JavaScript’s Function object has call and apply methods, and we will explain the differences and benefits of these.
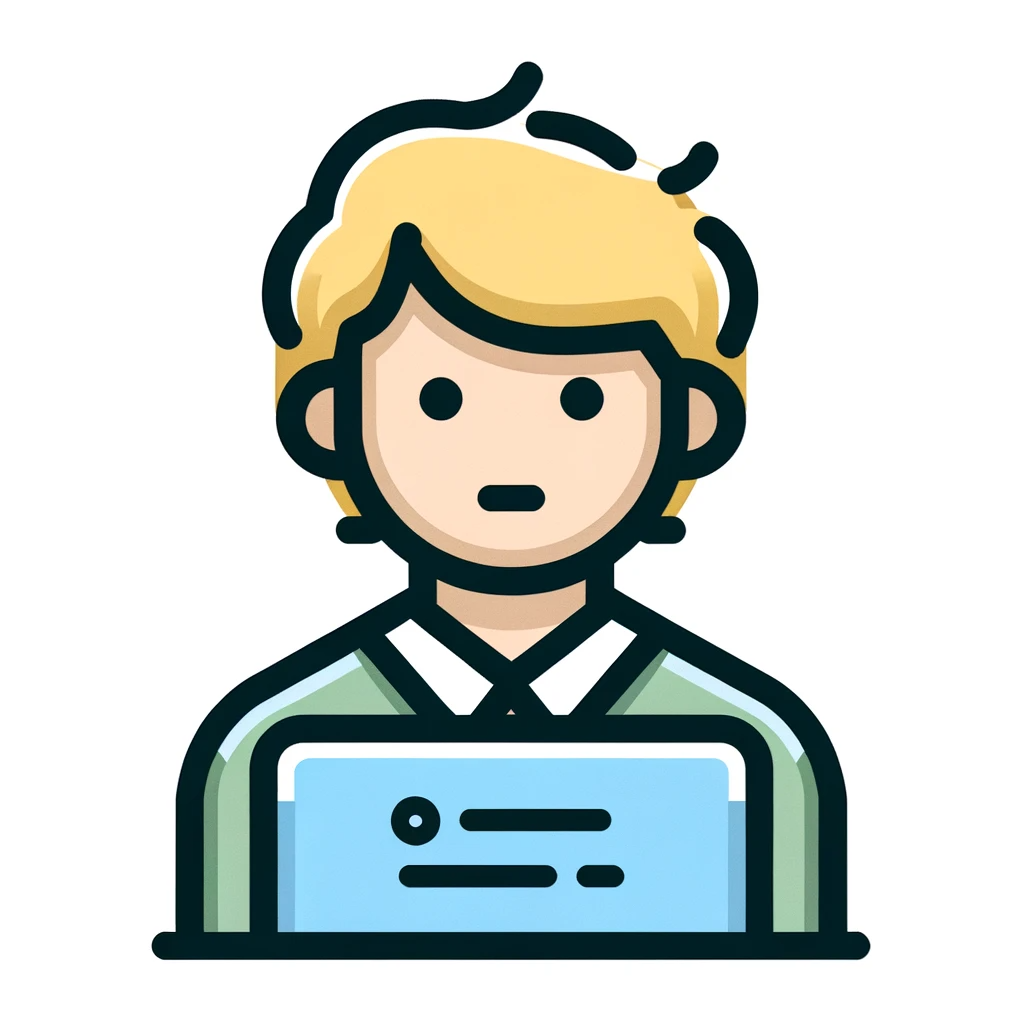
Function objects have call and apply methods, but how should they be used properly?
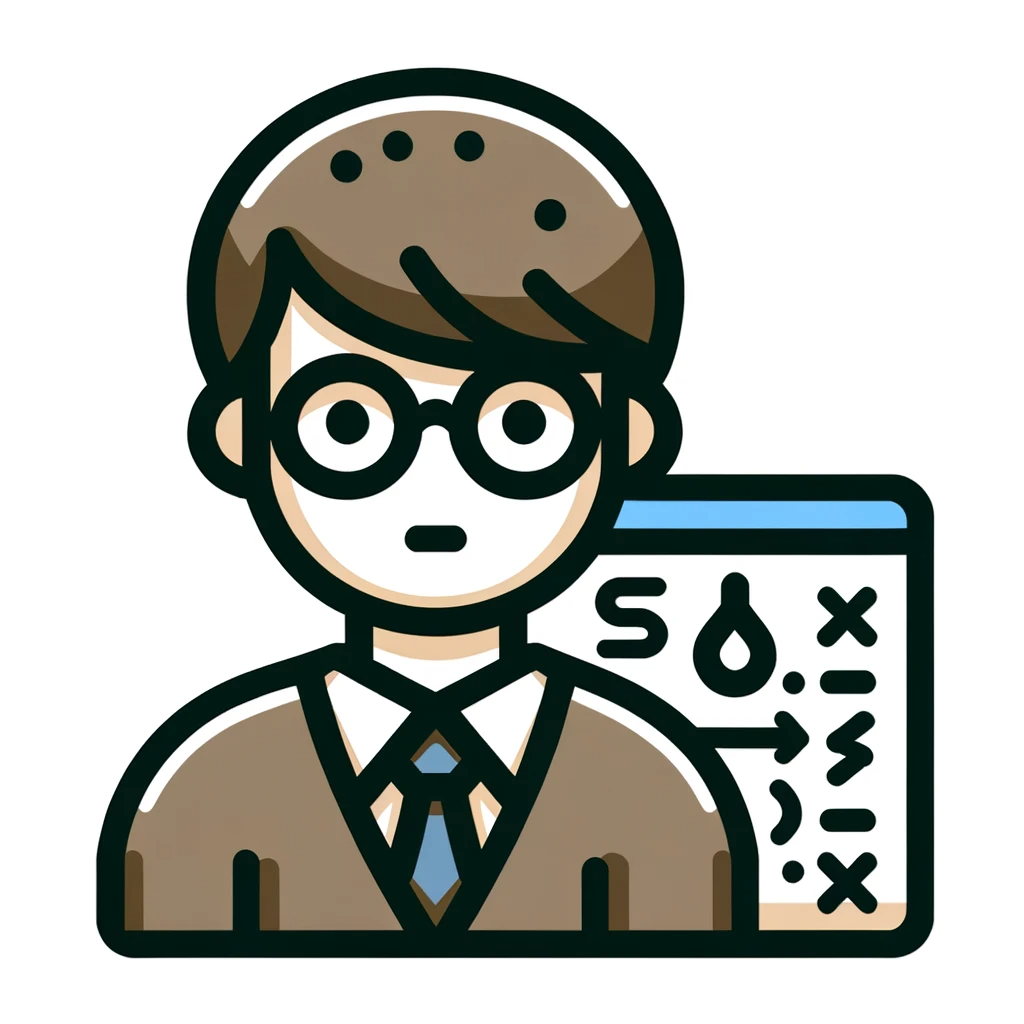
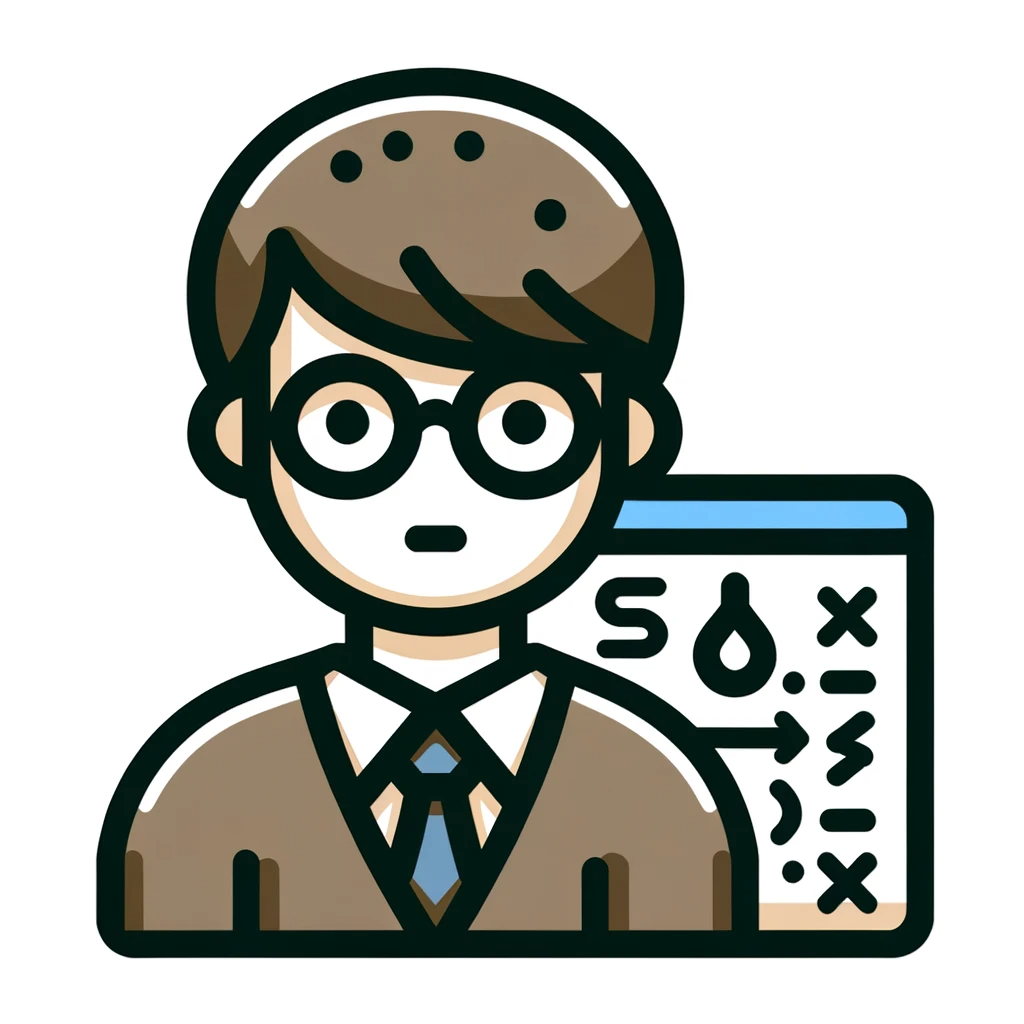
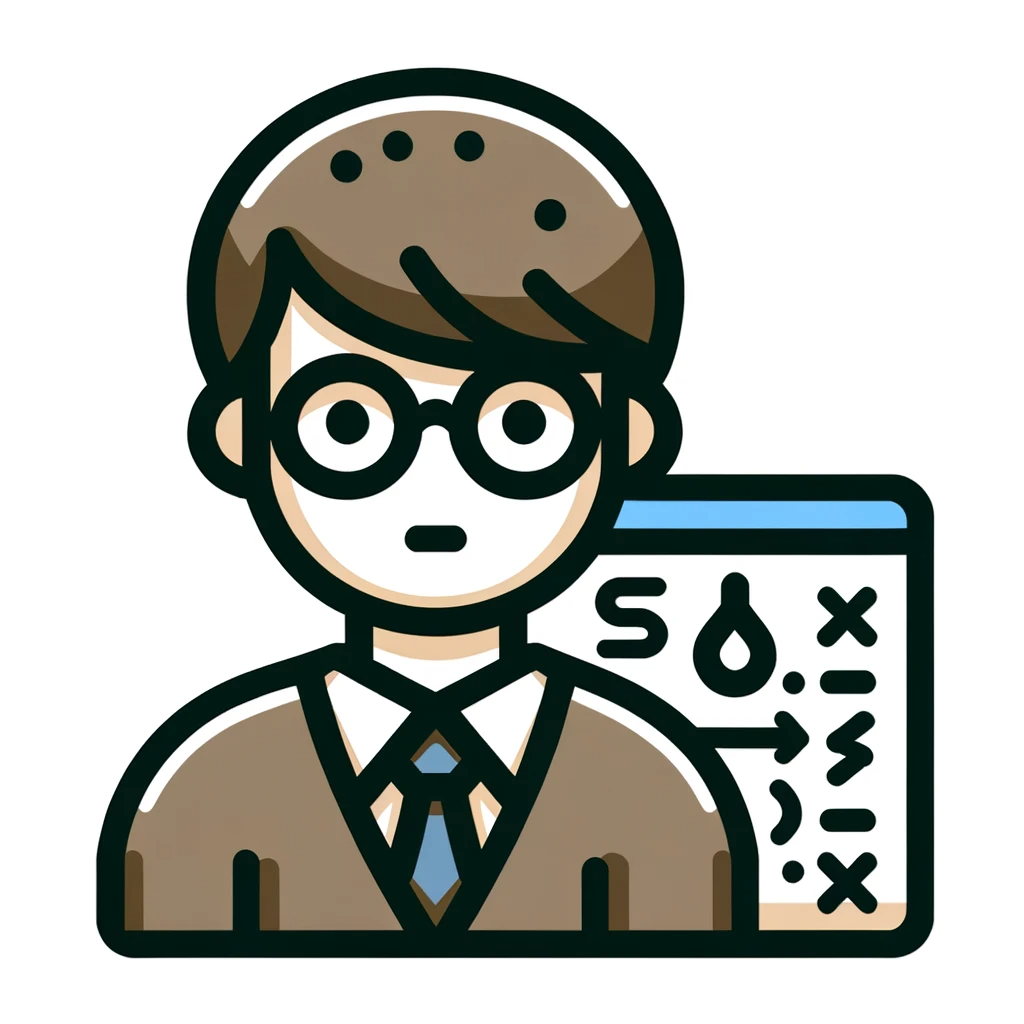
The call and apply methods have almost the same functionality, but they differ in how they pass arguments.
We will explain the differences and benefits.
How to use call and apply methods
When using the call method, write it like function name.call(this, argument 1, argument 2, …) . Also, when using the apply method, write function name.apply(this, [argument 1, argument 2, …]).
These methods allow you to execute any function in the context of any object.
Difference between call method and apply method
The difference between the call method and the apply method is how to write the arguments passed after the second argument.
The call method passes arguments individually, but the apply method passes them in an array format. This has the advantage that when using the apply method, you can change the number of arguments by changing the number of arrays.
Below is a sample of the call method and apply method.
let obj1 = {name: 'John'};
let obj2 = {name: 'Jane'};
function sayName() {
console.log(this.name);
}
sayName.call(obj1); // John
sayName.call(obj2); // Jane
let obj1 = {name: 'John'};
let obj2 = {name: 'Jane'};
function sayName() {
console.log(this.name);
}
sayName.apply(obj1, []); // John
sayName.apply(obj2, []); // Jane
Advantages of call method and apply method
Both methods allow you to specify a this value , which allows the function to be executed in the context of another object.
You can also use the same function to access properties of different objects.
Summary of this article
We explained the differences and advantages of the call and apply methods.
- The call method can pass arguments individually.
- The apply method can pass arguments in the form of an array.
- The call and apply methods can specify a this value.
- A function can be executed in the context of another object.
- You can also use the same function to access properties of different objects.
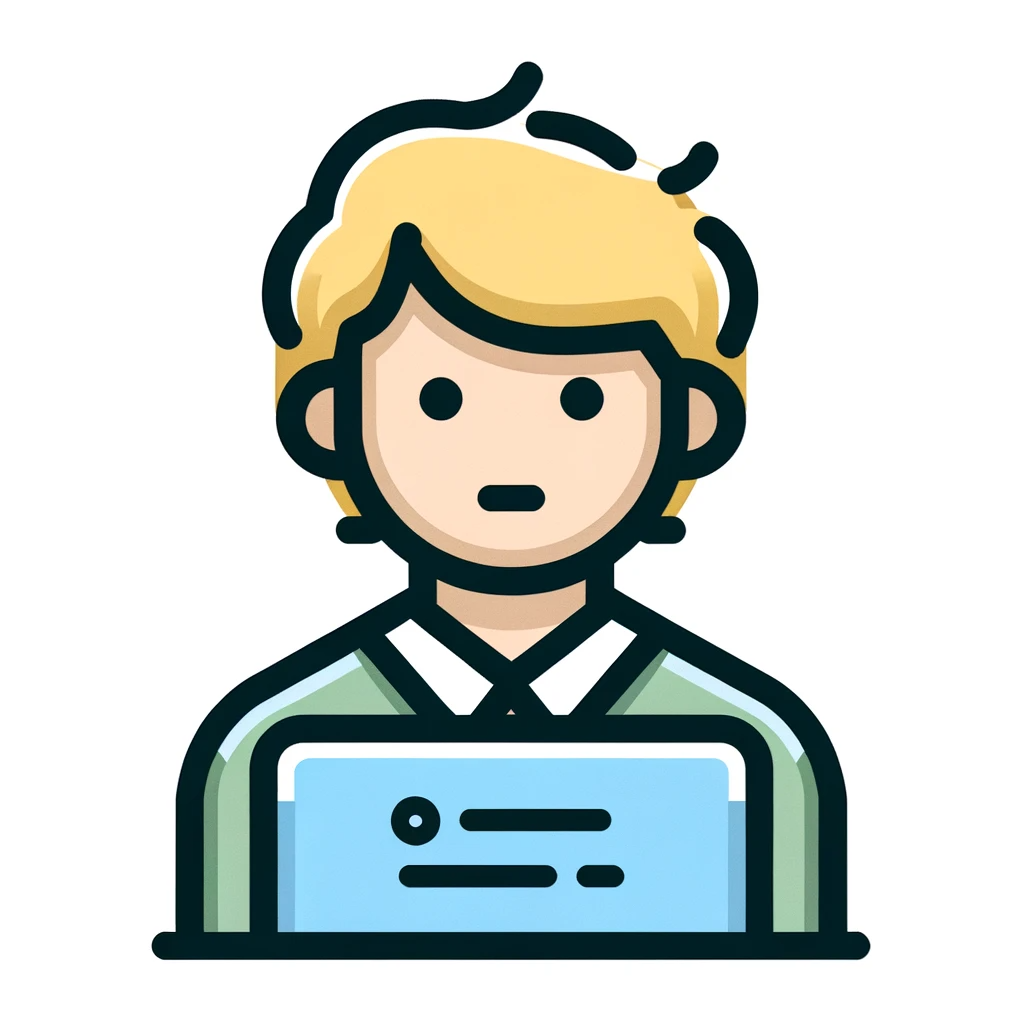
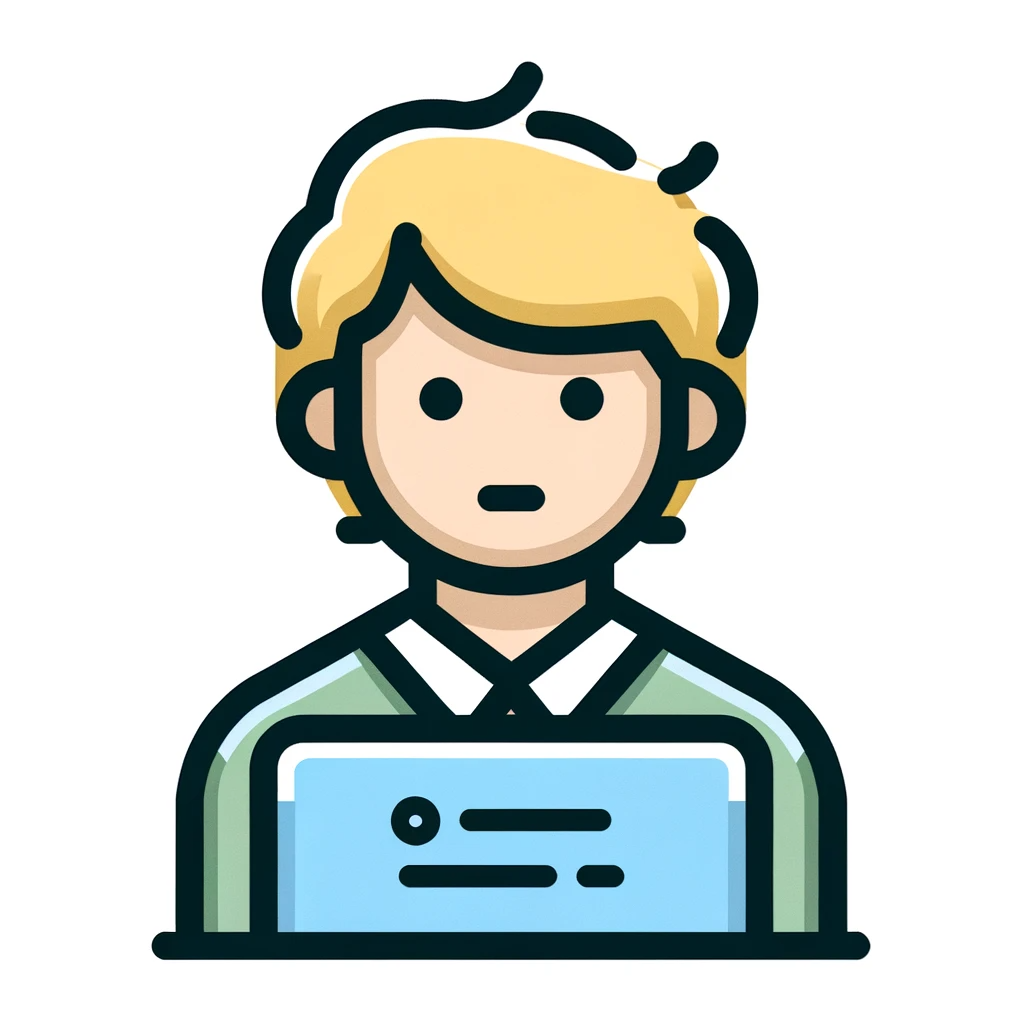
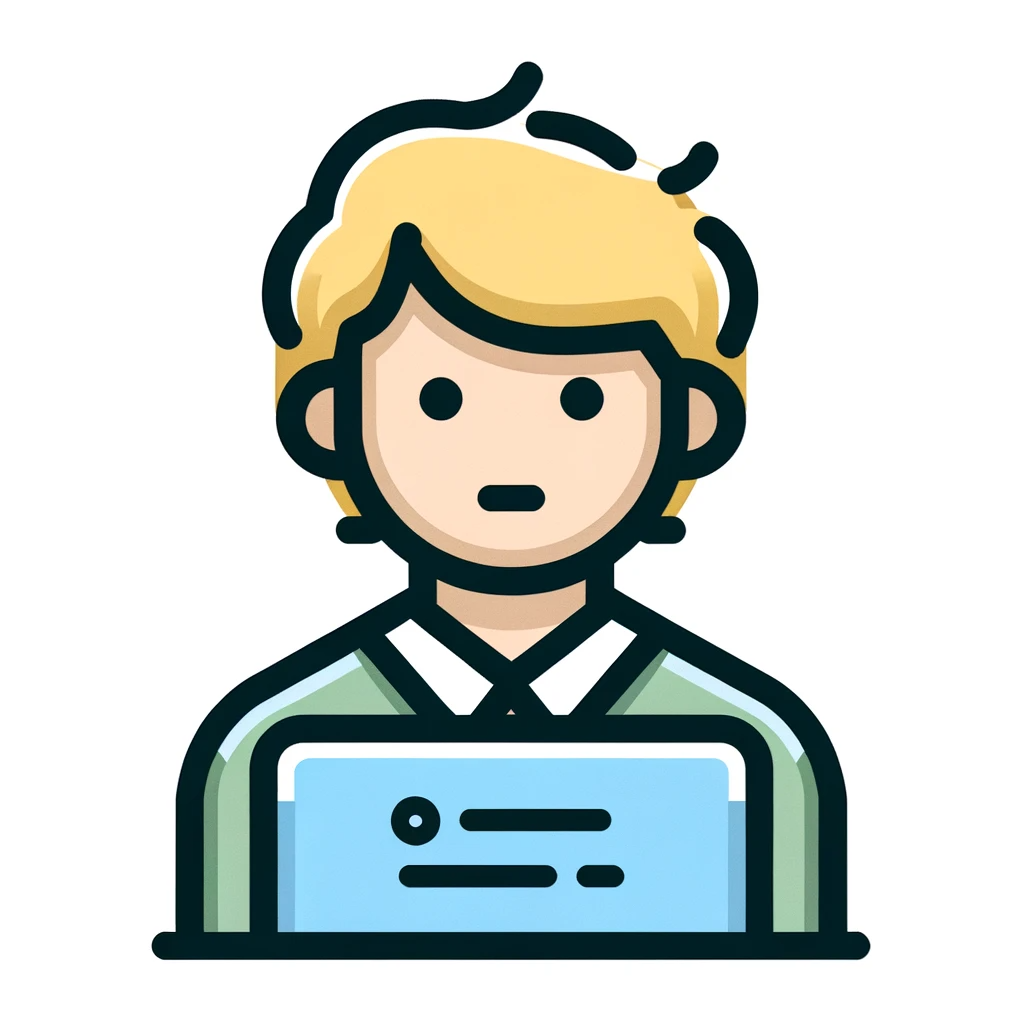
The call method and apply method are very useful methods.
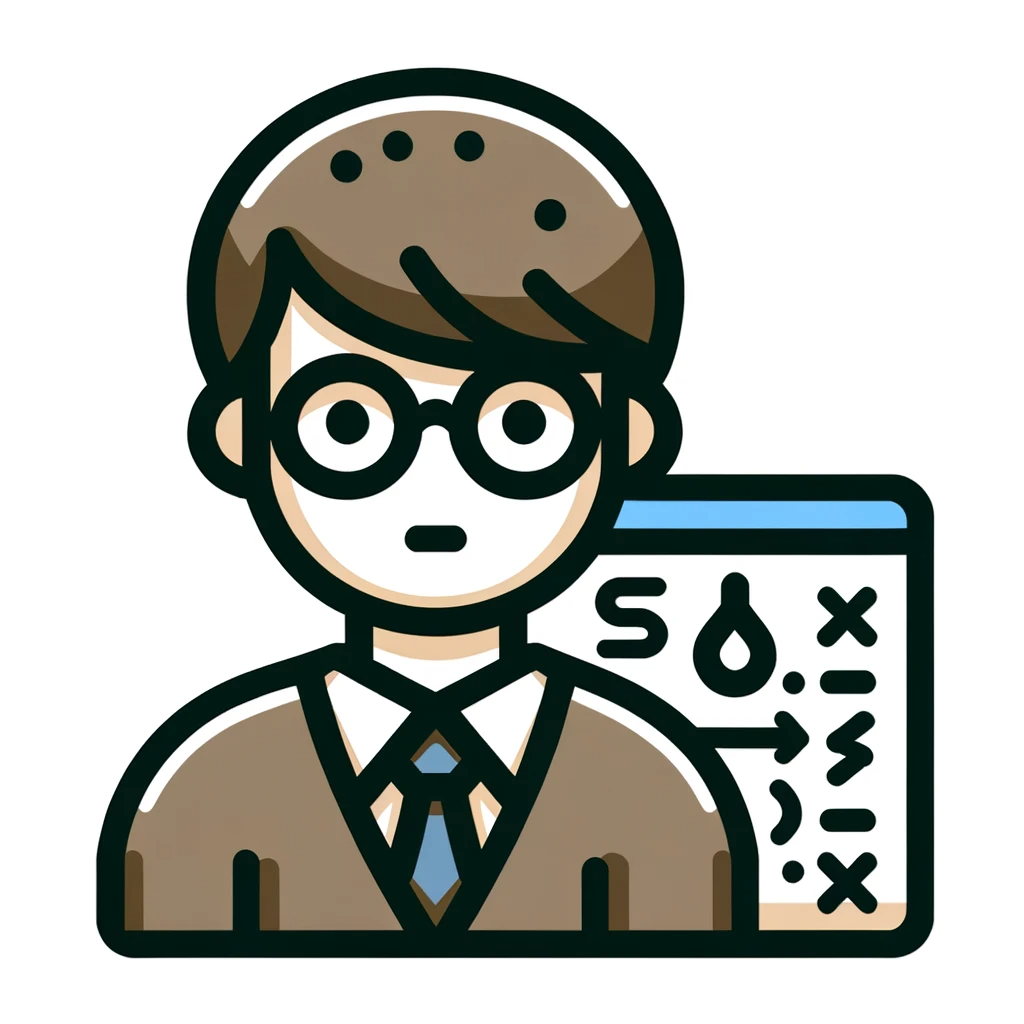
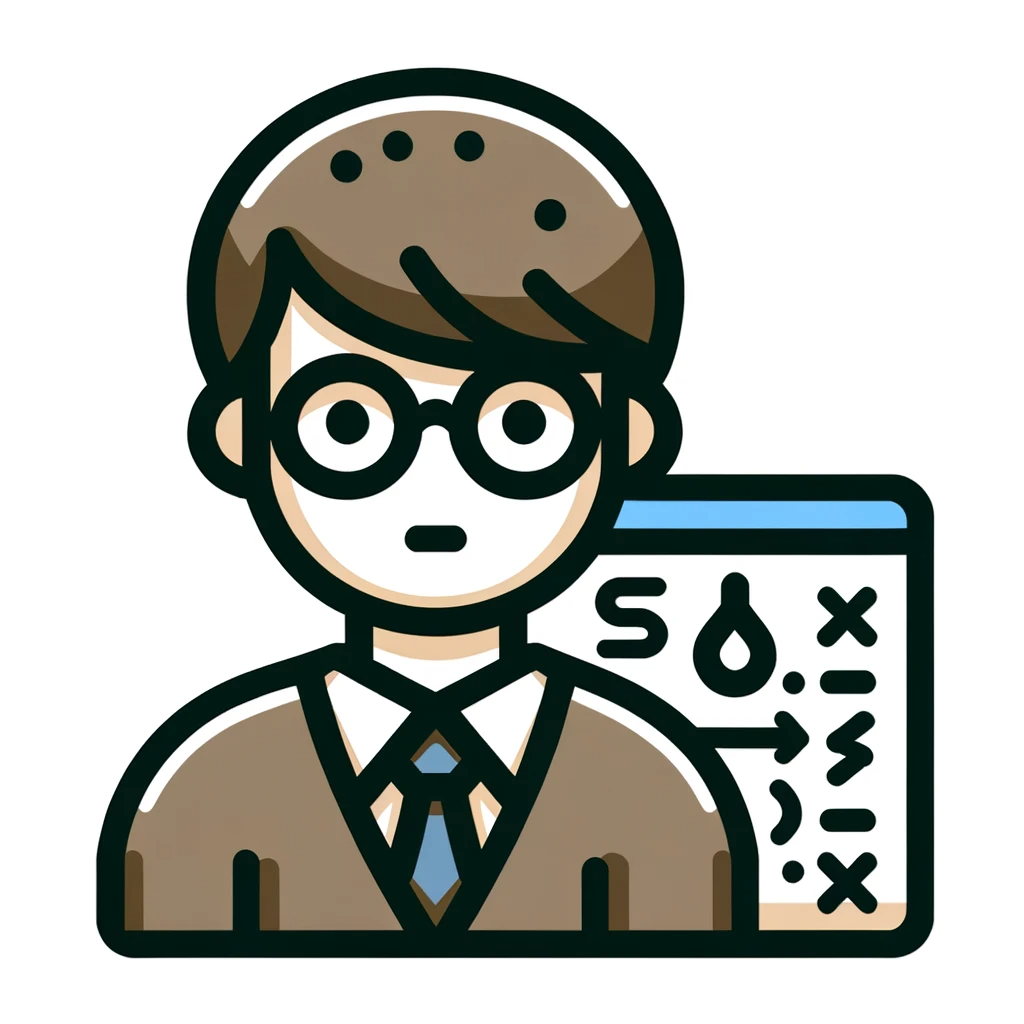
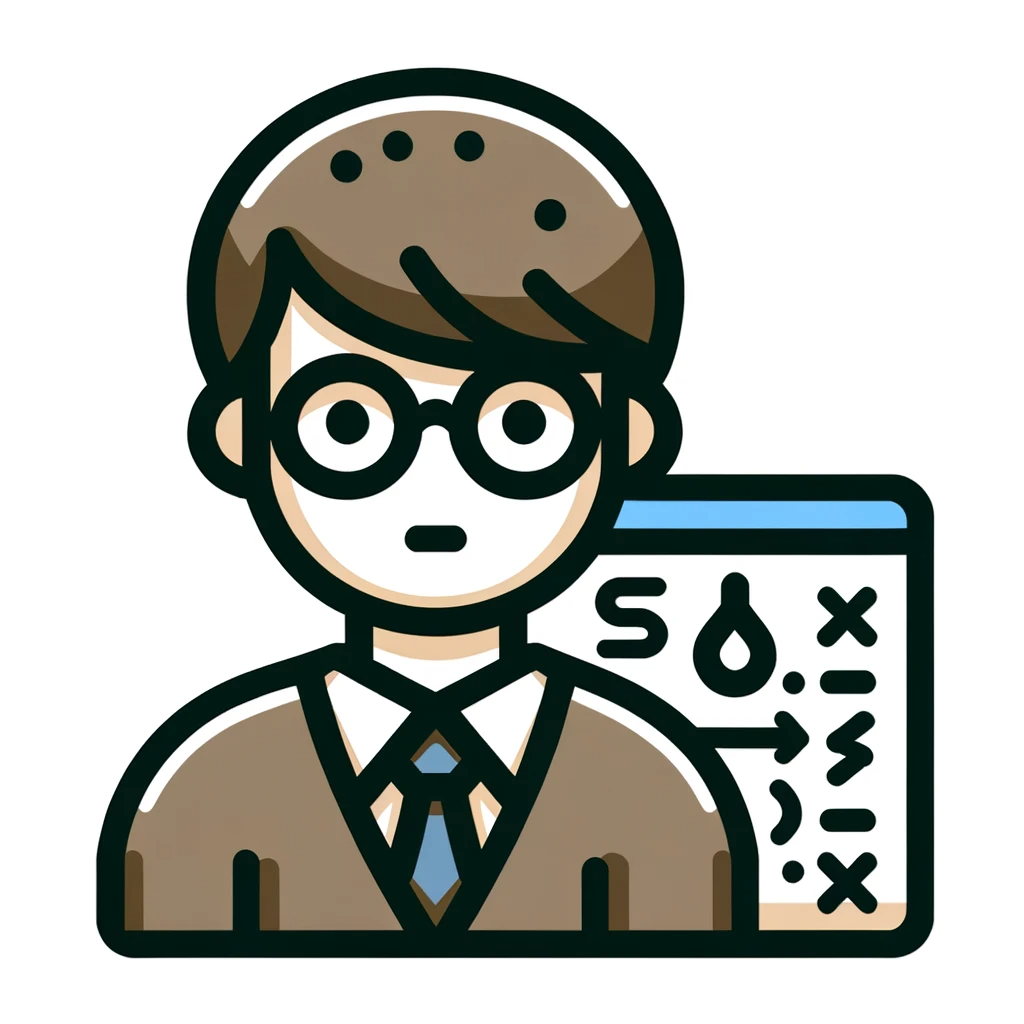
By using the call and apply methods, you can execute any function in the context of any object.
It is best to use them differently depending on the format of the argument.
Comments