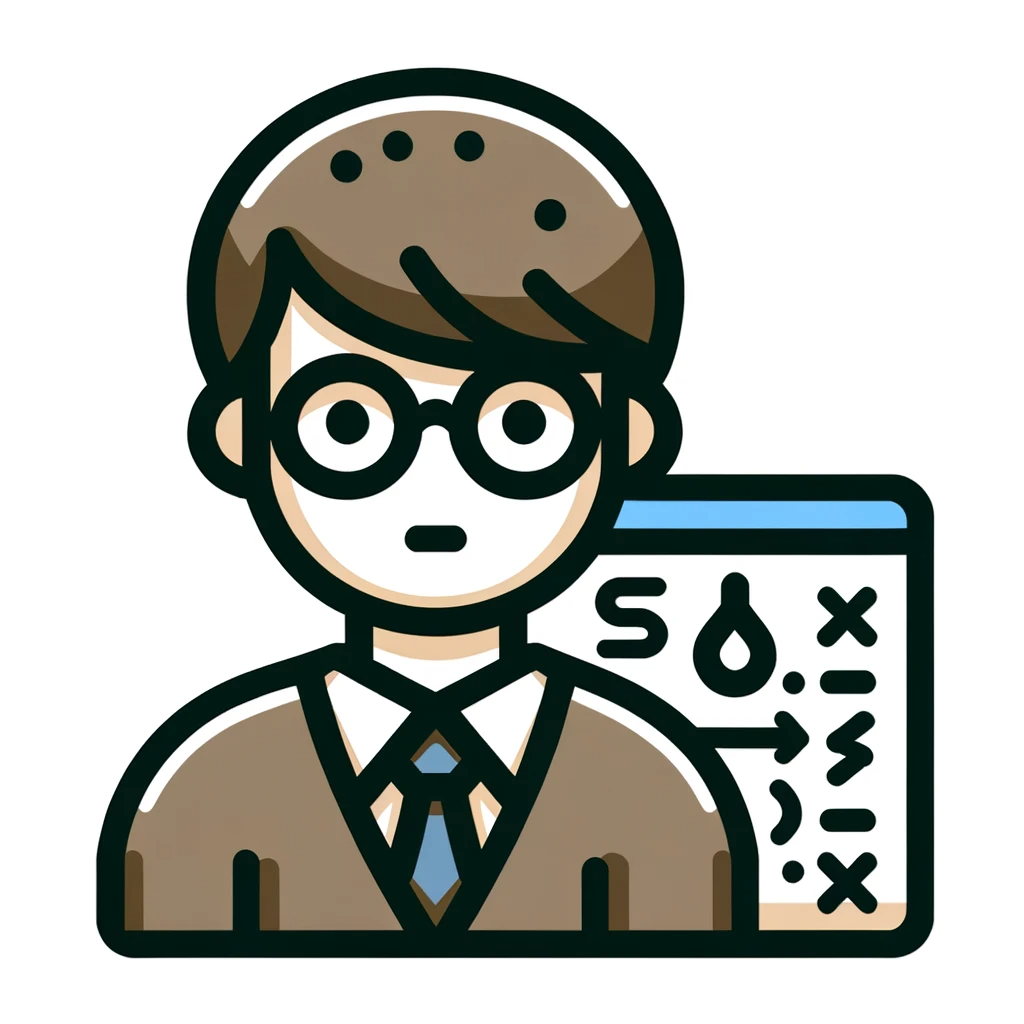
By using the getElementById method, you can easily manipulate elements in an HTML document.
Also, the ID value must be unique within the page, so please be sure to specify the correct ID value.
Comments