This article explains how to determine whether a specified element exists in an array using JavaScript.
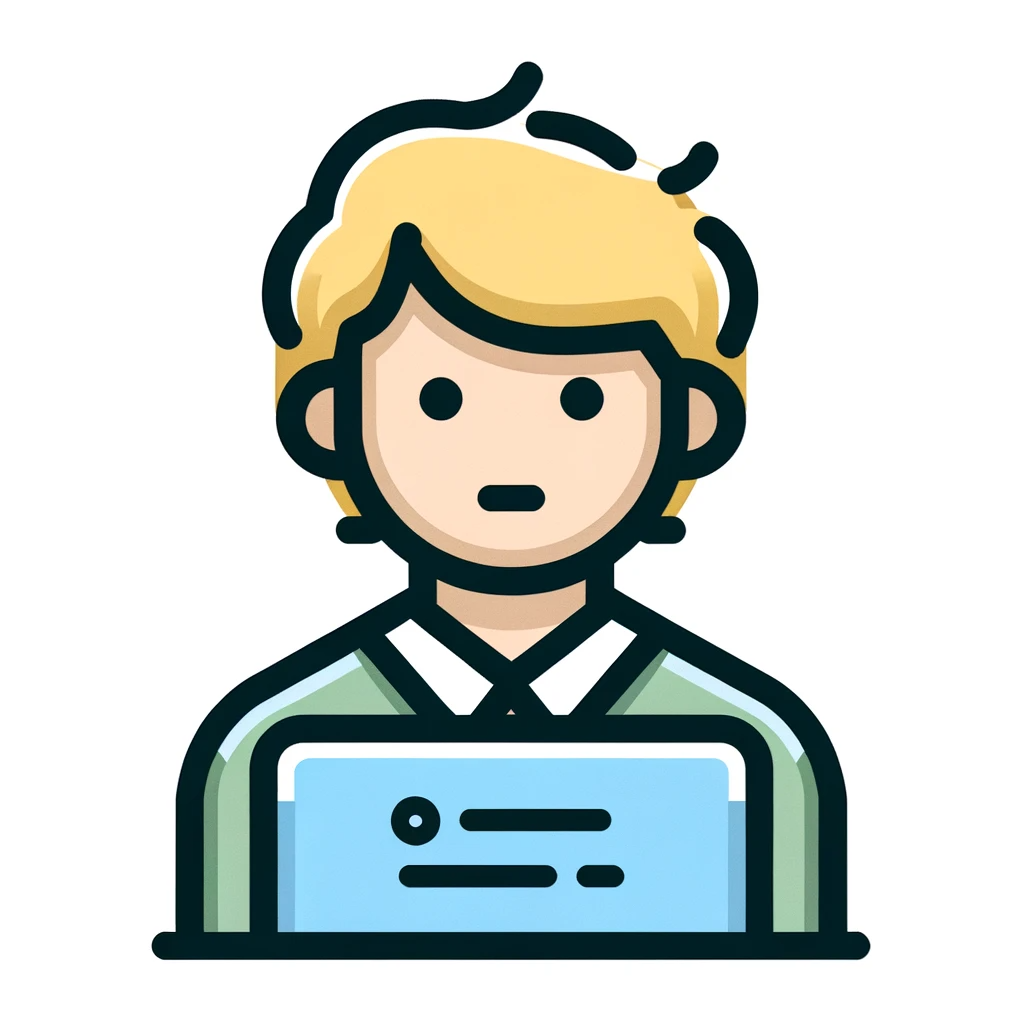
Is there a way to determine whether a specified element exists in an array using JavaScript?
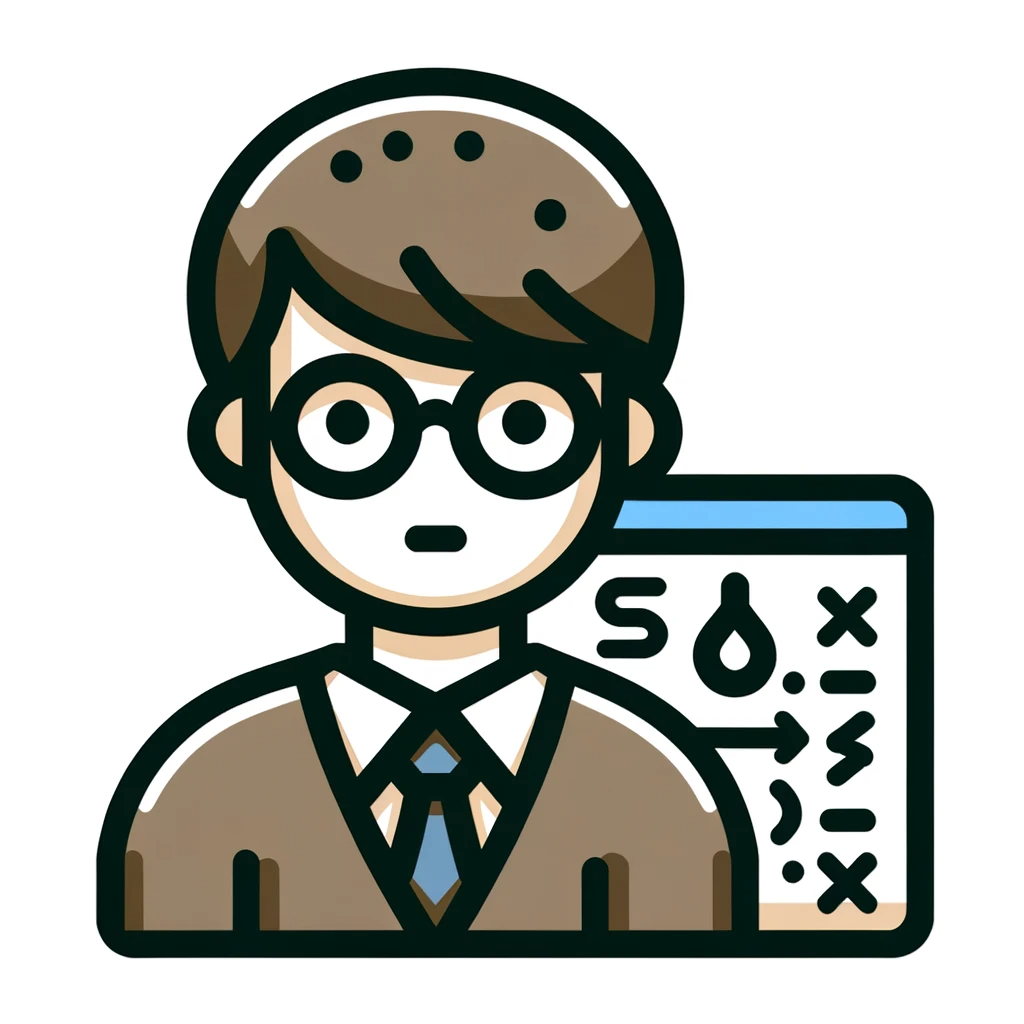
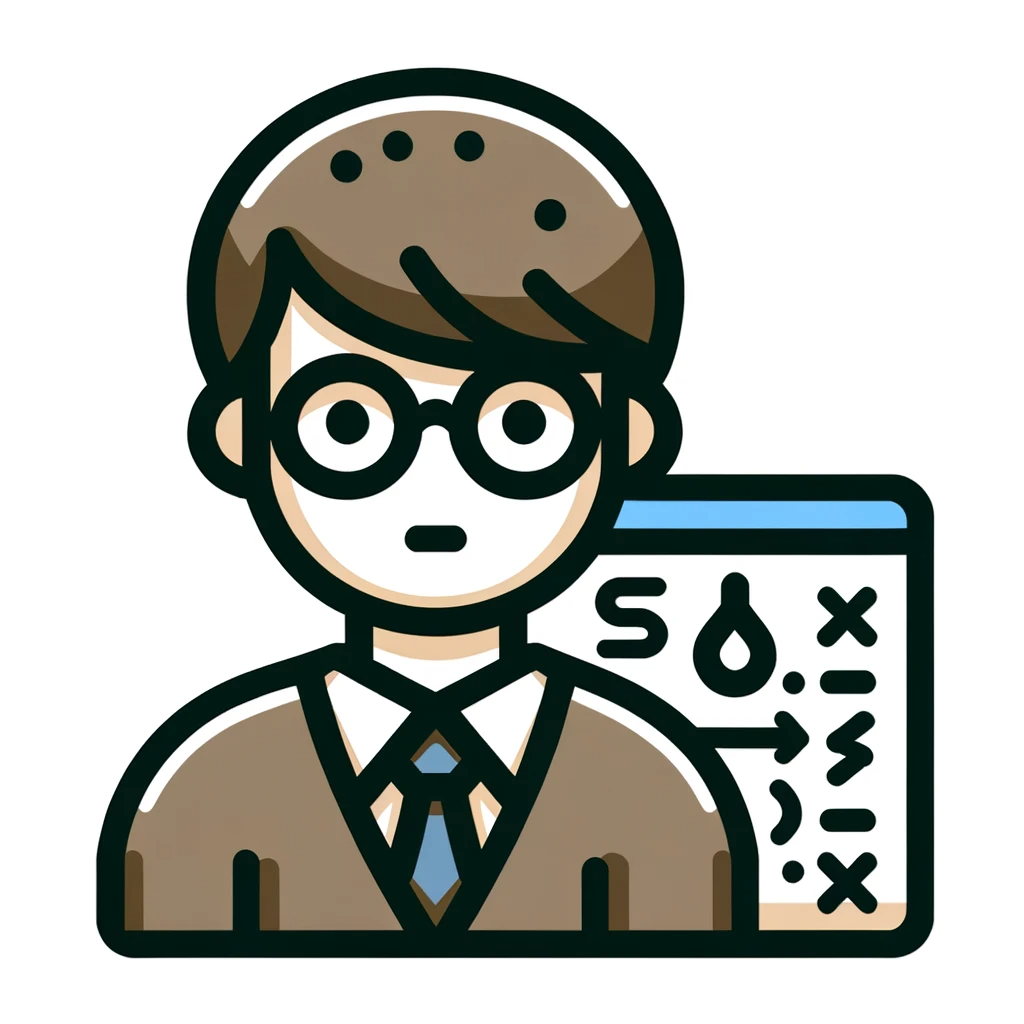
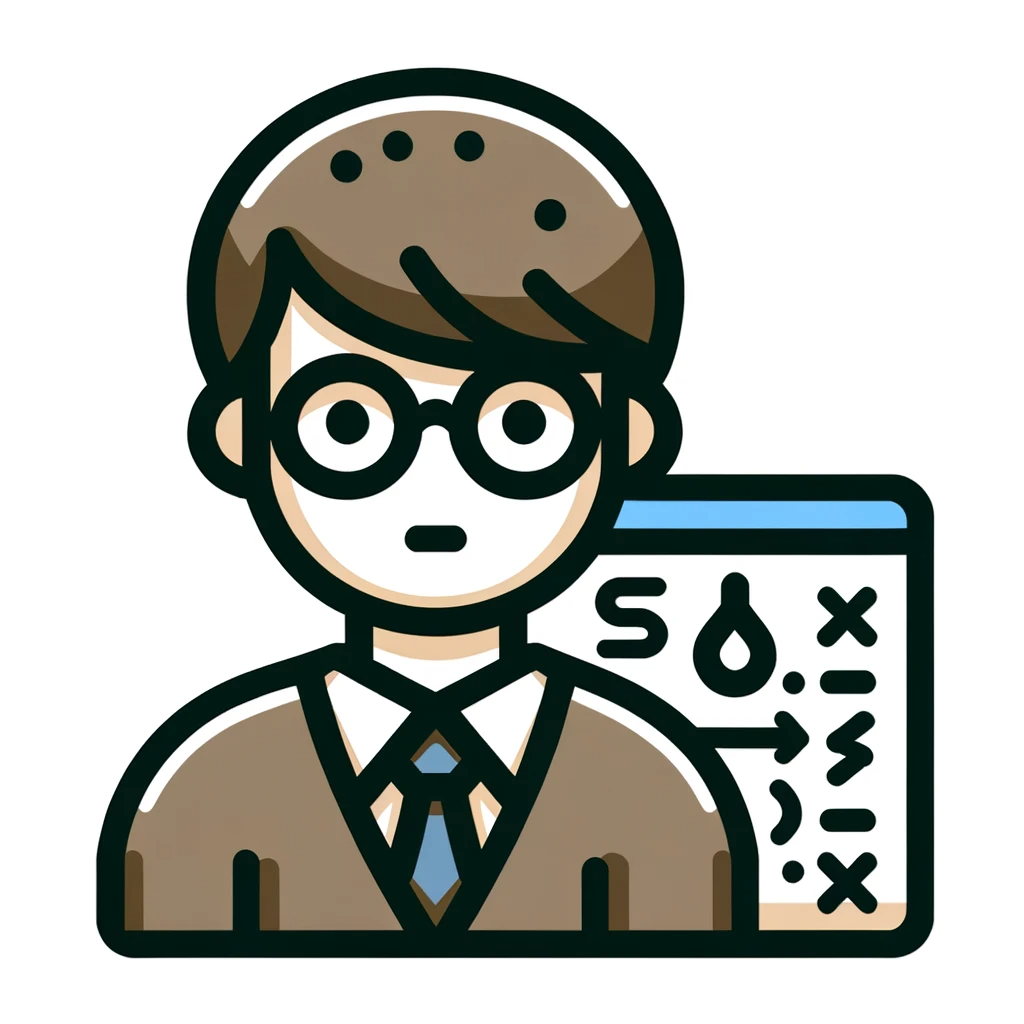
There are several methods to determine whether a specified element exists in an array, such as the includes method, find method, and indexOf method.
How to determine the existence of an element using the includes method
The includes method returns true if the specified element is present in the array, false otherwise.
const array = [1, 2, 3, 4, 5];
const element = 3;
const result = array.includes(element);
console.log(result); // true
In the above sample, array.includes(element) returns true because array array contains 3. And true is stored in result.
How to determine the existence of an element using the find method
The find method returns an element that satisfies the specified condition in the array if it exists, otherwise returns undefined.
const array = [1, 2, 3, 4, 5];
const element = 3;
const result = array.find(e => e === element);
console.log(result); // 3
In the above sample, array array contains 3, so array.find(e => e === element) returns 3. And 3 is stored in result.
How to determine the existence of an element using the indexOf method
The indexOf method returns the index number of the specified element in the array if it exists, and -1 if it does not.
const array = [1, 2, 3, 4, 5];
const element = 3;
const result = array.indexOf(element) !== -1;
console.log(result); // true
In the sample above, array array contains 3, so array.indexOf(element) returns 2. And 2 !== -1 is true. Therefore, true is stored in result.
How to determine the existence of a method element using a for statement
The for statement method iterates through the array and returns true if the specified element exists, false otherwise.
const array = [1, 2, 3, 4, 5];
const element = 3;
let result = false;
for (let i = 0; i < array.length; i++) {
if (array[i] === element) {
result = true;
break;
}
}
console.log(result); // true
In the above sample, the array array contains 3, so 3 and array[i] match, so true is stored in result and the for statement ends with break. Eventually console.log(result) returns true.
Summary
This is a summary of “How to determine whether a specified element exists in an array.”
- indexOf method: Returns the index number if the specified element exists in the array, otherwise returns -1.
- includes method: Returns true if the specified element exists in the array, otherwise returns false.
- List find method: Returns the specified element if it exists in the array, otherwise returns undefined.
- Method using for statement: Iterate through the array and return true if the specified element exists, otherwise return false.
We introduced a method to determine whether a specified element exists in an array using JavaScript. The best method to use depends on the program. Choose the appropriate method by referring to the methods introduced in this article.
Note that the includes method was introduced from ES2016, so it is not available in older browsers.
In that case, we recommend using the indexOf method or a for statement.
Comments